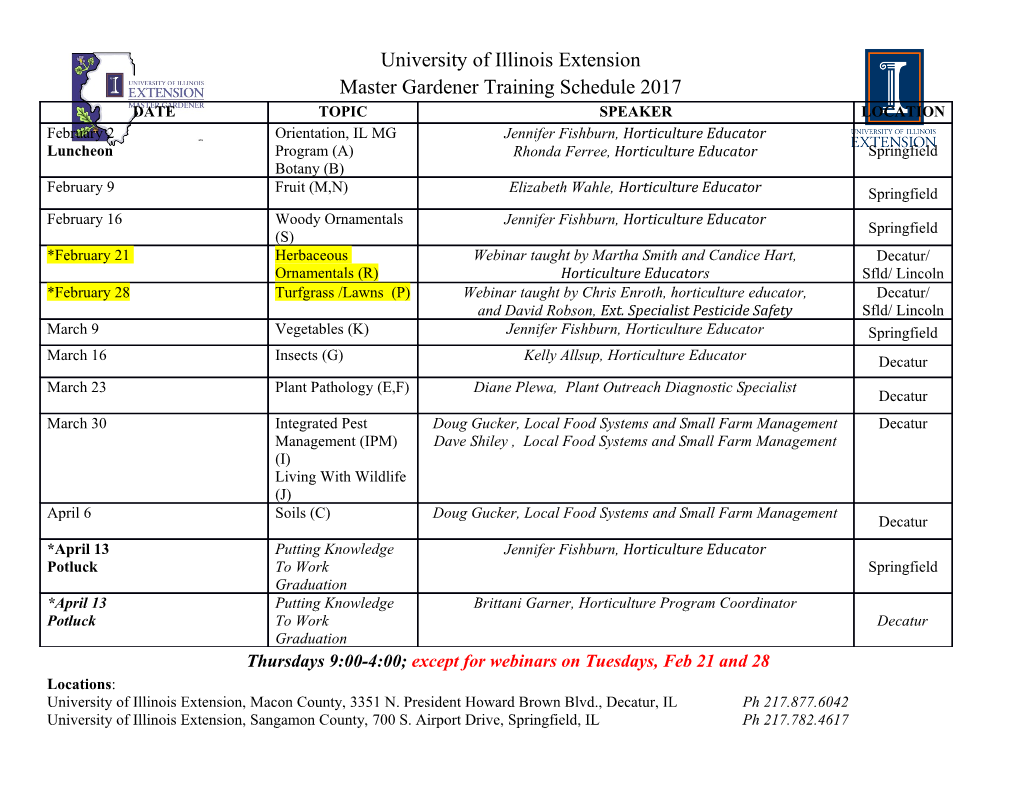
<p>Section: A Compiler's First Pass: Report Unbalanced Symbol errors. An Application of a Stack</p><p>Assuming LinkedStack<E> implements OurStack<E> finish the main method below to look for and report all unbalanced symbol errors. Only report on these opener/closer pairs: [],(), and {}. The OurStack interface is listed on the next page. It has the four methods of LinkedStack since LinkedStack<E> implements OurStack<E> 1. Construct an empty stack 2. For each character in the input file if it's an opening symbol push it onto the stack else if it is a closing symbol && the stack is empty report error otherwise pop the stack if symbol is not a closer for pop's value report error 3. At end of file, if the stack is not empty, report an error for every element still on the stack public class BalancedSymbolChecker { public static void main(String[] args) { // Use allText as your java source code. // Change it while testing. String allText = Output from the program to the left: "public class A } \n" + " public static void main(String[args)] \n" public class A } + " System.out PRINTln( Hello ); \n" public static void main(String[args)] + " for( int j = 0; j < 6 j++ ) j++ \n" System.out PRINTln( Hello ); + " doub x = 0.0; \n" for( int j = 0; j < 6 j++ ) j++ + " inTeger j = 0; \n" doub x = 0.0; + " System.out.println(Goodbye]; \n" inTeger j = 0; </p><p>+ " } \n" System.out.println(Goodbye]; } + "} \n" } + "[{( \n"; [{( </p><p>System.out.println(allText); // Apply the algorithm above to detect errors. // Print the most accurate compile time error messages as possible. // Definitely print the number of errors found. </p><p>Possible compile time errors:</p><p>Missing opener found by line 1 Mismatched closer on line 2 Mismatched closer on line 7 Missing opener found by line 8 Missing opener found by line 9 Found extra opener ( Found extra opener { Found extra opener [</p><p>Error count = 8 import java.util.EmptyStackException; public interface OurStack<E> {</p><p>/** * Check if the stack is empty to help avoid popping an empty stack. * @returns true if there are zero elements in this stack. */ public boolean isEmpty();</p><p>/** * Put element on "top" of this Stack object. * @param element The element to be added at the top of this stack. */ public void push(E element);</p><p>/** * Return reference to the element at the top of this stack. * @returns A reference to the top element. * @throws EmptyStackException if the stack is empty. */ public E peek() throws EmptyStackException;</p><p>/** * Remove element at top of stack and return a reference to it. * @returns A reference to the most recently pushed element. * @throws EmptyStackException if the stack is empty. */ public E pop() throws EmptyStackException; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages2 Page
-
File Size-