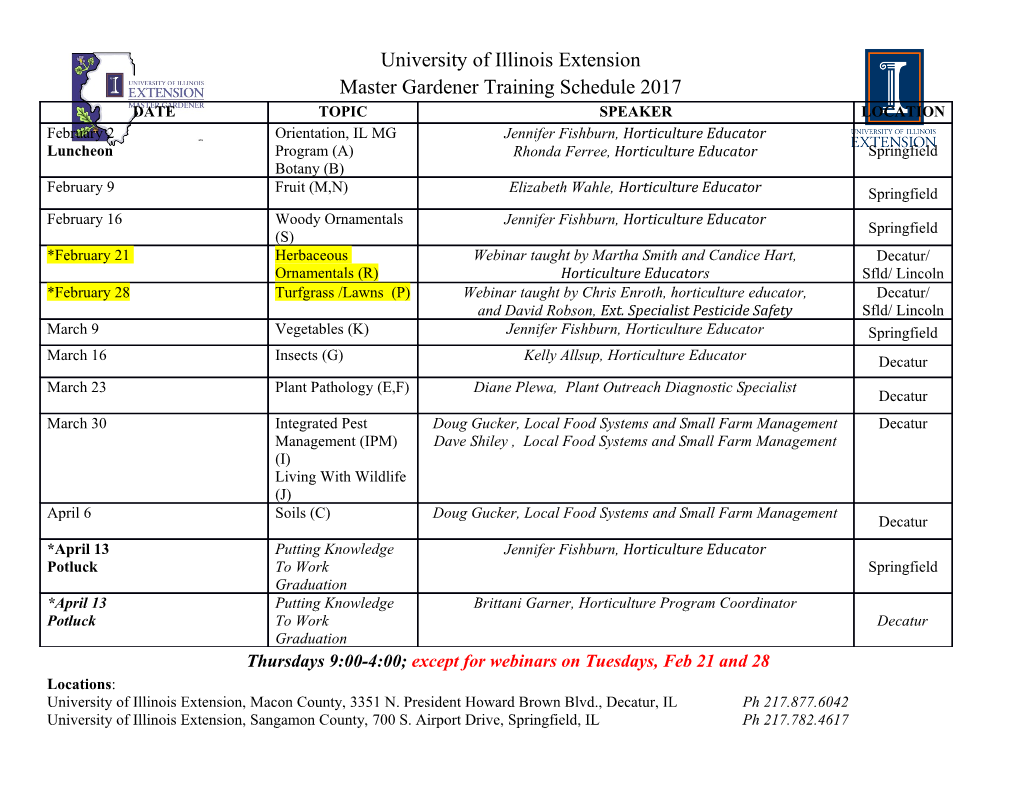
<p>2/7/07 CS 240 Exam 1 Page 1 Computer Science II Spring 2007, Wednesday, 2/7/07 100 points Name ______</p><p>Explain or give the UNIX or vi commands or keystrokes to do the following tasks. [28 pts] 1. Display all filenames (including hidden ones) found in your current directory. ______[2]</p><p>2. Display java source files found in your subdirectory cs2 ______[2]</p><p>3. Create a subdirectory in your home directory called projects. ______[2]</p><p>4. Move all .java files in your home directory to this subdirectory projects ______[2]</p><p>5. Delete all files that contain tmp anywhere in the name from your home directory. ______[2]</p><p>6. Change your current working directory up to the parent directory ______[1]</p><p>7. Display only the last 10 lines of the file test.java . ______[2]</p><p>8. Begin capturing the screen display to a file for printing later. ______[1]</p><p>9. Start the visual editor on file test.java ______[2]</p><p>10. Compile the code in test.java ______[2] 11. What keystroke designates end of input in Unix? ____ What keystroke cancels a program in Unix? ____ What character designates the current directory? _____ What keystroke erases the current input line? _____ [4] 12. Print your typescript file, 2 pages per sheet. ______[1] 13. Run the Java program first using the filename mydata as a command line argument. ______[2] 14. Run the Unix program filter redirecting the file data for input and sorts output stream. Just displays results. ______[3] 15. Give the keystroke(s) in vi to perform the following actions [8 pts] _____ move the cursor down 1 line (1 character) _____undo the previous action (1 char) _____ delete the current character (1 char) _____ start insertion mode (1 char) _____ end insert mode (1 char) _____ delete the current line (2 chars) _____ exit and do not save the file or its changes (3 chars) _____exit and save the file (2 chars) 2/7/07 CS 240 Exam 1 Page 2</p><p>16. Arrays. [7 pts] a. Give a Java code segment to define an integer array nums of size elements and use a for loop to initialize each element to its index (element 0 contains a 0, element 9 contains a 9, etc.). [4]</p><p> b. List the steps to make a filled array larger. [3]</p><p>17. Abstract Data Types. (True/False) [5 pts] a. ____ An ADT defines the data organization, its operations as well as the implementation. b. ____ All ADTs implemented as Java classes ultimately inherit from the Object class. c. ____ An abstract class can be used to define shared methods and instance variables inheritable by related subclasses. d. ____ An interface is another name for an abstract class. e. ____ ADT specifications depend on the implementation language, e.g. Java.</p><p>18. Object-oriented programming design principles. [6 pts] a. The Information Hiding Principle specifies that the implementer of a module is provided only the necessary information to ______the module and that the ______is provided only the necessary information to use the module. b. A ______-condition specifies what must be true about the inputs at the beginning of a method and a ______specifies what must be true at the end of a method, if the ______-condition holds. These assertions provide a form of a contract between the ______and the implementer. 2/7/07 CS 240 Exam 1 Page 3</p><p>19. Miscellaneous Java concepts (True/False) [10 pts] a. _____ Each Java class should be defined in its own separate file with the extension .java and that file name must match the class name. b _____ The contents of the .class file are called bytecodes, which are dependent on a particular machine. c. _____ Garbage collection in Java is the automatic recovery of memory from inaccessible objects. d. _____ The toString method that should be defined for each class is an example of an accessor. e. _____ The toString method is an example of a method that is inherited but can be overridden. f. _____ Two strings compared by the compareTo() results in an integer.. g. _____ An iterator ensures that all elements of a collection can be accessed in some order. h. _____ The class that extends an existing class is called the superclass. i. _____ The primitive types of int and double are examples where the variable refers or points to the value. j. _____Reusability is a quality software goal in object-oriented class design.</p><p>20. Number the steps of the software development cycle (Waterfall method) from 1 to 6 in correct order. [5 pts] _____ Testing _____ Maintenance _____ Design _____ Requirements identification _____ Analysis _____ Implementation </p><p>21. Rank the algorithm complexities from "fastest" to "slowest" (increasing dominance). 1 is fastest (good, low cost) and 6 is slowest (bad, high cost). [5 pts] O(2n) O(n) O(n2) O(log n) O(1) O(n2 log n)</p><p>22. For each of the cost functions below give the “best” big-O equivalence where n is the data set size and k is some constant not related to the size of data. [8 pts] ______f(n) = 2n3 + 9n2 + 50n + 100 ______f(n) = 17 + kn + 5n2 ______f(n) = kn + 4k4 ______f(n) = 8n8 +2n 2/7/07 CS 240 Exam 1 Page 4 23. Estimate the running times using the big-O notation for each of these code segments. The size of the data set is n. Assume all variables are integer. [8 pts] for(j=n; j>0; j--){ // 3 assignments } ______for (i=1; i<=n; i++) for(j=1; j<=n; j++) for(k=i; k<=n; k++){ ______// 4 assignment and 2 i/o statements } i = 1000; while(i>0){ if(i % 2 == 1){ // if i is odd ______for(j=i; j<=n; j++){ foo(j,n); //foo executes in quadratic time } // 3 assignment statements i = i-1; } for (k=n; k>0; k--){ j=0; while (j<100){ ______bar(j,n); //bar executes in linear time j=j+1; } } 2/7/07 CS 240 Exam 1 Page 5</p><p>21. Below is a segment of code similar from the text and your project. Assume Date is a class that holds the day, month and year along with a String description. A constructor can take three integers and a String, or another date instance and a string. IncDate inherits from Date and adds the increment() method that advances the day by one. In both cases the toString() method prints the date in mo/day/yr format and a method getDescription() returns the description string. [10 pts] 0 try { 1 IncDate [] events; 2 events = new IncDate[10]; 3 events[0] = new IncDate (1,15,2007, “Classes start”); 4 events[1] = new IncDate (2,2,2007, “Groundhog Day”); 5 6 IncDate today = new IncDate(2,7,2007,”CS 240 Exam 1”); 7 IncDate tomorrow = new IncDate(today,”Work on Program 2”); 8 tomorrow.______; //really make it tomorrow 9 events[2] = today; 10 events[3] = tomorrow; 11 events[4] = new IncDate (5,1,2005,”Reading Day”); 12 } catch (Exception e) 13 System.out.println(“Error in initialization: “+e.getMessage()); 14 } 15 for(i=0; i<5; i++){ 16 if(events[i].monthIs()<=2){ 17 System.out.print(events[____].toString() + “-->” + 18 events [ _____ ] . ______()+”\n”); 19 } 20 } 21</p><p> a. Fill in lines 17 and 18 to have the date of an event and its description printed. [3]</p><p> b. Fill in line 8 to make it correctly “tomorrow” from today. [1]</p><p> c. Draw a picture of the memory layout for array events, variables today and tomorrow, and objects resulting from lines 3-11. [3]</p><p> d. What is actually printed by the program segment in lines 15-20 when run? Show, don’t describe. [3]</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-