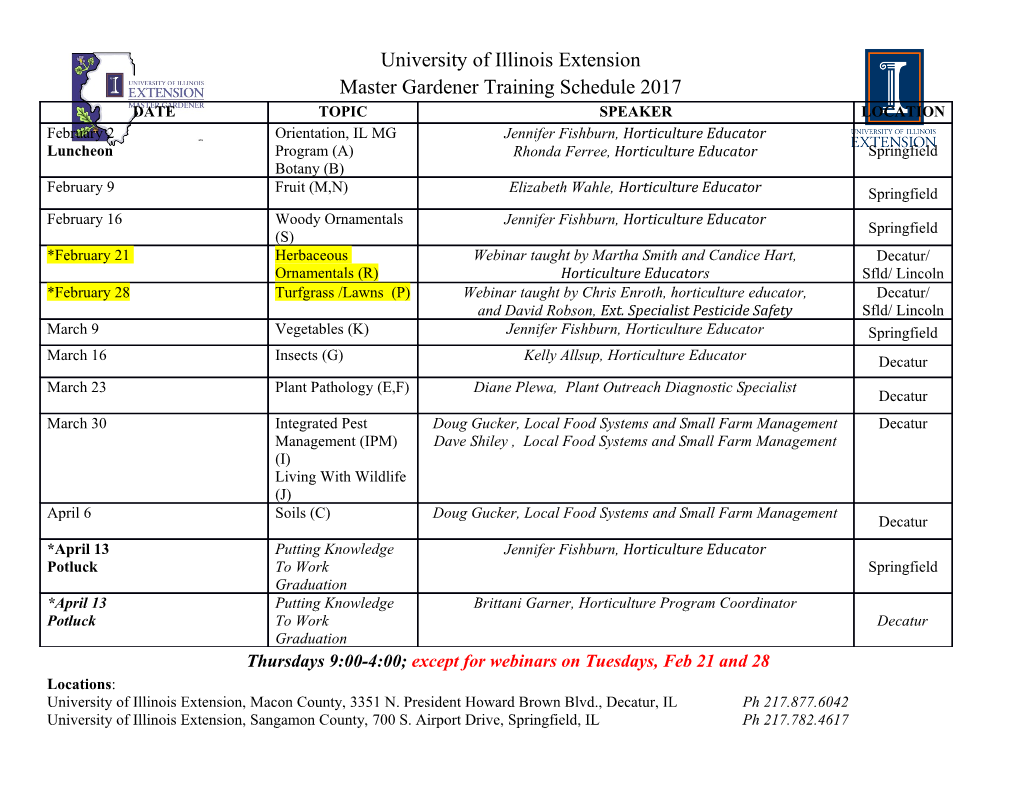
<p>Introductory Material: ... pg 1</p><p>1.1 The Structure of This Book</p><p>1.1.1 Examples and References 1.1.2 Exercises 1.1.3 Implementation Note</p><p>1.2 Learning C++ 1.3 The Design of C++</p><p>1.3.1 Efficiency and Structure 1.3.2 Philosophical Note</p><p>1.4 Historical Note 1.5 Use of C++ 1.6 C and C++</p><p>1.6.1 Suggestions for C Programmers 1.6.2 Suggestions for C++ Programmers</p><p>1.7 Thinking about Programming in C++ 1.8 Advice</p><p>1.8.1 References</p><p>2 A Tour of C++</p><p>2.1 What is C++ 2.2 Programming Paradigms 2.3 Procedural Programming</p><p>2.3.1 Variables and Arithmetic 2.3.2 Tests and Loops 2.3.3 Pointers and Arrays</p><p>2.4 Modular Programming</p><p>2.4.1 Separate Compilation 2.4.2 Exception Handling</p><p>2.5 Data Abstraction</p><p>2.5.1 Modules Defining Types 2.5.2 User-Defined Types 2.5.3 Concrete Types 2.5.4 Abstract Types 2.5.5 Virtual Functions</p><p>2.6 Object-Oriented Programming 2.6.1 Problems with Concrete Types 2.6.2 Class Hierarchies</p><p>2.7 Generic Programming</p><p>2.7.1 Containers 2.7.2 Generic Algorithms</p><p>2.8 Postscript 2.9 Advice</p><p>3 A Tour of the Standard Library</p><p>3.1 Introduction 3.2 Hello, world! 3.3 The Standard Library Namespace 3.4 Output 3.5 Strings</p><p>3.5.1 C-Style Strings</p><p>3.6 Input 3.7 Containers</p><p>3.7.1 Vector 3.7.2 Range Checking 3.7.3 List 3.7.4 Map 3.7.5 Standard Containers</p><p>3.8 Algorithms</p><p>3.8.1 Use of Iterators 3.8.2 Iterator Types 3.8.3 Iterators and I/O 3.8.4 Traversals and Predicates 3.8.5 Algorithms Using Member Functions 3.8.6 Standard Library Algorithms</p><p>3.9 Math</p><p>3.9.1 Complex Numbers 3.9.2 Vector Arithmetic 3.9.3 Basic Numeric Support</p><p>3.10 Standard Library Facilities 3.11 Advice</p><p>Part I: Basic Facilities ... pg 67</p><p>4 Types and Declarations 4.1 Types</p><p>4.1.1 Fundamental Types</p><p>4.2 Booleans 4.3 Character Types</p><p>4.3.1 Character Literals</p><p>4.4 Integer Types</p><p>4.4.1 Integer Literals</p><p>4.5 Floating-Point Types</p><p>4.5.1 Floating-Point Literals</p><p>4.6 Sizes 4.7 Void 4.8 Enumerations 4.9 Declarations</p><p>4.9.1 The Structure of a Declaration 4.9.2 Declaring Multiple Names 4.9.3 Names 4.9.4 Scope 4.9.5 Initialization 4.9.6 Objects and Lvalues 4.9.7 Typedef</p><p>4.10 Advice 4.11 Exercises</p><p>5 Pointers, Arrays, and Structures</p><p>5.1 Pointers</p><p>5.1.1 Zero</p><p>5.2 Arrays</p><p>5.2.1 Array Initializers 5.2.2 String Literals</p><p>5.3 Pointers into Arrays</p><p>5.3.1 Navigating Arrays</p><p>5.4 Constants</p><p>5.4.1 Pointers and Constants 5.5 References 5.6 Pointer to Void 5.7 Structures</p><p>5.7.1 Type Equivalence</p><p>5.8 Advice 5.9 Exercises</p><p>6 Expressions and Statements</p><p>6.1 A Desk Calculator</p><p>6.1.1 The Parser 6.1.2 The Input Function 6.1.3 Low-level Input 6.1.4 Error Handling 6.1.5 The Driver 6.1.6 Headers 6.1.7 Command-Line Arguments 6.1.8 A Note on Style</p><p>6.2 Operator Summary</p><p>6.2.1 Results 6.2.2 Evaluation Order 6.2.3 Operator Precedence 6.2.4 Bitwise Logical Operators 6.2.5 Increment and Decrement 6.2.6 Free Store</p><p>6.2.6.1 Arrays 6.2.6.2 Memory Exhaustion</p><p>6.2.7 Explicit Type Conversion 6.2.8 Constructors</p><p>6.3 Statement Summary</p><p>6.3.1 Declarations as Statements 6.3.2 Selection Statements</p><p>6.3.2.1 Declarations in Conditions</p><p>6.3.3 Iteration Statements</p><p>6.3.3.1 Declarations in For-Statements</p><p>6.3.4 Goto</p><p>6.4 Comments and Indentation 6.5 Advice 6.6 Exercises</p><p>7 Functions</p><p>7.1 Function Declarations</p><p>7.1.1 Function Definitions 7.1.2 Static Variables</p><p>7.2 Argument Passing</p><p>7.2.1 Array Arguments</p><p>7.3 Value Return 7.4 Overloaded Function Names</p><p>7.4.1 Overloading and Return Type 7.4.2 Overloading and Scopes 7.4.3 Manual Ambiguity Resolution 7.4.4 Resolution for Multiple Arguments</p><p>7.5 Default Arguments 7.6 Unspecified Number of Arguments 7.7 Pointer to Function 7.8 Macros</p><p>7.8.1 Conditional Compilation</p><p>7.9 Advice 7.10 Exercises</p><p>8 Namespaces and Exceptions</p><p>8.1 Modularization and Interfaces 8.2 Namespaces</p><p>8.2.1 Qualified Names 8.2.2 Using Declarations 8.2.3 Using Directives 8.2.4 Multiple Interfaces</p><p>8.2.4.1 Interface Design Alternatives</p><p>8.2.5 Avoiding Name Clashes</p><p>8.2.5.1 Unnamed Namespaces</p><p>8.2.6 Name Lookup 8.2.7 Namespace Aliases 8.2.8 Namespace Composition 8.2.8.1 Selection 8.2.8.2 Composition and Selection</p><p>8.2.9 Namespaces and Old Code</p><p>8.2.9.1 Namespaces and C 8.2.9.2 Namespaces and Overloading 8.2.9.3 Namespaces Are Open</p><p>8.3 Exceptions</p><p>8.3.1 Throw and Catch 8.3.2 Discrimination of Exceptions 8.3.3 Exceptions in the Calculator</p><p>8.3.3.1 Alternative Error-Handling Strategies</p><p>8.4 Advice 8.5 Exercises</p><p>9 Source Files and Programs</p><p>9.1 Separate Compilation 9.2 Linkage</p><p>9.2.1 Header Files 9.2.2 Standard Library Headers 9.2.3 The One-Definition Rule 9.2.4 Linkage to Non-C++ Code 9.2.5 Linkage and Pointers to Functions</p><p>9.3 Using Header Files</p><p>9.3.1 Single Header File 9.3.2 Multiple Header Files</p><p>9.3.2.1 Other Calculator Modules 9.3.2.2 Use of Headers</p><p>9.3.3 Include Guards</p><p>9.4 Programs</p><p>9.4.1 Initialization of Nonlocal Variables</p><p>9.4.1.1 Program Termination</p><p>9.5 Advice 9.6 Exercises Part II: Abstraction Mechanisms ... pg 221</p><p>10 Classes</p><p>10.1 Introduction 10.2 Classes</p><p>10.2.1 Member Functions 10.2.2 Access Control 10.2.3 Constructors 10.2.4 Static Members 10.2.5 Copying Class Objects 10.2.6 Constant Member Functions 10.2.7 Self-Reference</p><p>10.2.7.1 Physical and Logical Constness 10.2.7.2 Mutable</p><p>10.2.8 Structures and Classes 10.2.9 In-Class Function Definitions</p><p>10.3 Efficient User-Defined Types</p><p>10.3.1 Member Functions 10.3.2 Helper Functions 10.3.3 Overloaded Operators 10.3.4 The Significance of Concrete Classes</p><p>10.4 Objects</p><p>10.4.1 Destructors 10.4.2 Default Constructors 10.4.3 Construction and Destruction 10.4.4 Local Variables</p><p>10.4.4.1 Copying Objects</p><p>10.4.5 Free Store 10.4.6 Class Objects as Members</p><p>10.4.6.1 Necessary Member Initialization 10.4.6.2 Member Constants 10.4.6.3 Copying Members</p><p>10.4.7 Arrays 10.4.8 Local Static Store 10.4.9 Nonlocal Store</p><p>10.4.10 Temporary Objects 10.4.11 Placement of Objects 10.4.12 Unions 10.5 Advice 10.6 Exercises</p><p>11 Operator Overloading</p><p>11.1 Introduction 11.2 Operator Functions</p><p>11.2.1 Binary and Unary Operators 11.2.2 Predefined Meanings for Operators 11.2.3 Operators and User-Defined Types 11.2.4 Operators in Namespaces</p><p>11.3 A Complex Number Type</p><p>11.3.1 Member and Nonmember Operators 11.3.2 Mixed-Mode Arithmetic 11.3.3 Initialization 11.3.4 Copying 11.3.5 Constructors and Conversions 11.3.6 Literals 11.3.7 Additional Member Functions 11.3.8 Helper Functions</p><p>11.4 Conversion Operators</p><p>11.4.1 Ambiguities</p><p>11.5 Friends</p><p>11.5.1 Finding Friends 11.5.2 Friends and Members</p><p>11.6 Large Objects 11.7 Essential Operators</p><p>11.7.1 Explicit Constructors</p><p>11.8 Subscripting 11.9 Function Call 11.10 Dereferencing 11.11 Increment and Decrement 11.12 A String Class 11.13 Advice 11.14 Exercises</p><p>12 Derived Classes</p><p>12.1 Introduction 12.2 Derived Classes 12.2.1 Member Functions 12.2.2 Constructors and Destructors 12.2.3 Copying 12.2.4 Class Hierarchies 12.2.5 Type Fields 12.2.6 Virtual Functions</p><p>12.3 Abstract Classes 12.4 Design of Class Hierarchies</p><p>12.4.1 A Traditional Class Hierarchy</p><p>12.4.1.1 Critique</p><p>12.4.2 Abstract Classes 12.4.3 Alternative Implementations</p><p>12.4.3.1 Critique</p><p>12.4.4 Localizing Object Creation</p><p>12.5 Class Hierarchies and Abstract Classes 12.6 Advice 12.7 Exercises</p><p>13 Templates</p><p>13.1 Introduction 13.2 A Simple String Template</p><p>13.2.1 Defining a Template 13.2.2 Template Instantiation 13.2.3 Template Parameters 13.2.4 Type Equivalence 13.2.5 Type Checking</p><p>13.3 Function Templates</p><p>13.3.1 Function Template Arguments 13.3.2 Function Template Overloading</p><p>13.4 Using Template Arguments to Specify Policy</p><p>13.4.1 Default Template Parameters</p><p>13.5 Specialization</p><p>13.5.1 Order of Specializations 13.5.2 Template Function Specialization 13.6 Derivation and Templates</p><p>13.6.1 Parameterization and Inheritance 13.6.2 Member Templates 13.6.3 Inheritance Relationships</p><p>13.6.3.1 Template Conversions</p><p>13.7 Source Code Organization 13.8 Advice 13.9 Exercises</p><p>14 Exception Handling</p><p>14.1 Error Handling</p><p>14.1.1 Alternative Views on Exceptions</p><p>14.2 Grouping of Exceptions</p><p>14.2.1 Derived Exceptions 14.2.2 Composite Exceptions</p><p>14.3 Catching Exceptions</p><p>14.3.1 Re-Throw 14.3.2 Catch Every Exception</p><p>14.3.2.1 Order of Handlers</p><p>14.4 Resource Management</p><p>14.4.1 Using Constructors and Destructors 14.4.2 Auto_ptr 14.4.3 Caveat 14.4.4 Exceptions and New 14.4.5 Resource Exhaustion 14.4.6 Exceptions in Constructors</p><p>14.4.6.1 Exceptions and Member Initialization 14.4.6.1 Exceptions and Copying</p><p>14.4.7 Exceptions in Destructors</p><p>14.5 Exceptions That Are Not Errors 14.6 Exception Specifications</p><p>14.6.1 Checking Exception Specifications 14.6.2 Unexpected Exceptions 14.6.3 Mapping Exceptions 14.6.3.1 User Mapping of Exceptions 14.6.3.2 Recovering the Type of an Exception</p><p>14.7 Uncaught Exceptions 14.8 Exceptions and Efficiency 14.9 Error-Handling Alternatives 14.10 Standard Exceptions 14.11 Advice 14.12 Exercises</p><p>15 Class Hierarchies</p><p>15.1 Introduction and Overview 15.2 Multiple Inheritance</p><p>15.2.1 Ambiguity Resolution 15.2.2 Inheritance and Using-Declarations 15.2.3 Replicated Base Classes</p><p>15.2.3.1 Overriding</p><p>15.2.4 Virtual Base Classes</p><p>15.2.4.1 Programming Virtual Bases</p><p>15.2.5 Using Multiple Inheritance</p><p>15.2.5.1 Overriding Virtual Base Functions</p><p>15.3 Access Control</p><p>15.3.1 Protected Members</p><p>15.3.1.1 Use of Protected Members</p><p>15.3.2 Access to Base Classes</p><p>15.3.2.1 Multiple Inheritance and Access Control 15.3.2.2 Using-Declarations and Access Control</p><p>15.4 Run-Time Type Information</p><p>15.4.1 Dynamic_cast</p><p>15.4.1.1 Dynamic_cast of References</p><p>15.4.2 Navigating Class Hierarchies</p><p>15.4.2.1 Static and Dynamic Casts</p><p>15.4.3 Class Object Construction and Destruction 15.4.4 Typeid and Extended Type Information</p><p>15.4.4.1 Extended Type Information</p><p>15.4.5 Uses and Misuses of RTTI</p><p>15.5 Pointers to Members</p><p>15.5.1 Base and Derived Classes</p><p>15.6 Free Store</p><p>15.6.1 Array Allocation 15.6.2 ``Virtual Constructors''</p><p>15.7 Advice 15.8 Exercises</p><p>Part III: The Standard Library ... pg 427</p><p>16 Library Organization and Containers 16.1 Standard Library Design</p><p>16.1.1 Design Constraints 16.1.2 Standard Library Organization 16.1.3 Language Support</p><p>16.2 Container Design</p><p>16.2.1 Specialized Containers and Iterators 16.2.2 Based Containers 16.2.3 STL Containers</p><p>16.3 Vector</p><p>16.3.1 Types 16.3.2 Iterators 16.3.3 Element Access 16.3.4 Constructors 16.3.5 Stack Operations 16.3.6 List Operations 16.3.7 Addressing Elements 16.3.8 Size and Capacity 16.3.9 Other Member Functions 16.3.10 Helper Functions 16.3.11 Vector</p><p>16.4 Advice 16.5 Exercises</p><p>17 Standard Containers 17.1 Standard Containers</p><p>17.1.1 Operations Summary 17.1.2 Container Summary 17.1.3 Representation 17.1.4 Element Requirements</p><p>17.1.4.1 Comparisons 17.1.4.2 Other Relational Operators</p><p>17.2 Sequences</p><p>17.2.1 Vector 17.2.2 List</p><p>17.2.2.1 Splice, Sort, and Merge 17.2.2.2 Front Operations 17.2.2.3 Other Operations</p><p>17.2.3 Deque</p><p>17.3 Sequence Adapters</p><p>17.3.1 Stack 17.3.2 Queue 17.3.3 Priority Queue</p><p>17.4 Associative Containers</p><p>17.4.1 Map</p><p>17.4.1.1 Types 17.4.1.2 Iterators and Pairs 17.4.1.3 Subscripting 17.4.1.4 Constructors 17.4.1.5 Comparisons 17.4.1.6 Map Operations 17.4.1.7 List Operations 17.4.1.8 Other Functions</p><p>17.4.2 Multimap 17.4.3 Set 17.4.4 Multiset</p><p>17.5 Almost Containers</p><p>17.5.1 String 17.5.2 Valarray 17.5.3 Bitset 17.5.3.1 Constructors 17.5.3.2 Bit Manipulation Operations 17.5.3.3 Other Operations</p><p>17.5.4 Built-In Arrays</p><p>17.6 Defining a New Container</p><p>17.6.1 Hash_map 17.6.2 Representation and Construction</p><p>17.6.2.1 Lookup 17.6.2.2 Erase and Resize 17.6.2.3 Hashing</p><p>17.6.3 Other Hashed Associative Containers</p><p>17.7 Advice 17.8 Exercises</p><p>18 Algorithms and Function Objects</p><p>18.1 Introduction 18.2 Overview of Standard Library Algorithms 18.3 Sequences and Containers</p><p>18.3.1 Input Sequences</p><p>18.4 Function Objects</p><p>18.4.1 Function Object Bases 18.4.2 Predicates</p><p>18.4.2.1 Overview of Predicates</p><p>18.4.3 Arithmetic Function Objects 18.4.4 Binders, Adapters, and Negaters</p><p>18.4.4.1 Binders 18.4.4.2 Member Function Adapters 18.4.4.3 Pointer to Function Adapters 18.4.4.4 Negaters</p><p>18.5 Nonmodifying Sequence Algorithms</p><p>18.5.1 For_each 18.5.2 The Find Family 18.5.3 Count 18.5.4 Equal and Mismatch 18.5.5 Search 18.6 Modifying Sequence Algorithms</p><p>18.6.1 Copy 18.6.2 Transform 18.6.3 Unique</p><p>18.6.3.1 Sorting Criteria</p><p>18.6.4 Replace 18.6.5 Remove 18.6.6 Fill and Generate 18.6.7 Reverse and Rotate 18.6.8 Swap</p><p>18.7 Sorted Sequences</p><p>18.7.1 Sorting 18.7.2 Binary Search 18.7.3 Merge 18.7.4 Partitions 18.7.5 Set Operations on Sequences</p><p>18.8 Heaps 18.9 Min and Max 18.10 Permutations 18.11 C-Style Algorithms 18.12 Advice 18.13 Exercises</p><p>19 Iterators and Allocators</p><p>19.1 Introduction 19.2 Iterators and Sequences</p><p>19.2.1 Iterator Operations 19.2.2 Iterator Traits 19.2.3 Iterator Categories 19.2.4 Inserters 19.2.5 Reverse Iterators 19.2.6 Stream Iterators</p><p>19.2.6.1 Stream Buffers</p><p>19.3 Checked Iterators</p><p>19.3.1 Exceptions, Containers, and Algorithms</p><p>19.4 Allocators</p><p>19.4.1 The Standard Allocator 19.4.2 A User-Defined Allocator 19.4.3 Generalized Allocators 19.4.4 Uninitialized Memory 19.4.5 Dynamic Memory 19.4.6 C-Style Allocation</p><p>19.5 Advice 19.6 Exercises 20 Strings</p><p>20.1 Introduction 20.2 Characters</p><p>20.2.1 Character Traits</p><p>20.3 Basic_string</p><p>20.3.1 Types 20.3.2 Iterators 20.3.3 Element Access 20.3.4 Constructors 20.3.5 Errors 20.3.6 Assignment 20.3.7 Conversion to C-Style Strings 20.3.8 Comparisons 20.3.9 Insert 20.3.10 Concatenation 20.3.11 Find 20.3.12 Replace 20.3.13 Substrings 20.3.14 Size and Capacity 20.3.15 I/O Operations 20.3.16 Swap</p><p>20.4 The C Standard Library</p><p>20.4.1 C-Style Strings 20.4.2 Character Classification</p><p>20.5 Advice 20.6 Exercises</p><p>21 Streams</p><p>21.1 Introduction 21.2 Output</p><p>21.2.1 Output Streams 21.2.2 Output of Built-In Types 21.2.3 Output of User-Defined Types</p><p>21.2.3.1 Virtual Output Functions 21.3 Input</p><p>21.3.1 Input Streams 21.3.2 Input of Built-In Types 21.3.3 Stream State 21.3.4 Input of Characters 21.3.5 Input of User-Defined Types 21.3.6 Exceptions 21.3.7 Tying of Streams 21.3.8 Sentries</p><p>21.4 Formatting</p><p>21.4.1 Format State</p><p>21.4.1.1 Copying Format State</p><p>21.4.2 Integer Output 21.4.3 Floating-Point Output 21.4.4 Output Fields 21.4.5 Field Adjustment 21.4.6 Manipulators</p><p>21.4.6.1 Manipulators Taking Arguments 21.4.6.2 Standard I/O Manipulators 21.4.6.3 User-Defined Manipulators</p><p>21.5 File Streams and String Streams</p><p>21.5.1 File Streams 21.5.2 Closing of Streams 21.5.3 String Streams</p><p>21.6 Buffering</p><p>21.6.1 Output Streams and Buffers 21.6.2 Input Streams and Buffers 21.6.3 Streams and Buffers 21.6.4 Stream Buffers</p><p>21.7 Locale</p><p>21.7.1 Stream Callbacks</p><p>21.8 C Input/Output 21.9 Advice 21.10 Exercises</p><p>22 Numerics 22.1 Introduction 22.2 Numeric Limits</p><p>22.2.1 Limit Macros</p><p>22.3 Standard Mathematical Functions 22.4 Vector Arithmetic</p><p>22.4.1 Valarray Construction 22.4.2 Valarray Subscripting and Assignment 22.4.3 Member Operations 22.4.4 Nonmember Operations 22.4.5 Slices 22.4.6 Slice_array 22.4.7 Temporaries, Copying, and Loops 22.4.8 Generalized Slices 22.4.9 Masks 22.4.10 Indirect Arrays</p><p>22.5 Complex Arithmetic 22.6 Generalized Numeric Algorithms</p><p>22.6.1 Accumulate 22.6.2 Inner_product 22.6.3 Incremental Change</p><p>22.7 Random Numbers 22.8 Advice 22.9 Exercises</p><p>Part IV: Design Using C++ ... pg 689</p><p>23 Development and Design</p><p>23.1 Overview 23.2 Introduction 23.3 Aims and Means 23.4 The Development Process</p><p>23.4.1 The Development Cycle</p><p>23.4.2 Design Aims 23.4.3 Design Steps</p><p>23.4.3.1 Step 1: Find Classes 23.4.3.2 Step 2: Specify Operations 23.4.3.3 Step 3: Specify Dependencies 23.4.3.4 Step 4: Specify Interfaces 23.4.3.5 Reorganization of Class Hierarchies 23.4.3.6 Use of Models 23.4.4 Experimentation and Analysis 23.4.5 Testing 23.4.6 Software Maintenance 23.4.7 Efficiency</p><p>23.5 Management</p><p>23.5.1 Reuse 23.5.2 Scale 23.5.3 Individuals 23.5.4 Hybrid Design</p><p>23.6 Annotated Bibliography 23.7 Advice</p><p>24 Design and Programming</p><p>24.1 Overview 24.2 Design and Programming Language</p><p>24.2.1 Ignoring Classes 24.2.2 Avoiding Inheritance 24.2.3 Ignoring Static Type Checking 24.2.4 Avoiding Programming 24.2.5 Using Class Hierarchies Exclusively</p><p>24.3 Classes</p><p>24.3.1 What Do Classes Represent? 24.3.2 Class Hierarchies</p><p>24.3.2.1 Dependencies within a Class Hierarchy</p><p>24.3.3 Containment Relationships 24.3.4 Containment and Inheritance</p><p>24.3.4.1 Member/Hierarchy Tradeoffs 24.3.4.2 Containment/Hierarchy Tradeoffs</p><p>24.3.5 Use Relationships 24.3.6 Programmed-In Relationships 24.3.7 Relationships within a Class</p><p>24.3.7.1 Invariants 24.3.7.2 Assertions 24.3.7.3 Preconditions and Postconditions 24.3.7.4 Encapsulation</p><p>24.4 Components</p><p>24.4.1 Templates 24.4.2 Interfaces and Implementations 24.4.3 Fat Interfaces</p><p>24.5 Advice</p><p>25 Roles of Classes</p><p>25.1 Kinds of Classes 25.2 Concrete Types</p><p>25.2.1 Reuse of Concrete Types</p><p>25.3 Abstract Types 25.4 Node Classes</p><p>25.4.1 Changing Interfaces</p><p>25.5 Actions 25.6 Interface Classes</p><p>25.6.1 Adjusting Interfaces</p><p>25.7 Handle Classes</p><p>25.7.1 Operations in Handles</p><p>25.8 Application Frameworks 25.9 Advice 25.10 Exercises </p><p>Appendices:</p><p>A The C++ Grammar ... pg 793</p><p>A.1 Introduction A.2 Keywords A.3 Lexical Conventions A.4 Programs A.5 Expressions A.6 Statements A.7 Declarations</p><p>A.7.1 Declarators</p><p>A.8 Classes</p><p>A.8.1 Derived Classes</p><p>A.8.2 Special Member Functions A.8.3 Overloading A.9 Templates A.10 Exception Handling A.11 Preprocessing Directives</p><p>B Compatibility</p><p>B.1 Introduction B.2 C/C++ Compatibility</p><p>B.2.1 ``Silent'' Differences B.2.2 C Code That Is Not C++ B.2.3 Deprecated Features B.2.4 C++ Code That Is Not C</p><p>B.3 Coping with Older C++ Implementations</p><p>B.3.1 Headers B.3.2 The Standard Library B.3.3 Namespaces B.3.4 Allocation Errors B.3.5 Templates B.3.6 For-Statement Initializers</p><p>C Technicalities</p><p>C.1 Introduction and Overview C.2 The Standard C.3 Character Sets</p><p>C.3.1 Restricted Character Sets C.3.2 Escape Characters C.3.3 Large Character Sets C.3.4 Signed and Unsigned Characters</p><p>C.4 Types of Integer Literals C.5 Constant Expressions C.6 Implicit Type Conversion</p><p>C.6.1 Promotions C.6.2 Conversions</p><p>C.6.2.1 Integral Conversions C.6.2.2 Floating-Point Conversions C.6.2.3 Pointer and Reference Conversions C.6.2.4 Pointer-to-Member Conversions C.6.2.5 Boolean Conversions C.6.2.6 Floating-Integral Conversions</p><p>C.6.3 Usual Arithmetic Conversions</p><p>C.7 Multidimensional Arrays C.7.1 Vectors C.7.2 Arrays C.7.3 Passing Multidimensional Arrays</p><p>C.8 Saving Space</p><p>C.8.1 Fields C.8.2 Unions C.8.3 Unions and Classes</p><p>C.9 Memory Management</p><p>C.9.1 Automatic Garbage Collection</p><p>C.9.1.1 Disguised Pointers C.9.1.2 Delete C.9.1.3 Destructors C.9.1.4 Memory Fragmentation</p><p>C.10 Namespaces</p><p>C.10.1 Convenience vs. Safety C.10.2 Nesting of Namespaces C.10.3 Namespaces and Classes</p><p>C.11 Access Control</p><p>C.11.1 Access to Members C.11.2 Access to Base Classes C.11.3 Access to Member Class C.11.4 Friendship</p><p>C.12 Pointers to Data Members C.13 Templates</p><p>C.13.1 Static Members C.13.2 Friends C.13.3 Templates as Template Parameters C.13.4 Typename and Template C.13.6 Template as a Qualifier C.13.7 Instantiation C.13.8 Name Binding</p><p>C.13.8.1 Dependent Names C.13.8.2 Point of Definition Binding C.13.8.3 Point of Instantiation Binding C.13.8.4 Templates and Namespaces</p><p>C.13.9 When Is a Specialization Needed?</p><p>C.13.9.1 Template Function Instantiation C.13.10 Explicit Instantiation</p><p>C.14 Advice</p><p>D Locales</p><p>D.1 Handling Cultural Differences</p><p>D.1.1 Programming Cultural Differences</p><p>D.2 The locale Class</p><p>D.2.1 Named Locales</p><p>D.2.1.1 Constructing New Locales D.2.2 Copying and Comparing Locales D.2.3 The global() and the classic() Locales D.2.4 Comparing Strings</p><p>D.3 Facets</p><p>D.3.1 Accessing Facets in a Locale D.3.2 A Simple User-Defined Facet D.3.3 Uses of Locales and Facets</p><p>D.4 Standard Facets</p><p>D.4.1 String Comparison</p><p>D.4.1.1 Named Collate</p><p>D.4.2 Numeric Input and Output</p><p>D.4.2.1 Numeric Punctuation D.4.2.2 Numeric Output D.4.2.3 Numeric Input</p><p>D.4.3 Input and Output of Monetary Values</p><p>D.4.3.1 Money Punctuation D.4.3.2 Money Output D.4.3.3 Money Input</p><p>D.4.4 Date and Time Input and Output</p><p>D.4.4.1 Clocks and Timers D.4.4.2 A Date Class D.4.4.3 Date and Time Output D.4.4.4 Date and Time Input D.4.4.5 A More Flexible Date Class D.4.4.6 Specifying a Date Format D.4.4.7 A Date Input Facet</p><p>D.4.5 Character Classification</p><p>D.4.5.1 Convenience Interfaces</p><p>D.4.6 Character Code Conversion D.4.7 Messages</p><p>D.4.7.1 Using Messages from Other Facets</p><p>D.5 Advice D.6 Exercises</p><p>E Standard-Library Exception Safety</p><p>E.1 Introduction E.2 Exception Safety E.3 Exception-Safe Implementation Techniques</p><p>E.3.1 A Simple Vector E.3.2 Representing Memory Explicitly E.3.3 Assignment E.3.4 push_back() E.3.5 Constructors and Invariants</p><p>E.3.5.1 Using init() Functions E.3.5.2 Relying on a Default Valid State E.3.5.3 Delaying resource acquisition</p><p>E.4 Standard Container Guarantees</p><p>E.4.1 Insertion and Removal of Elements E.4.2 Guarantees and Tradeoffs E.4.3 Swap E.4.4 Initialization and Iterators E.4.5 References to Elements E.4.6 Predicates</p><p>E.5 The Rest of the Standard Library</p><p>E.5.1 Strings E.5.2 Streams E.5.3 Algorithms E.5.4 Valarray and Complex E.5.5 The C Standard Library</p><p>E.6 Implications for Library Users E.7 Advice E.8 Exercises </p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages25 Page
-
File Size-