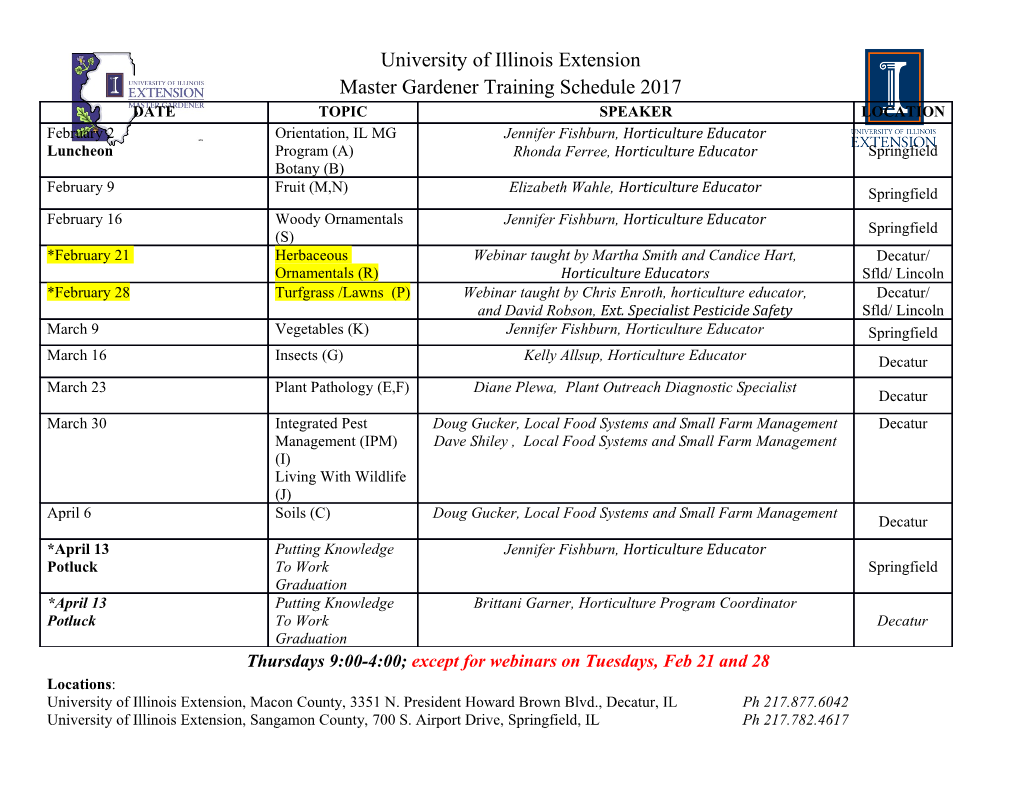
<p>Section Linked Structures</p><p>Use this singly-linked structure to answer questions 1-4</p><p> front </p><p>"A" "B" "C" </p><p>1. What is the value of front.data? ______2. What is the value of front.next.next.data? ______3. What is the value of front.next.data? ___ 4. What is the value of front.next.next.next? ______5. Write the code that will generate the following linked structure.</p><p> front back </p><p>"1st" "2nd" </p><p>6. Draw a picture of the linked structure built from this code: Node n1 = new Node("aaa"); n1.next = new Node("bbb"); n1.next.next = new Node("ccc"); n1.next.next.data = "fff";</p><p>7. Using the code in question 6, What happens with this code n1.next.next.next.data = "ggg";</p><p>8. Complete methods toString addLast as if it was in the LinkedList class began in lecture so it adds a new element at the end of a linked structure referred to by front. The following code should generate this picture of memory. LinkedList<String> list = new LinkedList<String>(); list.addLast("First"); list.addLast("Second"); </p><p> list.addLast("Third"); front public class LinkList<E> { "First" "Second" "Third" private class Node { private E data; private Node next;</p><p> public Node(E objectReference) { data = objectReference; }</p><p> public Node(E objectReference, Node nextReference) { data = objectReference; next = nextReference; } }</p><p> private Node front; private int n;</p><p> public LinkList() { front = null; n = 0; }</p><p> public void addFirst(E element) { front = new Node(element, front); n++; }</p><p> public void addLast(String element) {</p><p>ANSWERS</p><p>1. What is the value of front.data? __"A"____ 2. What is the value of front.next.next.data? __"C"____ 3. What is the value of front.next.data? _"B"__ </p><p>4. What is the value of front.next.next.next? _null_____ 5. Write the code that will generate the following linked structure.</p><p>Node front = new Node("1st"); Node back = new Node("2nd"); front.next = back;</p><p>6. Draw a picture of the linked structure built from this code: Node n1 = new Node("aaa"); n1.next = new Node("bbb"); n1.next.next = new Node("ccc"); n1.next.next.data = "fff";</p><p> n1 </p><p>"aaa" "bbb" "fff" </p><p>7. Null pointer exception</p><p>8. public void addLast(E element) { if (isEmpty()) this.addFirst(element); else { Node ref = front; while (ref.next != null) ref = ref.next; ref.next = new Node(element); } size++; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages2 Page
-
File Size-