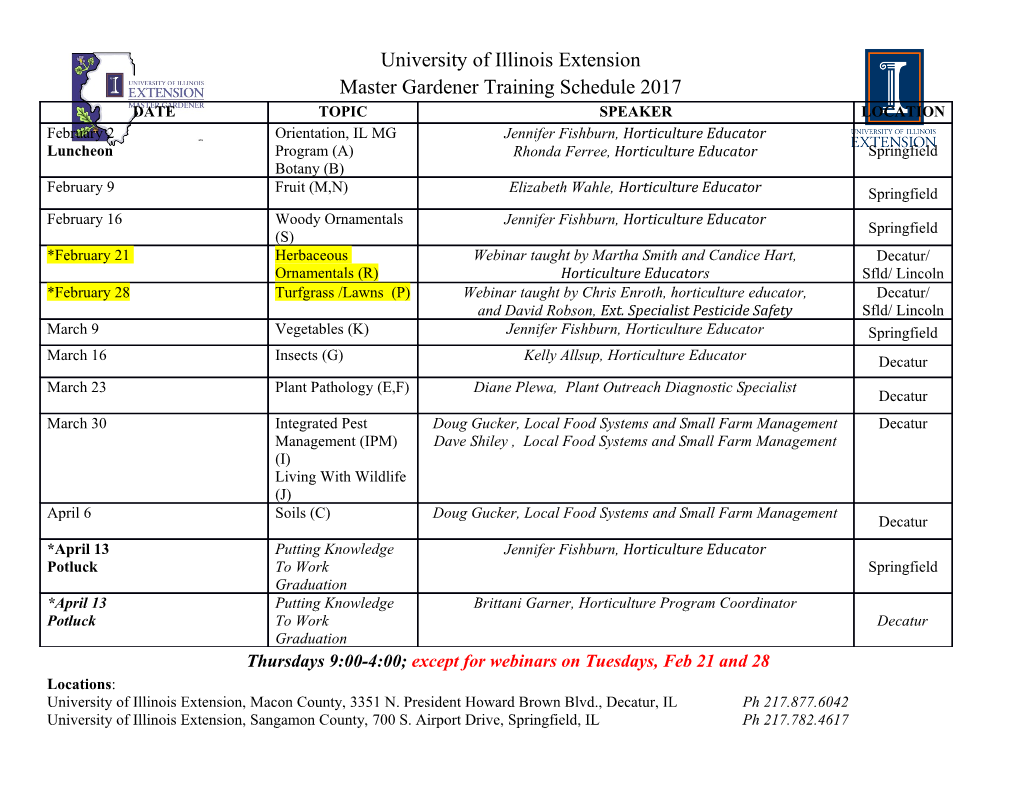
<p>第一題 /* * ======ICT_ADC.c ======* ADC for the F2812 DSK * Copyright 2008 by Jin-Yi You. * Author : Jin-Yi You(Tony Yu) [email protected] * Date : 2008-7-7 */</p><p>#include "DSP281x_Device.h" // DSP281x Headerfile Include File #include "DSP281x_Examples.h" // DSP281x Examples Include File #include "BSL_IO_F281x_V2.h" // ICT C2000 I/O Board V2.0 fonction definitions</p><p>// ADC start parameters #define ADC_MODCLK 0x3 // HSPCLK = SYSCLKOUT/2*ADC_MODCLK2 = 150/(2*3) = 25MHz #define ADC_CKPS 0x0 // ADC module clock = HSPCLK/1 = 25MHz/(1) = 25MHz #define ADC_SHCLK 0x1 // S/H width in ADC module periods = 2 ADC cycle #define BUF_SIZE 2 // Sample buffer size</p><p>// Global variable for this example Uint16 SampleTable[BUF_SIZE]; Uint16 i=0,average,x,x1=0,x2=0,x3=0,x4=0,var,re,avg; Uint16 ICT_IO_keypad(void); void Delay(Uint32); void main(void) { // Step 1. Initialize System Control: // PLL, WatchDog,peripheral Clocks to default state. // This function is found in the DSP281x_SysCtrl.c file. InitSysCtrl();</p><p>// Step 2. Clear all interrupts and Initialize PIE control registers : // Disable CPU interrupts DINT; IER = 0x0000; IFR = 0x0000;</p><p>// Step 3. Initialize PIE control and PIE vector table: // Initialize PIE control registers to their default state. // This function is found in the DSP281x_PieCtrl.c file. InitPieCtrl();</p><p>// Initialize the PIE vector table with pointers to the shell Interrupt // Service Routines (ISR). // This function is found in DSP281x_PieVect.c. InitPieVectTable();</p><p>// Step 4. Initialize all the ICT C2000 I/O Device Peripherals: ICT_IO_BOARD_Initialize(); InitAdc(); // For this example, init the ADC</p><p>// Specific clock setting for this example: EALLOW; SysCtrlRegs.HISPCP.all = ADC_MODCLK; // HSPCLK = SYSCLKOUT/ADC_MODCLK EDIS; // Specific ADC setup for this example: AdcRegs.ADCTRL1.bit.ACQ_PS = ADC_SHCLK; // S/H width AdcRegs.ADCTRL3.bit.ADCCLKPS = ADC_CKPS; // ADC module clock </p><p>AdcRegs.ADCTRL3.bit.SMODE_SEL = 0; // Enable sequential sampling modes AdcRegs.ADCTRL1.bit.SEQ_CASC = 1; // 1 Cascaded mode AdcRegs.ADCMAXCONV.all =0x0; // 1 conv’s (1 total)</p><p>AdcRegs.ADCCHSELSEQ1.bit.CONV00 = 0x0; // Setup conv from ADCINA0 AdcRegs.ADCTRL1.bit.CONT_RUN = 1; // Setup continuous run</p><p> for (i=0; i<BUF_SIZE; i++) { SampleTable[i] = 0; }</p><p>// Step 5. Enable Interrupt events: AdcRegs.ADCTRL2.all = 0x2000; // Start SEQ1 EINT; // Enable Global interrupt INTM ERTM; // Enable Global realtime interrupt DBGM</p><p>// Step 6. User specific code </p><p>// Take ADC data and log the in SampleTable array </p><p> while(1) { if(var==10) avg=(x4*10+x3+x2*10+x1); ICT_IO_LCD_Display(4,avg,0x0);</p><p> for (i=0; i<BUF_SIZE; i++) { while (AdcRegs.ADCST.bit.INT_SEQ1== 0) {} // Wait for interrupt // Software wait = (HISPCP*2) * (ADCCLKPS*2) * (CPS+1) cycles // = (3*2) * (1*2) * (0+1) = 12 cycles asm(" RPT #11 || NOP"); AdcRegs.ADCST.bit.INT_SEQ1_CLR = 1; SampleTable[i] =((AdcRegs.ADCRESULT0>>4) ); //set breakpoint average=average+SampleTable[i]; } average=(average/BUF_SIZE); //ICT_IO_LCD_Display(3,average & 0x00FF,average>>8); ICT_IO_LED_Clear(); // ICT_IO_7Seg1_Clear();</p><p> if(average>0 && average<1000) { ICT_IO_LED_On(8); x=1; } else if(average>1000 && average<2000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); x=2; } else if(average>2000 && average<3000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); x=3; } else if(average>3000 && average<4000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); ICT_IO_LED_On(5); x=4; } else if(average>4000 && average<5000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); ICT_IO_LED_On(5); ICT_IO_LED_On(4); x=5; } else if(average>5000 && average<6000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); ICT_IO_LED_On(5); ICT_IO_LED_On(4); ICT_IO_LED_On(3); x=6; } else if(average>6000 && average<7000) { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); ICT_IO_LED_On(5); ICT_IO_LED_On(4); ICT_IO_LED_On(3); ICT_IO_LED_On(2); x=7; } else { ICT_IO_LED_On(8); ICT_IO_LED_On(7); ICT_IO_LED_On(6); ICT_IO_LED_On(5); ICT_IO_LED_On(4); ICT_IO_LED_On(3); ICT_IO_LED_On(2); ICT_IO_LED_On(1); x=8; }</p><p> var=ICT_IO_keypad(); if(re!=var) { re=var; if(var!=16) { if(var==1) { x4=x3; x3=x2; x2=x1; x1=x;</p><p>} } } ICT_IO_7Seg4_On(x1); ICT_IO_7Seg3_On(x2); ICT_IO_7Seg2_On(x3); ICT_IO_7Seg1_On(x4); }</p><p>} Uint16 ICT_IO_keypad() {</p><p>ICT_IO_Keypad_Enable(); int PauseKey=16; // Output = 0001 掃描第 1 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 1; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 0 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 1;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 2 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 3 ;} // </p><p>// Output = 0010 掃描第 2 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 1; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 4 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 5;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 6 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 7 ;} // </p><p>// Output = 0100 掃描第 3 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 1; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 8 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 9;} if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 10 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 11;} // Output = 1000 掃描第 4 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 1; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 12;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 13;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 14 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 15;} </p><p>ICT_IO_Keypad_Disable(); //keypad Control Cmd return(PauseKey);</p><p>} void Delay(Uint32 k) { Uint16 index; while(k--) for( index=0;index<10000;index++) {asm(" RPT #250 ||NOP");} } //======// No more. //======第二題 /* * ======ICT_EVTimer1.c ======* EV Timer1 for the F2812 DSK * Copyright 2008 by Jin-Yi You. * Author : Jin-Yi You(Tony Yu) [email protected] * Date : 2008-7-7 */</p><p>#include "DSP281x_Device.h" // DSP281x Headerfile Include File #include "DSP281x_Examples.h" // DSP281x Examples Include File #include "BSL_IO_F281x_V2.h" // ICT C2000 I/O Board V2.0 fonction definitions</p><p>//Interrupt function prototype interrupt void eva_timer1_isr(void); Uint16 ICT_IO_keypad(void); void init_eva_timer1(void); void Delay(Uint32);</p><p>// Global counts used in this example Uint32 EvaTimer1InterruptCount,k1=0,k2=0,PauseKey=16,var,x=0,z,re; void main(void) { // Step 1. Initialize System Control: // PLL, WatchDog,peripheral Clocks to default state. // This function is found in the DSP281x_SysCtrl.c file. InitSysCtrl();</p><p>// Step 2. Clear all interrupts and Initialize PIE control registers : // Disable CPU interrupts DINT; IER = 0x0000; IFR = 0x0000;</p><p>// Step 3. Initialize PIE control and PIE vector table: // Initialize PIE control registers to their default state. // This function is found in the DSP281x_PieCtrl.c file. InitPieCtrl();</p><p>// Initialize the PIE vector table with pointers to the shell Interrupt // Service Routines (ISR). // This function is found in DSP281x_PieVect.c. InitPieVectTable();</p><p>// Interrupts that are used in this example are re-mapped to // ISR functions found within this file. EALLOW; // This is needed to write to EALLOW protected registers PieVectTable.T1PINT = &eva_timer1_isr; EDIS; // This is needed to disable write to EALLOW protected registers</p><p>// Step 4. Initialize all the ICT C2000 I/O Device Peripherals: ICT_IO_BOARD_Initialize(); init_eva_timer1();</p><p>// Step 5. Enable Interrupt events: // Enable PIE group 2 interrupt 4 for T1PINT PieCtrlRegs.PIEIER2.bit.INTx4 = 1; // Enable CPU INT2 for T1PINT IER |= M_INT2; EINT; // Enable Global interrupt INTM ERTM; // Enable Global realtime interrupt DBGM</p><p>// Step 6. User specific code while(1) { ICT_IO_7Seg4_On(k1%10); ICT_IO_7Seg3_On(k1/10); ICT_IO_7Seg2_On(k2%10); ICT_IO_7Seg1_On(k2/10); var=ICT_IO_keypad(); if(re!=var) { re=var; if(var!=16) { if(var==10) { x=0; z=0;</p><p>} }</p><p> if(var==11) { x=1; z=0; } }</p><p>} // Step 7. IDLE loop. Just sit and loop forever (optional): //for(;;); } void init_eva_timer1(void) { // Initialize EVA Timer 1: // Setup Timer 1 Registers (EV A) EvaRegs.GPTCONA.all = 0;</p><p>// Set the Period for the GP timer 1 to 0xFFFF; EvaRegs.T1PR = 0xFFFFF; // Timer 1 Period EvaRegs.T1CMPR = 0x0000; // Compare Reg</p><p>EvaRegs.EVAIMRA.bit.T1PINT = 1; // Enable Period interrupt bits for GP timer 1 */ EvaRegs.EVAIFRA.bit.T1PINT = 1; // Clear flag for Timer1</p><p>// Clear the counter for GP timer 1 EvaRegs.T1CNT = 0x0000; EvaRegs.T1CON.all = 0x1702; // Count up, x128, internal clk, enable compare, use own period</p><p>// Start timer 1 Period interrupt EvaRegs.T1CON.bit.TENABLE=1; } interrupt void eva_timer1_isr(void) { z++; if(z==9) { if(x==0) { z=0; k1++;</p><p>} if (k1==60) { k1=1; k2++; }</p><p>} // Enable more interrupts from this timer EvaRegs.EVAIMRA.bit.T1PINT = 1; EvaRegs.EVAIFRA.all = BIT7; PieCtrlRegs.PIEACK.all = PIEACK_GROUP2; }</p><p>Uint16 ICT_IO_keypad() { ICT_IO_Keypad_Enable(); PauseKey = 16; // Output = 0001 掃描第 1 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 1; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 0 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 1;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 2 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 3 ;} // </p><p>// Output = 0010 掃描第 2 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 1; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 4 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 5;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 6 ;} // if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 7 ;} // </p><p>// Output = 0100 掃描第 3 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 1; GpioDataRegs.GPBDAT.bit.GPIOB3 = 0; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 8 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 9;} if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 10 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 11;} // Output = 1000 掃描第 4 列 GpioDataRegs.GPBDAT.bit.GPIOB0 = 0; GpioDataRegs.GPBDAT.bit.GPIOB1 = 0; GpioDataRegs.GPBDAT.bit.GPIOB2 = 0; GpioDataRegs.GPBDAT.bit.GPIOB3 = 1; Delay(1); if (GpioDataRegs.GPBDAT.bit.GPIOB4 ) {PauseKey = 12;} // if (GpioDataRegs.GPBDAT.bit.GPIOB5 ) {PauseKey = 13;} // if (GpioDataRegs.GPBDAT.bit.GPIOB6 ) {PauseKey = 14 ;} if (GpioDataRegs.GPBDAT.bit.GPIOB7 ) {PauseKey = 15;} </p><p>ICT_IO_Keypad_Disable(); //keypad Control Cmd return(PauseKey);</p><p>} void Delay(Uint32 k) { Uint16 index; while(k--) for( index=0;index<10000;index++) {asm(" RPT #250 ||NOP");} }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages11 Page
-
File Size-