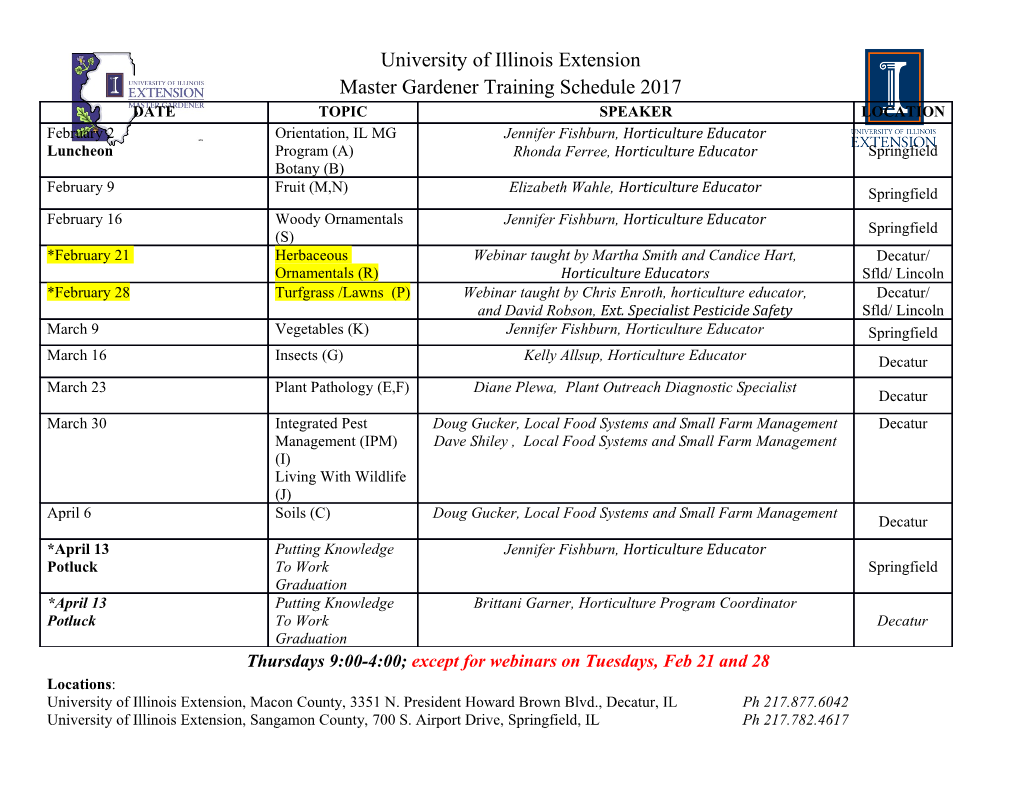
www.getmyuni.com In this section we cover the following: • State graphs introduction • Serial Adder • Multiplier • Divider STATE GRAPHS FOR CONTROL NETWORKS What are the conditions for having a proper state graph? • If an arc is named XiXj/ZpZq then • if given inputs (XiXj) are 1( & other inputs would be don’t care), • then the specified outputs (ZpZq) are 1 (& the other outputs are zero). • If we have 4 inputs X1,X2,X3,X4 and 4 outputs Z1,Z2,Z3,Z4 then the label X1X4’/Z1Z3 is equivalent to 1- - 0/1010. (- is don’t care) • If we label an arc I, then the arc is traversed when I =1. • To have a completely specified proper state graph in which the next state is uniquely defined for every input combination the following conditions must be satisfied: 1. If Xi and Xj are any pair of input labels on arcs exiting state Sk, then XiXj=0 if i # j. This condition ensures that at most one condition is satisfied at any given point of time. 2. If n arcs exit state Sk and the n arcs have input labels X1,X2,….Xn then X1+X2+…..Xn =1. This ensures that at least one condition is satisfied. www.getmyuni.com A few examples illustrating the nomenclature in state graphs is presented in figures below. Fig. Partial state graph showing that conditions 1 &2 are satisfied for state Sk. Fig. a Fig. Partial State Graph (Fig. a above) and its state table row for Sk. www.getmyuni.com Serial adder with Accumulator Question: Illustrate the design of a control circuit for a serial adder with Accumulator Definition: A Control circuit in a digital system is a sequential network that outputs a sequence of control signals. These Control signals cause operations such as addition and shifting to take place at appropriate times. Block Diagram of a 4-bit Serial Adder with Accumulator The Operation of Serial Adder whose block diagram is given above is illustrated with the help of a table shown below. www.getmyuni.com State Graph for Control Circuit of the Serial Adder www.getmyuni.com Question: Draw the block diagram for a 16 bit serial adder with accumulator. • The control network uses a 4 bit counter which outputs k=1 when it is in state 1111. • When a start signal N is received, the registers should be loaded. Assume that N will remain 1 until the addition is complete. • When the addition is complete, the control network should go to a stop and remain there until N is changed to 0. • Draw a state diagram for the control network (excluding counter). Write the VHDL code. Fig. state diagram for the control network www.getmyuni.com Fig. Block diagram for a 16 bit serial adder with accumulator VHDL CODE for the 16 bit serial adder library IEEE; use IEEE.STD_LOGIC_1164.ALL; use IEEE.STD_LOGIC_ARITH.ALL; use IEEE.STD_LOGIC_UNSIGNED.ALL; --library UNISIM; --use UNISIM.VComponents.all; Entity seradder is Port (N,clk: in STD_LOGIC; A: in STD_LOGIC_vector(15 downto 0); R: out STD_LOGIC_vector(15 downto 0); B: in STD_LOGIC_vector(15 downto 0); Co:out STD_LOGIC); End seradder; Architecture mealy of seradder is Signal si,ci,cip,ld,sh: STD_LOGIC:='0'; Signal k:STD_LOGIC:='0'; Signal acc,regb: STD_LOGIC_vector(15 downto 0); Signal state: integer range 0 to 2:=0; Signal nxst: integer range 0 to 2:=0; Signal cnt: STD_LOGIC_vector(4 downto 0):="00000"; Begin K<='1' when (cnt="10000") else '0'; Process(clk) Begin www.getmyuni.com If (clk='1' and clk' event) then Case state is when 0=> if (N='1') then ld<='1';sh<='1';acc<=A; regb<=B;ci<='0'; state <=state+1; Else state<=0; end if; when 1 => if(k='1') then sh<='1'; state<= state+1;R<= acc; Co<=cip; else sh<='1'; acc<=si & acc(15 downto 1); regb<= regb(0) & regb(15 downto 1); Ci<=cip; cnt<=cnt+1; end if; when 2=> if(N='0') then state <=0; Else nxst<=state; end if; End case; End if; End process; Si<= acc(0) xor regb(0) xor ci; Cip<=( acc(0) and regb(0)) or (acc(0) and ci) or (regb(0) and ci); End mealy; www.getmyuni.com Binary Multiplier Multiplication of Two 4 bit numbers requires the following architecture • 4-bit multiplicand register • 4-bit multiplier register • 4-bit full adder • 8-bit product register which serves as an accumulator to accumulate the sum of partial products. Note: In the conventional adder Shifting multiplicand left every time would require an 8-bit Adder. Instead we shift the contents of the product register right each time The operation of the 4-bit binary multiplier shown in figure is elaborated in the below steps. • 4-bits from accumulator and 4-bits from multiplicand register are inputs to adder. • 4 sum bits and carry are connected back to accumulator. • When an Ad signal occurs, adder outputs are transferred to ACC at next clk. • Extra bit carries temporarily any carry that is generated. • Sh signal causes all 9 bits to be shifted right at next clk. • Multiplier is stored in lower 4 bits of ACC. • Control circuit outputs proper sequence of add and shift signals after the start signal St=1. www.getmyuni.com . Fig. Block Diagram of Binary Multiplier Multiplication Steps www.getmyuni.com Algorithm: 1. If current multiplier bit M (LSB of acc) is 1, multiplicand is added to accumulator and shifted right. 2. If M=0, addition is skipped and contents shifted right. The below figure briefly illustrates the contents of the binary multiplier for the example illustrated above. www.getmyuni.com Question: What should the control circuit of the multiplier do?? • Output proper sequence of Ad and Sh signals. • Start when S=1. • Load; Ld=1. • Check if M=1. If yes then make Ad=1 (to add). Then make Sh=1. • If M =0, then don’t change Ad to 1. Just make Sh=1. • A shift is always generated after Add operation. • A done signal generated after 4 shifts (indicating multiplication is complete). State Graph for Binary Multiplier Control www.getmyuni.com VHDL code for 4 X 4 Binary Multiplier The below code is a behavioral model of a multiplier for unsigned binary numbers. It multiplies a 4-bit multiplicand by a 4-bit multiplier to give an 8-bit product. The maximum number of clock cycles needed for a multiply is 10. library BITLIB; use BITLIB.bit_pack.all; entity mult4X4 is port (Clk, St: in bit; Mplier,Mcand : in bit_vector(3 downto 0); Done: out bit); end mult4X4; architecture behave1 of mult4X4 is signal State: integer range 0 to 9; signal ACC: bit_vector(8 downto 0); --accumulator alias M: bit is ACC(0); --M is bit 0 of ACC begin process begin wait until Clk = '1'; --executes on rising edge of clock case State is when 0=> --initial State if St='1' then ACC(8 downto 4) <= "00000"; --Begin cycle ACC(3 downto 0) <= Mplier; --load the multiplier State <= 1; end if; when 1 | 3 | 5 | 7 => --"add/shift" State if M = '1' then --Add multiplicand ACC(8 downto 4) <=add4(ACC(7 downto 4),Mcand,'0'); State <= State+1; else ACC <= '0' & ACC(8 downto 1); --Shift accumulator right State <= State + 2; end if; when 2 | 4 | 6 | 8 => --"shift" State ACC <= '0' & ACC(8 downto 1); --Right shift State <= State + 1; when 9 => State <= 0; end case; end process; www.getmyuni.com Done <= '1' when State = 9 else '0'; --End of cycle end behave1; Multiplier Control with Counter (a) Multiplier control using Counter (b) Partial State-graph for add-shift control (c) Final state graph for add – shift control (using counter output K) www.getmyuni.com Operation of Multiplier using a Counter Time State CounterProduct St M K Load Ad Sh Done Register t0 S0 00 000000000 0 0 0 0 0 0 0 t1 S0 00 000000000 1 0 0 1 0 0 0 t2 S1 00 000001011 0 1 0 0 1 0 0 t3 S2 00 011011011 0 1 0 0 0 1 0 t4 S1 01 001101101 0 1 0 0 1 0 0 t5 S2 01 100111101 0 1 0 0 0 1 0 t6 S1 10 010011110 0 0 0 0 0 1 0 t7 S1 11 001001111 0 1 1 0 1 0 0 t8 S2 11 100011111 0 1 1 0 0 1 0 t9 S3 00 010001111 0 1 0 0 0 0 1 VHDL code for the binary Multiplier (Control circuit with Counter) architecture behave2 of mult4X4 is signal ACC: bit_vector(8 downto 0); --accumulator alias M: bit is ACC(0); --M is bit 0 of ACC signal cnt: bit_vector(2 downto 0) ; begin process (st) begin if St='1' then cnt<= “000”; ACC(8 downto 4) <= "00000"; --Begin cycle ACC(3 downto 0) <= Mplier; --load the multiplier end if; end process; process (clk) begin while (cnt < “100”) loop if (clk=’1’ and clk’event) then if (M = '1' )then --Add multiplicand ACC(8 downto 4) <=add4(ACC(7 downto 4),Mcand,'0'); end if; ACC <= '0' & ACC(8 downto 1); --Shift accumulator right cnt<=cnt +’1’; end if; www.getmyuni.com end loop; end process; Done <=’1’when cnt=“100”; else “0”; end behave2; 4-bit Multiplier Partial Products Block diagram of 4X4 Array Multiplier www.getmyuni.com Operation • n bit x n bit multiplication would require n2 AND gates, n(n-2) full adders and n half-adders. • Number of components increases quadilaterally. • Longest path goes through 2n adders and the worst case multiply time is 2ntad+tg where tad delay through an adder and tg is the longest AND gate delay.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages37 Page
-
File Size-