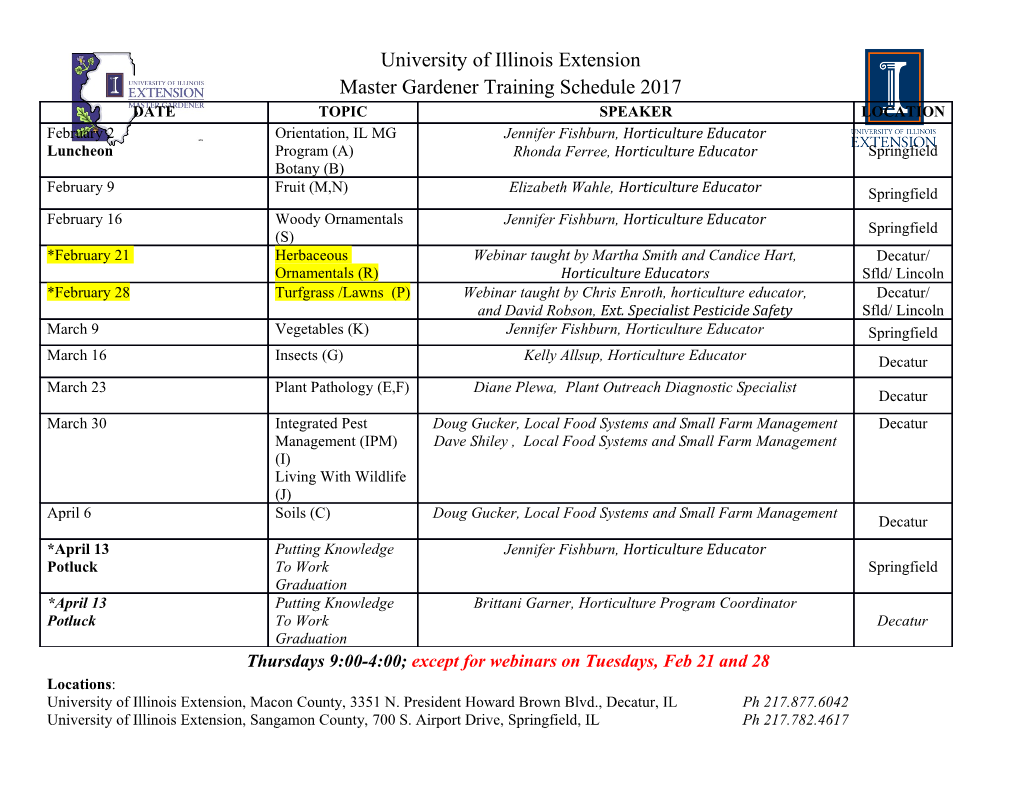
COMPUTER SCIENCE COMPUTER SCIENCE SEDGEWICK/WAYNE SEDGEWICK/WAYNE PART I: PROGRAMMING IN JAVA PART I: PROGRAMMING IN JAVA Computer Science 2. Conditionals & Loops •Conditionals: the if statement Computer 2. Conditionals and loops •Loops: the while statement Science •An alternative: the for loop An Interdisciplinary Approach •Nesting R O B E R T S E D G E W I C K 1.3 KEVIN WAYNE •Debugging http://introcs.cs.princeton.edu CS.2.A.Loops.If Context: basic building blocks for programming Conditionals and Loops Control flow • The sequence of statements that are actually executed in a program. any program you might want to write • Conditionals and loops enable us to choreograph control flow. objects true boolean 1 functions and modules statement 1 graphics, sound, and image I/O false statement 2 statement 1 arrays boolean 2 true statement 2 conditionalsconditionals and loops statement 3 Math text I/O false statement 4 statement 3 primitive data types assignment statements This lecture: to infinity and beyond! Previous lecture: straight-line control flow control flow with conditionals and a loop equivalent to a calculator [ previous lecture ] [this lecture] 3 4 The if statement Example of if statement use: simulate a coin flip Execute certain statements depending on the values of certain variables. • Evaluate a boolean expression. public class Flip • If true, execute a statement. { • The else option: If false, execute a different statement. public static void main(String[] args) { if (Math.random() < 0.5) System.out.println("Heads"); Example: if (x > y) max = x; Example: if (x < 0) x = -x; else else max = y; System.out.println("Tails"); % java Flip } Heads } true true x < 0 ? false x > y ? false % java Flip Heads x = -x; max = x; max = y; % java Flip Tails % java Flip Heads Replaces x with the absolute value of x Computes the maximum of x and y 5 6 Example of if statement use: 2-sort Pop quiz on if statements Q. What does this program do? Q. Add code to this program that puts a, b, and c in numerical order. public class TwoSort public class ThreeSort { { public static void main(String[] args) public static void main(String[] args) { { int a = Integer.parseInt(args[0]); int a = Integer.parseInt(args[0]); int b = Integer.parseInt(args[1]); int b = Integer.parseInt(args[1]); % java ThreeSort 1234 99 1 if (b < a) int c = Integer.parseInt(args[2]); 1 { % java TwoSort 1234 99 99 int t = a; alternatives for if and else 99 1234 a = b; can be a sequence of 1234 statements, enclosed in braces b = t; % java ThreeSort 99 1 1234 } % java TwoSort 99 1234 1 System.out.println(a); 99 99 System.out.println(b); 1234 System.out.println(a); 1234 } System.out.println(b); } System.out.println(c); } } A. Reads two integers from the command line, then prints them out in numerical order. 7 8 Pop quiz on if statements Example of if statement use: error checks Q. Add code to this program that puts a, b, and c in numerical order. public class IntOps { public static void main(String[] args) public class ThreeSort { % java IntOps 5 2 { 5 + 2 = 7 public static void main(String[] args) int a = Integer.parseInt(args[0]); { int b = Integer.parseInt(args[1]); 5 * 2 = 10 int a = Integer.parseInt(args[0]); int sum = a + b; 5 / 2 = 2 int b = Integer.parseInt(args[1]); % java ThreeSort 1234 99 1 int prod = a * b; 5 % 2 = 1 int c = Integer.parseInt(args[2]); 1 System.out.println(a + " + " + b + " = " + sum); if (b < a) % java IntOps 5 0 A. 99 System.out.println(a + " * " + b + " = " + prod); { int t = a; a = b; b = t; } 1234 5 + 0 = 5 makes a smaller if (b == 0) System.out.println("Division by zero"); if (c < a) than b 5 * 0 = 0 else System.out.println(a + " / " + b + " = " + a / b); { int t = a; a = c; c = t; } makes a smaller % java ThreeSort 99 1 1234 Division by zero if (c < b) than both b and c 1 if (b == 0) System.out.println("Division by zero"); Division by zero { int t = b; b = c; c = t; } makes b smaller 99 else System.out.println(a + " % " + b + " = " + a % b); System.out.println(a); than c 1234 } System.out.println(b); System.out.println(c); } } } Good programming practice. Use conditionals to check for and avoid runtime errors. 9 10 COMPUTER SCIENCE COMPUTER SCIENCE SEDGEWICK/WAYNE SEDGEWICK/WAYNE PART I: PROGRAMMING IN JAVA PART I: PROGRAMMING IN JAVA Image sources http://commons.wikimedia.org/wiki/File:Calculator_casio.jpg http://en.wikipedia.org/wiki/File:Buzz-lightyear-toy-story-3-wallpaper.jpg http://www.ncbi.nlm.nih.gov/pmc/articles/PMC2789164/#!po=30.0000 [181e306f1.jpg] 2. Conditionals & Loops •Conditionals: the if statement •Loops: the while statement •An alternative: the for loop •Nesting •Debugging CS.2.A.Loops.If CS.2.B.Loops.While The while loop Example of while loop use: print powers of two A trace is a table of variable values after each statement. Execute certain statements repeatedly until certain conditions are met. public class PowersOfTwo i v i <= n • Evaluate a boolean expression. i = 0; { 0 1 true If true, execute a sequence of statements. • public static void main(String[] args) 1 2 true • Repeat. v = 1; { int n = Integer.parseInt(args[0]); 2 4 true int i = 0; 3 8 true i <= n ? false Example: int v = 1; 4 16 true int i = 0; while (i <= n) int v = 1; true { 5 32 true while (i <= n) System.out.println(v); System.out.println(v); 6 64 true { i = i + 1; % java PowersOfTwo 6 7 128 false 1 System.out.println(v); v = 2 * v; i = i + 1; 2 i = i + 1; } 4 v = 2 * v; } 8 } v = 2 * v; 16 } 32 64 Prints the powers of two from 20 to 2n . Prints the powers of two from 20 to 2n . [stay tuned for a trace] 13 14 Pop quiz on while loops Pop quiz on while loops Q. Anything wrong with the following code? Q. Anything wrong with the following code? public class PQwhile public class PQwhile { { public static void main(String[] args) public static void main(String[] args) { { Q. What does it do (without the braces)? int n = Integer.parseInt(args[0]); int n = Integer.parseInt(args[0]); int i = 0; int i = 0; int v = 1; int v = 1; A. Goes into an infinite loop. while (i <= n) while (i <= n) System.out.println(v); { System.out.println(v); i = i + 1; i = i + 1; % java PQwhile 6 v = 2 * v; v = 2 * v; } 1 } } 1 1 challenge: figure out } } how to stop it 1 on your computer 1 1 1 A. Yes! Needs braces. 1 1 1 15 1 16 Example of while loop use: implement Math.sqrt() Example of while loop use: implement Math.sqrt() Goal. Implement square root function. % java Sqrt 60481729.0 Newton-Raphson method to compute √c 7777.0 Scientists studied % java Sqrt 2.0 • Initialize t0 = c. computation well before 1.4142136 • Repeat until ti = c/ti (up to desired precision): the onset of the computer. Set ti+1 to be the average of ti and c / ti. Newton-Raphson method to compute √c Isaac Newton 1642-1727 • Initialize t0 = c. if t = c/t then t2 = c public class Sqrt • Repeat until ti = c/ti (up to desired precision): { Set ti+1 to be the average of ti and c / ti. public static void main(String[] args) { double EPS = 1E-15; error tolerance (15 places) % java Sqrt 60481729.0 i ti 2/ti average 7777.0 double c = Double.parseDouble(args[0]); 0 2 1 1.5 double t = c; % java Sqrt 2.0 1 1.5 1.3333333 1.4166667 while (Math.abs(t - c/t) > t*EPS) 1.414213562373095 t = (c/t + t) / 2.0; 2 1.4166667 1.4117647 1.4142157 System.out.println(t); 3 1.4142157 1.4142114 1.4142136 } } 4 1.4142136 1.4142136 computing the square root of 2 to seven places 17 18 Newton-Raphson method COMPUTER SCIENCE SEDGEWICK/WAYNE PART I: PROGRAMMING IN JAVA Explanation (some math omitted) • Goal: find root of function f(x) (value of x for which f(x) = 0). use f(x) = x2 − c for √c Image sources • Start with estimate t0. http://www.sciencecartoonsplus.com • Draw line tangent to curve at x = ti . http://en.wikipedia.org/wiki/Isaac_Newton • Set ti+1 to be x-coordinate where line hits x-axis. y = f(x) http://www.onlinemathtutor.org/help/wp-content/uploads/math-cartoon-28112009.jpg • Repeat until desired precision. root: f(x) = 0 ti+3 ti+2 ti+1 ti 19 CS.2.B.Loops.While COMPUTER SCIENCE The for loop SEDGEWICK/WAYNE PART I: PROGRAMMING IN JAVA An alternative repetition structure. Why? Can provide code that is more compact and understandable. • Evaluate an initialization statement. • Evaluate a boolean expression. • If true, execute a sequence of statements, then execute an increment statement. 2. Conditionals & Loops • Repeat. •Conditionals: the if statement Example: initialization statement Every for loop has an equivalent while loop: int v = 1; int v = 1; •Loops: the while statement for ( int i = 0; i <= n; i++ ) int i = 0; •An alternative: the for loop { boolean expression while ( i <= n; ) System.out.println( i + " " + v ); { •Nesting v = 2*v; System.out.println( i + " " + v ); increment statement •Debugging } v = 2*v; i++; Prints the powers of two from 20 to 2n } CS.2.C.Loops.For 22 Examples of for loop use Example of for loop use: subdivisions of a ruler sum i int sum = 0; 1 1 Create subdivisions of a ruler to 1/N inches.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-