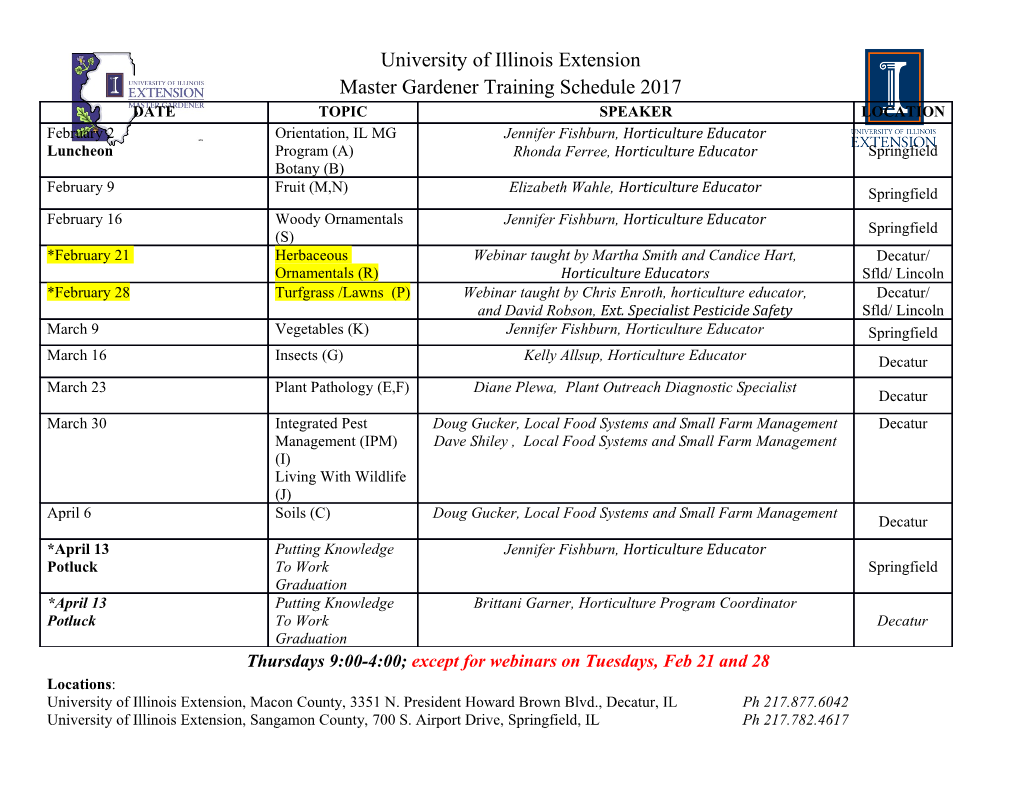
Call Stack Stack “Bottom” Memory region managed with stack discipline %rsp holds lowest stack address higher Procedures and the Call Stack addresses (address of "top" element) Topics stack grows • Procedures toward lower addresses • Call stack Stack Pointer: %rsp • Procedure/stack instructions • Calling conventions • Register-saving conventions Stack “Top” 9 Stack frames support procedure calls. Procedure Control Flow Instructions Contents Caller Local variables Procedure call: callq label Frame Function arguments (after first 6) 1. Push return address on stack Return information 2. Jump to label Temporary space Frame for Return address: Address of instruction after . Example: current call procedure Management 400544: callq 400550 <mult2> %rsp Space allocated when procedure is entered 400549: movq %rax,(%rbx) Stack Pointer “Setup” code Space deallocated before return Stack “Top” “Finish” code Procedure return: retq Why not just give every procedure a permanent 1. Pop return address from stack chunk of memory to hold its local variables, etc? 2. Jump to address 26 28 Call Example (step 1) Return Example (step 1) • • 0x130 0x130 0000000000400540 <multstore>: • 0000000000400540 <multstore>: • • 0x128 • • 0x128 • • 0x120 • 0x120 400544: callq 400550 <mult2> 400544: callq 400550 <mult2> 400549: mov %rax,(%rbx) 400549: mov %rax,(%rbx) 0x118 0x400549 • • • %rsp 0x120 • %rsp 0x118 %rip 0x400544 %rip 0x400557 0000000000400550 <mult2>: 0000000000400550 <mult2>: 400550: mov %rdi,%rax 400550: mov %rdi,%rax • • • • 400557: retq 400557: retq 29 31 Procedure Data Flow Stack Frame First 6 arguments passed Remaining arguments passed in registers on stack (in memory) High … Addresses Arg 1 %rdi Diane’s • • • Caller %rsi Silk Frame Dress Arg n Extra Arguments %rdx Costs to callee %rcx $8 9 • • • Return Address %r8 Arg 8 Arg 6 %r9 Arg 7 Saved Registers Return value + Low Local Variables Callee %rax Addresses Frame Only allocate stack space when needed Stack pointer %rsp Extra Arguments for next call 35 Example: increment Procedure Call Example (initial state) Initial Stack Structure long increment(long* p, long val) { long call_incr() { long x = *p; long v1 = 240; long y = x + val; long v2 = increment(&v1, 61); • • • *p = y; return v1+v2; return x; } %rsp Return addr <main+8> } call_incr: subq $16, %rsp increment: %rdi Register Use(s) movq $240, 8(%rsp) movq (%rdi), %rax movl $61, %esi Argument addq %rax, %rsi %rdi p leaq 8(%rsp), %rdi movq %rsi, (%rdi) %rsi Argument val, y call increment %rsi ret %rax x, Return value addq 8(%rsp), %rax addq $16, %rsp ret %rax 37 38 Procedure Call Example (step 4) Procedure Call Example (step 6) long call_incr() { Stack Structure Stack Structure longlongincrement( v1 = 240;long* p, long val) { long call_incr() { longlong v2 x= =increment( *p; &v1, 61); long v1 = 240; returnlong v1+v2;y = x + val; • • • long v2 = increment(&v1, 61); • • • *p = y; } return v1+v2; return x; %rsp } %rsp call_incr} : Return addr <main+8> Return addr <main+8> subq $16, %rsp v1 in call_incr à 301 v1 in call_incr à 301 movq $240, 8(%rsp) Unused Unused movl $61, %esi leaq 8(%rsp), %rdi Return addr <call_incr+?> call_incr: call increment subq $16, %rsp %rdi %rdi addq 8(%rsp), %rax movq $240, 8(%rsp) addq $16, %rsp &v1 movl $61, %esi &v1 ret leaq 8(%rsp), %rdi %rsi call increment %rsi increment: 301 addq 8(%rsp), %rax Update %rax: v1+v2 301 movq (%rdi), %rax # x = *p addq $16, %rsp addq %rax, %rsi # y = x+61 %rax ret %rax movq %rsi, (%rdi) # *p = y 240 541 ret 42 44 A Puzzle Register Saving Conventions C function body: *p = d; Write the C function yoo calls who: return x - c; header, types, and Caller Callee order of parameters. assembly: Will register contents still be there after a procedure call? movsbl %dl,%edx yoo: who: movl %edx,(%rsi) • • • • • • movq $12345, %rbx addq %rdi, %rbx movswl %di,%edi call who ? • • • subl %edi,%ecx addq %rbx, %rax ret • • • movl %ecx,%eax ret Conventions: Caller Save movsbl = move sign-extending a byte to a long (4-byte) Callee Save movswl = move sign-extending a word (2-byte) to a long (4-byte) 49 x86-64 64-bit Register Conventions Callee-Saved Example Begin/End Stack Structure long call_incr2(long x) { %rax Return value – Caller saved %r8 Argument #5 – Caller saved long v1 = 240; long v2 = increment(&v1, 61); . %rbx Callee saved %r9 Argument #6 – Caller saved return x+v2; } Rtn address %rsp %rcx Argument #4 – Caller saved %r10 Caller saved call_incr2: %rdx Argument #3 – Caller saved %r11 Caller Saved pushq %rbx Stack Structure in call_incr2 subq $16, %rsp %rsi Argument #2 – Caller saved %r12 Callee saved movq %rdi, %rbx movq $240, 8(%rsp) . %rdi Argument #1 – Caller saved %r13 Callee saved movl $61, %esi leaq 8(%rsp), %rdi Rtn address %rsp Stack pointer %r14 Callee saved call increment addq %rbx, %rax Saved %rbx addq $16, %rsp %rbp Callee saved %r15 Callee saved 240 %rsp+8 popq %rbx ret Unused %rsp 51 52 x86-64 stack storage example Recursive Function pcount_r: (1) movl $0, %eax /* Recursive popcount */ testq %rdi, %rdi long int call_proc() call_proc: long pcount_r(unsigned long x) { je .L6 { subq $32,%rsp if (x == 0) { pushq %rbx long x1 = 1; movq $1,16(%rsp) # x1 return 0; movq %rdi, %rbx int x2 = 2; movl $2,24(%rsp) # x2 } else { andl $1, %ebx short x3 = 3; movw $3,28(%rsp) # x3 return (x & 1) shrq %rdi char x4 = 4; movb $4,31(%rsp) # x4 + pcount_r(x >> 1); call pcount_r proc(x1, &x1, x2, &x2, • • • } addq %rbx, %rax x3, &x3, x4, &x4); } popq %rbx return (x1+x2)*(x3-x4); .L6: } rep; ret Return address to caller of call_proc ←%rsp 54 61 Procedure Summary call, ret, push, pop Stack discipline fits procedure call / return.* … If P calls Q: Q (and calls by Q) returns before P Caller Conventions support arbitrary function calls. Frame Extra Arguments Register-save conventions. to callee Stack frame saves extra args or local variables. Return Address Result returned in %rax %rax Return value – Caller saved %r8 Argument #5 – Caller saved %rbx Callee saved %r9 Argument #6 – Caller saved Saved Registers + %rcx Argument #4 – Caller saved %r10 Caller saved Callee Local Variables %rdx Argument #3 – Caller saved %r11 Caller Saved Frame %rsi Argument #2 – Caller saved %r12 Callee saved %rdi Argument #1 – Caller saved %r13 Callee saved Extra Arguments %rsp Stack pointer %r14 Callee saved Stack pointer %rsp for next call %rbp Callee saved %r15 Callee saved 128-byte red zone *Take 251 to learn about languages where it doesn't. 66.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-