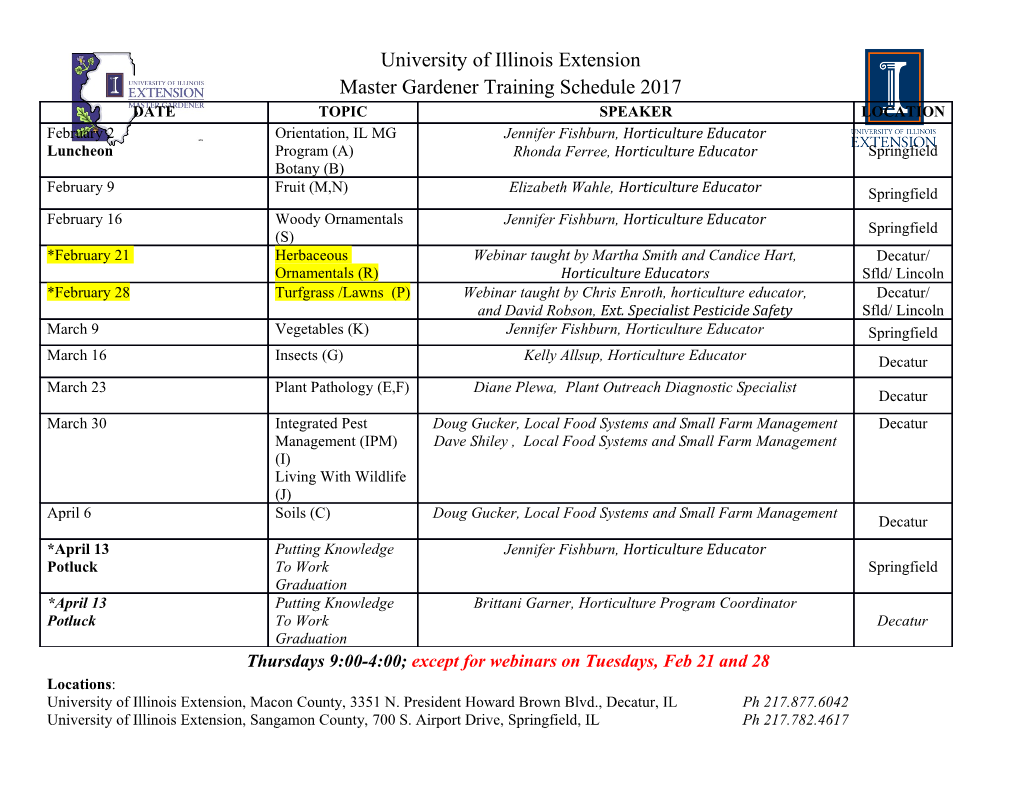
Computer Science II Dr. Chris Bourke Department of Computer Science & Engineering University of Nebraska|Lincoln Lincoln, NE 68588, USA http://chrisbourke.unl.edu [email protected] 2019/08/15 13:02:17 Version 0.2.0 This book is a draft covering Computer Science II topics as presented in CSCE 156 (Computer Science II) at the University of Nebraska|Lincoln. This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License i Contents 1 Introduction1 2 Object Oriented Programming3 2.1 Introduction.................................... 3 2.2 Objects....................................... 4 2.3 The Four Pillars.................................. 4 2.3.1 Abstraction................................. 4 2.3.2 Encapsulation................................ 4 2.3.3 Inheritance ................................. 4 2.3.4 Polymorphism................................ 4 2.4 SOLID Principles................................. 4 2.4.1 Inversion of Control............................. 4 3 Relational Databases5 3.1 Introduction.................................... 5 3.2 Tables ....................................... 9 3.2.1 Creating Tables...............................10 3.2.2 Primary Keys................................16 3.2.3 Foreign Keys & Relating Tables......................18 3.2.4 Many-To-Many Relations .........................22 3.2.5 Other Keys .................................24 3.3 Structured Query Language ...........................26 3.3.1 Creating Data................................28 3.3.2 Retrieving Data...............................30 3.3.3 Updating Data ...............................36 3.3.4 Destroying Data ..............................36 3.3.5 Processing Data...............................37 3.4 Normalization & Database Design........................47 3.4.1 Design Example...............................50 3.5 Miscellaneous ...................................52 3.6 Exercises......................................53 4 List-Based Data Structures 57 4.1 Array-Based Lists.................................58 4.1.1 Designing a Java Implementation.....................58 iii Contents 4.2 Linked Lists....................................65 4.2.1 Designing a Java Implementation.....................71 4.2.2 Variations..................................74 4.3 Stacks & Queues .................................77 4.3.1 Stacks....................................77 4.3.2 Queues....................................82 4.3.3 Variations..................................86 5 Algorithm Analysis 91 5.1 Introduction....................................91 5.1.1 Example: Computing a Sum........................97 5.1.2 Example: Computing a Mode.......................99 5.2 Pseudocode ...................................102 5.3 Analysis .....................................105 5.4 Asymptotics...................................109 5.4.1 Big-O Analysis ..............................109 5.4.2 Other Notations..............................111 5.4.3 Observations ...............................113 5.4.4 Limit Method...............................116 5.5 Examples ....................................118 5.5.1 Linear Search...............................118 5.5.2 Set Operation: Symmetric Difference..................120 5.5.3 Euclid's GCD Algorithm.........................121 5.5.4 Selection Sort...............................123 5.6 Other Considerations..............................124 5.6.1 Importance of Input Size.........................124 5.6.2 Control Structures are Not Elementary Operations . 127 5.6.3 Average Case Analysis..........................128 5.6.4 Amortized Analysis............................129 5.7 Analysis of Recursive Algorithms .......................130 5.7.1 The Master Theorem...........................131 5.8 Exercises.....................................134 6 Trees 137 6.1 Introduction...................................137 6.2 Definitions & Terminology...........................137 6.3 Implementation.................................146 6.4 Tree Traversal..................................147 6.4.1 Preorder Traversal ............................148 6.4.2 Inorder Traversal.............................150 6.4.3 Postorder Traversal............................153 6.4.4 Tree Walk Traversal ...........................157 6.4.5 Breadth-First Search Traversal .....................159 iv Contents 6.5 Binary Search Trees...............................161 6.5.1 Retrieval..................................162 6.5.2 Insertion..................................163 6.5.3 Removal..................................165 6.5.4 In Practice ................................167 6.6 Heaps ......................................169 6.6.1 Operations ................................170 6.6.2 Implementations .............................175 6.6.3 Variations.................................179 6.6.4 Applications................................180 6.7 Exercises.....................................183 Appendix 185 1 Author-Book Database SQL..........................185 Glossary 187 Acronyms 191 Index 195 References 195 v List of Algorithms 1 Insert-At-Head Linked List Operation ......................66 2 Insert Between Two Nodes Operation ......................67 3 Index-Based Retrieval Operation.........................69 4 Key-Based Delete Operation ...........................71 5 Computing the Mean ..............................103 6 Computing the Mode ..............................104 7 Trivial Sorting (Bad Pseudocode)........................104 8 Trivially Finding the Minimal Element.....................105 9 Finding the Minimal Element..........................105 10 Linear Search...................................119 11 Symmetric Difference of Two Sets .......................120 12 Euclid's GCD Algorithm............................. 121 13 Selection Sort...................................123 14 Sieve of Eratosthenes ..............................125 15 Fibonacci(n) ...................................131 16 Binary Search { Recursive............................133 17 Merge Sort ....................................133 18 Stack-based Preorder Tree Traversal ......................149 19 preOrderTraversal(u): Recursive Preorder Tree Traversal . 150 20 Stack-based Inorder Tree Traversal.......................151 21 inOrderTraversal(u): Recursive Inorder Tree Traversal . 153 22 Stack-based Postorder Tree Traversal......................154 23 postOrderTraversal(u): Recursive Postorder Tree Traversal . 157 24 Tree Walk based Tree Traversal.........................160 25 Queue-based BFS Tree Traversal........................161 26 Search algorithm for a binary search tree....................163 27 Finding the maximum key value in a node's left subtree. 167 28 Heapify......................................171 29 Find Next Open Spot - Numerical Technique . 179 30 Heap Sort..................................... 181 vii List of Code Samples 3.1 A simple table definition. We use this as an illustrative example, it is not necessarily a well-designed table. ........................10 3.2 A many-to-many Author/Book table design..................23 3.3 Full Author/Book table database........................27 4.1 Parameterized Array-Based List in Java ....................88 4.2 A linked list node Java implementation. Getter and setter methods have been omitted for readability. A convenience method to determine if a node has a next element is included. This implementation uses null as its terminating value. ................................89 5.1 Summing a collection of integers ........................94 5.2 Summation Algorithm 1.............................97 5.3 Summation Algorithm 2.............................97 5.4 Summation Algorithm 3.............................97 5.5 Mode Finding Algorithm 1 ...........................99 5.6 Mode Finding Algorithm 2 ..........................100 5.7 Mode Finding Algorithm 3 ..........................101 5.8 Naive Exponentiation .............................126 5.9 Computing an Average ............................127 ix List of Figures 3.1 CSV Formatted Data.............................. 6 3.2 JSON and XML data representation examples................ 8 3.3 Parent Child Table Relation..........................20 3.4 Entity-Relation Diagram............................21 3.5 A many-to-many relationship using a join table................24 3.6 Author/Book Many-to-Many Data ......................25 3.7 Final Author-Book Database..........................28 3.8 A 3-D cube projected onto a 2-D surface producing a square. 38 3.9 Enrollment Database..............................52 3.10 Enrollment Data ................................53 3.11 Simple Video Game Database .........................54 4.1 A simple linked list containing 3 nodes.....................66 4.2 Insert-at-head Operation in a Linked List. We wish to insert a new element, 42 at the head of the list........................67 4.3 Inserting Between Two Nodes in a Linked List. Here, we wish to insert a new element 42 between the given two nodes containing 8 and 6. 68 4.4 Delete Operation in a Linked List.......................70 4.5 Key-Based Find and Remove Operation. We wish to remove the first node we find containing 42...............................72 4.6 A Doubly Linked List Example ........................75 4.7 A Circularly Linked List Example.......................76 4.8 An Unrolled Linked List Example.......................76 4.9 A stack holding integer elements. Push and pop operations are depicted as happening at the \top" of the stack. In actuality, a stack stored
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages209 Page
-
File Size-