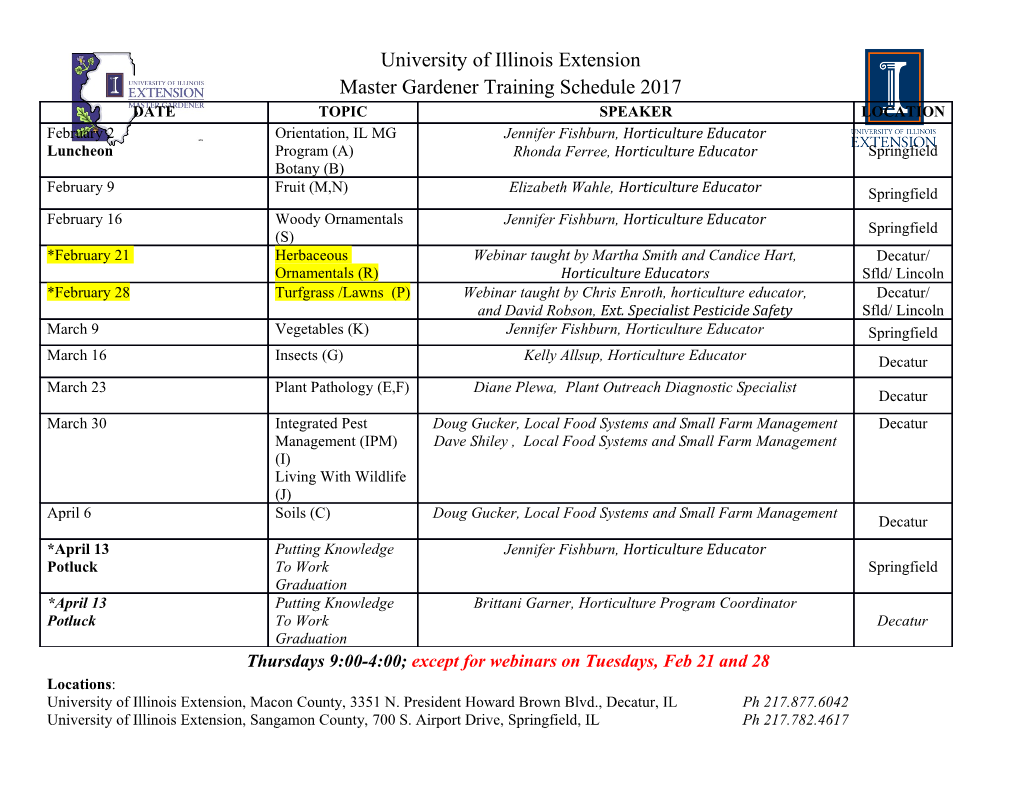
SLAC-PUB-5629 August 1991 ESTABLISHED 1962 (T/E/I) Object Oriented Programming* Paul F. Kunz Stanford Linear Accelerator Center Stanford University Stanford, California 94309, USA ABSTRACT This paper is an introduction to object oriented programming. The object oriented approach is very pow- erful and not inherently difficult, but most programmers find a relatively high threshold in learning it. Thus, this paper will attempt to convey the concepts with examples rather than explain the formal theory. 1.0 Introduction program is a collection of interacting objects that commu- nicate with each other via messaging. To the FORTRAN In this paper, the object oriented programming tech- programmer, a message is like a CALL to a subroutine. In niques will be explored. We will try to understand what it object oriented programming, the message tells an object really is and how it works. Analogies will be made with tra- what operation should be performed on its data. The word ditional programming in an attempt to separate the basic method is used for the name of the operation to be carried concepts from the details of learning a new programming out. The last key idea is that of inheritance. This idea can language. Most experienced programmers find there is a not be explained until the other ideas are better understood, relatively high threshold in learning the object oriented thus it will be treated later on in this paper. techniques. There are a lot of new words for which one An object is executable code with local data. This data needs to know the meaning in the context of a program; is called instance variables. An object will perform oper- words like object, instance variable, method, inheritance, ations on its instance variables. These operations are called etc. As one reads this paper, these words will be defined, methods. To clarify these concepts, consider the FORTRAN but the reader will probably not understand at that point the code in Fig. 1 . This is a strange way to write FORTRAN, but where and why of it all. Thus the paper is like a mystery it will serve to illustrate the key concepts. The style of cap- story, where we will not know who’s done it until the end. italization is that which is recommended for objective pro- My word of advice to the reader is to have patience and gramming, but for the moment is not important for the keep reading. discussion. For this sample code, the name of the object is The first key idea is that of an object. An object is re- anObject. The subroutine has two arguments. The first ar- ally nothing more than a piece of executable code with lo- gument, msg, is used as the message, while the second, I, is cal data. To the FORTRAN programmer, an object could be used as an input or output parameter. This object has one considered a subroutine with local variable declarations. instance variable with the name aValue which is of type By local, it is meant data that is neither in COMMON integer. There are two methods defined, setValue, and blocks, nor passed as an argument. This data is private to getValue. What operations are performed on the data is the subroutine. In object oriented parlance it is encapsu- defined in the FORTRAN statements. That is, if the value of lated. Encapsulation of data is one of the key concepts of the character string msg is “setValue” then the instance object oriented programming. The second key idea is that a variable aValue is set to the value of the argument I; when * Work supported by Department of Energy, contract DE-AC03-76SF00515. Invited lecture presented at the 1991 CERN School of Computing, Ystad, Sweden, 23 Aug. - 2 Sept. 1991. Object Oriented Programming Subroutine anObject( msg, I ) time to invent a new syntax. An example of such a new syn- Character msg*(*) tax is the Objective-C [1] language, which is derived from Integer I Integer*4 aValue the SmallTalk language. On most platforms, it is imple- If ( msg .eq. "setValue" ) then mented as a translator that generates C code that works aValue = I with a run time system to handle the messaging. Objective- return C as a language is a proper super-set of C. It adds only one ElseIf ( msg .eq. "getValue" ) then new data type, the object, and only one new operation, the I = aValue return message expression, to the base C language. Else An example of Objective-C code is given in Fig. 2 . print( "0Error" ) This Objective-C code is equivalent to the FORTRAN code EndIf shown in Fig. 1 . In the Objective-C syntax, the code is di- return vided into two parts. The first part is called the interface; it end @interface is all the code between the and the next @end. The interface part of the code serves two purposes. It Fig. 1 Sample FORTRAN code declares the number and type of instance variables, in this case only one, and it declares to what methods the object the string is “getValue” then the current value of the in- will respond. The interface is usually placed in a separate stance variable is returned via I. file, then included via the standard C include mechanism. To send a message to anObject from some other Once again, the author can only say that the reasons for do- FORTRAN routine, one might find code fragments that look ing this are certainly not apparent at this time, but will be like explained later. The second part of the code is the imple- mentation; this is all the code between the @implemen- Call anObject("setValue", 2) tation @end Call anObject("getValue", I) and the next . Within the implementation, one writes the code for all the methods that make up the ob- In the first line, anObject will set its instance variable ject. Each method begins with a “–” and the name of the to value 2, while in the second line, the current value of the method. Between the braces (“{}”) can be any amount of instance variable will be returned into the argument I. plain C code, including calls to C functions, and message Now the reader should have some of the key concepts expressions. Even calls to other compiled languages, such understood, at least in their simplest sense. The variables that are local to a FORTRAN subroutine, that is, neither in COMMON nor passed as parameters, are encapsulated. The #import <objc/Object.h> data is protected from being changed or accessed by an- @interface anObject:Object { other routine. It is this that makes it an object. An object has int aValue; boundaries and clearly limits the accessibility of the vari- } ables in the routine. A FORTRAN COMMON on the other - setValue:(int) i; hand, has no boundaries. It is more like a fog. It spreads out - (int) getValue; from where you are and you don’t know where it ends. You @end also don’t know what else might be in the fog; or what you @implementation anObject might run into. - setValue:(int) i Why we are programming this way is probably not yet { apparent; that will come later. But for now, the reader aValue = i; should note the very different style of manipulating data. In return self; } languages like FORTRAN, we think of passing data to a rou- - (int) getValue tine, via arguments or COMMON blocks. Here the routine, { i.e. the object, holds the data as instance variables and we return aValue; change or retrieve the data via methods implemented for } the object. @end This messaging style of programming is a rather te- dious way to get to the data that we want to operate on. Its Fig. 2 Objective-C example 2 SLAC-PUB-5629 Object Oriented Programming as FORTRAN can be placed here. The example in Fig. 2 ad- may not be familiar with this language, or even the C lan- mittedly doesn’t show very much of that possibility. guage. As an aid in following the text, the author offers Ta- To send a message to the above object, from another ble 1 ., which crudely shows the correspondence between object, one might find the following code fragments the Objective-C and C languages and FORTRAN. Of special note is the use of colons (“:”) in the method names. In the id anObject; first instance above, the colon seems to be a separator be- int i; . tween the method name and the parameter; which it does [ anObject setValue: 2 ]; except that the colon is also considered part of the method i = [ anObject getValue ]; name. In the second instance in the table, there are two co- lons, each separating the method name from the parame- In these fragments, anObject is declared to be data of type ters. The full name of the method contains the two colons as object, while i is declared to be type integer. The message shown by the pseudo-FORTRAN code: setLow:high:. expression is signaled by an expression starting with the This is the style of the SmallTalk language and it is done left bracket (“[”) and ending with the right bracket (“]”). that way for readability. It is very upsetting to FORTRAN The syntax may seem strange to a FORTRAN programmer, programmers, but once one gets used to it, one begins to ap- or even a C programmer; it comes from the SmallTalk lan- preciate its self describing value. guage. There is a lot more behind it then can be understood So far, we’ve introduced a lot of new terms and a very now, so the reader would do best by not questioning it at different syntax.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages19 Page
-
File Size-