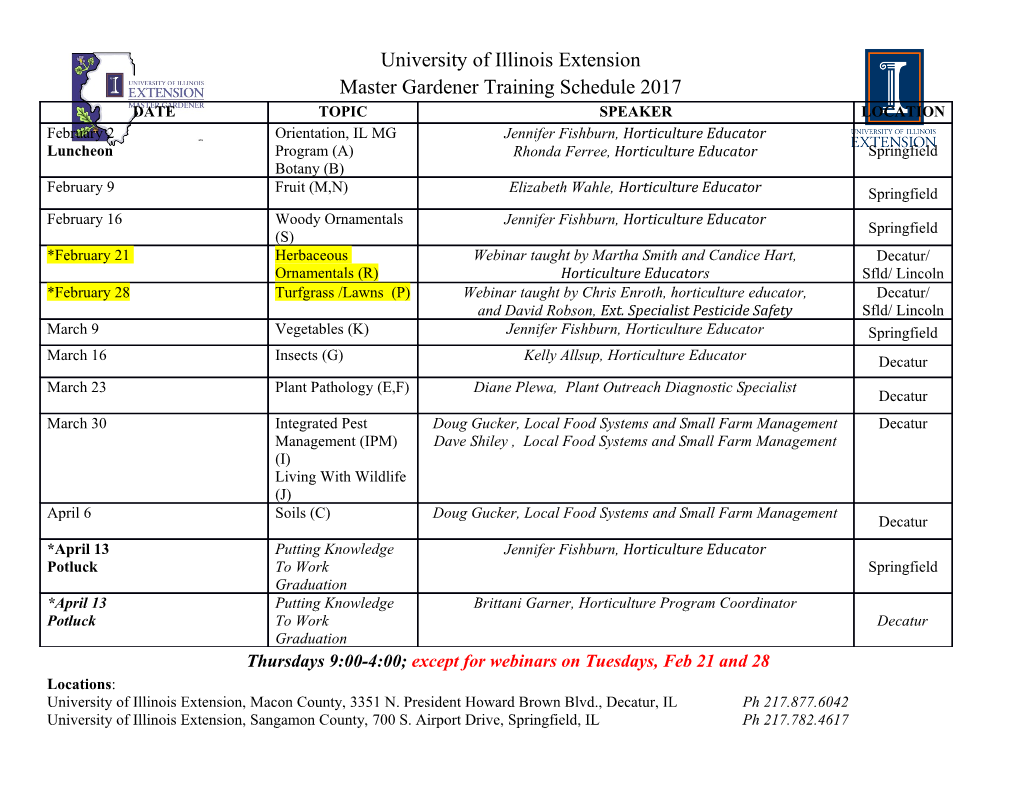
<p> Name: ______</p><p>Midterm 2 CS 20 Fall 2008</p><p>Short Answer: _____/25</p><p>Output: _____/25 removeHead: _____/15 iterative countOccurrences _____/20 recursive countOccurrences _____/15</p><p>Total: _____/100 1) Write the base case and recursive case of each of the following problems (5 pts each): a) Calculating the value 4x Base Case:</p><p>Recursive Case: b) Counting the number of occurrences of an element in a linked list Base Case:</p><p>Recursive Case: c) Your CD with initial balance b earns 4.5% interest per year. Calculate the value in year n. Base Case:</p><p>Recursive Case:</p><p>2) 10 pts - Write the recursive method, in Java, to calculate the value 4x public static int fourToTheX(int x) {</p><p>}</p><p>3) (15 pts) (2-3-5-5) public static int mystery(int x) { if (x <= 1) return 3; else return (3 + mystery(x-1)); }</p><p>What is the output for mystery(1)?</p><p>What is the output for mystery(5)?</p><p>In one sentence, describe what the previous method calculates:</p><p>What is the computational complexity of the above method? Give an explanation.</p><p>4) 10 pts - What is the output of the following code? element1 = 3; element3 = 0; element2 = element1 + 1; queue.enqueue(element2); queue.enqueue(element2+1); queue.enqueue(element1); element2 = queue.dequeue(); element1 = element2 + 1; queue.enqueue(element1); queue.enqueue(element3); while (!queue.isEmpty()); { element1 = queue.dequeue(); System.out.print(element1+”, “); } Output:</p><p>You have a doubly linked-list data structure to which you want to add some methods you will use to implement a queue. Some code is already done – defining the Node class, instance data, and the insertTail method. public class MyDoublyLinkedList{ private class Node{ private String info = null; private Node next = null; private Node prev = null; }</p><p> private Node head = null; private Node tail = null;</p><p> public void insertTail(String s){ Node n = new Node(); n.info = s; n.next = null; n.prev = tail; if (head == null) head = n; else tail.next = n; tail = n; } } // end of class MyDoublyLinkedList</p><p>5) 15 pts – add the method removeHead to class MyDoublyLinkedList – remove the first element from the linked list and return it. </p><p> public String removeHead() { } 6) (20 pts) – add a method CountOccurrences in MyDoublyLinkedList that counts the number of occurrences of the String s in the linked list. Implement this method iteratively.</p><p> public int countOccurrences(String s) { } // end of method countOccurrences 7) (15 pts) – add a method CountOccurrences in the Node class that counts the number of occurrences of the String s in the linked list. Implement this method recursively. It would first be called on the head of the linked list.</p><p> private int countOccurrences(String s) { } // end of method countOccurrences</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-