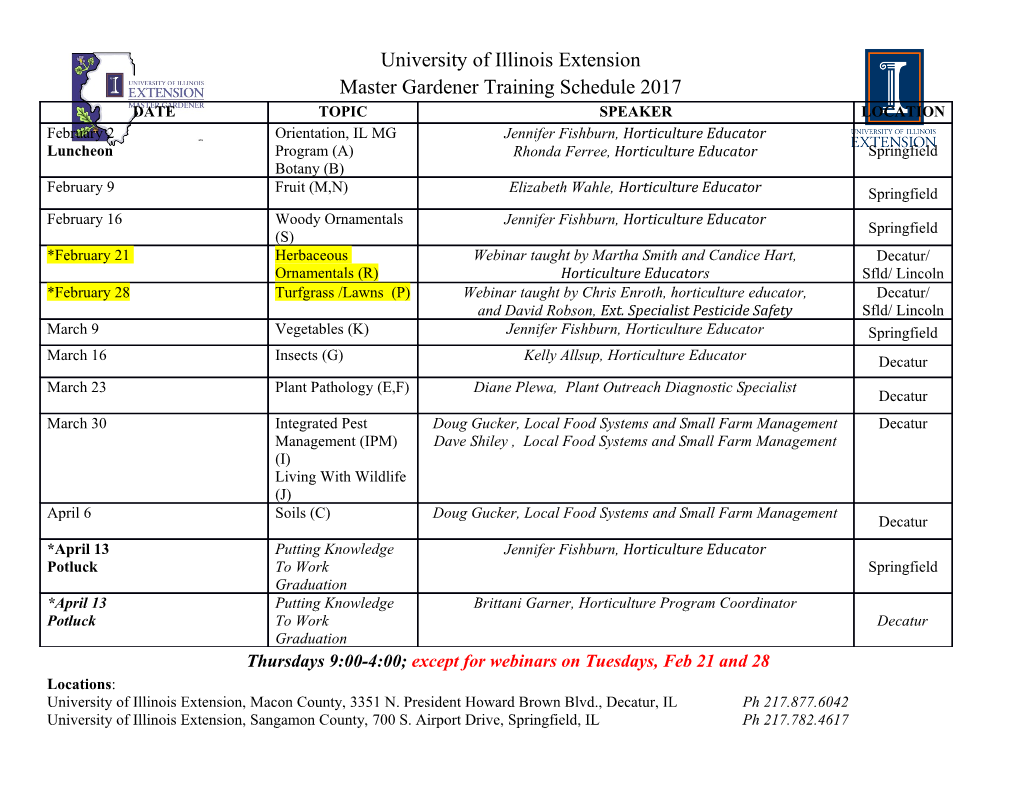
<p>#define CV_NO_BACKWARD_COMPATIBILITY</p><p>#include "cv.h" #include "highgui.h"</p><p>#include <fstream> #include <iostream> #include <cstdio> #include <cassert> #include <vector> using namespace std; using namespace cv; int RectDetect(int ROI_x, int ROI_y, int ROI_height, int ROI_width, int found_x, int found_y, int found_height, int found_width); int main( int argc, const char** argv ) {</p><p>IplImage *current_image; IplImage *draw_image; IplImage *gray_image; IplImage *small_image; const int scale = 1; const char *WINDOW_NAME = "Video Camera";</p><p>//Hard coded regions of interest (parking spaces) int dummy = 0; //currently all work on the image is scaled by 2 so ROIs are based on 1/2 the resolution of the original image int spot1 = 0; int spot1x = 140; int spot1y = 160; int spot1height = 55; int spot1width = 55;</p><p> int spot2 = 0; int spot2x = 230; int spot2y = 160; int spot2height = 55; int spot2width = 55;</p><p> int spot3 = 0; int spot3x = 320; int spot3y = 160; int spot3height = 55; int spot3width = 65;</p><p> int spot4 = 0; int spot4x = 420; int spot4y = 160; int spot4height = 55; int spot4width = 60;</p><p> int spot5 = 0; int spot5x = 100; int spot5y = 290; int spot5height = 70; int spot5width = 60;</p><p> int spot6 = 0; int spot6x = 200; int spot6y = 290; int spot6height = 70; int spot6width = 80;</p><p> int spot7 = 0; int spot7x = 320; int spot7y = 290; int spot7height = 70; int spot7width = 70;</p><p> int spot8 = 0; int spot8x = 440; int spot8y = 290; int spot8height = 70; int spot8width = 70;</p><p>//Read in a cascade xml file and allocate memory //const char *CASCADE_NAME = "C:/OpenCV2.0/data/haarcascades/haarcascade_frontalface_default.xml"; const char *CASCADE_NAME = "C:/OpenCV2.0/data/haarcascades/windshield.xml"; CvHaarClassifierCascade *cascade = (CvHaarClassifierCascade *) cvLoad(CASCADE_NAME, 0, 0, 0); CvMemStorage *storage = cvCreateMemStorage(0); assert(storage);</p><p> if(!cascade) { cout << "Fail"; system("pause"); } //video stream location CvCapture *capture = cvCreateFileCapture("http://192.168.1.2/video2.mjpg");</p><p>//Capture an image current_image = cvQueryFrame(capture);</p><p>//image setup for processing: Original -> BW original -> BW scaled draw_image = cvCreateImage(cvSize(current_image->width, current_image- >height), IPL_DEPTH_8U, 3); gray_image = cvCreateImage(cvSize(current_image->width, current_image- >height), IPL_DEPTH_8U, 1); small_image = cvCreateImage(cvSize(current_image->width / scale, current_image->height / scale), IPL_DEPTH_8U, 1); assert(current_image && gray_image && draw_image);</p><p>//Uncomment the while loop to continually grab a new image and run detection while(current_image = cvQueryFrame(capture)) { cvCvtColor(current_image, gray_image, CV_BGR2GRAY); cvResize(gray_image, small_image, CV_INTER_LINEAR);</p><p>CvSeq *cars = cvHaarDetectObjects(small_image, cascade, storage, 1.1, 2, CV_HAAR_DO_CANNY_PRUNING, cvSize(30, 30));</p><p> cvFlip(current_image, draw_image, 1); for(int i = 0; i < (cars ? cars->total : 0); i++) { cout << "cars: " << cars->total << endl;</p><p>//draw circle around detected object (set up currently for face detection)change to rect for cars CvRect *r = (CvRect *) cvGetSeqElem(cars, i);</p><p> cout << "X: " << r->x << " Y: " << r->y << " Height: " << r->height << " Width: " << r->width << endl;</p><p>//THIS IS WHERE ROI AND COMPARING GO if (spot1 == 0){ dummy = RectDetect(spot1x, spot1y, spot1height, spot1width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot1 = 1; }</p><p> if (spot2 == 0){ dummy = RectDetect(spot2x, spot2y, spot2height, spot2width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot2 = 1; }</p><p> if (spot3 == 0){ dummy = RectDetect(spot3x, spot3y, spot3height, spot3width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot3 = 1; }</p><p> if (spot4 == 0){ dummy = RectDetect(spot4x, spot4y, spot4height, spot4width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot4 = 1; }</p><p> if (spot5 == 0){ dummy = RectDetect(spot5x, spot5y, spot5height, spot5width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot5 = 1; } if (spot6 == 0){ dummy = RectDetect(spot6x, spot6y, spot6height, spot6width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot6 = 1; }</p><p> if (spot7 == 0){ dummy = RectDetect(spot7x, spot7y, spot7height, spot7width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot7 = 1; }</p><p> if (spot8 == 0){ dummy = RectDetect(spot8x, spot8y, spot8height, spot8width, r->x, r->y, r->height, r->height);</p><p> if (dummy == 1) spot8 = 1; }</p><p>CvPoint center; int radius; center.x = cvRound((small_image->width - r->width * 0.5 - r->x) * scale); center.y = cvRound((r->y + r->height * 0.5) * scale); radius = cvRound((r->width + r->height) * 0.25 * scale); cvCircle(draw_image, center, radius, CV_RGB(0, 255, 0), 3, 8, 0);</p><p>}</p><p> ofstream Parking_a; Parking_a.open("C:\\inetpub\\wwwroot\\parking_a.csv"); Parking_a <<"1," << spot1 << endl; Parking_a <<"2," << spot2 << endl; Parking_a <<"3," << spot3 << endl; Parking_a <<"4," << spot4 << endl; Parking_a <<"5," << spot5 << endl; Parking_a <<"6," << spot6 << endl; Parking_a <<"7," << spot7 << endl; Parking_a <<"8," << spot8 << endl; Parking_a.close();</p><p> spot1 = 0; spot2 = 0; spot3 = 0; spot4 = 0; spot5 = 0; spot6 = 0; spot7 = 0; spot8 = 0; //cvWaitKey is in ms and controls the speed at which the loop runs (higher number less cpu power needed) cvShowImage(WINDOW_NAME, draw_image); int key = cvWaitKey(1); if (key == 'q' || key == 'Q') break;</p><p>//While loop's close bracket }</p><p> system("pause"); return 0; } int RectDetect(int ROI_x, int ROI_y, int ROI_height, int ROI_width, int found_x, int found_y, int found_height, int found_width) { int i = 0; int j = 0; int hit = 0;</p><p>/*</p><p> int ROI_x = 10; int ROI_y = 10; int ROI_height = 10; int ROI_width = 10;</p><p>//Dynamically allocation of 2d Arrays int **ROI_Rec_x; ROI_Rec_x = new int* [ROI_width + 1]; for (i = 0; i < ROI_width + 1; i++){ ROI_Rec_x[i] = new int [ROI_height +1]; }</p><p> int **ROI_Rec_y; ROI_Rec_y = new int* [ROI_width + 1]; for (i = 0; i < ROI_width + 1; i++){ ROI_Rec_y[i] = new int [ROI_height +1]; }</p><p> int ROI_Rec_x[ROI_width + 1][ROI_height + 1]; int ROI_Rec_y[ROI_width + 1][ROI_height + 1];</p><p> int found_x = 15; int found_y = 15; int found_height = 10; int found_width = 10;</p><p> int **found_Rec_x; found_Rec_x = new int* [found_width + 1]; for (i = 0; i < found_width + 1; i++){ found_Rec_x[i] = new int [found_height +1]; }</p><p> int **found_Rec_y; found_Rec_y = new int* [found_width + 1]; for (i = 0; i < found_width + 1; i++){ found_Rec_y[i] = new int [found_height +1]; }</p><p> int found_Rec_X[found_width + 1][found_height + 1]; int found_Rec_Y[found_width + 1][found_height + 1];</p><p>// build original_Rec while (i <= ROI_width) { //cout << "i = " << i + ROI_x << endl; while (j <= ROI_height) { //cout << "j = " << j + ROI_y << endl; ROI_Rec_x[i][j] = i + ROI_x; ROI_Rec_y[i][j] = j + ROI_y; //cout << ROI_Rec_y[i][j] << endl; j++; } i++; j = 0; }</p><p> i = 0; j = 0;</p><p>// build found_Rec while (i <= found_width) { //cout << "i = " << i + ROI_x << endl; while (j <= found_height) { //cout << "j = " << j + ROI_y << endl; found_Rec_x[i][j] = i + found_x; found_Rec_y[i][j] = j + found_y; //cout << found_Rec_Y[i][j] << endl; j++; } i++; j = 0; } */ int ROI_max_x = ROI_x + ROI_width; int ROI_min_x = ROI_x; int found_max_x = found_x + found_width; int found_min_x = found_x;</p><p> int ROI_max_y = ROI_y + ROI_height; int ROI_min_y = ROI_y; int found_max_y = found_y + found_height; int found_min_y = found_y; if ((found_min_x <= ROI_max_x && found_min_x >= ROI_min_x) || (found_max_x <= ROI_max_x && found_max_x >= ROI_min_x)) { if ((found_min_y <= ROI_max_y && found_min_y >= ROI_min_y) || (found_max_y <= ROI_max_y && found_max_y >= ROI_min_y)) { hit = 1; } }</p><p> if ((ROI_min_x <= found_max_x && ROI_min_x >= found_min_x) || (ROI_max_x <= found_max_x && ROI_max_x >= found_min_x)) { if ((ROI_min_y <= found_max_y && ROI_min_y >= found_min_y) || (ROI_max_y <= found_max_y && ROI_max_y >= found_min_y)) { hit = 1; } } /* //Deallocation for( int i = ROI_width - 1; i >= 0; i-- ) delete [ ] ROI_Rec_x[ i ]; delete [ ] ROI_Rec_x;</p><p> for( int i = ROI_width - 1; i >= 0; i-- ) delete [ ] ROI_Rec_y[ i ]; delete [ ] ROI_Rec_y;</p><p> for( int i = found_width - 1; i >= 0; i-- ) delete [ ] found_Rec_x[ i ]; delete [ ] found_Rec_x;</p><p> for( int i = found_width - 1; i >= 0; i-- ) delete [ ] found_Rec_y[ i ]; delete [ ] found_Rec_y; */ if (hit == 1) { cout << "Hit" << endl; return 1; }</p><p> cout << "Miss" << endl;</p><p> return 0; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-