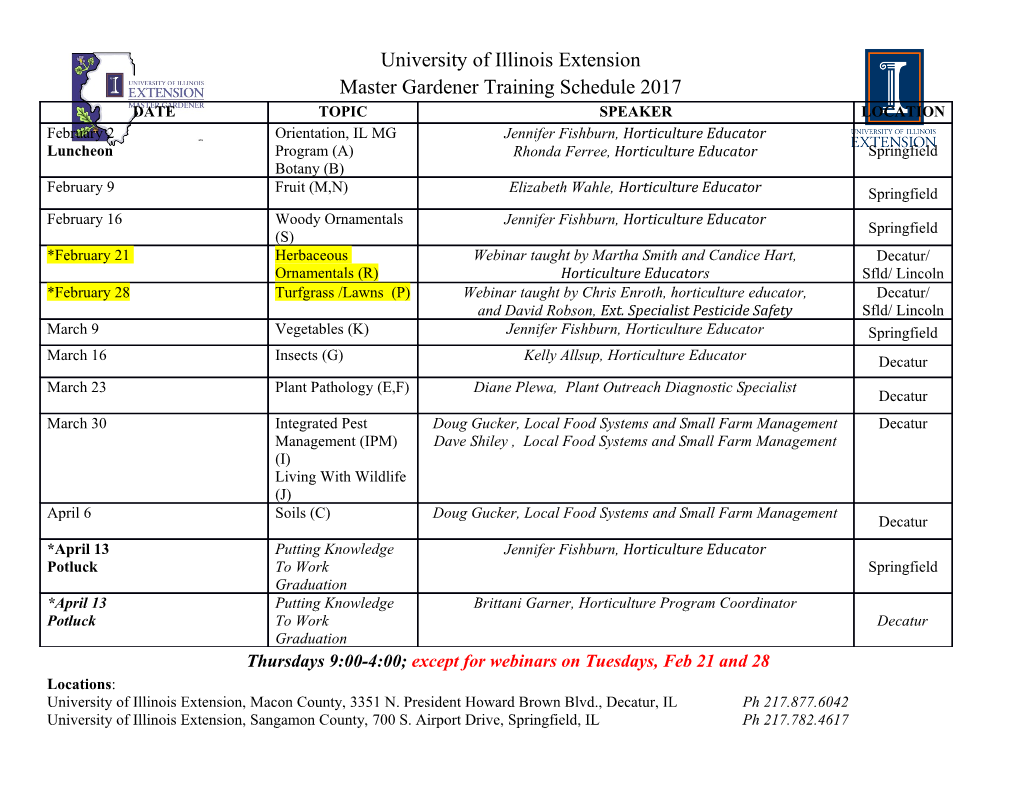
Computer Science 250 - Project 4 Recursive Exponentiation in MIPS Due: Fri. Dec. 6, at the beginning of class The end of this handout contains a C program that prompts the user to enter two non-negative numbers, and than raises the first number to the power of the second using the recursive definition of exponentiation that we discussed in class. The program uses only C operations that correspond to MIPS instructions that we are familiar with. Translate this program into a MIPS assembly language program that does the same thing. Here is the recursive definition of exponentiation you will be implement- ing. 8 1 b = 0 > b < b b a = a 2 · a 2 b even > b−1 b−1 :> a · a 2 · a 2 b odd Follow these implementation guidelines: • Write a MIPS procedure for each C-function. • Local variables are unchanged by function calls, so you should store any values that correspond to local variables in $s-registers. • Create an activation record for each function, if the function needs to spill preserved registers. What to turn in: When you are ready to turn your program in, send me an email with your assembler source code as an attachment. The subject of your email message should be <Lastname> - <Section Number> - CS 250 - Project 4. Then, print out a copy of the source to submit in class. 1 /* Prompt user for two non-negative integers. Raised the first to the power of the second and print it. */ #include <stdio.h> #include <stdlib.h> #define TRUE 1 #define FALSE 0 /* Is the parameter even? */ int is_even (int n); /* Return a*b. */ int multiply (int a, int b); /* Return a^b. */ int power (int base, int exponent); int main() { int base, exponent; printf ("Please enter non-negative base: "); scanf ("%d", &base); printf ("Please enter non-negative exponent: "); scanf ("%d", &exponent); if (base == 0 && exponent == 0) { printf ("Can't raise 0 to zero power.\n"); exit(0); } int result = power (base, exponent); printf ("%d^%d=%d\n", base, exponent, result); } /* Determine if a non-negative number is even by inspecting the least-significant bit. A leaf procedure. */ int is_even (int n) { if ((n & 0x1) == 0) { return TRUE; } return FALSE; 2 } /* Multiply the parameters together by repeated addition. A leaf procedure. */ int multiply (int a, int b) { int result = 0; while (a > 0) { result += b; a--; } return result; } /* Calculate b raised to the power of a recursively. */ int power (int b, int e) { int even_exponent, temp, result; /* Base case of the recursion: exponent is 0. */ if (e == 0) { return 1; } /* There are two recursive cases. First, determine whether the exponent is even or odd. */ even_exponent = is_even (e); if (even_exponent) { /* Even case. */ temp = power (b, e / 2); result = multiply (temp, temp); } else { /* Odd case. */ temp = power (b, (e - 1) / 2); result = multiply (b, temp); result = multiply (result, temp); } return result; } 3.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages3 Page
-
File Size-