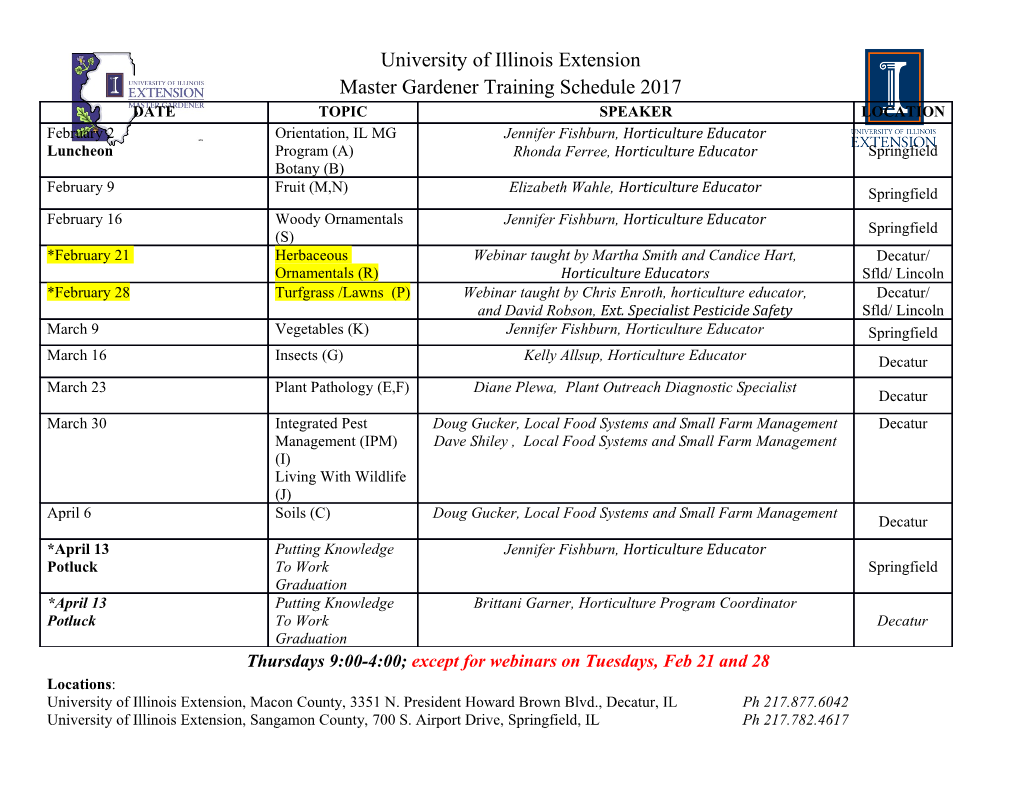
Client-side scripting JavaScript Elements • client-side script: code runs in browser a"er page is sent back from server – often this code manipulates the page or responds to user actions Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 2 Why use client-side programming? What is JavaScript? • PHP already allows us to create dynamic web pages. Why • a lightweight programming language ("scripting language") also use client-side scripting? • • Client-side scripting (JavaScript) benefits: used to make web pages interactive – – usability: can modify a page without having to post back to the server insert dynamic text into HTML (ex: user name) (faster UI) – react to events (ex: page load user click) – efficiency: can make small, quick changes to page without wai?ng for – get information about a user's computer (ex: browser type) server – perform calculations on user's computer (ex: form validation) – event-driven: can respond to user ac?ons like clicks and key presses • a web standard (but not supported identically by all • Server-side programming (PHP) benefits: browsers) – security: has access to server's private data; client can't see source code – compa7bility: not subject to browser compa?bility issues • NOT related to Java other than by name and some syntactic – power: can write files, open connec?ons to servers, connect to databases, ... similarities Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 3 4 Short History of JavaScript JavaScript and JAVA • Originally developed by Netscape, as LiveScript • JavaScript and Java are only related through syntax • Javascript is interpreted • Became a joint venture of Netscape and Sun in • JAVA is strongly typed - types are known at compile time and 1995, renamed JavaScript operand types (τύποι τελεστέων) are checked for • Now standardized by the European Computer compatibility Manufacturers Association as ECMA-262 (also • Variables in JavaScript are dynamically typed ISO 16262) – Compile-time type checking impossible • JavaScript has more relaxed syntax and rules • We’ll call collections of JavaScript code scripts, – fewer and "looser" data types not programs – variables don't need to be declared – errors often silent (few exceptions) http://www.ecma-international.org/publications/standards/Ecma-262.htm 5 6 JavaScript vs. Java JavaScript vs. PHP • interpreted, not compiled • similarities: – • more relaxed syntax and rules both are interpreted, not compiled – fewer and "looser" data types + = – both are relaxed about syntax, rules, and types – variables don't need to be declared – both are case-sensitive – errors often silent (few exceptions) – both have built-in regular expressions for powerful text processing • key construct is the function rather than the class • differences: – "first-class" functions are used in many situations – JS is more object-oriented: noun.verb(), less • contained within a web page and integrates with its HTML/ procedural: verb(noun) CSS content – JS focuses on UIs and interacting with a document; PHP on • Objects in: HTML output and files/forms – – JAVA are static: their collection of data members and methods JS code runs on the client's browser; PHP code runs on the web server is fixed at compile time – JavaScript are dynamic: members of an object can change during execution Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 7 8 Overview of JavaScript Object Orientation and JavaScript • JavaScript can be used to: • JavaScript is NOT an object-oriented programming – replace some of what is typically done with applets (except language graphics) – Does not support class-based inheritance - cannot support – replace some of what is done with CGI - namely server-side polymorphism programming (but no file operations or networking) – Has prototype-based inheritance, which is much different • User interactions through forms are easy • JavaScript objects are collections of properties, which • The Document Object Model makes it possible to support are like the members of classes in Java and C++ dynamic HTML documents with JavaScript • JavaScript has primitives for simple types – Much of what we will do with JavaScript is event-driven computation 9 10 Linking to a JavaScript file: script • script tag should be placed in HTML page's head • script code is stored in a separate .js file • JS code can be placed directly in the HTML file's body or head (like CSS) Linking JavaScript to HTML – but this is bad style (should separate content, presentation, and behavior) Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 12 JavaScript in HTML body (example) Browsers and HTML/JavaScript Documents • What happens when the browser encounters a JavaScript script in a document? • JS code can be embedded within your HTML • Two approaches for embedding JavaScript in HTML page's head or body documents: – Explicit embedding (ρητή ενσωμάτωση) • runs as the page is loading <script type = "text/javaScript"> • this is considered bad style and shouldn't be done in this -- JavaScript script – course </script> – mixes HTML content and JS scripts (bad) – Implicit embedding (υπόρρητη ενσωμάτωση) – can cause your page not to validate <script type = "text/javaScript" src = "myScript.js"> </script> Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 13 14 Implicit vs Explicit JavaScript JavaScript embedding • Explicit embedding: • Scripts are usually hidden from browsers that do – Mixes two types of notation inside the same document (bad for readability) not include JavaScript interpreters by putting – Makes maintenance difficult - often different people manage the them in special comments: HTML and the JavaScript <!-- • Depending on its use, a JavaScript script that is explicitly -- JavaScript script – embedded appears in: //--> – The head of an HTML document - for scripts that produce • This is also required by the HTML validator content only upon request or for those that react to user interactions • Note that the comment closure is in a new line • These scripts contain function definitions and code associated with form and is also a JS comment elements – The body of an HTML document - for scripts that are to be interpreted just once, as they are found 15 16 Event-driven programming • JS programs have no main; they respond to user actions called events • Event-driven programming: writing programs driven by user events Events in JavaScript • To respond to events, we can write code to respond to various events as the user generates them : event handlers Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 18 Event-driven programming A simple JavaScript Program • To respond to an event in JavaScript, we follow the following • Problem: create a page with a button that will cause some steps: code to run when it is clicked. – decide which element or control we want to respond to • Step 1: create the HTML Control – write a JavaScript function with the code we want to run (event – button's text appears inside tag; can also contain images handler) <!DOCTYPE html> – attach that function to the control’s event <html> <head><title>Javascript example</title></head> • Event handlers typically cause some sort of change to be <body> <div> made to the web page: <button type=“button">Say the magic word!</button> – </div> changing text on the page </body> – dynamically adjusting styles of elements on the page </html> – adding/removing content from the page • Step 2: Write JavaScript Event-Handling Function: – Need to add code to take action when button is clicked – Create a JS file with a function to execute when button is clicked function sayMagicWord() { alert(“PLEASE!”); Event Handler 19 } 20 Pop-up messages in JS A simple JavaScript Program • JavaScript has three methods for creating dialog boxes: • Step 3: Attach Event Handler to Control – alert, confirm, and prompt – connect JS file to HTML file, using <script> tag of HTML; syntax: <script src=“URL” type=“text/javascript”></script> • type attribute it optional in HTML5; used for backward compatibility • script typically contains no inner content, but not allowed to be a self- closing tag – attach the sayMagicWord JS function to the click of the button • Every HTML element has special event attributes that represent actions you can perform on it: onclick, onkeydown, onchange, onsubmit • To attach a handler that will “listen” to an event on an element, you set a value for one of the element’s event attributes, which points to the event handler <element onevent=“eventHandler();”>…</element> 21 22 <!DOCTYPE html> <html> <head><title>Javascript example</title></head> <script src=“please.js” type=“text/javascript”></script> <body> <div> <button onclick=“sayMagicWord();”>Say the magic word!</button> </div> </body> </html> – Note: sayMagicWord executes when the user clicks the button, not when the page is loaded 23 24 No Javascript? <!DOCTYPE html> <html> <head><title>Javascript example</title></head> <script src=“please.js” type=“text/javascript”></script> <body> <noscript> <p class=“error”>Warning: this page requires JavaScript. Please visit this site with a JS-enabled web browser.</p> <div> JavaScript Syntax <button onclick=“sayMagicWord();”>Say the magic word!</button> </div> </body> </html> 25 8.2 - 8.4: JavaScript Syntax Variables • 8.1: Key JavaScript Concepts • Names: Variable names begin with a letter or underscore, • 8.2, 8.3, 8.4: JavaScript Syntax followed by any number of letters, underscores, and digits – case sensitive • Object-oriented JavaScript Features • Types: JS determines the type of a variable dynamically, based on the kind of value you assign to it. • Variable declarations: – Explicit: using the keyword var var name = value; – Implicit: it is legal to declare a variable without the var keyword • It is better to avoid this for reasons of programming clarity var name1 = value; name2 = “Marty”; Copyright 2012 Marty Stepp, Jessica Miller, and Victoria Kirst 27 28 Variable scoping Types • Scope of a variable (πεδίο εμβέλειας): the range of statements over • Types are not specified, but JS does have types ("loosely which the variable is visible.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages24 Page
-
File Size-