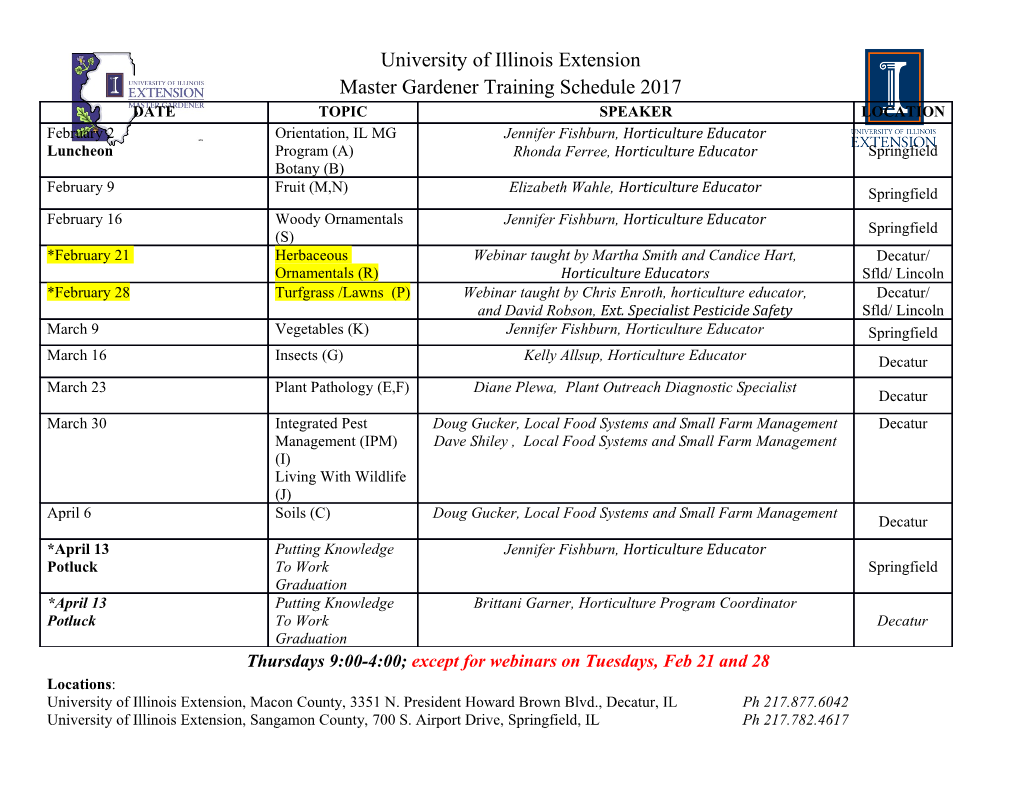
Debugging with DDD User’s Guide and Reference Manual First Edition, for DDD Version 3.2 Last updated 2000-01-03 Andreas Zeller Debugging with DDD User’s Guide and Reference Manual Copyright c 2000 Universität Passau Lehrstuhl für Software-Systeme Innstraße 33 D-94032 Passau GERMANY Distributed by Free Software Foundation, Inc. 59 Temple Place – Suite 330 Boston, MA 02111-1307 USA ddd and this manual are available via the ddd www page. Permission is granted to make and distribute verbatim copies of this manual provided the copyright notice and this permission notice are preserved on all copies. Permission is granted to copy and distribute modified versions of this manual under the conditions for verbatim copying, provided also that the sections entitled “Copying” and “GNU General Public License” (see Appendix G [License], page 181) are included exactly as in the original, and provided that the entire resulting derived work is distributed under the terms of a permission notice identical to this one. Permission is granted to copy and distribute translations of this manual into another language, under the above conditions for modified versions, except that this permission notice may be stated in a translation approved by the Free Software Foundation. Send questions, comments, suggestions, etc. to [email protected]. Send bug reports to [email protected]. i Short Contents Summary of DDD .............................................. 1 1 A Sample DDD Session ...................................... 5 2 Getting In and Out of DDD ................................... 15 3 The DDD Windows ........................................ 39 4 Navigating through the Code .................................. 71 5 Stopping the Program ....................................... 79 6 Running the Program ....................................... 89 7 Examining Data .......................................... 103 8 Machine-Level Debugging ................................... 137 9 Changing the Program ...................................... 141 10 The Command-Line Interface ................................. 143 Appendix A Application Defaults ................................ 155 Appendix B Bugs and How To Report Them ......................... 165 Appendix C Configuration Notes ................................. 171 Appendix D Dirty Tricks ...................................... 175 Appendix E Extending DDD ................................... 177 Appendix F Frequently Answered Questions ......................... 179 Appendix G GNU General Public License ........................... 181 Appendix H Help and Assistance ................................. 189 Label Index ................................................ 191 Key Index ................................................. 195 Command Index ............................................. 197 Resource Index .............................................. 199 File Index .................................................. 203 Concept Index ............................................... 205 ii Debugging with DDD iii Table of Contents Summary of DDD .......................................... 1 About this Manual .................................................. 2 Free software ....................................................... 2 Getting DDD ....................................................... 2 Contributors to DDD ................................................ 3 History of DDD ..................................................... 3 1 A Sample DDD Session ................................... 5 1.1 Sample Program .............................................. 14 2 Getting In and Out of DDD .............................. 15 2.1 Invoking DDD ............................................... 15 2.1.1 Choosing an Inferior Debugger ...................... 15 2.1.2 DDD Options....................................... 16 2.1.3 X Options .......................................... 24 2.1.4 Inferior Debugger Options........................... 24 2.1.4.1 GDB Options ............................. 24 2.1.4.2 DBX and Ladebug Options ................ 25 2.1.4.3 XDB Options ............................. 25 2.1.4.4 JDB Options .............................. 25 2.1.4.5 PYDB Options ............................ 26 2.1.4.6 Perl Options .............................. 27 2.1.5 Multiple DDD Instances............................. 27 2.1.6 X warnings ......................................... 27 2.2 Quitting DDD ................................................ 27 2.3 Persistent Sessions............................................ 28 2.3.1 Saving Sessions..................................... 28 2.3.2 Resuming Sessions.................................. 29 2.3.3 Deleting Sessions ................................... 30 2.3.4 Customizing Sessions ............................... 31 2.4 Remote Debugging ........................................... 31 2.4.1 Running DDD on a Remote Host .................... 31 2.4.2 Using DDD with a Remote Inferior Debugger ........ 31 2.4.2.1 Customizing Remote Debugging ........... 32 2.4.3 Debugging a Remote Program ....................... 33 2.5 Customizing Interaction with the Inferior Debugger ............ 33 2.5.1 Invoking an Inferior Debugger ....................... 33 2.5.2 Initializing the Inferior Debugger .................... 34 2.5.2.1 GDB Initialization ........................ 34 2.5.2.2 DBX Initialization ........................ 35 2.5.2.3 XDB Initialization ........................ 35 2.5.2.4 JDB Initialization ......................... 35 iv Debugging with DDD 2.5.2.5 PYDB Initialization ....................... 36 2.5.2.6 Perl Initialization.......................... 36 2.5.2.7 Opening the Selection ..................... 36 2.5.3 Communication with the Inferior Debugger .......... 36 3 The DDD Windows ..................................... 39 3.1 The Menu Bar ................................................ 39 3.1.1 The File Menu ...................................... 40 3.1.2 The Edit Menu...................................... 41 3.1.3 The View Menu..................................... 42 3.1.4 The Program Menu ................................. 43 3.1.5 The Commands Menu............................... 44 3.1.6 The Status Menu.................................... 45 3.1.7 The Source Menu ................................... 45 3.1.8 The Data Menu ..................................... 46 3.1.9 The Maintenance Menu ............................. 47 3.1.10 The Help Menu.................................... 48 3.1.11 Customizing the Menu Bar ......................... 48 3.1.11.1 Auto-Raise Menus ....................... 48 3.1.11.2 Customizing the Edit Menu............... 49 3.2 The Tool Bar ................................................. 49 3.2.1 Customizing the Tool Bar ........................... 51 3.3 The Command Tool........................................... 53 3.3.1 Customizing the Command Tool ..................... 55 3.3.1.1 Disabling the Command Tool .............. 55 3.3.2 Command Tool Position............................. 56 3.3.2.1 Customizing Tool Decoration .............. 57 3.4 Getting Help ................................................. 57 3.5 Undoing and Redoing Commands ............................. 58 3.6 Customizing DDD ............................................ 58 3.6.1 How Customizing DDD Works ...................... 58 3.6.1.1 Resources................................. 58 3.6.1.2 Changing Resources....................... 59 3.6.1.3 Saving Options............................ 59 3.6.2 Customizing DDD Help ............................. 59 3.6.2.1 Button Tips ............................... 59 3.6.2.2 Tip of the day ............................. 60 3.6.2.3 Help Helpers.............................. 60 3.6.3 Customizing Undo .................................. 61 3.6.4 Customizing the DDD Windows ..................... 62 3.6.4.1 Splash Screen ............................. 62 3.6.4.2 Window Layout ........................... 63 3.6.4.3 Customizing Fonts ........................ 64 3.6.4.4 Toggling Windows ........................ 66 3.6.4.5 Text Fields ................................ 67 3.6.4.6 Icons ..................................... 67 3.6.4.7 Adding Buttons ........................... 68 3.6.4.8 More Customizations...................... 68 v 3.6.5 Debugger Settings .................................. 68 4 Navigating through the Code ............................. 71 4.1 Compiling for Debugging ..................................... 71 4.2 Opening Files ................................................ 71 4.2.1 Opening Programs .................................. 71 4.2.2 Opening Core Dumps ............................... 72 4.2.3 Opening Source Files ............................... 72 4.2.4 Filtering Files....................................... 73 4.3 Looking up Items ............................................. 73 4.3.1 Looking up Definitions.............................. 73 4.3.2 Textual Search ...................................... 74 4.3.3 Looking up Previous Locations ...................... 74 4.3.4 Specifying Source Directories ....................... 74 4.4 Customizing the Source Window .............................. 75 4.4.1 Customizing Glyphs ................................ 76 4.4.2 Customizing Searching.............................. 77 4.4.3 Customizing Source Appearance..................... 77 4.4.4 Customizing Source Scrolling ....................... 78 4.4.5 Customizing Source Lookup......................... 78 4.4.6 Customizing File Filtering........................... 78 5 Stopping
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages222 Page
-
File Size-