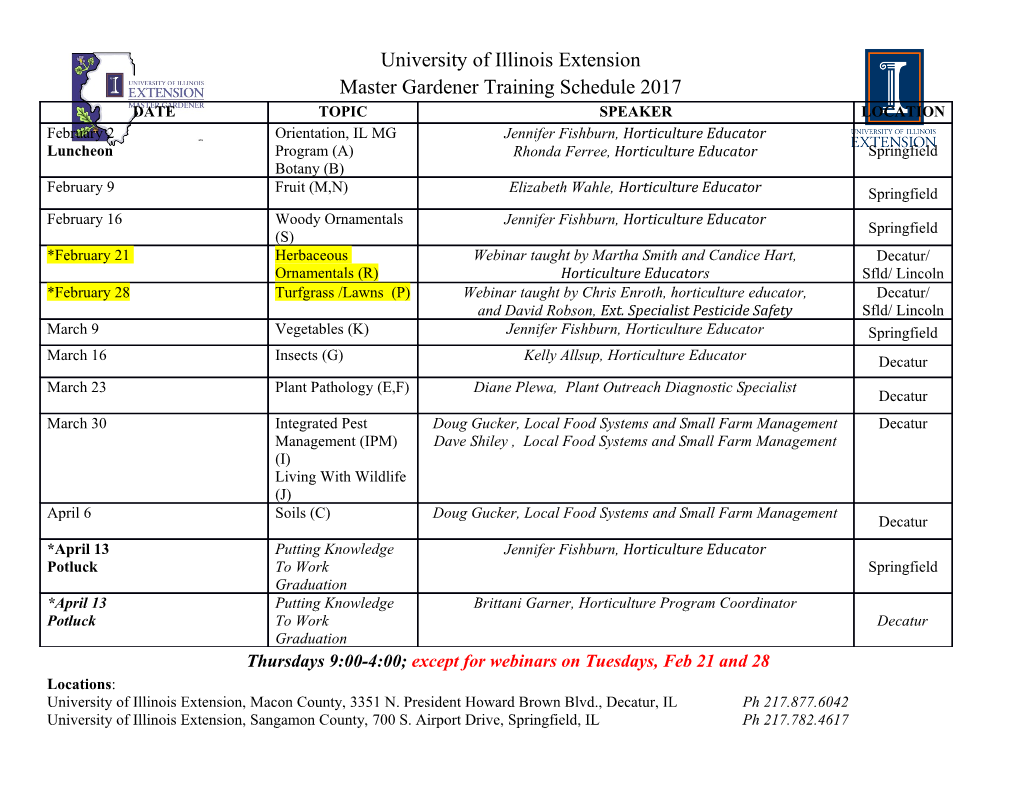
<p> Review of classes & OO design</p><p>Classes class Point {</p><p>Instance variables must (nearly private double x, y; // coordinates. always) be private, not public.</p><p>Point(double x0, double y0){ All-args constructor. x = x0; y = y0; }</p><p>No-args constructor. Default values Point(){}; for instance variables apply.</p><p> void get() { x = Console.readDouble(); Or use Scanner for keyboard input. Console simpler but not standard. y = Console.readDouble(); }</p><p>Name toString essential, as is public String toString() { public. Understand why. Understand return "(" + x + "," + y + ")"; that toString is an instance method, } not a static method, and why. </p><p> double distance(Point r) { Note distance is an instance // distance from r method. In x-r.x the x referred to is the x-coordinate of this object, i.e. double xdist = x-r.x; the one in which the invoked instance double ydist = y-r.y; of distance resides. return(Math.sqrt(xdist*xdist+ ydist*ydist)); Distinguish between function } methods such as distance and } toString, and procedure methods such as get. class PointTest { // test class Point</p><p> public static void main(String args[] ) { Variables in methods have no Point p1 = new Point(); access qualifiers such as private or public. new Point p2 = new Point(); Point() is invocation of no- System.out.println("Enter two points: "); args constructor p1.get(); p2.get(); System.out.println( "Distance between is " + Example of invoking an instance p1.distance(p2)); method, here distance. Note +. // System.out.printf( // "Distance between is %.2f\n", Improved version of preceding statement, using printf and // p1.distance(p2)); formatting strings for neater output. } Note comma. }</p><p>Classes 1 Object-oriented programming: delegate work to appropriate class class Triangle { No-args constructor of Triangle private Point v1 = new Point(); provided by default (happens only when no constructors are written). private Point v2 = new Point(); private Point v3 = new Point(); Reading Point coordinates v1 etc. is not done directly, but is delegated to void get() { class Point. “Failure to delegate” v1.get(); v2.get(); v3.get(); an action to the class to which it } properly belongs is a serious error of engineering and style. public String toString() {</p><p> return v1.toString() + "-" + It is usual to omit toString as the v2.toString() + "-" + compiler supplies it by default when v3.toString(); toString header written exactly as above. So v1+ - - } " "+v2+" "+v3 ok.</p><p> double area() { // area of the triangle double a = v1.distance(v2); Calculating the distance between double b = v2.distance(v3); points is delegated to Point. Doing so directly in Triangle would double c = v3.distance(v1); constitute a “failure to delegate”. double s = (a+b+c)/2; return Math.sqrt(s*(s-a)*(s-b)*(s-c)); } } class TriangleAreas {</p><p> public static void main(String args[] ) { Triangle t1 = new Triangle(); Triangle t2 = new Triangle(); System.out.println( "Enter coordinates of two" Reading triangles is not done directly, + " triangles: "); but is delegated to class Triangle. t1.get(); t2.get(); Doing so directly here would constitute a “failure to delegate”. System.out.print("Triangle with" + " largest area: "); if (t1.area()>t2.area()) t1.toString() also correct, but System.out.print(t1); verbose. else System.out.print(t2); } } The above code illustrates the basic rules of object-oriented programming in Java. Make sure you understand every word of it. If you don’t, you have work to do to master the basics of object-oriented programming; do the work NOW. </p><p>Classes 2</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages2 Page
-
File Size-