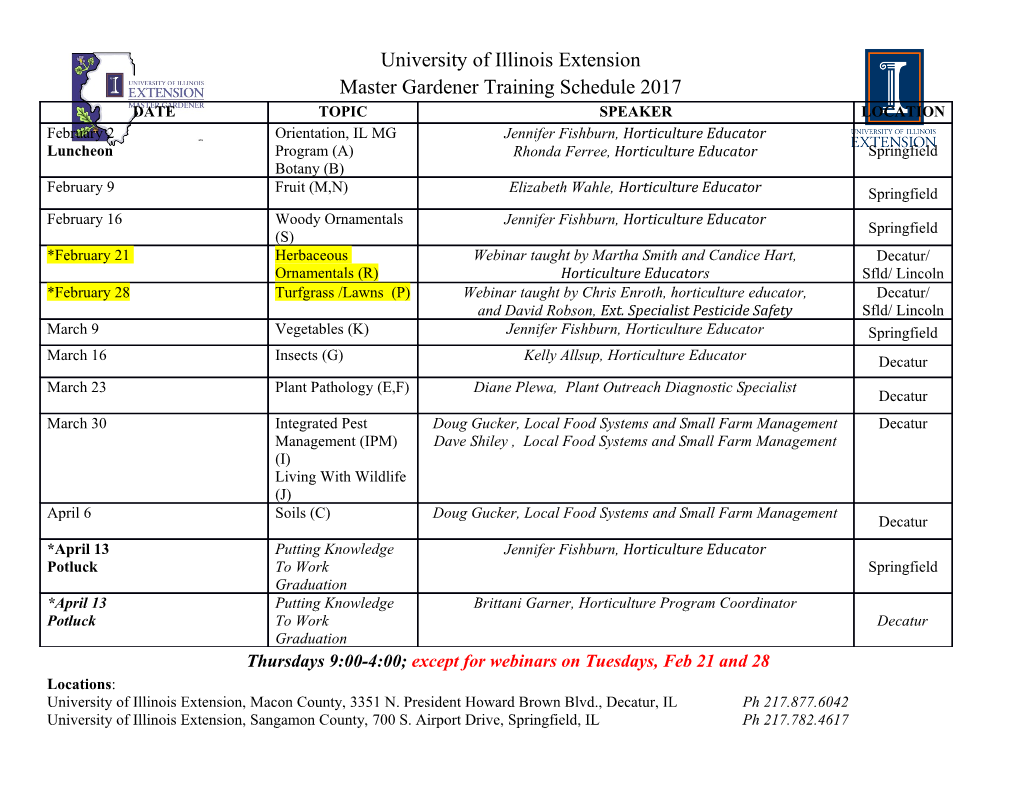
DEGREE PROJECT IN TECHNOLOGY, FIRST CYCLE, 15 CREDITS STOCKHOLM, SWEDEN 2017 An approach of using Delaunay refinement to mesh continuous height fields NOAH TELL ANTON THUN KTH ROYAL INSTITUTE OF TECHNOLOGY SCHOOL OF COMPUTER SCIENCE AND COMMUNICATION An approach of using Delaunay refinement to mesh continuous height fields NOAH TELL ANTON THUN Degree Programme in Computer Science Date: June 5, 2017 Supervisor: Alexander Kozlov Examiner: Örjan Ekeberg Swedish title: En metod att använda Delaunay-raffinemang för att skapa polygonytor av kontinuerliga höjdfält School of Computer Science and Communication Abstract Delaunay refinement is a mesh triangulation method with the goal of generating well-shaped triangles to obtain a valid Delaunay triangulation. In this thesis, an approach of using this method for meshing continuous height field terrains is presented using Perlin noise as the height field. The Delaunay approach is compared to grid-based meshing to verify that the theoretical time complexity O(n log n) holds and how accurately and deterministically the Delaunay approach can represent the height field. However, even though grid-based mesh generation is faster due to an O(n) time complexity, the focus of the report is to find out if De- launay refinement can be used to generate meshes quick enough for real-time applications. As the available memory for rendering the meshes is limited, a solution for providing a co- hesive mesh surface is presented using a hole filling algorithm since the Delaunay approach ends up leaving gaps in the mesh when a chunk division is used to limit the total mesh count present in the application. The methods were implemented in the programming language C++ using the open source library libnoise to generate the Perlin noise and the off-the-shelf solution CGALmesh provided a Delaunay refinement implementation. The video game en- gine Unity was used to render the output meshes created by the Delaunay and grid approach by interfacing with C++ via a Windows DLL. The time complexity of Delaunay refinement was verified to hold, although it was not possi- ble to draw any conclusions regarding the Delaunay refinement’s impact on the mesh’s accu- racy due to the test parameters used. It was also found that the CGALmesh implementation failed to provide a deterministic generation which is a significant drawback compared to the grid-based approach. Disregarding this, the Delaunay approach was found to be suitable for real-time applications as the generation time took less than 1 second, and is promising for volumetric terrain mesh generation. ii Sammanfattning Delaunay-raffinemang är en trianguleringsmetod med målet att generera reguljära trianglar för att uppnå en giltig Delaunay-triangulering. I denna avhandling presenteras en metod användandes Delaunay-raffinemang för att skapa polygonytor av kontinuerliga höjdfälts- terränger, där Perlin noise används som höjdfält. Delaunay-metoden jämförs med en rut- nätsbaserad metod för att verifiera att tidskomplexiteten O(n log n) gäller och hur exakt och deterministiskt som Delaunay-metoden förhåller sig till att representera höjdfältet. Även fast rutnätsmetoden är snabbare på grund av en O(n) tidskomplexitet är rapportens fokus att ta reda på om Delaunay-raffinemang är snabb nog för att användas i realtidsapplikationer för att generera polygonytor. Eftersom det tillgängliga minnet för att rendera polygonytorna är begränsat presenteras en lösning för att få sammanhängande ytor genom en hålutfyllningsalgoritm då Delaunay- metoden lämnar hål i ytan när chunk-uppdelning används för att begränsa det totala an- talet polygonytor i applikationen. Metoderna implementerades i programmeringsspråket C++ användades biblioteket libnoise för att generera Perlin noise och den färdiga lösning- en CGALmesh användes som implementation av Delaunay-raffinemang. Datorspelsmotorn Unity användes för att rendera polygonytorna som skapades av Delaunay- och rutnätsme- toden genom ett C++-gränssnitt via en Windows DLL. Tidskomplexiteten av Delaunay-raffinemang gällde, men det var inte möjligt att dra några slutsatser gällande hur exakt metoden förhållde sig till höjdfältet på grund av testparamet- rarna som användes. Ytterligare visade det sig att CGALmesh-implementationen var oför- mögen att deterministiskt generera ytorna vilket är en stor nackdel jämfört med rutnätsmeto- den. Bortsett från detta så visade sig Delaunay-metoden användbar för realtidsapplikationer då generingstiden tog mindre än 1 sekund, och metoden har dessutom potential för voly- metrisk terränggenerering. iii Contents 1 Introduction 1 1.1 Purpose . .1 1.2 Problem statement . .2 1.3 Scope and constraints . .2 1.4 Disposition . .2 1.5 Terminology . .3 2 Background 4 2.1 Height field generation . .4 2.1.1 Gradient noise . .4 2.1.2 Perlin noise . .4 2.2 Delaunay-based mesh generation . .5 2.2.1 Delaunay triangulation . .5 2.2.2 Restricted Delaunay refinement . .6 2.2.3 Numerical precision issues . .7 2.3 Related work . .7 3 Methodology 8 3.1 Delaunay meshing . .8 3.1.1 The oracle . .8 3.1.2 Surface hole filling . .8 3.2 Grid meshing . 10 3.3 Test setup . 10 3.3.1 Performance test . 11 3.3.2 Accuracy and determinism test . 12 3.3.3 Test parameters . 12 3.4 Development setup . 13 3.4.1 Hardware . 13 3.4.2 Tests . 14 4 Results 15 4.1 Grid meshing . 15 4.2 Delaunay meshing . 15 4.3 Relative observations . 19 4.3.1 High complexity noise . 19 4.3.2 Low complexity noise . 20 5 Discussion 21 5.1 Applicability . 21 5.2 Performance overhead . 21 5.3 Errors sources . 21 5.3.1 Determinism . 21 5.3.2 Surface hole filling . 21 v CONTENTS 5.3.3 Choice of parameters . 22 5.4 Future work . 22 5.4.1 Volumetric terrain . 22 5.4.2 Delaunay edge refinement . 22 6 Conclusion 23 Bibliography 24 vi 1 Introduction In computer graphics, height fields serve an important role for visualizing terrain in real- time applications, simulations and geographic information systems. Traditionally, height fields are finite regular 2D grids containing height values for each grid point, as such it is common to create a triangular mesh from sampling those values directly as vertices. Though as shown by the work of Santamaría-Ibirika et al. [1] and video games such as Minecraft and No Man’s Sky, height fields may also be algorithmically generated, from which grids of any resolution can be extrapolated and triangulated. However, due to the latter use, it is also possible to utilize other meshing techniques such as restricted Delaunay refinement which is of focus for this report. It is expected to have comparable height field representation to that of grid meshing, although it is incapable of surpassing grids in performance due to a theoretical time complexity of O(n log n) in contrast to O(n), where n is the number of triangles in the created mesh [2]. Though since the available graphics card memory and computational power is limited, it is often desired to limit the number of triangles rendered at once, which due to the nature of grids is straightforward to do through rendering a limited amount of larger grids containing the meshes, commonly referred to as chunks [1]. This presents a challenge for Delaunay re- finement which while capable of meshing versatile domains as shown by decades of research [3] has no immediate mesh pattern allowing for the chunks to create a cohesive mesh surface when each chunk is triangulated independently. In addition, applications using algorithmic height fields may rely on the deterministic mesh- ing that grids provide which ensures that the created mesh within a chunk can be regener- ated exactly at another point in time [4]. This is something that Delaunay refinement must be able to provide if it is going to be applicable in the same extent as the grid-based approach. (a) Fixed-grid (b) Delaunay refinement Figure 1.1: An example of the triangle pattern achieved by each method 1.1 Purpose The main interest for using Delaunay refinement stems from its less regular pattern of mesh- ing the surface which stands out less for low complexity surfaces while still preserving im- portant terrain features as figure 1.1 shows. It is also hoped that the comparison between 1 1 INTRODUCTION Delaunay surface meshing and that of the grid will be of use for meshing volumetric ter- rains, where the 3D grid equivalent requires the use of cubes to generate a mesh, that unlike Delaunay refinement relies on additional algorithms such as marching cubes [5] to create smooth volume meshes [6]. 1.2 Problem statement Since the grid meshing method is widely used for creating height field meshes, it serves as a control to ensure that the designed Delaunay method in this study has comparable robust- ness to representing the height field. The robustness will be measured by the accuracy of the generated mesh to the height field together with the determinism and chunking require- ment specified above. Additionally, to verify that the time complexity holds, the generation time of each method has to be measured. Thus, the following properties are of interest for answering the research question and verifying that this is achieved: • The accuracy of the generated mesh in relation to the height field. • The determinism of the generation. • The computational cost during generation. Research question Is it practical to use Delaunay refinement to mesh chunk divided height fields for real-time applications? 1.3 Scope and constraints Although there are many variants of grid-based mesh generation, this report will use a 2D fixed sized grid interspersed equally along the entire height field to create the mesh. Moreover, parallelization will not be investigated in this report, as grids are embarrassingly parallel and Delaunay-based methods are proven to be parallelizable in theory as described in section 2.2. In addition, the height fields in this report are created by the Perlin noise algorithm which is detailed in section 2.1.2.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages34 Page
-
File Size-