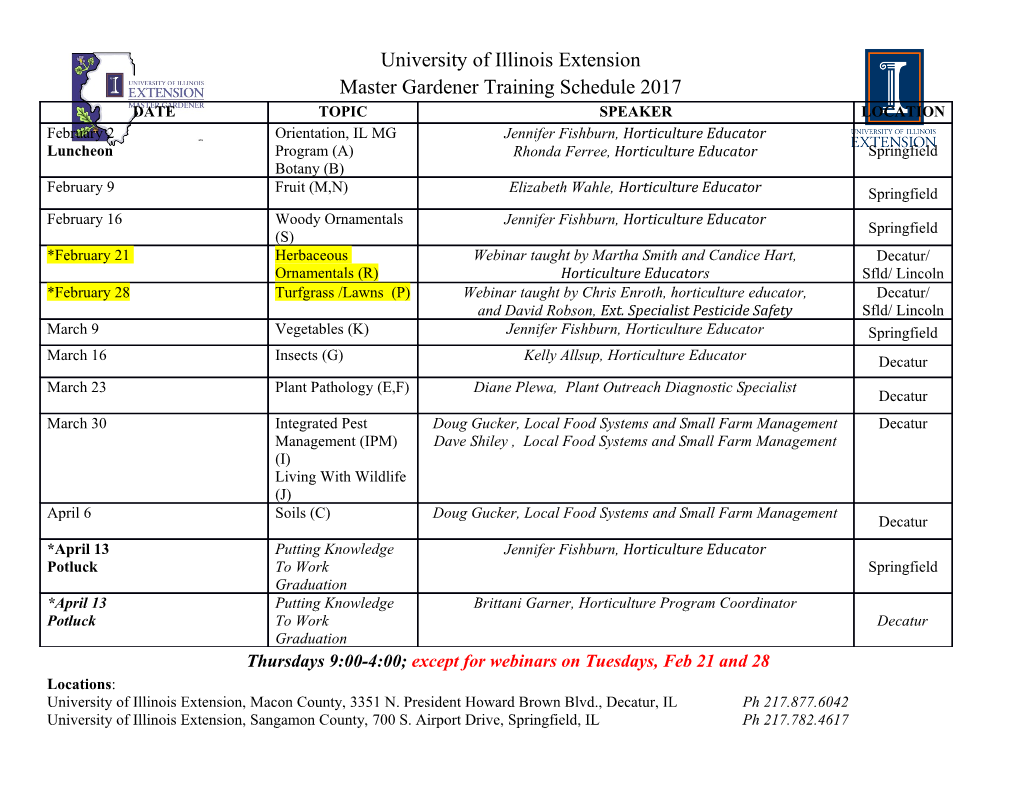
<p>CSC142, Computer Science II, Project 5</p><p>Due via D2L. Late programs are not acceptable.</p><p>1. For each program (5 points each, total 25) below, show what is displayed on the screen when the code executes. import java.util.Arrays; public class HelloWorld { public static void main(String [] args) { int [] arr = {3, 7, 5}; String arrStr = Arrays.toString(arr); System.out.println(arrStr);</p><p> int max = Math.max(3, -5); System.out.println(max);</p><p>String s = HelloWorld.getHello(); System.out.println(s); }</p><p> public static String getHello() { return "Hello, world!"; } }</p><p> public class HelloInline { // The order of method declarations does not matter!! public static String getHello() { return "Hello, world!"; }</p><p>// Program execution always begins with the first line of main(). public static void main(String [] args) { System.out.println(HelloInline.getHello()); System.out.println(getHello()); // Each time the command name is executed, the method is called. // We can call the method as many times as we like. System.out.println(getHello()); System.out.println(getHello()); } } public class SimpleMath { public static int max(int a, int b, int c) { int temp = Math.max(a, b); return Math.max(temp, c); }</p><p> public static double average(int a, int b, int c) { double sum = a + b + c; return sum / 3.0; }</p><p> public static void main(String [] args) { int x = 7; int y = 9; int z = 4;</p><p>System.out.println(max(x,y,z)); System.out.println(average(x,y,z)); } } public class NamingAndScope { public static void main(String [] args) { int x = 3; x = x(); System.out.println(x); x = x(x+1); System.out.println(x); for(int i=0; i<2; i++) { x = x(x+3); System.out.println(x); } }</p><p> public static int x() { int x = 5; return x + 7; }</p><p> public static int x(int x) { return x + 1; } } public class WhatsPrinted01 { public static void func(int A[]) { for (int i=1; i<A.length; i++) A[i]+=A[i-1]; } public static void main(String args[]) { int A[] = {10,20,30}; func(A); System.out.println(A[2]); } } public class WhatsPrinted02 { public static int func(int A[], int B[]) { A = B; return A[1]; } public static void main(String args[]) { int A[] = {10,20,30}; int B[] = {40,50,60};</p><p> int x = func(A, B); System.out.println(x + " " + A[1]); } }</p><p> import java.util.Arrays; public class MysteryClass1 { public static void main(String [] args) { Point[] p = {new Point(2,4), new Point(3,5), new Point(9,11)}; mystery1(p); System.out.println(Arrays.toString(p));</p><p> p = mystery2(p); System.out.println(Arrays.toString(p)); } public static void mystery1(Point [] ps) { for(int i=0; i<ps.length; i++) { ps[i].setLocation(ps[i].x + i, ps[i].y); } } public static Point [] mystery2(Point [] ps) { Point [] ret = new Point[ps.length]; for(int i=0; i<ps.length; i++) { ps[i].translate(-1, 1); } return ret; } }</p><p> public class Point { public double x; // the point’s x-coordinate public double y; // the point’s y-coordinate</p><p> public Point(double x, double y) // constructor of point { … } public double distance (Point p) // how far away this point is from point p { … } public void setLocation (double x, double y) // sets the point’s x and y to the given values { … } public void translate (double dx, double dy) // adjusts the point’s x and y by the given amounts { … } }</p><p>2. Develop the following programs. 1. (15 points) Programming challenge 10 in book, on page 493, develop ArrayOperation.java to support the ArrayOperations.java (http://www.cs.wcupa.edu/~zjiang/ArrayOperations.java) </p><p>2. (15 points) Programming challenge 3 in book, on page 491, develop Account.java to support the AccountApplication.java (http://www.cs.wcupa.edu/~zjiang/AccountApplication.java)</p><p>3. (15 points) Programming challenge 2 in book, on page 490, develop Payroll.java to support the PayrollApplication.java (http:// http://www.cs.wcupa.edu/~zjiang/PayrollApplication.java) </p><p>4. (15 points) Programming challenge 5 in book, on page 491, develop DriversExam.java to support the DL.java (http://www.cs.wcupa.edu/~zjiang/DL.java) . 5. (20 points) Programming challenge 7 in book, on page 492, develop GradeBook.java to support the Grade.java (http://www.cs.wcupa.edu/~zjiang/Grade.java)</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages4 Page
-
File Size-