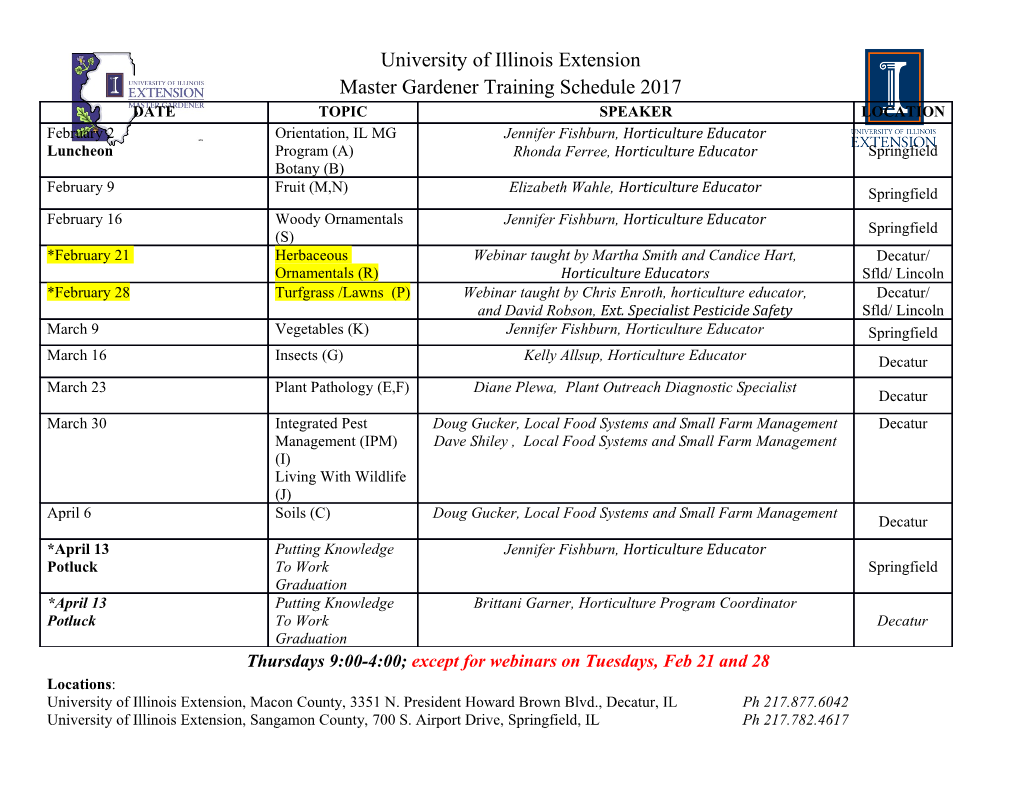
C Programming: Data Structures and Algorithms An introduction to elementary programming concepts in C Jack Straub, Instructor Version 2.07 DRAFT C Programming: Data Structures and Algorithms, Version 2.07 DRAFT C Programming: Data Structures and Algorithms Version 2.07 DRAFT Copyright © 1996 through 2006 by Jack Straub ii 08/12/08 C Programming: Data Structures and Algorithms, Version 2.07 DRAFT Table of Contents COURSE OVERVIEW ........................................................................................ IX 1. BASICS.................................................................................................... 13 1.1 Objectives ...................................................................................................................................... 13 1.2 Typedef .......................................................................................................................................... 13 1.2.1 Typedef and Portability ............................................................................................................. 13 1.2.2 Typedef and Structures .............................................................................................................. 14 1.2.3 Typedef and Functions .............................................................................................................. 14 1.3 Pointers and Arrays ..................................................................................................................... 16 1.4 Dynamic Memory Allocation ...................................................................................................... 17 1.5 The Core Module .......................................................................................................................... 17 1.5.1 The Private Header File ............................................................................................................. 18 1.5.2 The Principal Source File .......................................................................................................... 18 1.5.3 The Public Header File .............................................................................................................. 19 1.6 Activity .......................................................................................................................................... 21 2. DOUBLY LINKED LISTS ......................................................................... 23 2.1 Objectives ...................................................................................................................................... 23 2.2 Overview ....................................................................................................................................... 23 2.3 Definitions ..................................................................................................................................... 24 2.3.1 Enqueuable Item ........................................................................................................................ 25 2.3.2 Anchor ....................................................................................................................................... 26 2.3.3 Doubly Linked List ................................................................................................................... 26 2.3.4 Methods ..................................................................................................................................... 26 2.3.5 ENQ_create_list: Create a New Doubly linked List .................................................................. 27 2.3.6 ENQ_create_item: Create a New Enqueuable Item .................................................................. 28 2.3.7 ENQ_is_item_enqed: Test Whether an Item is Enqueued ........................................................ 29 2.3.8 ENQ_is_list_empty: Test Whether a List is Empty ................................................................... 29 2.3.9 ENQ_add_head: Add an Item to the Head of a List .................................................................. 29 2.3.10 ENQ_add_tail: Add an Item to the Tail of a List .................................................................. 30 2.3.11 ENQ_add_after: Add an Item After a Previously Enqueued Item ........................................ 30 2.3.12 ENQ_add_before: Add an Item Before a Previously Enqueued Item .................................. 30 2.3.13 ENQ_deq_item: Dequeue an Item from a List ..................................................................... 31 2.3.14 ENQ_deq_head: Dequeue the Item at the Head of a List ..................................................... 31 2.3.15 ENQ_deq_tail: Dequeue the Item at the Tail of a List ......................................................... 32 2.3.16 ENQ_GET_HEAD: Get the Address of the Item at the Head of a List ................................ 32 2.3.17 ENQ_GET_TAIL: Get the Address of the Item at the Tail of a List .................................... 32 2.3.18 ENQ_GET_NEXT: Get the Address of the Item After a Given Item .................................. 33 2.3.19 ENQ_GET_PREV: Get the Address of the Item Before a Given Item ................................ 33 2.3.20 ENQ_GET_LIST_NAME: Get the Name of a List .............................................................. 33 2.3.21 ENQ_GET_ITEM_NAME: Get the Name of an Item ......................................................... 34 2.3.22 ENQ_destroy_item: Destroy an Item ................................................................................... 34 iii 08/12/08 C Programming: Data Structures and Algorithms, Version 2.07 DRAFT 2.3.23 ENQ_empty_list: Empty a List............................................................................................. 35 2.3.24 ENQ_destroy_list: Destroy a List ......................................................................................... 35 2.4 Case Study .................................................................................................................................... 35 2.5 Activity .......................................................................................................................................... 39 3. SORTING ................................................................................................. 41 3.1 Objectives ...................................................................................................................................... 41 3.2 Overview ....................................................................................................................................... 41 3.3 Bubble Sort ................................................................................................................................... 41 3.4 Select Sort ..................................................................................................................................... 42 3.5 Mergesort ...................................................................................................................................... 42 3.6 A Mergesort Implementation in C .............................................................................................. 43 3.6.1 The Mergesort Function’s Footprint .......................................................................................... 43 3.6.2 The Pointer Arithmetic Problem ............................................................................................... 43 3.6.3 The Assignment Problem .......................................................................................................... 44 3.6.4 The Comparison Problem .......................................................................................................... 45 3.6.5 The Temporary Array ................................................................................................................ 46 4. MODULES ............................................................................................... 47 4.1 Objectives ...................................................................................................................................... 47 4.2 Overview ....................................................................................................................................... 47 4.3 C Source Module Components .................................................................................................... 47 4.3.1 Public Data ................................................................................................................................ 47 4.3.2 Private Data ............................................................................................................................... 48 4.3.3 Local Data ................................................................................................................................. 49 4.4 Review: Scope ............................................................................................................................... 49 4.5 A Bit about Header Files ............................................................................................................. 49 4.6 Module Conventions .................................................................................................................... 49 5. ABSTRACT DATA TYPES ...................................................................... 51 5.1 Objectives .....................................................................................................................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages167 Page
-
File Size-