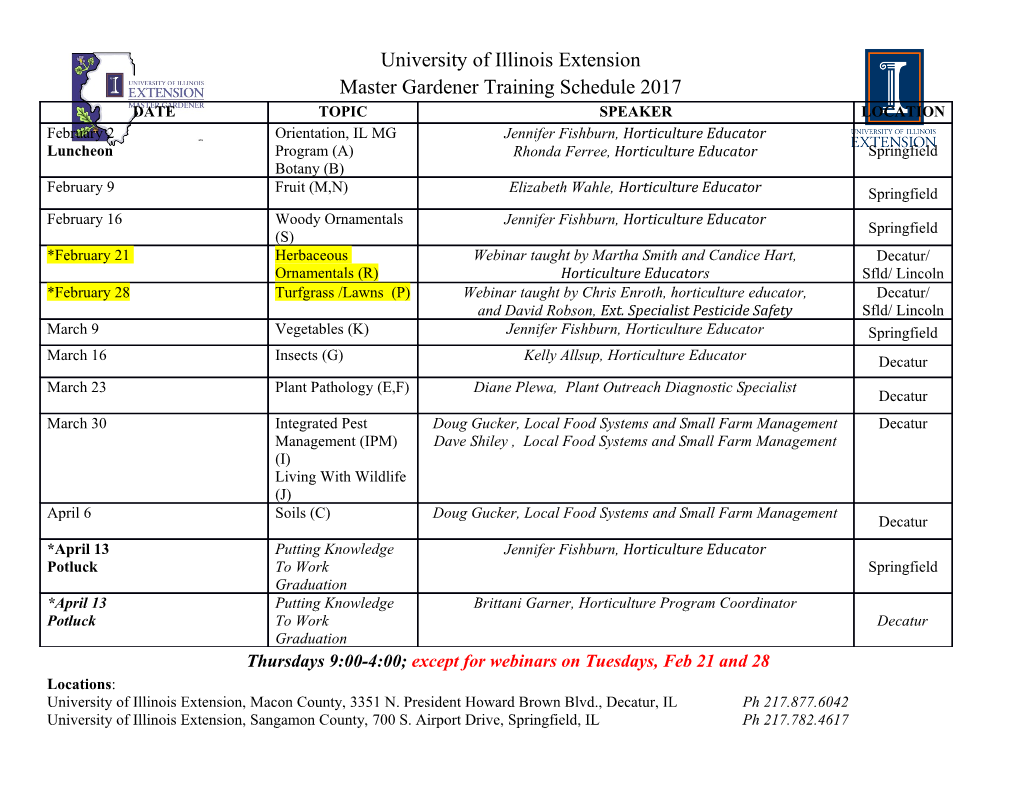
<p> Lecture10 example</p><p>1. Knowing while loop</p><p>#include <iostream> using namespace std; int main() { int i; i=0; while (i<=20) { cout<<i<<” ”; i=i+5; } cout<<endl; return(0); }</p><p>Now compare the program with the following program:</p><p>#include <iostream> using namespace std; int main() { int i; i=0; while (i<=20) { i=i+5; cout<<i<<” ”; } cout<<endl; return(0); } a) Counter-Controlled while Loops</p><p>#include <iostream> using namespace std; int main() { int i; i=0; while (i<=20) { cout<<i<<" "; i=i+5; } cout<<endl; return(0); } b) Sentinel-Controlled while Loops</p><p>#include <iostream> using namespace std; const int SENTINEL=-999; int main() { int number; cout << "If you want to terminate the program, please enter -999."<<endl; cout << "Enter a number: "; cin>>number; while (number != SENTINEL) { cout<<number<<" "<<endl; cout << "Enter a number: "; cin>>number; } cout<<endl; return(0); } c) Flag-Controlled while Loops</p><p>#include <iostream> using namespace std; int main() { bool found; int number;</p><p> found=false; while (!found) { cout << "Enter a number: "; cin>>number; cout << "Your input is " <<number<<endl; if (number > 10) found=true; } cout<<endl; return(0); } d) EOF-Controlled while Loops</p><p>#include <iostream> #include <string> using namespace std; int main() { int x; cin>>x; while(cin) { cout<<x; cin>>x; }</p><p> return 0; } e) The eof Function</p><p>#include <fstream> #include <iostream> using namespace std; int main() { ifstream infile; char ch;</p><p> infile.open("c:\\tmp\\input.dat"); infile.get(ch);</p><p> while (!infile.eof()) { cout << ch; infile.get(ch); } cout<<endl; return(0); } 2. Counter-Controlled while Loops Example // Program: AVG1 #include <iostream> using namespace std; int main() { int limit; //variable to store the number of items in the list int number; //variable to store the number int sum; //variable to store the sum int counter; //loop control variable</p><p> cout << "Line 1: Enter data for processing" << endl; //Line 1 cin >> limit; //Line 2 sum = 0; //Line 3 counter = 0; //Line 4</p><p> while (counter < limit) //Line 5 { cin >> number; //Line 6 sum = sum + number; //Line 7 counter++; //Line 8 }</p><p> cout << "Line 9: The sum of the " << limit << " numbers = " << sum << endl; //Line 9 if (counter != 0) //Line 10 cout << "Line 11: The average = " << sum / counter << endl; //Line 11 else //Line 12 cout << "Line 13: No input." << endl; //Line 13 return 0; }</p><p>3. Sentinel-Controlled while Loops Example //Program: AVG2 #include <iostream> using namespace std; const int SENTINEL = -999; int main() { int number; //variable to store the number int sum = 0; //variable to store the sum int count = 0; //variable to store the total //numbers read</p><p> cout << "Line 1: Enter numbers ending with " << SENTINEL << endl; //Line 1 cin >> number; //Line 2 while (number != SENTINEL) //Line 3 { sum = sum + number; //Line 4 count++; //Line 5 cin >> number; //Line 6 }</p><p> cout << "Line 7: The sum of the " << count << " numbers is " << sum << endl; //Line 7</p><p> if (count != 0) //Line 8 cout << "Line 9: The average is " << sum / count << endl; //Line 9 else //Line 10 cout << "Line 11: No input." << endl; //Line 11</p><p> return 0; }</p><p>//********************************************************** // Program: Telephone Digits // This is an example of a sentinel-controlled loop. This // program converts uppercase letters to their corresponding // telephone digits. //**********************************************************</p><p>#include <iostream> using namespace std; int main() { char letter; //Line 1</p><p> cout << "Program to convert uppercase letters to their " << "corresponding telephone digits." << endl; //Line 2</p><p> cout << "To stop the program enter #." << endl; //Line 3</p><p> cout << "Enter a letter: "; //Line 4 cin >> letter; //Line 5 cout << endl; //Line 6</p><p> while (letter != '#') //Line 7 { cout << "The letter you entered is: " << letter << endl; //Line 8 cout << "The corresponding telephone " << "digit is: "; //Line 9</p><p> if (letter >= 'A' && letter <= 'Z') //Line 10 switch (letter) //Line 11 { case 'A': case 'B': case 'C': cout << "2" <<endl; //Line 12 break; //Line 13 case 'D': case 'E': case 'F': cout << "3" << endl; //Line 14 break; //Line 15 case 'G': case 'H': case 'I': cout << "4" << endl; //Line 16 break; //Line 17 case 'J': case 'K': case 'L': cout << "5" << endl; //Line 18 break; //Line 19 case 'M': case 'N': case 'O': cout << "6" << endl; //Line 20 break; //Line 21 case 'P': case 'Q': case 'R': case 'S': cout << "7" << endl; //Line 22 break; //Line 23 case 'T': case 'U': case 'V': cout << "8" << endl; //Line 24 break; //Line 25 case 'W': case 'X': case 'Y': case 'Z': cout << "9" << endl; //Line 26 } else //Line 27 cout << "Invalid input." << endl; //Line 28</p><p> cout << "\nEnter another uppercase letter to find " << "its corresponding telephone digit." << endl; //Line 29 cout << "To stop the program enter #." << endl; //Line 30</p><p> cout << "Enter a letter: "; //Line 31 cin >> letter; //Line 32 cout << endl; //Line 33 }//end while</p><p> return 0; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-