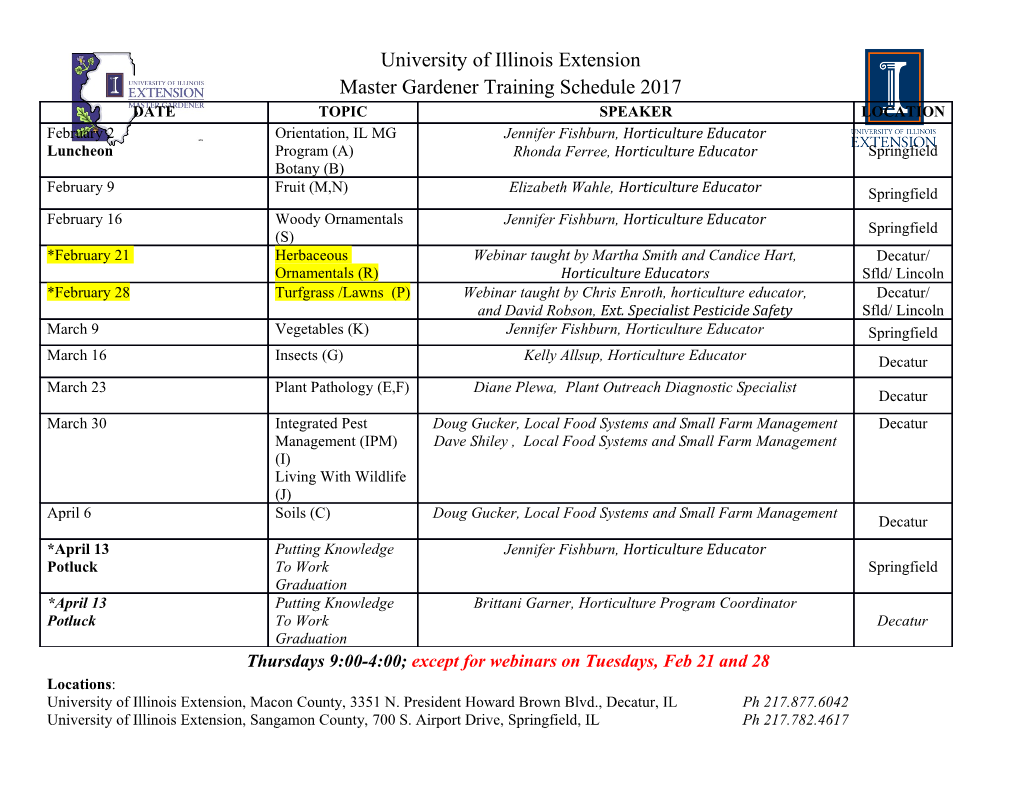
<p>//******************************************************************** // Mortgage.java Author: Lewis/Loftus // // Solution to Programming Project 5.14 //******************************************************************** public class Mortgage { //------// Creates and displays the mortgage calculator GUI. //------public static void main (String[] args) { MortgageGUI calculator = new MortgageGUI(); calculator.display(); } } //******************************************************************** // MortgageGUI.java Author: Lewis/Loftus // // Solution to Programming Project 5.14 //******************************************************************** import java.awt.Color; import java.awt.Dimension; import java.awt.event.*; import javax.swing.*; import java.text.DecimalFormat; public class MortgageGUI { private int WIDTH = 260; private int HEIGHT = 200;</p><p> private JFrame frame; private JPanel panel; private JLabel amountLabel, interestLabel, durationLabel; private JLabel monthlyLabel, paymentLabel, interestPaidLabel, lostLabel; private JTextField amountField, interestField, durationField; private JButton calculateButton;</p><p>//------// Sets up the GUI. //------public MortgageGUI() { frame = new JFrame ("Mortgage Calculator"); frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE);</p><p> amountLabel = new JLabel ("Mortgage amount: $"); interestLabel = new JLabel ("Annual interest rate: "); JLabel percentLabel = new JLabel("%"); durationLabel = new JLabel ("Loan duration:"); monthlyLabel = new JLabel ("Total of monthly payments: $"); JLabel yearLabel = new JLabel(" years"); interestPaidLabel = new JLabel ("Total interest paid: $"); paymentLabel = new JLabel("------"); lostLabel = new JLabel("------");</p><p> amountField = new JTextField (10); interestField = new JTextField (5); durationField = new JTextField(5);</p><p> calculateButton = new JButton(" Calculate "); calculateButton.addActionListener (new calculateListener());</p><p> panel = new JPanel(); panel.setPreferredSize (new Dimension(WIDTH, HEIGHT)); panel.setBackground (Color.red); panel.add (amountLabel); panel.add (amountField); panel.add (interestLabel); panel.add (interestField); panel.add (percentLabel); panel.add (durationLabel); panel.add (durationField); panel.add (yearLabel); panel.add (monthlyLabel); panel.add (paymentLabel); panel.add (interestPaidLabel); panel.add (lostLabel); panel.add (calculateButton);</p><p> frame.getContentPane().add (panel); }</p><p>//------// Displays the primary application frame. //------public void display() { frame.pack(); frame.show(); }</p><p>//***************************************************************** // Represents an action listener for the calculate button. //***************************************************************** private class calculateListener implements ActionListener { //------// Performs the calcuation when the calculate button is pressed. //------public void actionPerformed (ActionEvent event) { DecimalFormat money = new DecimalFormat("#,##0.00");</p><p> float amount = Float.parseFloat(amountField.getText()); float interest = Float.parseFloat(interestField.getText()); float duration = Float.parseFloat(durationField.getText());</p><p> float rate = interest / (12 * 100) ; float numMonths = duration * 12;</p><p> double totalPayment = numMonths * amount / ( (1/rate) - ( 1 / (rate * Math.pow((1 + rate), numMonths))));</p><p> double totalInterest = totalPayment - amount;</p><p> paymentLabel.setText(money.format(totalPayment)); lostLabel.setText(money.format(totalInterest));</p><p>} } } //******************************************************************** // Histogram.java Author: Lewis and Loftus // // Solution to Programming Project 6.4 //******************************************************************** import cs1.Keyboard; public class Histogram { public static void main (String [] args) { int[] ranges = new int [10]; int box;</p><p>System.out.println ("Enter some numbers between 0 and 100."); System.out.print ("Signal the end by entering "); System.out.println ("a number out of that range.");</p><p> int entered = Keyboard.readInt (); while (entered >= 1 && entered <= 100) { box = (entered - 1) / 10; ranges[box] ++; entered = Keyboard.readInt (); }</p><p>// print histogram for (box = 0; box < 10; box++) { System.out.print ((10 * box + 1) + "-"); System.out.print ((10 * box + 10) + "\t|"); for (int count = 0; count < ranges[box]; count++) System.out.print ("*"); System.out.println (); }</p><p>} } //******************************************************************** // DrawStars.java Author: Lewis and Loftus // // Solution to Programming Project 6.16 //******************************************************************** import javax.swing.JApplet; import java.awt.Color; import java.awt.Point; import java.awt.Graphics; import java.util.Random; public class DrawStars extends JApplet { private final int APPLET_WIDTH = 200; private final int APPLET_HEIGHT = 200;</p><p> private Random gen = new Random ();</p><p>//------// Draws several stars of random size and color in random // locations. //------public void paint (Graphics page) { setBackground (Color.black); Star star; int radius, red, green, blue, xCenter, yCenter; Color color; Point center;</p><p> for (int count = 1; count <= 10; count++) { radius = gen.nextInt(10) + 10;</p><p> red = gen.nextInt (156) + 100; green = gen.nextInt (156) + 100; blue = gen.nextInt (156) + 100; color = new Color (red, green, blue);</p><p> xCenter = gen.nextInt (180) + 10; yCenter = gen.nextInt (180) + 10; center = new Point (xCenter, yCenter);</p><p> star = new Star (radius, color); star.draw (center, page); } } } //******************************************************************** // Star.java Author: Lewis and Loftus // // Solution to Programming Project 6.16 //******************************************************************** import java.awt.Color; import java.awt.Point; import java.awt.Graphics; public class Star { private int radius = 10; private Color color = Color.yellow;</p><p>//------// Sets up the star with a specified radius and color. //------public Star (int r, Color c) { radius = r; color = c; }</p><p>//------// Draw the star on the specified Graphics object //------public void draw (Point center, Graphics page) { int[] x = new int [12]; int[] y = new int [12];</p><p> int oneThird = radius/3; int half = radius/2; int twoThirds = radius*2/3;</p><p> int centerX = center.x; int centerY = center.y;</p><p> x[0] = centerX; y[0] = centerY - radius; x[1] = centerX + oneThird; y[1] = centerY - oneThird; x[2] = centerX + twoThirds; y[2] = centerY - twoThirds; x[3] = centerX + half; y[3] = centerY; x[4] = centerX + twoThirds; y[4] = centerY + twoThirds; x[5] = centerX + oneThird; y[5] = centerY + oneThird; x[6] = centerX; y[6] = centerY + radius; x[7] = centerX - oneThird; y[7] = centerY + oneThird; x[8] = centerX - twoThirds; y[8] = centerY + twoThirds; x[9] = centerX - half; y[9] = centerY; x[10] = centerX - twoThirds; y[10] = centerY - twoThirds; x[11] = centerX - oneThird; y[11] = centerY - oneThird;</p><p> page.setColor (color); page.fillPolygon (x, y, x.length); } }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-