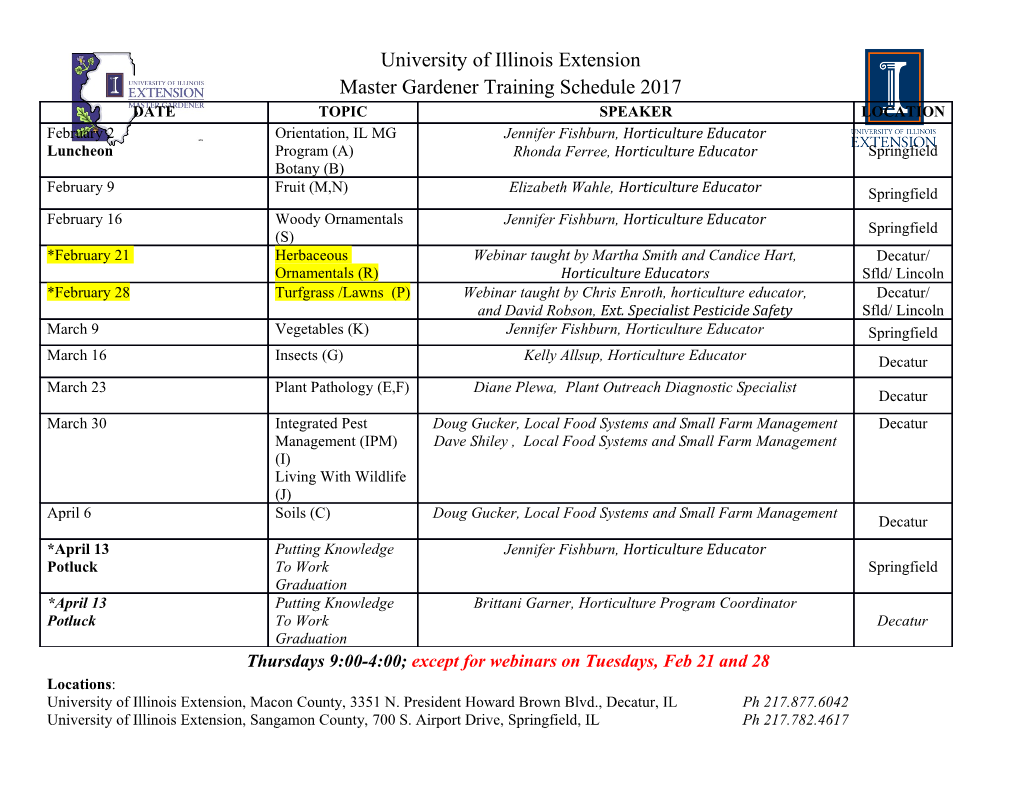
CSE 370 Spring 2006 Administrivia Introduction to Digital Design Homework 3 due Friday Lecture 8: Introduction to Verilog Last Lecture Design Examples a K-maps Minimization Algorithm Today Introduction to Verilog Algorithm for two-level simplification Algorithm for two-level Algorithm: minimum sum-of-products expression from a Karnaugh map simplification (example) A A A Step 1: choose an element of the ON-set X101 X101 X101 Step 2: find "maximal" groupings of 1s and Xs adjacent to that 0111 0111 0111 element D D D consider top/bottom row, left/right column, and corner 0XX0 0XX0 0XX0 adjacencies C C C this forms prime implicants (number of elements always a power 0101 0101 0101 of 2) B B B 2 primes around A'BC'D' 2 primes around ABC'D Repeat Steps 1 and 2 to find all prime implicants A A A Step 3: revisit the 1s in the K-map X101 X101 X101 if covered by single prime implicant, it is essential, and 0111 0111 0111 participates in final cover D D D 1s covered by essential prime implicant do not need to be 0XX0 0XX0 0XX0 revisited C C C Step 4: if there remain 1s not covered by essential prime implicants 0101 0101 0101 B B B select the smallest number of prime implicants that cover the 3 primes around AB'C'D' 2 essential primes minimum cover (3 primes) remaining 1s Visit All in the On Set? Activity List all prime implicants for the following K-map: A A 00X001X0 X0X0 0111X1 01X1 CD’ D BC BD AB AC’D D CD’ BC BD AB AC’D 010X11X0 0XX0 C C 00X10111 X111 B B CD’ BD AC’D CD’ BD AC’D Which are essential prime implicants? CD’ BD AC’D CD’ BD AC’D What is the minimum cover? Loose end: POS minimization Ways of specifying circuits using k-maps Schematics Using k-maps for POS minimization Structural description Encircle the zeros in the map Describe circuit as interconnected elements Interpret indices complementary to SOP form Build complex circuits using hierarchy A AB Large circuits are unreadable 00 01 11 10 F = (B’+C+D)(B+C+D’)(A’+B’+C) CD HDLs 00 1001 Check using de Morgan’s on SOP Hardware description languages 01 0100 D F’ = BC’D’+B’C’D+ABC’ Not programming languages 11 1111 C (F’)’ = (BC’D’+B’C’D+ABC’)’ Parallel languages tailored to digital design 10 1111 (F’)’ = (BC’D’)’+(B’C’D)’+(ABC’)’ Synthesize code to produce a circuit B F = (B’+C+D)(B+C+D’)(A’+B’+C) Hardware description languages Verilog (HDLs) module toplevel(clock,reset); input clock; Abel (~1983) input reset; reg flop1; Developed by Data-I/O reg flop2; Targeted to PLDs always @ (posedge reset or posedge clock) if (reset) Limited capabilities (can do state machines) begin flop1 <= 0; Verilog (~1985) flop2 <= 1; Developed by Gateway (now part of Cadence) end else Similar to C begin flop1 <= flop2; Moved to public domain in 1990 flop2 <= flop1; end VHDL (~1987) endmodule DoD sponsored Similar to Ada Verilog versus VHDL Simulation versus synthesis Simulation Both “IEEE standard” languages “Execute” a design to verify correctness Most tools support both Synthesis Verilog is “simpler” Generate a netlist from HDL code Less syntax, fewer constructs VHDL is more structured HDL synthesis circuit Can be better for large, complex systems description Better modularization “execution” simulation functional functional/timing validation validation Simulation versus synthesis Simulation (con’t) Simulation You provide an environment Models what a circuit does Using non-circuit constructs Multiply is “*”, ignoring implementation options Read files, print, control simulation Can include static timing Using Verilog simulation code Note: We will ignore Allows you to test design options A “test fixture” timing and test benches Synthesis until next Verilog lecture Converts your code to a netlist Simulation Can simulate synthesized design Test Fixture Circuit Description Tools map your netlist to hardware (Specification) (Synthesizeable) Verilog and VHDL simulate and synthesize CSE370: Learn simulation CSE467 (Advanced Digital Design): Learn synthesis Levels of abstraction Structural versus behavioral Verilog supports 4 description levels Verilog Switch Structural structural Gate Describe explicit circuit elements Dataflow behavioral Describe explicit connections between elements Algorithmic Connections between logic gates Can mix & match levels in a design Just like schematics, but using text Designs that combine dataflow and algorithmic Behavioral constructs and synthesis are called RTL Describe circuit as algorithms/programs Register Transfer Level What a component does Input/output behavior Many possible circuits could have same behavior Different implementations of a Boolean function Verilog tips Basic building blocks: Modules Instanced into a design Do not write C-code Never called A AND2 Think hardware, not algorithms Illegal to nest module defs. E OR2 g1 Verilog is inherently parallel B 1 g3 X Modules execute in parallel 3 Compilers don’t map algorithms to circuits well Names are case sensitive NOT C Y Do describe hardware circuits // for comments 2 g2 First draw a dataflow diagram Name can’t begin with a Then start coding // first simple example number module smpl (X,Y,A,B,C); References Use wires for connections input A,B,C; output X,Y; Tutorial and reference manual are found in ActiveHDL and, or, not are keywords wire E help All keywords are lower case and g1(E,A,B); not g2(Y,C); And in this week’s reading assignment Gate declarations (and, or, etc) or g3(X,E,Y); “Starter’s Guide to Verilog 2001” by Michael Ciletti List outputs first endmodule copies for borrowing in hardware lab Inputs second Modules are circuit components Structural Verilog Module has ports A AND2 module xor_gate (out,a,b); E OR2 g1 input a,b; External connections B 1 g3 X 3 output out; 8 basic gates (keywords): wire abar, bbar, t1, t2; A,B,C,X,Y in example NOT and, or, nand, nor C Y not inva (abar,a); buf, not, xor, xnor Port types 2 g2 not invb (bbar,b); input and and1 (t1,abar,b); and and2 (t2,bbar,a); output // previous example as a or or1 (out,t1,t2); inout (tristate) // Boolean expression endmodule module smpl2 (X,Y,A,B,C); NOT abar AND2 a t1 Use assign statements for input A,B,C; 4 and1 inva b 6 Boolean expressions output X,Y; OR2 assign X = (A&B)|~C; or1 out and ⇔ & assign Y = ~C; 8 NOT bbar AND2 endmodule b or ⇔ | 5 and2 invb a 7 t2 not ⇔ ~ Behavioral Verilog Behavioral 4-bit adder A module add4 (SUM, OVER, A, B); Describe circuit behavior Sum input [3:0] A; B Adder Cout input [3:0] B; Not implementation Cin output [3:0] SUM; output OVER; assign {OVER, SUM[3:0]} = A[3:0] + B[3:0]; module full_addr (Sum,Cout,A,B,Cin); endmodule input A, B, Cin; output Sum, Cout; assign {Cout, Sum} = A + B + Cin; “[3:0] A” is a 4-wire bus labeled “A” endmodule Bit 3 is the MSB Bit 0 is the LSB {Cout, Sum} is a concatenation Can also write “[0:3] A” Buses are implicitly connected Bit 0 is the MSB If you write BUS[3:2], BUS[1:0] Bit 3 is the LSB They become part of BUS[3:0] Data types Numbers Format: <sign><size><base format><number> Values on a wire 14 0, 1, x (don’t care), z (tristate or unconnected) Decimal number Vectors –4’b11 A[3:0] vector of 4 bits: A[3], A[2], A[1], A[0] Unsigned integer value 4-bit 2’s complement binary of 0011 (is 1101) Indices must be constants 12’b0000_0100_0110 12 bit binary number (_ is ignored) Concatenating bits/vectors e.g. sign extend 3’h046 B[7:0] = {A[3], A[3], A[3], A[3], A[3:0]}; 3-digit (12-bit) hexadecimal number B[7:0] = {4{A[3]}, A[3:0]}; Verilog values are unsigned Style: Use a[7:0] = b[7:0] + c; C[4:0] = A[3:0] + B[3:0]; Not a = b + c; if A = 0110 (6) and B = 1010(–6), then C = 10000 (not 00000) Legal syntax: C = &A[6:7]; // logical and of bits 6 and 7 of A B is zero-padded, not sign-extended Continuous assignment Operators Assignment is continuously evaluated Corresponds to a logic gate Assignments execute in parallel Boolean operators (~ for bit-wise negation) assign A = X | (Y & ~Z); bits can assume four values assign B[3:0] = 4'b01XX; (0, 1, X, Z) variables can be n-bits wide assign C[15:0] = 4'h00ff; (MSB:LSB) assign #3 {Cout, Sum[3:0]} = A[3:0] + B[3:0] + Cin; arithmetic operator Similar to C operators Gate delay (used by simulator) multiple assignment (concatenation) Example: A comparator Comparator example (con’t) // Make a 4-bit comparator from 4 1-bit comparators module Compare1 (Equal, Alarger, Blarger, A, B); input A, B; module Compare4(Equal, Alarger, Blarger, A4, B4); output Equal, Alarger, Blarger; input [3:0] A4, B4; assign Equal = (A & B) | (~A & ~B); output Equal, Alarger, Blarger; assign Alarger = (A & ~B); wire e0, e1, e2, e3, Al0, Al1, Al2, Al3, B10, Bl1, Bl2, Bl3; assign Blarger = (~A & B); Compare1 cp0(e0, Al0, Bl0, A4[0], B4[0]); endmodule Compare1 cp1(e1, Al1, Bl1, A4[1], B4[1]); Compare1 cp2(e2, Al2, Bl2, A4[2], B4[2]); Compare1 cp3(e3, Al3, Bl3, A4[3], B4[3], ); Top-down design and bottom-up design are both okay assign Equal = (e0 & e1 & e2 & e3); assign Alarger = (Al3 | (Al2 & e3) | ⇒ module ordering doesn’t matter (Al1 & e3 & e2) | ⇒ because modules execute in parallel (Al0 & e3 & e2 & e1)); assign Blarger = (~Alarger & ~Equal); endmodule Sequential Verilog-- Blocking Functions and non-blocking assignments Blocking assignments (Q = A) Use functions for complex combinational logic Variable is assigned immediately module and_gate (out, in1, in2); New value is used by subsequent statements input in1, in2; output out; Non-blocking assignments (Q <= A) assign out = myfunction(in1, in2); Variable is assigned after all scheduled statements are executed function myfunction; input in1, in2; Value to be assigned is computed but saved for later begin Usual use:
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages9 Page
-
File Size-