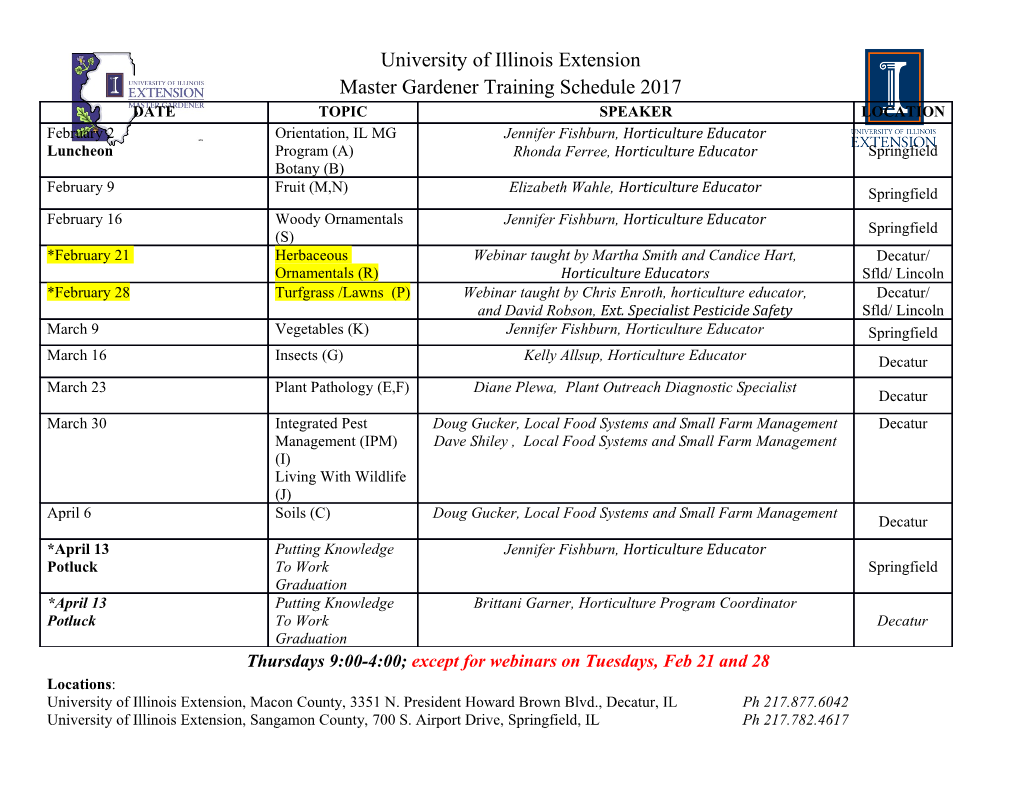
<p>Basic Workflows</p><p>Setting up a basic workflow</p><p>1) Open Infrastructure Administrator</p><p>2) Open a project. This script assumes you are working with a Water model</p><p>3) Click on the Workflow folder in the Industry Model Explorer pane</p><p>4) Click the Create button and give your workflow a name (something that is descriptive of what you will be doing)</p><p>5) Click Create. Your workflow will be added to the Workflow list</p><p>6) Select your workflow. The Script Code text box will automatically be filed with the empty definition of a VB.NET function</p><p>7) Add code to the function between Sub Run and End Sub. Use the following code to create some basic workflows. </p><p>Basic Message Box</p><p>Me.Application.MsgBox("Seemples") Basic Message Box and input box</p><p>Dim name As String</p><p> name = InputBox("Input Something:", "Anything")</p><p>If (not string.IsNullOrEmpty(name)) Then</p><p>Me.Application.MsgBox(name)</p><p>End If</p><p>Select Features</p><p> me.Document.Map.SelectFeatures("WA_VALVE")</p><p>Select and list</p><p>Dim features as FeatureList me.Document.Map.SelectFeatures("WA_VALVE") features = Me.Document.Map.GetFeatures()</p><p>Me.Application.MessageBox(features.Item(0).Attributes.Item("FID").Value)</p><p>Digitize</p><p>Dim dig as FeatureList</p><p>Dim fc as FeatureClass fc = Me.Document.Connection.FeatureClasses.Item("WA_LINE") dig = me.Document.Map.DigitizeFeatures(fc, fc.Type ,"", "",true)</p><p>Digitize and show dialog</p><p>Dim dig as FeatureList Dim fc as FeatureClass fc = Me.Document.Connection.FeatureClasses.Item("WA_LINE") dig = me.Document.Map.DigitizeFeatures(fc, fc.Type ,"", "",true)</p><p>Me.Document.OpenDialog(dig) Challenge:</p><p>Write a short script that:</p><p> Digitizes a Pipe</p><p> Shows the new FID of the pipe in a Message box</p><p>Creating a Plugin Workflow</p><p>1) In Visual Studio create new class library: call it MyWorkflow and save.</p><p>2) On the menu bar go to Project> MyWorkflow Properties</p><p>3) Set the ouput path for the compiled dll to C:\Program Files\Autodesk\AutoCAD Map 3D 2012\bin\ (or 2013)</p><p>4) Click the Reference Tab. Set reference folder to C:\Program Files\Autodesk\AutoCAD Map 3D 2012\bin and click Add</p><p>5) In Solution Explorer set up the references: Right Click on References and Add:</p><p>System.Drawing</p><p>System.Windows.Forms Topobase.Data</p><p>Topobase.Forms</p><p>Topobase.Graphic</p><p>Topobase.Map</p><p>Topobase.Workflow</p><p>6) Rename the .cs file to MyPlugin.cs and double click to open</p><p>7) Add the following to create the basic class container code</p><p> using System; using System.Collections; using Topobase.Workflows; using Topobase.Workflows.Forms; using Topobase.Data;</p><p> namespace MyWorkflow { public class MyPlugin : WorkflowPlugIn</p><p>{ public void MyWorkflow1() { this.WorkflowSupport.WorkflowExplorerFlyIn.ShowMessage("Please Digitize a point"); } } }</p><p>8) In Solution explorer right click on the MyWorkflow node and select ADD>User Control. In the Add New Item Dialog, select User Control</p><p>Name the User Control MyUserControl and click Add</p><p>9) Open MyUserControl and using the Toolbox in Visual studio add the following objects to the User Control Panel:</p><p> a. Text: set the text to Coordinates in the Properties dialog b. Textbox: select and check that Modifiers in the properties dialog is set to Public c. Button: set the caption to OK 10) View the code for the User Control. Much of the code has been generated. Add the code snippets in red:</p><p> using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Data; using System.Linq; using System.Text; using System.Windows.Forms;</p><p> using Topobase.Workflows;</p><p> namespace MyWorkflow { public partial class MyUserControl : UserControl { private Label label1; private TextBox textBox1; private Button button1;</p><p> private WorkflowSupport wfs;</p><p> public MyUserControl(WorkflowSupport wfs) { InitializeComponent(); this.wfs = wfs; }</p><p> public MyUserControl() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) {</p><p> this.wfs.WorkflowExplorerFlyIn.HideUserControls(); }</p><p>11) Go back to the MyPlugin.cs script. Add the code in red this.WorkflowSupport.WorkflowExplorerFlyIn.ShowMessage("Please Digitize a point");</p><p>Topobase.Graphic.Point point = this.WorkflowSupport.Document.Map.GetPoint("Digitize Point");</p><p>MyUserControl form = new MyUserControl(this.WorkflowSupport); form.textBox1.Text = point.X.ToString("F2") + "/" + point.Y.ToString("F2"); this.WorkflowSupport.WorkflowExplorerFlyIn.ShowControl(form);</p><p>12) Create and .tbp file</p><p>If you want to create your own plugin, you have to create a .dll with one or more plugin classes in it and a .tbp file that tells Autodesk Topobase which plugin classes are implemented and where to find them.</p><p>To create a .tbp file, create a new text file and name in MyWorkflow.tbp. Add the following text:</p><p><?xml version="1.0" encoding="utf-8"?> <PlugIn></p><p><Default AssemblyName="MyWorkflow.dll" Namespace="MyWorkflow" DocumentKey="" MapName="" Priority="100" ExecutionTargetWeb="True" ExecutionTargetDesktop="True" Company="Autodesk, Inc." Author="Topobase Engineering" /> </PlugIn> 13) Add the .tbp file to you project:</p><p>In Visual Studio go to the Solution Explorer panel and right click on MyWorkflow>ADD>Existing Item.</p><p>Navigate to your MyWorkflow.tbp file (make sure the file filter is set to All Files) and click Add</p><p>Select MyWorkflow.tbp in Solution Explorer and view its properties.</p><p>Set Copy To Output Directory as Copy Always</p><p>14) Compile the project.</p><p>In Solution Explorer right click on either Solution or MyWorkflow and select Buid.</p><p>15) Open Administrator and add the new workflow and code:</p><p>Call the new workflow something relevant to the task and add the following code</p><p>Me.RunMethod("MyWorkflow.dll","MyWorkflow.MyPlugin", "MyWorkflow1")</p><p>16) In Map3D, open TB_WA.dwg and run your new workflow</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-