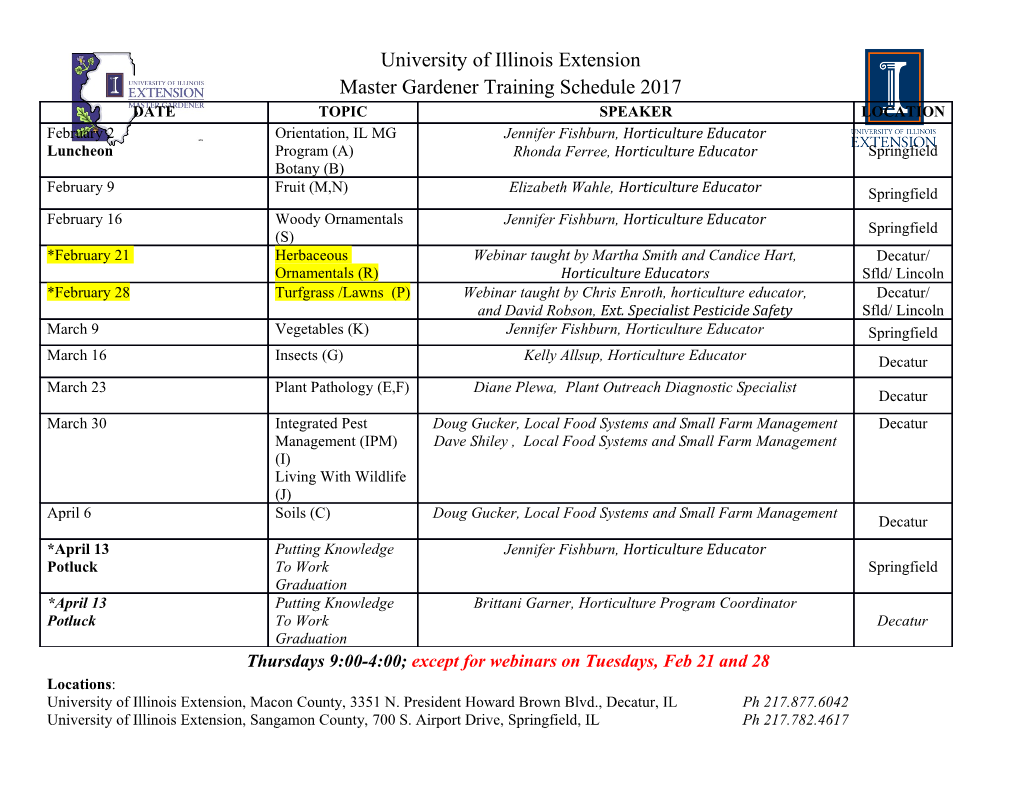
Introduction to Standard C++ Lecture 04: Template Functions and Template Classes Massimiliano Culpo1 1CINECA - SuperComputing Applications and Innovation Department 07.04.2014 M.Culpo (CINECA) Introduction to C++ 07.04.2014 1 / 26 Templates Table of contents - Language rules 1 Templates Template declarations Function templates Class templates Name resolution 2 Summary 3 Bibliography M.Culpo (CINECA) Introduction to C++ 07.04.2014 2 / 26 Templates Template declarations Table of contents - Language rules 1 Templates Template declarations Function templates Class templates Name resolution 2 Summary 3 Bibliography M.Culpo (CINECA) Introduction to C++ 07.04.2014 3 / 26 Templates Template declarations Template declarations (x14) A template defines a family of classes or functions: template <typenameT > T const& max (T const& a , T const& b) f // ifa < b then useb else usea returna < b ? b : a ; g 1 A template declaration shall: declare or define a function or a class define a member function, a member class or a static data member define a member template of a class or class template 2 A template declaration is also a definition if its declaration defines a function or a class M.Culpo (CINECA) Introduction to C++ 07.04.2014 4 / 26 Templates Template declarations Template parameters (x14.1) template<constX & x , i n ti > voidf () f i ++; // error: can't assign or modify &x ; // OK &i ; // error: can't take the address i n t& r i = i ; // error const int& c r i = i ; // OK g 1 There are three kinds of allowed template parameters: non-type template parameters type template parameters template template parameters 2 A non-type template parameter shall be either: an integral or enumeration type a pointer/reference to object or function M.Culpo (CINECA) Introduction to C++ 07.04.2014 5 / 26 Templates Template declarations Template parameters (x14.1) template<c l a s sK , c l a s sV > c l a s s Map f std :: vector<K> key ; std :: vector<V> value ; g ; template<c l a s sK , c l a s sV , template<c l a s sT > c l a s sC > c l a s s Map f C<K> key ; C<V> value ; g ; 1 The keyword class names a type parameter 2 Template classes may also be used as template parameters M.Culpo (CINECA) Introduction to C++ 07.04.2014 6 / 26 Templates Template declarations Template parameters (x14.1) A default argument may be specified for any kind of template parameter: template<c l a s s T1, c l a s s T2 = int > c l a s sA ; template <c l a s sT = f l o a t > s t r u c tB f g ; template <template <c l a s s TT = f l o a t > c l a s sT > s t r u c tA f i n l i n e voidf (); i n l i n e voidg (); g ; template <template <c l a s s TT> c l a s sT > voidA <T>:: f () f T<> t ; g // error template <template <c l a s s TT = char> c l a s sT > voidA <T>:: g () f T<> t ; g // OK − T<char> M.Culpo (CINECA) Introduction to C++ 07.04.2014 7 / 26 Templates Function templates Table of contents - Language rules 1 Templates Template declarations Function templates Class templates Name resolution 2 Summary 3 Bibliography M.Culpo (CINECA) Introduction to C++ 07.04.2014 8 / 26 Templates Function templates Function templates (x14.5.6) A function template defines an unbounded set of related functions: // sort.hxx template<c l a s sT > void sort ( T& input ); The mere use of a function template can trigger an instantiation: #include "sort.hxx" std :: vector< double > vec ; s o r t ( vec ); A function template can be overloaded: 1 with other function templates 2 with normal functions M.Culpo (CINECA) Introduction to C++ 07.04.2014 9 / 26 Templates Function templates Overloading of function templates /∗ Maximum of two ints ∗/ i n t const& max ( i n t const& a , i n t const& b ); /∗ Maximum of two values of any type ∗/ template <typenameT > T const& max (T const& a , T const& b ); /∗ Maximum of three values of any type ∗/ template <typenameT > T const& max (T const& a , T const& b , T const& c ); i n t main () f :: max( 7 , 42 , 6 8 ) ; // template for three arguments :: max (7.0, 42.0); // max<double> :: max('a','b'); // max<char> :: max( 7 , 4 2 ) ; // non−template for two ints :: max<>(7, 4 2 ) ; // max<int > :: max<double >(7 , 4 2 ) ; // max<double> :: max('a', 42.7); // non−template for two ints g M.Culpo (CINECA) Introduction to C++ 07.04.2014 10 / 26 Templates Class templates Table of contents - Language rules 1 Templates Template declarations Function templates Class templates Name resolution 2 Summary 3 Bibliography M.Culpo (CINECA) Introduction to C++ 07.04.2014 11 / 26 Templates Class templates Class templates (x14.5.1) A class template defines an unbounded set of related types: template<c l a s sT > c l a s s Array f T∗ v ; i n t sz ; p u b l i c : e x p l i c i t Array ( i n t ); T& operator []( i n t ); T& elem ( i n ti ) f r e t u r nv [ i ]; g g ; A member function may be defined outside the class in which it is declared: template<c l a s sT > T& Array<T>:: operator []( i n ti ) f i f ( i <0 j j sz<=i ) e r r o r ("Array: range error"); r e t u r nv [ i ]; g M.Culpo (CINECA) Introduction to C++ 07.04.2014 12 / 26 Templates Class templates Class templates (x14.5.1) Similar rules apply to class member: template<c l a s sT > s t r u c tA f c l a s sB ; g ; A<int >::B∗ b1 ; template<c l a s sT > c l a s sA <T>::B f g ; A<int >::B b2 ; For a member function of a class template: Array<int > v1 ( 2 0 ) ; Array<dcomplex> v2 ( 3 0 ) ; // Array<int >::operator[]() v1 [ 3 ] = 7 ; // Array<dcomplex >::operator[]() v2 [ 3 ] = dcomplex ( 7 , 8 ) ; the template arguments are determined by the type of the object for which the member function is called. M.Culpo (CINECA) Introduction to C++ 07.04.2014 13 / 26 Templates Class templates Member template (x14.5.2) A template can be declared within a class or class template: template<c l a s sT > s t r u c t string f template<c l a s s T2> i n t compare ( const T2&); template<c l a s s T2> s t r i n g ( const string <T2>& s ) f /∗ ... ∗/ g g ; template<c l a s sT > template<c l a s s T2> i n t string <T>:: compare ( const T2& s ) f /∗ ... ∗/ g M.Culpo (CINECA) Introduction to C++ 07.04.2014 14 / 26 Templates Class templates Member template (x14.5.2) template <c l a s sT > s t r u c t AA f template <c l a s sC > v i r t u a l voidg (C); // error g ; c l a s sB f v i r t u a l voidf ( i n t ); g ; c l a s sD : p u b l i cB f template <c l a s sT > voidf (T); // no override voidf ( i n ti ) f f <>( i ); g // overriding g ; 1 A local class shall not have member templates 2 A member function template: shall not be virtual does not override a virtual function M.Culpo (CINECA) Introduction to C++ 07.04.2014 15 / 26 Templates Class templates Class template partial specialization (x14.5.5) template<c l a s s T1, c l a s s T2, i n tI > c l a s sA f g ; // Primary class template template<c l a s sT , i n tI > c l a s sA <T, T∗ , I> f g ; // #1 template<c l a s s T1, c l a s s T2, i n tI > c l a s sA <T1∗ , T2, I> f g ; // #2 1 A class template may be partially specialized 2 Each class template partial specialization is a distinct template 3 Definitions shall be provided for its members M.Culpo (CINECA) Introduction to C++ 07.04.2014 16 / 26 Templates Name resolution Table of contents - Language rules 1 Templates Template declarations Function templates Class templates Name resolution 2 Summary 3 Bibliography M.Culpo (CINECA) Introduction to C++ 07.04.2014 17 / 26 Templates Name resolution The typename keyword (x14.6) The keyword typename clarifies that an identifier is a type: template <c l a s sT > c l a s sA f typenameT :: SubType ∗ ptr ; /∗ ... ∗/ g ; Without typename, the expression: T :: SubType ∗ ptr ; would be a multiplication of a static member of class T with ptr. In general, typename has to be used whenever a name that depends on a template parameter is a type. M.Culpo (CINECA) Introduction to C++ 07.04.2014 18 / 26 Templates Name resolution The .template construct (x14.6) Consider the following example: template <c l a s sT > c l a s sC f p u b l i c : template<c l a s sU > s t r i n g i f y (); g template<i n tN > void print (C<N> const& bs ) f cout << bs .
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages26 Page
-
File Size-