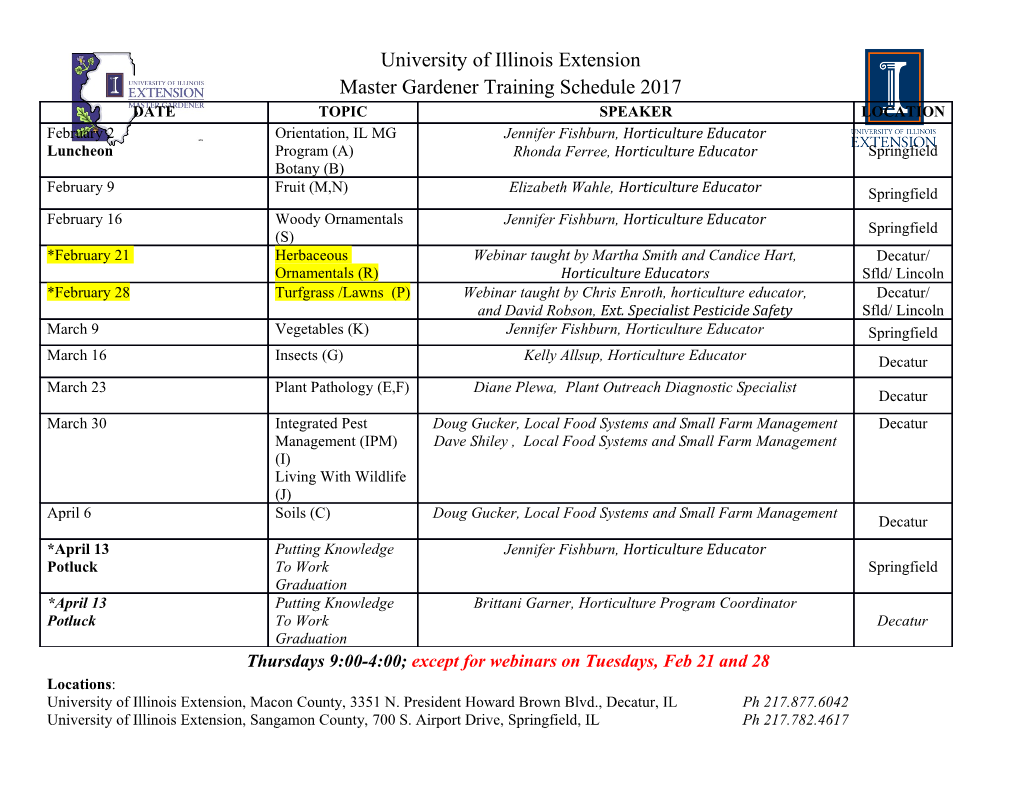
<p> ANALYSIS OF ALGORITHMS</p><p>Sorts</p><p>Merge Sort</p><p>Suppose that we want to merge (sort) an array containing two sorted halves (i.e., A[low:mid], and A[mid+1,high].</p><p>|Alow, ···, Amid|, |Amid+1,···, Ahigh |</p><p>We want to merge the two halves into an array B[low:high ]. To obtain a sorted list, we can used two pointers, l, that points to A[low], and h, that points to A[mid+1] (i.e., l=low, and h=mid+1). At each iteration, we compare A[l] with A[h], and we move the smallest of the two, to the B array. Either l or h will be increased by 1, depending upon which of A[l] or A[h] was the smallest (if A[l]=A[h], the we move either one to B, increasing the corresponding pointer). </p><p>Example:</p><p>A[ ] = 1 5 6 7 8 11 2 3 5 7 9 11 B[ ]= ^ ^ l h</p><p>A[ ] = 1 5 6 7 8 11 2 3 5 7 9 11 B[ ]=1 ^ ^ l h</p><p>A[ ] = 1 5 6 7 8 11 2 3 5 7 9 11 B[ ]= 1 2 ^ ^ l h </p><p>A[ ] = 1 5 6 7 8 11 2 3 5 7 9 11 B[ ]= 1 2 3 ^ ^ l h</p><p>A[ ] = 1 5 6 7 8 11 2 3 5 7 9 11 B[ ]= 1 2 3 5 ^ ^ l h and so on.</p><p>The upper limit for the l pointer is mid, while the upper limit for the h pointer is high. If one of the pointer reaches its limit, the remainder elements of the other half, will be pushed into the B array. If we consider the comparison as the basic operation (comparison of A[l] with A[h]), we realize that every time we make one comparison, exactly one element is pushed into the auxiliary array B. Thus the maximum number of comparison is high - low , or equivalently, the total number of elements in the array A, minus 1.</p><p>Thus, the worst-case of Merge, given a list of n elements is n-1.</p><p>A11: void Merge (int A[ ], int low, int mid, int high) // we assume that B is a global // variable { int l=low, i=low, h=mid+1, k; // i is the index corresponding to array B while ((l <=mid) && (h <=high)) { // exactly one of the pointers will reach its limit if (A[l] <=A[h]) { B[i]=A[l]; // push A[l] to B l++; //increment l } else { // we must A[h] to B B[i]=A[h]; h++; } i++; } //end while // now one of the pointer has reached its limit so we push the remaining // elements into B. if (l > mid) { // we pushed the remaining elements starting at A[h] for (k=h: k <=high; k++) { B[i]=A[k]; i++; } // end for else for (k=l; k <= mid; k++) // we push remaining elements starting at A[l] B[i]=A[k]; i++; } // end else</p><p>// Next we move B[low:high] to A[low:high] for (k=low; k <=high; k++) { A[k]=B[k]; } // enf for } // end algorithm</p><p>We are now ready to sort using Mergesort </p><p>A12: void Mergesort (int low, int high) {</p><p> if (low < high) { // if the sub-list has more than one element int mid=(low + high)/2; (1) Mergesort (low, mid); // we call Mergesort for the first half (2) Mergesort (mid+1, high); we call Mergesort for the second half // at this point the two halves are sorted (3) Merge (low, mid, high); } } // end algorithm</p><p>Example: 31 28 17 65 35 42 89 25 45 52 17 25 28 31 35 42 45 52 65 89</p><p>31 28 17 65 35 17 28 31 35 65 42 89 25 45 52 25 42 45 52 89</p><p>31 28 17 65 35 42 89 25 45 52 28 31 35 65 25 42 89 45 52 17 </p><p>31 28 17 65 35 42 89 25 45 52 28 31 17 65 35 42 89 25 45 52</p><p>42 89 31 42 89 31 28 28</p><p>Worst-case scenario</p><p>By looking at A12, we realize that the worst-case of processing n elements is equivalent to processing the two halves (lines (1) and (2)), plus to merge the two already sorted halves (of complexity n-1).</p><p>Thus</p><p>1) W(n)=W(n/2)+W(n/2)+n-1, and when n=1</p><p>W(1)=0, since if n=1, A12 returns automatically.</p><p>Problem: Solve recurrence 1) in terms of n.</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages4 Page
-
File Size-