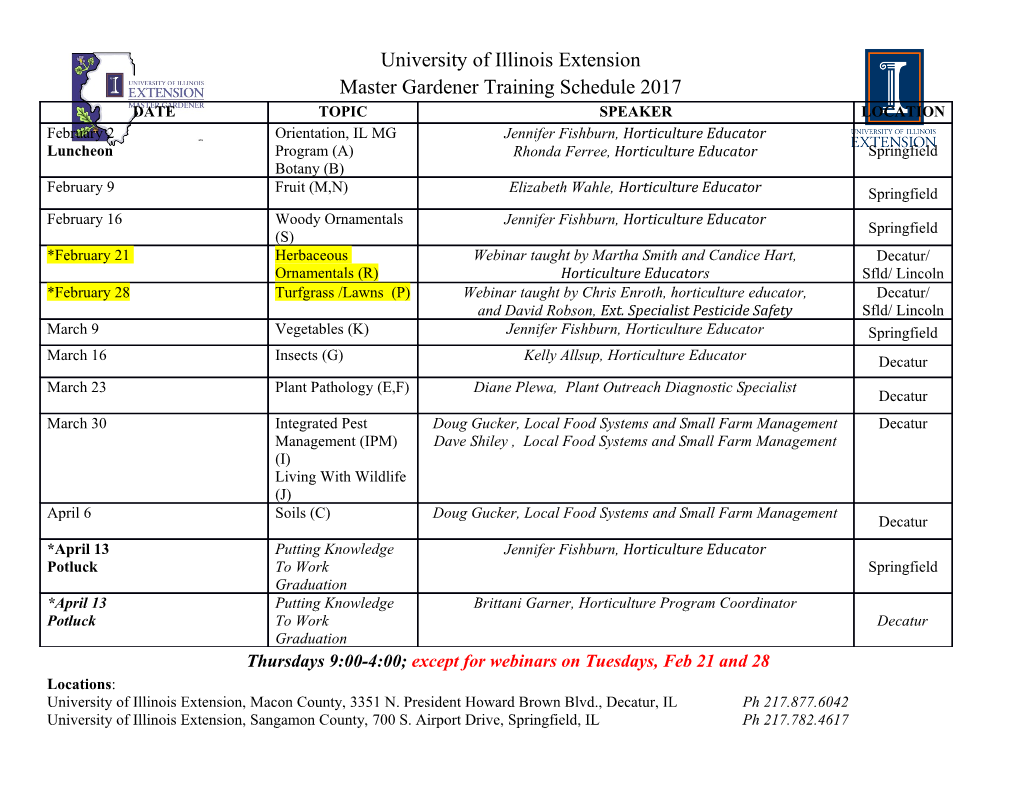
Computer Science Technical Reports Computer Science 7-2001 Alias-free parameters in C using multibodies Medhat Assaad Iowa State University Follow this and additional works at: http://lib.dr.iastate.edu/cs_techreports Part of the Programming Languages and Compilers Commons, and the Theory and Algorithms Commons Recommended Citation Assaad, Medhat, "Alias-free parameters in C using multibodies" (2001). Computer Science Technical Reports. 301. http://lib.dr.iastate.edu/cs_techreports/301 This Article is brought to you for free and open access by the Computer Science at Iowa State University Digital Repository. It has been accepted for inclusion in Computer Science Technical Reports by an authorized administrator of Iowa State University Digital Repository. For more information, please contact [email protected]. Alias-free parameters in C using multibodies Abstract Aliasing may cause problems for both optimization and reasoning about programs. Since static alias analysis is expensive, and sometimes, even impossible, compilers tend to be conservative. This usually leads to missing some optimization opportunities. Furthermore, when trying to understand code, one must consider all possible aliasing patterns. This can make reasonning about code difficult and non-trivial. We have implemented an approach for having alias-free parameters in C. This approach allows aliasing between the arguments at the call site, but guarantees that there will be no aliasing between arguments and between arguments and globals inside procedure bodies. This is done by having multiple bodies, up to one for each aliasing pattern. Procedure calls will be dispatched to the body that matches the aliasing pattern at the call site. By having alias-free parameters in the bodies, good optimization can be achieved. Furthermore, verification and reasoning about the code is easier when there is no aliasing. The ainm advantages of our approach are that code to determine the aliasing pattern is automatically generated, and the programmer does not have to code it by hand. We tested our implementation, and found that using this approach can be very practical. It is easy to convert already-existing code to use it. And in some cases, we had run-time execution speedup as much as 29%. Keywords pointer parameter aliasing, global variable aliasing, multi-body procedures, dynamic dispatch, static dispatch, ACL language, C/ACL, alias-free programs, compiler opti-mizations, GCC Disciplines Programming Languages and Compilers | Theory and Algorithms This article is available at Iowa State University Digital Repository: http://lib.dr.iastate.edu/cs_techreports/301 Alias-free parameters in C using multibodies Medhat G. Assaad TR #01-05 July 2001 Keywords: pointer parameter aliasing, global variable aliasing, multi-body procedures, dynamic dispatch, static dispatch, ACL language, C/ACL, alias-free programs, compiler opti- mizations, GCC. 2000 CR Categories: D.3.1 [Programming Languages] Formal Definitions and Theory — sematics; D.3.3 [Programming Languages] Language Constructs and Features — control struc- tures, procedures, functions, and subroutines; D.3.4 [Programming Languages] Processors — compilers, optimization, experimetation, performance; D.3.m [Programming Languages] Mis- cellaneous — dynamic dispatch, multiple dispatch; F.3.1 [Logics and Meanings of Programs] Specifying and Verifying and Reasoning about Programs — logics of programs. c Medhat G. Assaad, 2001. All rights reserved. Department of Computer Science 226 Atanasoff Hall Iowa State University Ames, Iowa 50011-1040, USA ii TABLE OF CONTENTS CHAPTER 1 INTRODUCTION . 1 1.1 Problems caused from aliasing . 1 1.2 Literature review . 3 1.3 The ACL approach . 6 1.3.1 The ‘imports’ clause . 7 1.3.2 Multi-bodies . 7 1.4 Hypothesis . 7 1.4.1 Specially written bodies . 8 1.4.2 Alias-free arguments . 8 1.4.3 Dynamic dispatch . 9 1.4.4 Static dispatch . 9 1.5 Paper organization . 9 CHAPTER 2 EXPERIMENTAL DESIGN . 10 2.1 Setup . 10 2.2 Overview of GCC . 11 2.2.1 The C grammar file . 11 2.2.2 Trees in GCC . 11 2.2.3 Identifiers & Symbol lookup in C . 12 2.2.4 Chains and lists . 13 2.2.5 Binding levels in C . 13 2.2.6 The compilation process . 13 2.2.7 Memory allocation in GCC . 14 iii 2.3 Code changes . 14 2.3.1 The ‘imports’ clause . 15 2.3.2 Multi-bodies . 17 2.3.3 The Dynamic Dispatch code . 19 2.3.4 Static dispatch . 21 CHAPTER 3 RESULTS . 23 3.1 Testing methodology . 23 3.2 Test results . 24 CHAPTER 4 DISCUSSION . 27 4.1 Decisions we made in our implementation . 27 4.2 Pragmatic value of ACL . 27 CHAPTER 5 CONCLUSION AND FUTURE WORK . 29 APPENDIX SOURCE CODE . 30 A.1 Changes made to the grammar . 30 A.2 The binding level structure . 38 A.3 The Identifier structure . 40 A.4 The acl function structure . 41 A.5 Code to reuse acl function structures . 42 A.6 The acl body structure . 43 A.7 Name lookup functions . 44 A.8 Code to store and restore the globals from the imports clause . 46 A.9 Code to start an ACL function . 50 A.10 Code to finish an ACL function . 53 A.11 Code used to start ACL bodies . 56 A.12 Code to finish an ACL body . 58 A.13 The decision tree structure . 59 A.14 The process alias lists function . 60 A.15 Code to generate the dynamic dispatch . 65 iv A.16 Other helper functions . 70 BIBLIOGRAPHY . 74 1 CHAPTER 1 INTRODUCTION Two names are aliases if they point to the same storage cell in memory, which means that this storage cell can be modified through more than one path; so if a variable x is not modified in a code segment, still, the memory location associated with x can be modified if another variable y that is an alias to x is modified in the same code segment. The presence of aliases adds to the complexity of a program, making it more difficult to reason about and verify. Moreover, the presence of aliases limits the amount of optimization that can be done by a compiler, unless it does high-quality alias analysis [11, p. 293], which is usually very costly. This paper discusses some of the different approaches that have been used to deal with aliasing problems, and it studies the effectiveness of the ACL approach [10]. The ACL approach limits aliasing based on dispatch to different bodies of the same function according to the aliasing pattern at the call site. In this paper we use the same approach and apply it to the C programming language, and then measure the performance of the resulting code. The rest of this introduction is organized as follows. Section 1.1 discusses problems caused from aliasing. Section 1.2 gives an overview of some of the approaches that are used to deal with aliasing problems, and shows the reasons why we found that ACL has something new to offer. Section 1.3 shows the ACL approach. Finally we give an outline of the rest of the paper. 1.1 Problems caused from aliasing Aliasing can occur for many reasons, one of which is passing pointers. Many approaches (which will be discussed later) have been suggested for dealing with aliasing. In this paper, we apply the ACL approach to the C programming language, to measure the performance that can be achieved by eliminating aliasing between procedure arguments, and between arguments 2 and global variables, which enables the compiler to do more aggressive optimization. Aliasing from parameters can happen in two ways. First, the same pointer can be passed into two parameters, in this case, this same location will have two different names, aliases, inside the called procedure. The other way is when a pointer to a global is passed as one of the arguments. If this global is used inside the called procedure, then again, it will have two names inside that procedure. These pointers can result from the use of call-by-reference, indirect storage models, or, as in C, from explicit constructs in the language. Aliases can cause, in general, two kinds of problems. The first problem is that in the presence of aliases, it is much harder to reason about the correctness of the code. For example, in Figure 1.1, the get sum() procedure is supposed to calculate the sum of the elements of array a, and return this sum in the location pointed to by the parameter sum. While this code may look like it will always return the required result, in the presence of aliasing, this may not always be true. It may return an incorrect result if *sum is itself one of the locations in the array a; for example if invoked with get sum(b, 10, &b[3]). In C, *sum refers to the memory location pointed to by sum, while &x returns the address of x. void get_sum (int a[], int num, int *sum){ int i; *sum=0; for (i=0; i < num; i++) *sum += a[i]; } Figure 1.1 An example of correctness problems caused by aliases The second kind of problem that may be caused by aliases may not affect the correctness of code but will limit the ability of the compiler to achieve good optimization. In the code given in Figure 1.2, while the equality check between c and *a may seem to be always true and redundant, it cannot be removed by an optimizing compiler, since *a and g may be aliases, and maybe modify g() may potentially alter the value of g. Thus the redundancy of the check is 3 not provable unless the compiler can figure out the aliasing relationship between the argument to foo() and all the globals that are used in maybe modify g().
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages81 Page
-
File Size-