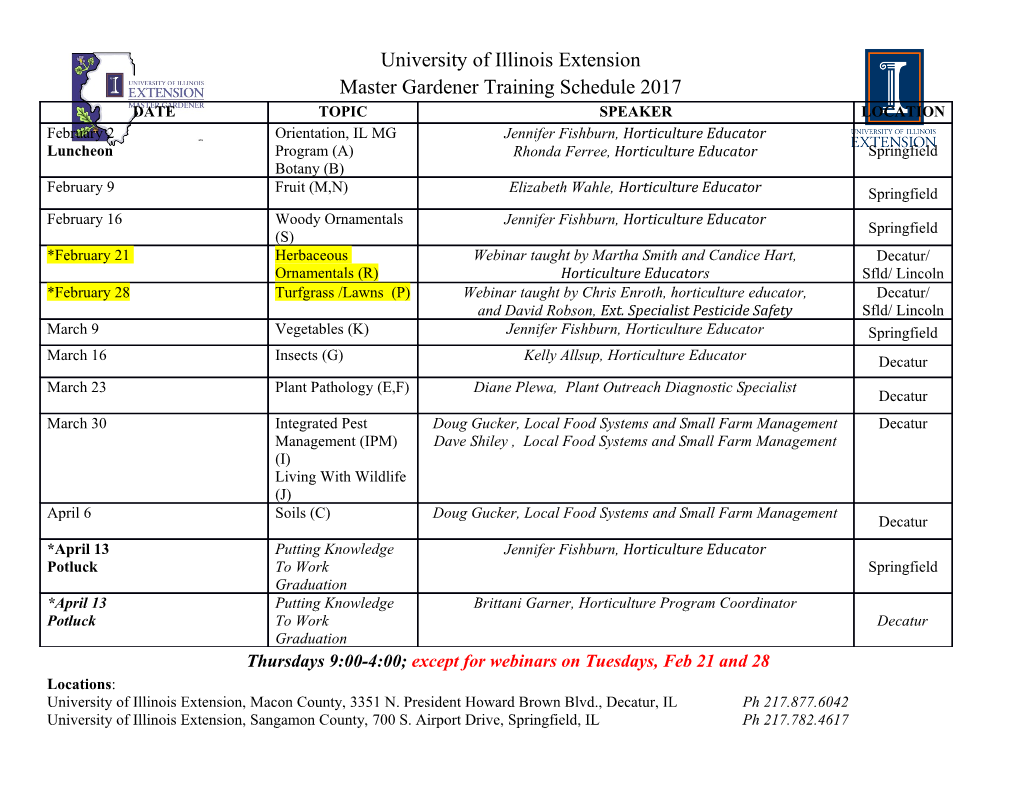
<p>17(i). Write a C program that uses Stack operations to perform the Conversion of infix expression into postfix expression.</p><p>/* 17(i). Write a C program that uses Stack operations to perform the Conversion of infix expression into postfix expression. */</p><p>#include<stdio.h> #include<stdlib.h></p><p>#define SIZE 20 char infix[20], postfix[20]; char stack[SIZE], op; int top=-1; void push_pop(int *); void push(char); void pop(int *); int priority(char); void main() { int p=-1, i; char ch; stack[++top] = '$'; clrscr(); printf("\nEnter an Infix Expression:"); i=0; while((ch=getchar())!='\n') infix[i++]=ch; infix[i]='\0'; /*gets(infix);*/ i=0; while(infix[i]!='\0') { op=infix[i]; if( (op>=48 && op<=57)||(op>=65 && op<=90)||(op>=97&&op<=122) ) postfix[++p]=op; else push_pop(&p); i++; } op='\0'; while(top!=0) pop(&p); printf("\nPostfix Expression : %s", postfix); printf("\n\n"); getch(); }/*main() function close*/ void push_pop(int *index) { if(op ==')') pop(index); else while( priority(op)<=priority(stack[top]) ) { if( stack[top] == '(') { push(op); return; } else pop(index); } if(op!=')') push(op); }/*push_pop() function close*/ int priority(char x) { int retval; switch(x) { case ')': retval=0; break; case '$': retval=1; break; case '+': case '-': retval=2; break; case '*': retval=3; break; case '/': retval=4; break; case '(': retval=5; break; case '^': retval=6; break; case '%': retval=7; break; default: printf("\nInvalid operator %c is in Infix Expression\n", x); exit(0); }/*switch close*/ return(retval); }/*priority function close*/ void push(char op) { if(top < SIZE-1) stack[++top]=op; else printf("\n\nThe stack reaches overflow.\n\n"); }/*push function close*/ void pop(int *index) { if(op==')') { while(stack[top]!='(') { postfix[++(*index)] = stack[top--]; if(top ==0) { printf("\nInvalid Expression. Left Paranthesis Missed.\n\n"); exit(0); } } top--; } else if(stack[top] == '(') { printf("\nInvalid Expression. Right Paranthesis Missed.\n\n"); exit(0); } else postfix[++(*index)] = stack[top--]; } /* pop() function close*/</p><p>Input/Output:</p><p>Enter an Infix Expression:1-2*3-4/5</p><p>Postfix Expression : 123*-45/-</p><p>17(ii). Write a C program that uses Stack operations to perform evaluating the postfix Expression</p><p>/* 17(ii). Evaluation of Postfix Expression. */</p><p>#include<stdio.h> #include<stdlib.h></p><p>#define SIZE 20 int top=-1; float stack[SIZE]; void main() { char postfix[25], *ts="", d; char ch; int i; float v; void evaluate(char); clrscr(); printf("Input the Postfix Expression:"); i=0; while((ch=getchar())!='\n') postfix[i++]=ch; postfix[i]='\0'; printf("\n Postfix : %s", postfix); i=0; while(postfix[i] !='\0') { d=postfix[i]; if(d>=48 && d<=57) { ts[0]=d; ts[1]='\0'; v=(float)atof(ts); stack[++top]=v; } else evaluate(d); i++; }/*while close*/ printf("\nThe result = %f\n",stack[0]); getch(); }/*main close*/ void evaluate(char op) { float m ,n, x; int i; n =stack[top--]; m =stack[top--]; switch(op) { case '+': stack[++top]=m + n; break; case '-': stack[++top]=m -n; break; case '*': stack[++top]=m *n; break; case '/': stack[++top]=m /n; break; case '^': x=1; for(i=1;i<=n;i++) x=x*m ; stack[++top]=x; break; default: printf("\nInvalid operator found in expression.\n"); }/*switch close*/ }/*evaluate close*/</p><p>Input/Output:</p><p>Input the Postfix Expression:24+5*</p><p>Postfix : 24+5* The result = 30.000000</p><p>18.Write C-Programs that use both recursive and non recursive functions to perform the following searching operations for a Key value in a given list of integers : i) Linear search ii) Binary search iii)Recursive Linear Search iv) Recursive Binary Search</p><p>18(i) .Write C program that uses non recursive function to perform Linear search</p><p>/* 18(i) Write a Program for Linear Search using function(Non Recursive). */</p><p>#define MAX 25 #include<stdio.h> void main() { int n, i, index, key, a[MAX]; clrscr(); printf("\nEnter size of the list:"); scanf("%d", &n); printf("\nEnter %d numbers of list:\n",n); for(i=0; i<n; i++) scanf("%d", &a[i]);</p><p> printf("\nEnter the number to be searched:"); scanf("%d", &key);</p><p> index=linearsearch(key, a, n); if(index==-1) printf("\nThe %d is not found in the list",key); else printf("\nThe %d is found at %d location.",key, index); getch(); } int linearsearch(int x, int a[], int n) { int i;</p><p> for(i=0; i<n; i++) if(x==a[i]) return(i+1);</p><p> return(-1); }</p><p>Input/Output: Enter size of the list:10</p><p>Enter 1398 numbers of list: 12 14 16 17 18 19 20 34 54 27</p><p>Enter the number to be searched:100</p><p>The 100 is not found in the list </p><p>18(ii) .Write C programs that uses and non recursive functions to perform the Linear search</p><p>/* 18(ii). Write a Program for Binary Search using function(Non Recursive). */</p><p>#define MAX 25 #include<stdio.h> void main() { int n, i, index, key, a[MAX]; clrscr(); printf("\nEnter size of the list:"); scanf("%d", &n); printf("\nEnter %d numbers of list:\n",n); for(i=0; i<n; i++) scanf("%d", &a[i]);</p><p> printf("\nEnter the number to be searched:"); scanf("%d", &key);</p><p> index=binarysearch(key, a, n); if(index==-1) printf("\nThe %d is not found in the list",key); else printf("\nThe %d is found at %d location.",key, index);</p><p> getch(); } int binarysearch(int x, int a[], int n) { int low, high, mid; low=0; high=n-1; while(low<=high) { mid=(low+high)/2; if(x<a[mid]) high=mid-1; else if(x>a[mid]) low=mid+1; else return(mid+1); } return(-1); }</p><p>Input/Output: Enter size of the list:6</p><p>Enter 6 numbers of list: 10 11 14 15 18 20</p><p>Enter the number to be searched:15</p><p>The 15 is found at 4 location.</p><p>18(iii). Write a C-Programs that uses Recursive function to perform Linear search</p><p>/* 18(iii).Write a Program for Linear Search using Recursive Function. */</p><p>#define MAX 25 #include<stdio.h> void linearsearch(int, int[], int); void main() { int n, i, key, a[MAX]; clrscr(); printf("\nEnter size of the list:"); scanf("%d", &n); printf("\nEnter %d numbers of list:\n",n); for(i=0; i<n; i++) scanf("%d", &a[i]);</p><p> printf("\nEnter the number to be searched:"); scanf("%d", &key); i=0; linearsearch(key, a, n-1); getch(); } void linearsearch(int x, int a[], int n) { if(n<0) { printf("\nThe %d is not found in the list.", x); getch(); exit(0); } else if(x==a[n]) { printf("\nThe %d is found at %d location.", x, n+1); getch(); exit(0); } linearsearch(x, a, n-1); }</p><p>Input/Output:</p><p>Enter size of the list: 8</p><p>Enter 8 numbers of list: 22 13 42 56 75 70 57 100</p><p>Enter the number to be searched:56</p><p>The 56 is found at 4 location.</p><p>18(iv). Write a C-Programs that uses Recursive function to perform Binary search</p><p>/* 18(iv). Write a Program for Binary Search using Recursive Function. */</p><p>#define MAX 25 #include<stdio.h> void binarysearch(int, int[], int, int); void main() { int n, i, key, a[MAX]; clrscr(); printf("\nEnter size of the list:"); scanf("%d", &n); printf("\nEnter %d numbers of list:\n",n); for(i=0; i<n; i++) scanf("%d", &a[i]);</p><p> printf("\nEnter the number to be searched:"); scanf("%d", &key);</p><p> binarysearch(key, a, 0, n-1);</p><p> getch(); } void binarysearch(int x, int a[], int low, int high) { int mid; if(low>high) { printf("\nThe %d is not found in the list.",x); getch(); exit(); } mid=(low+high)/2; if(x<a[mid]) binarysearch(x,a,low,mid-1); else if(x>a[mid]) binarysearch(x,a,mid+1, high); else { printf("\nThe %d is found at %d location.",x, mid+1); getch(); exit(0); } }</p><p>Input/Output:</p><p>Enter size of the list: 8</p><p>Enter 8 numbers of list: 10 15 25 35 42 50 75 100</p><p>Enter the number to be searched:75</p><p>The 75 is found at 7 location,</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages9 Page
-
File Size-