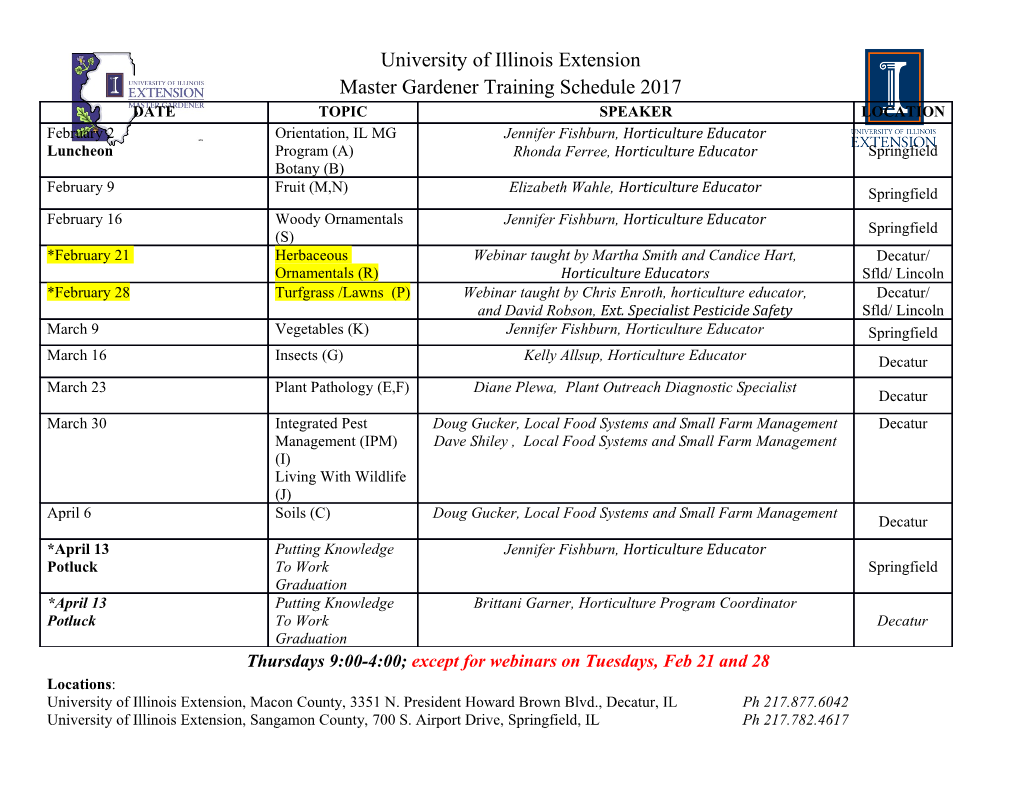
<p>Name______APCS A (Java Concepts Worksheet)</p><p>Beneath each header below, type or write a brief code snippet. Be sure to properly declare && || initialize appropriate local variables. For example; int x, y, temp, sum; double average; boolean isEven = false;</p><p>If the problem calls for an array, assume the following array instantiation; int[] myArray = new int[10]; …</p><p>How to average correctly – the simple version! Type code here.</p><p>// the key here is to cast the sum to a double.</p><p>How to average correctly in an array Type code here. Don’t forget your “for” loop!</p><p>// the key here is to cast the sum to a double to not lose decimal data</p><p>How to find max/min in an array Type code here. Don’t forget your “for” loop!</p><p>// the key here is to properly initialize max and min before entering the for loop</p><p>How to swap elements – the simple version! Begin code here.</p><p>// the key here is to perform the swap in the correct order</p><p>How to swap elements in an array – the simple version! Type code here. No loop this time – just a simple swap. </p><p>// the key here is to perform the swap in the correct order</p><p>How to reverse an array Begin code here. Remember you’re only looping through half of the array!</p><p>// the key here is to perform the swap in the correct order through just half of the array How to increase the size of an array Begin code here.</p><p>Explain the differences between selection sort and insertion sort algorithms. When would you use one over the other? Type answer here.</p><p>How to turn a number into a String int x; String y;</p><p>Type answer here…</p><p>How to prompt users for input. What lines are necessary to “scan” for input?</p><p>Type answer here…</p><p>How to compute/generate a random number.</p><p>// HINT: you will need to use the math class to do this. int num1; double num2;</p><p>// First, generate random integer from 0 to 9 Type answer here…</p><p>// Second, generate random integer from 1 to 10 Type answer here…</p><p>// Third, generate random integer from 20 to 34 Type answer here…</p><p>// Fourth, generate random decimal from 0 to 0.99 Type answer here…</p><p>// Fifth, generate random decimal from 0 to 5.99 Type answer here…</p><p>How to extract individual digits from an integer.</p><p>// HINT: the concept is called modulus and you will need to use % operator(remainder) int num = 12345; int digitNum; // will be the extracted number </p><p>// get the ones digit Type answer here…</p><p>// get the tens digit Type answer here… // get the hundreds digit Type answer here…</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages3 Page
-
File Size-