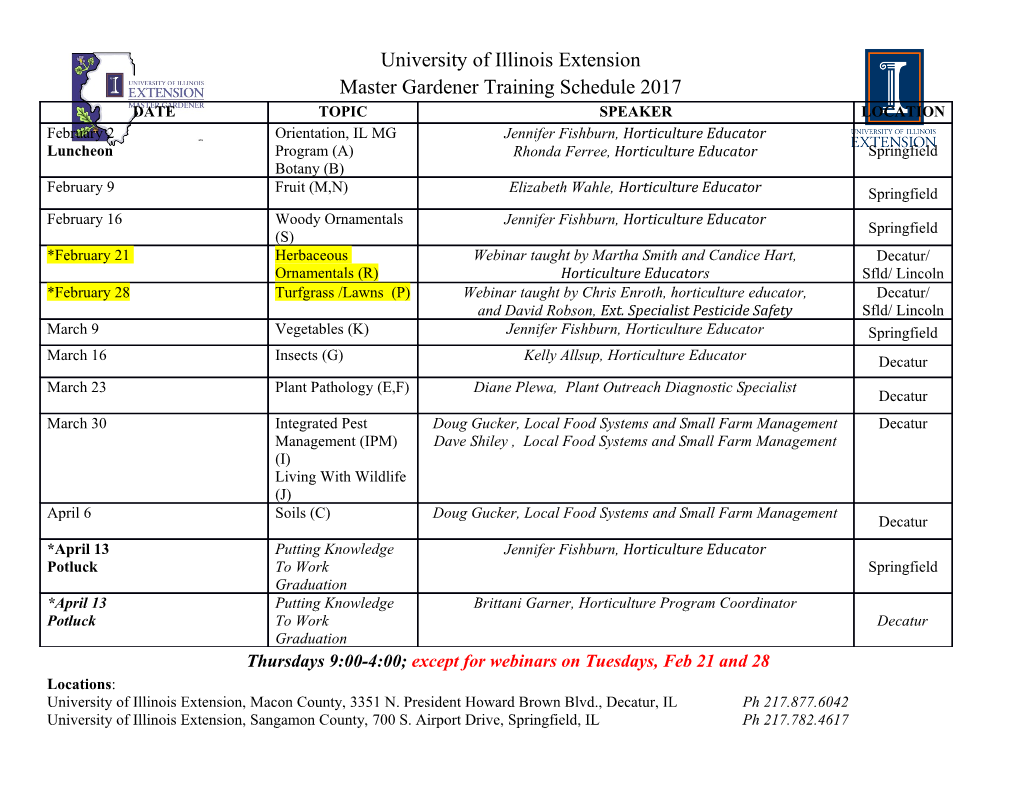
<p>CIS265 Lecture Notes: Custom Made Linked Lists </p><p>PERSON class public class Person implements Comparable<Person> {</p><p> private String name; private int age; // ------public Person(String name, int age) { super(); this.name = name; this.age = age; } // ------</p><p> public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } // ------public String toString() { return " Name:" + name + " Age:" + age; } // ------@Override public int compareTo(Person otherPerson) { if ( this.age == otherPerson.getAge() ) return 0; else if ( this.age > otherPerson.getAge()) return 1; else return -1; } } NODE class public class Node< E extends Comparable<E> > implements Comparable <Node<E> > {</p><p> private E element; private Node<E> next; private Node<E> previous;</p><p>// ------public Node(E dataObject) { //Person p = new Person(theName, theAge); element = dataObject; next = null; previous = null; } // ------</p><p> public E getElement() { return element; }</p><p> public void setElement(E element) { this.element = element; }</p><p> public Node<E> getNext() { return next; }</p><p> public void setNext(Node<E> next) { this.next = next; }</p><p> public Node<E> getPrevious() { return previous; }</p><p> public void setPrevious(Node<E> previous) { this.previous = previous; }</p><p>// ------</p><p> public String showNode() { return element.toString(); }</p><p>@Override public int compareTo(Node<E> other) { E otherElement = other.getElement(); E thisElement = this.getElement(); return thisElement.compareTo(otherElement); } } LINKED LIST class public class LinkedList<E extends Comparable<E> > {</p><p> private Node<E> head; private Node<E> last; private int count; // ------public LinkedList() { head = null; last = null; count = 0; } // ------public Node<E> getHead() { return head; }</p><p> public void setHead(Node<E> head) { this.head = head; }</p><p> public Node<E> getLast() { return last; }</p><p> public void setLast(Node<E> last) { this.last = last; }</p><p> public int getCount() { return count; }</p><p> public void setCount(int count) { this.count = count; }</p><p>// ------// insert new node at the end of the list public void add(Node<E> newNode) {</p><p> if (count == 0) { head = newNode; last = newNode; count = 1; return; }</p><p> count++; last.setNext(newNode); newNode.setPrevious(last); last = n; } // ------// add new node in the list’s natural order public void addInOrderVersion1(Node<E> newNode) { if (count == 0) { head = newNode; last = newNode; count = 1; return; }</p><p> count++; Node<E> ptr = head; E element = null; boolean keepGoing = true; while (keepGoing && ptr != null) { element = ptr.getElement(); if (element.compareTo(newNode.getElement()) < 0) { ptr = ptr.getNext(); } else { keepGoing = false; } }// while</p><p> if (ptr == null) { last.setNext(newNode); newNode.setPrevious(last); last = newNode; } else { newNode.setNext(ptr); newNode.setPrevious(ptr.getPrevious()); if (ptr == head) { head = newNode;</p><p>} else { ptr.getPrevious().setNext(newNode); ptr.setPrevious(newNode); } }</p><p>} // ------public void addInOrderVersion2(Node<E> newNode) { count++; // new node is the first to be inserted in the list if (count == 0) { head = newNode; last = newNode; return; }</p><p>// new node goes at the beginning of the list if ( head.compareTo(newNode) > 0 ){ //new node is smaller than first element newNode.setNext(head); head.setPrevious(newNode); head = newNode; return; } // new node goes at the end of the list if ( last.compareTo(newNode) < 0 ){ //new node is larger than last element newNode.setPrevious(last); last.setNext(newNode); last = newNode; return; }</p><p>Node<E> ptr = head; Node<E> prior = null;</p><p> while ( ptr != null) { if ( newNode.compareTo(ptr) < 0) break; prior = ptr; ptr = ptr.getNext();</p><p>}// while</p><p>// place news node in between existing elements newNode.setNext(ptr); newNode.setPrevious(prior); prior.setNext(newNode); ptr.setPrevious(newNode);</p><p>}</p><p>// ------public void showList() { System.out.printf("\n<<List Head:%s Last:%s Count:%d >>\n", head, last, count); E element; Node<E> ptr = head; while (ptr != null) { element = ptr.getElement(); System.out.println(ptr.toString() + "\t" + element.toString()); ptr = ptr.next; } }</p><p>} DRIVER package csu.matos; public class Driver1 {</p><p>/** * Creating a custom-made linked list holding Person data * */ public static void main(String[] args) {</p><p>Node<Person> p1 = new Node<Person>(new Person("AAA", 111)); Node<Person> p2 = new Node<Person>(new Person("BBB", 222)); Node<Person> p3 = new Node<Person>(new Person("CCC", 333));</p><p>Node<Person> head = null; Node<Person> tail = null; Node<Person> ptr = null;</p><p> head = p1; tail = p3;</p><p> p1.setNext(p2); p2.setNext(p3); p2.setPrevious(p1); p3.setPrevious(p2);</p><p> ptr = head; while (ptr != null) { System.out.println(ptr + "\t" + ptr.showNode()); ptr = ptr.getNext(); }</p><p>System.out.println("Here is the list");</p><p>// ------</p><p>LinkedList<Person> list = new LinkedList<Person>();</p><p> list.add (p1); list.add(p2); list.add(p3);</p><p> list.showList();</p><p>System.out.println("Here is the list-after addInOrder");</p><p> list.addInOrderVersion2(new Node<Person> (new Person("XXX", 150)) );</p><p> list.showList();</p><p>}// main } CONSOLE</p><p> csu.matos.Node@2a9931f5 Name:AAA Age:111 csu.matos.Node@2f9ee1ac Name:BBB Age:222 csu.matos.Node@67f1fba0 Name:CCC Age:333</p><p>Here is the list</p><p><<List Head:csu.matos.Node@2a9931f5 Last:csu.matos.Node@67f1fba0 Count:3 >> csu.matos.Node@2a9931f5 Name:AAA Age:111 csu.matos.Node@2f9ee1ac Name:BBB Age:222 csu.matos.Node@67f1fba0 Name:CCC Age:333</p><p>Here is the list-after addInOrder</p><p><<List Head:csu.matos.Node@2a9931f5 Last:csu.matos.Node@1ee7b241 Count:4 >> csu.matos.Node@2a9931f5 Name:AAA Age:111 csu.matos.Node@1ee7b241 Name:XXX Age:150 csu.matos.Node@2f9ee1ac Name:BBB Age:222 csu.matos.Node@67f1fba0 Name:CCC Age:333 VERSION 2</p><p>Minor changes are made here to enhance the management of Generic Data Objects accepted by the custom made doubly- linked list</p><p>.</p><p>DRIVER package csu.matos; public class Driver {</p><p>/** * Author: V. Matos * Date: 4-11-2013 * Goal: Create a generic UDT (User-defined Data Type) * to support linked-list operations. * Minor changes to enhance the previous version * (look for Generic-Data-Type and treatment of toString) */ public static void main(String[] args) {</p><p>// create a custom-made doubly-linked list MyList<Person> mylist = new MyList<Person>();</p><p> mylist.addLast(new Person("Daenerys", "123456789")); mylist.addLast(new Person("Arya", "111222333")); mylist.addLast(new Person("Sansa", "222333444")); mylist.addLast(new Person("Tyrion", "333444555")); mylist.addLast(new Person("John", "444555666"));</p><p>System.out.println( mylist.showControlData() ); mylist.showForward(); mylist.showBackward();</p><p>System.out.println("\nSearching...."); String key = "222333444"; Node<Person> foundNode = mylist.find(key); if ( foundNode == null) { System.out.println("KEY NOT FOUND" + key); } else { Person foundPerson = foundNode.getData(); System.out.println( "FOUND " + foundPerson); }</p><p>// try to delete node found above if ( mylist.removeNode(foundNode) ) { System.out.println("After attempt to delete..."); mylist.showForward(); }</p><p>// insert Person at a given position (fake ArrayLit's insert) if ( mylist.insert( 2, new Person("Brain", "999888777")) ) { mylist.showForward(); }</p><p>}//main } MyList Class package csu.matos; public class MyList <E extends Comparable<String> > { // class variables private Node<E> first; private Node<E> last; private int count;</p><p>// contructor public MyList() { this.first = null; this.last = null; this.count = 0; } // mutators public Node<E> getFirst() { return first; } public void setFirst( Node<E> n1) { this.first = n1; } public Node<E> getLast() { return last; } public void setLast(Node<E> last) { this.last = last; } public int getCount() { return count; } public void setCount(int count) { this.count = count; } // user-defined methods public String showControlData(){ return String.format( "\nCONTROL\tFIRST:%s \n\tLAST: %s \n\tCOUNT:%d", this.getFirst(), this.getLast(), this.getCount()); }</p><p>// ------// Add new node at the end of the list: public int addLast(E data){ // place given data in a new node n Node<E> newNode = new Node<E>(data);</p><p> if ( count == 0){ first = newNode; last = newNode; } else { // place new element at the end of the existing list last.setNext(newNode); newNode.setPrevious(last); last = newNode; } return ++count; }</p><p>// Add new node at the end of the list: public int addFirst(E data) { // place given data in a new node n Node<E> newNode = new Node<E>(data);</p><p> if (count == 0) { first = newNode; last = newNode; } else { // place new element at the beginning of the existing list first.setPrevious(newNode); newNode.setNext(first); first = newNode; } return ++count; } public Node<E> find( String key){ E data = null; Node<E> n = first; while ( n != null){ data = n.getData(); if ( data.compareTo(key) == 0) return n; else n = n.getNext(); }</p><p> return null; }//find public void showForward(){ int index = 0; System.out.println("\nTraversing list (forward ...)" + count); Node<E> n = this.getFirst(); while ( n != null ){ System.out.println( index++ + n.showData() ); n = n.getNext(); } }//showForward public void showBackward(){ int index = count - 1; System.out.println("\nTraversing list (backward ...)" + count); Node<E> n = this.getLast(); while ( n != null ){ System.out.println( index-- + n.showData() ); n = n.getPrevious(); } }//showForward</p><p>// remove node at give memory location public boolean removeNode(Node<E> nodeToBeDeleted){ //NAIVE: we assume node is part of the list if (count == 0 || nodeToBeDeleted == null ) return false;</p><p> if ( count == 1 && ((nodeToBeDeleted == first) || (nodeToBeDeleted == last ))){ first = last = null; count= 0; return true; } // Check cases: nodeToBeDeleted is first, last, or in between Node<E> left = nodeToBeDeleted.getPrevious(); Node<E> right = nodeToBeDeleted.getNext(); count--;</p><p> if ( nodeToBeDeleted == first){ right.setPrevious(null); first = right; return true; }</p><p> if ( nodeToBeDeleted == last){ left.setNext(null); last = left; return true; }</p><p>// NAIVE: assuming nodeToBeDeleted is in current chain // (a better way is to keep extra ptr. to control node) left.setNext(right); right.setPrevious(left); return true; }//removeNode</p><p> public boolean insert(int pos, E newData) { Node<E> newNode = new Node<E>(newData); if ( pos > count || pos < 0) return false;</p><p>// add newData at the end of the list; if ( pos == count -1){ addLast(newData); return true; }</p><p> if ( pos == 0 ){ addFirst(newData); return true; }</p><p>Node<E> currentNode = first; for(int i=0 ; i< pos; i++){ currentNode = currentNode.getNext(); } // connect newNode with existing neighbors(left, current) Node<E> left = currentNode.getPrevious(); newNode.setPrevious(left); newNode.setNext(currentNode); currentNode.setPrevious(newNode); left.setNext(newNode); count++; return true; }//insert</p><p>} Node Class package csu.matos; public class Node <E extends Comparable<String> > { // class variables E data; Node<E> next; Node<E> previous;</p><p>// constructor public Node(E data) { this.data = data; this.next = null; this.previous = null; } // mutators</p><p> public Node() { this.data = null; this.next = null; this.previous = null; } public E getData() { return data; } public void setData(E data) { this.data = data; } public Node<E> getNext() { return next; } public void setNext(Node<E> next) { this.next = next; }</p><p> public Node<E> getPrevious() { return previous; }</p><p> public void setPrevious(Node<E> previous) { this.previous = previous; }</p><p>// user-defined methods public String showData() { String format = "\n[NODE \tCURR:\t %s ]\n" + "[NODE \tNEXT:\t %s\n\tPREVIOUS:%s \n\tDATA:\t %s ]"; return String.format(format, this, this.getNext(), this.getPrevious(), this.getData() ); } } Person Class</p><p> package csu.matos; public class Person implements Comparable<String> { // class variables private String name; private String ssn; private Object thisPerson;</p><p>// constructor(s) public Person(String name, String ssn) { super(); this.name = name; this.ssn = ssn; thisPerson = this; }</p><p> public Person() { super(); this.name = "n.a"; this.ssn = "-1"; thisPerson = this; }</p><p>// mutator(s) public String getName() { return name; }</p><p> public void setName(String name) { this.name = name; }</p><p> public String getSsn() { return ssn; }</p><p> public void setSsn(String ssn) { this.ssn = ssn; } // ======// user-defined methods</p><p> public String showData(){ return String.format( "\n\t[PERSON LOC: %s]\n\t[PERSON NAME:%s SSN:%s]", this, this.getName(), this.getSsn() ); }</p><p>@Override public String toString(){ // done this way to stop recursion-observe that plain: this // calls this.toString(). String thisLoc = getClass().getName() + '@' + Integer.toHexString( hashCode() ); //return thisLoc; return String.format( "\n\t[PERSON LOC: %s]\n\t[PERSON NAME:%s SSN:%s]", thisLoc, this.getName(), this.getSsn() );</p><p>}</p><p>// compare Person data with a give SSN key-value @Override public int compareTo(String key) { String mySsn = this.getSsn(); if (mySsn.compareTo(key) == 0) return 0; else if (mySsn.compareTo(key) > 0) return 1; else return -1; } }</p><p>CONSOLE</p><p>CONTROL FIRST:csu.matos.Node@62fcf06c LAST: csu.matos.Node@4c0c7539 COUNT:5</p><p>Traversing list (forward ...)5 0 [NODE CURR: csu.matos.Node@62fcf06c ] [NODE NEXT: csu.matos.Node@45e41830 PREVIOUS:null DATA: [PERSON LOC: csu.matos.Person@1f01b29] [PERSON NAME:Daenerys SSN:123456789] ] 1 [NODE CURR: csu.matos.Node@45e41830 ] [NODE NEXT: csu.matos.Node@3a8721bd PREVIOUS:csu.matos.Node@62fcf06c DATA: [PERSON LOC: csu.matos.Person@7db81d4f] [PERSON NAME:Arya SSN:111222333] ] 2 [NODE CURR: csu.matos.Node@3a8721bd ] [NODE NEXT: csu.matos.Node@428c6e04 PREVIOUS:csu.matos.Node@45e41830 DATA: [PERSON LOC: csu.matos.Person@118aeabe] [PERSON NAME:Sansa SSN:222333444] ] 3 [NODE CURR: csu.matos.Node@428c6e04 ] [NODE NEXT: csu.matos.Node@4c0c7539 PREVIOUS:csu.matos.Node@3a8721bd DATA: [PERSON LOC: csu.matos.Person@373968f1] [PERSON NAME:Tyrion SSN:333444555] ] 4 [NODE CURR: csu.matos.Node@4c0c7539 ] [NODE NEXT: null PREVIOUS:csu.matos.Node@428c6e04 DATA: [PERSON LOC: csu.matos.Person@1a18c28a] [PERSON NAME:John SSN:444555666] ]</p><p>Traversing list (backward ...)5 4 [NODE CURR: csu.matos.Node@4c0c7539 ] [NODE NEXT: null PREVIOUS:csu.matos.Node@428c6e04 DATA: [PERSON LOC: csu.matos.Person@1a18c28a] [PERSON NAME:John SSN:444555666] ] 3 [NODE CURR: csu.matos.Node@428c6e04 ] [NODE NEXT: csu.matos.Node@4c0c7539 PREVIOUS:csu.matos.Node@3a8721bd DATA: [PERSON LOC: csu.matos.Person@373968f1] [PERSON NAME:Tyrion SSN:333444555] ] 2 [NODE CURR: csu.matos.Node@3a8721bd ] [NODE NEXT: csu.matos.Node@428c6e04 PREVIOUS:csu.matos.Node@45e41830 DATA: [PERSON LOC: csu.matos.Person@118aeabe] [PERSON NAME:Sansa SSN:222333444] ] 1 [NODE CURR: csu.matos.Node@45e41830 ] [NODE NEXT: csu.matos.Node@3a8721bd PREVIOUS:csu.matos.Node@62fcf06c DATA: [PERSON LOC: csu.matos.Person@7db81d4f] [PERSON NAME:Arya SSN:111222333] ] 0 [NODE CURR: csu.matos.Node@62fcf06c ] [NODE NEXT: csu.matos.Node@45e41830 PREVIOUS:null DATA: [PERSON LOC: csu.matos.Person@1f01b29] [PERSON NAME:Daenerys SSN:123456789] ]</p><p>Searching.... FOUND [PERSON LOC: csu.matos.Person@118aeabe] [PERSON NAME:Sansa SSN:222333444] After attempt to delete...</p><p>Traversing list (forward ...)4 0 [NODE CURR: csu.matos.Node@62fcf06c ] [NODE NEXT: csu.matos.Node@45e41830 PREVIOUS:null DATA: [PERSON LOC: csu.matos.Person@1f01b29] [PERSON NAME:Daenerys SSN:123456789] ] 1 [NODE CURR: csu.matos.Node@45e41830 ] [NODE NEXT: csu.matos.Node@428c6e04 PREVIOUS:csu.matos.Node@62fcf06c DATA: [PERSON LOC: csu.matos.Person@7db81d4f] [PERSON NAME:Arya SSN:111222333] ] 2 [NODE CURR: csu.matos.Node@428c6e04 ] [NODE NEXT: csu.matos.Node@4c0c7539 PREVIOUS:csu.matos.Node@45e41830 DATA: [PERSON LOC: csu.matos.Person@373968f1] [PERSON NAME:Tyrion SSN:333444555] ] 3 [NODE CURR: csu.matos.Node@4c0c7539 ] [NODE NEXT: null PREVIOUS:csu.matos.Node@428c6e04 DATA: [PERSON LOC: csu.matos.Person@1a18c28a] [PERSON NAME:John SSN:444555666] ]</p><p>Traversing list (forward ...)5 0 [NODE CURR: csu.matos.Node@62fcf06c ] [NODE NEXT: csu.matos.Node@45e41830 PREVIOUS:null DATA: [PERSON LOC: csu.matos.Person@1f01b29] [PERSON NAME:Daenerys SSN:123456789] ] 1 [NODE CURR: csu.matos.Node@45e41830 ] [NODE NEXT: csu.matos.Node@4f8bff68 PREVIOUS:csu.matos.Node@62fcf06c DATA: [PERSON LOC: csu.matos.Person@7db81d4f] [PERSON NAME:Arya SSN:111222333] ] 2 [NODE CURR: csu.matos.Node@4f8bff68 ] [NODE NEXT: csu.matos.Node@428c6e04 PREVIOUS:csu.matos.Node@45e41830 DATA: [PERSON LOC: csu.matos.Person@702d2da4] [PERSON NAME:Brain SSN:999888777] ] 3 [NODE CURR: csu.matos.Node@428c6e04 ] [NODE NEXT: csu.matos.Node@4c0c7539 PREVIOUS:csu.matos.Node@4f8bff68 DATA: [PERSON LOC: csu.matos.Person@373968f1] [PERSON NAME:Tyrion SSN:333444555] ] 4 [NODE CURR: csu.matos.Node@4c0c7539 ] [NODE NEXT: null PREVIOUS:csu.matos.Node@428c6e04 DATA: [PERSON LOC: csu.matos.Person@1a18c28a] [PERSON NAME:John SSN:444555666] ] </p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages17 Page
-
File Size-