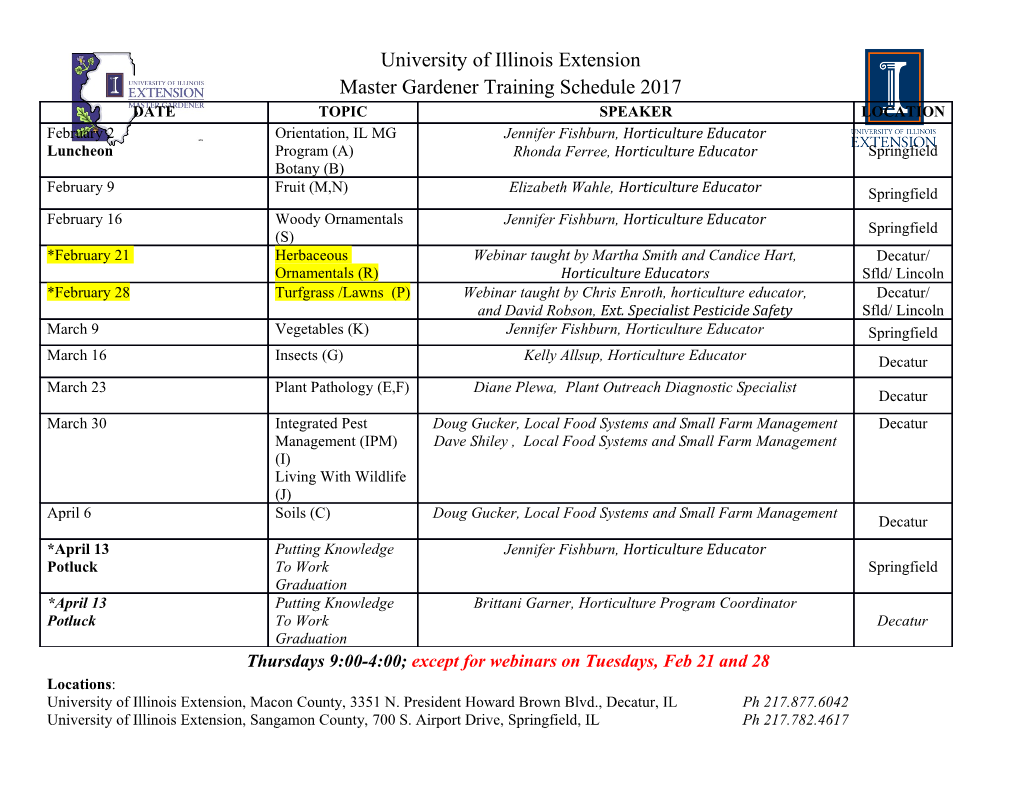
<p> fk1.c // c program main()</p><p>{ int PID, pid; pid = getpid(); printf("\n The current process ID is %d\n", pid); PID = fork(); if (PID==0) { printf("\n The child process prints this message from here...... \n"); printf("\nPID value in the child process = %d\n", PID); pid = getpid(); printf("\n The child process id is %d\n", pid); printf("\n The child process now terminates"); } else { printf("\n The parent process prints out this message from here...... \n"); printf("\nPID value in the parent process = %d\n", PID); pid = getpid(); printf("\n The parent process id is %d\n", pid); printf("\n The parent process now terminates"); } } fk2.c //c program</p><p>#include <stdio.h> int PID, pid; char buf[2]; void fk(); main() { fk(); } void fk() {</p><p> pid = getpid(); printf("\n The current process ID is %d\n", pid); PID = fork(); if (PID==0) { printf("\n The child process prints this message from here...... \n"); printf("Enter a value:\n"); scanf("%s", buf); printf("The child got the input buf...%s\n ", buf); printf("\nPID value in the child process = %d\n", PID); pid = getpid(); printf("\n The child process id is %d\n", pid); printf("\n The child process now terminates"); } else { printf("\n The parent process prints out this message from here...... \n"); /* scanf("%s", buf); printf("The parent got the input buf...%s\n", buf); */ printf("\nPID value in the parent process = %d\n", PID); pid = getpid(); printf("\n The parent process id is %d\n", pid); printf("\n The parent process now terminates"); } }</p><p> fk3.c // c program</p><p>#include <stdio.h> int PID, pid, status; char buf[2]; void fk(); main() { fk(); } void fk() {</p><p> pid = getpid(); printf("\n The current process ID is %d\n", pid); PID = fork(); if (PID==0) { printf("\n The child process prints this message from here...... \n"); printf("Enter a value:\n"); scanf("%s", buf); printf("The child got the input buf...%s\n ", buf); printf("\nPID value in the child process = %d\n", PID); pid = getpid(); printf("\n The child process id is %d\n", pid); printf("\n The child process now terminates"); } else { printf("\n The parent process prints out this message from here...... \n"); pid = wait(&status); printf("\n The parent process waits until the child process terminates...", pid); printf("\nPID value in the parent process = %d\n", PID); pid = getpid(); printf("\n The parent process id is %d\n", pid); printf("\n The parent process now terminates"); } }</p><p> fk4.c //c program</p><p>#include <stdio.h> int PID, pid; char buf[2]; void fk(); main() { fk(); } void fk() {</p><p> pid = getpid(); printf("\n The current process ID is %d\n", pid); PID = fork(); if (PID==0) { printf("\n The child process prints this message from here...... \n"); printf("Enter a value:\n"); scanf("%s", buf); printf("The child got the input buf...%s\n ", buf); printf("\nPID value in the child process = %d\n", PID); pid = getpid(); printf("\n The child process id is %d\n", pid); printf("\n The child process now terminates"); } else { printf("\n The parent process prints out this message from here...... \n"); scanf("%s", buf); printf("The parent got the input buf...%s\n", buf); printf("\nPID value in the parent process = %d\n", PID); pid = getpid(); printf("\n The parent process id is %d\n", pid); printf("\n The parent process now terminates"); } }</p><p> pro3-10.c</p><p>#include <sys/types.h> #include <stdio.h> #include <unistd.h> int main() { pid_t pid; //just int type</p><p>/* fork a child process */ pid = fork();</p><p> if (pid < 0) {/* error occurred */ fprintf(stderr, "Fork failed"); exit(-1); } else if (pid == 0) {/* code section for child process */ execlp("/bin/ls", "ls", NULL); } else {/* parent code section */ /* parent will wait until the child to complete */ wait(NULL); printf("child complete "); exit(0); } }// of main</p><p>Win32App.c</p><p>#include "stdafx.h" #include <windows.h> #include <stdio.h> int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { * This program creates a separate process using the CreateProcess() system call. * */ STARTUPINFO si; PROCESS_INFORMATION pi;</p><p>ZeroMemory( &si, sizeof(si) ); si.cb = sizeof(si); ZeroMemory( &pi, sizeof(pi) );</p><p>// Start the child process. if( !CreateProcess( NULL, // No module name (use command line). "C:\\WINDOWS\\system32\\mspaint.exe", // Command line. NULL, // Process handle not inheritable. NULL, // Thread handle not inheritable. FALSE, // Set handle inheritance to FALSE. 0, // No creation flags. NULL, // Use parent's environment block. NULL, // Use parent's starting directory. &si, // Pointer to STARTUPINFO structure. &pi ) // Pointer to PROCESS_INFORMATION structure. ) { printf( "CreateProcess failed (%d).\n", GetLastError() ); return -1; }</p><p>// Wait until child process exits. WaitForSingleObject( pi.hProcess, INFINITE );</p><p>// Close process and thread handles. CloseHandle( pi.hProcess ); CloseHandle( pi.hThread ); return 0; } shm-posix.c #include <stdio.h> #include <sys/shm.h> #include <sys/stat.h> int main() { /* the identifier for the shared memory segment */ int segment_id; /* a pointer to the shared memory segment */ char* shared_memory; /* the size (in bytes) of the shared memory segment */ const int segment_size = 4096;</p><p>/** allocate a shared memory segment */ segment_id = shmget(IPC_PRIVATE, segment_size, S_IRUSR | S_IWUSR);</p><p>/** attach the shared memory segment */ shared_memory = (char *) shmat(segment_id, NULL, 0); printf("shared memory segment %d attached at address %p\n", segment_id, shared_memory);</p><p>/** write a message to the shared memory segment */ sprintf(shared_memory, "Hi there!");</p><p>/** now print out the string from shared memory */ printf("*%s*\n", shared_memory); /** now detach the shared memory segment */ if ( shmdt(shared_memory) == -1) { fprintf(stderr, "Unable to detach\n"); }</p><p>/** now remove the shared memory segment */ shmctl(segment_id, IPC_RMID, NULL); </p><p> return 0; }</p><p> do_functions.c</p><p>#include <stdio.h> void do_one_thing(int *pnum_times) { int i, j, x;</p><p> for (i=0; i<4; i++) { printf("doing one thing\n"); for (j=0; j <10000; j++) { (*pnum_times)++; x = x + 1; }</p><p>} } void do_another_thing(int *pnum_times) { int i, j, x;</p><p> for (i=0; i<4; i++) { printf("doing another thing\n"); for (j=0; j <10000; j++) { (*pnum_times)++; x = x + 1; } } } void do_wrap_up(int one_times, int another_times) { int total;</p><p> total = one_times + another_times; printf("wrap up: one thing %d, another %d, total %d\n", one_times, another_times, total); }</p><p> simple.c</p><p>#include <stdlib.h> void do_one_thing(int *); void do_another_thing(int *); void do_wrap_up(int, int); int r1 = 0, r2 = 0; main() { do_one_thing(&r1); do_another_thing(&r2); do_wrap_up(r1, r2); }</p><p> con_simple.c</p><p>#include <sys/types.h> #include <sys/ipc.h> #include <sys/shm.h> #include <stdlib.h> #include <stdio.h></p><p>#include <sys/wait.h> void do_one_thing(int *); void do_another_thing(int *); void do_wrap_up(int, int); int shared_mem_id; int *shared_mem_ptr; int *r1p; int *r2p;</p><p> main() { pid_t child1_pid, child2_pid; int status;</p><p>/* initialize shared memory segment */</p><p> if(( shared_mem_id = shmget(IPC_PRIVATE, 2*sizeof(int), IPC_CREAT|0666)) <0) perror("shmget failed"); /* if (( shared_mem_ptr = (int *)shmat(shared_mem_id, (void *)0, 0)) == (int *) -1) perror("shmat failed"); */</p><p> if (( shared_mem_ptr = (int *)shmat(shared_mem_id, NULL, 0)) == (int *) -1) perror("shmat failed"); </p><p> r1p = shared_mem_ptr; r2p = shared_mem_ptr + 1;</p><p>*r1p = (int) 0; *r2p = (int) 0;</p><p> if ((child1_pid = fork()) == 0) { /* first child does this.... */ do_one_thing(r1p); exit(status); } /* Now parent does this checkings.... */ if ((child2_pid = fork()) == 0) { /* second child does this.... */ do_another_thing(r2p); exit(status); } </p><p>/* Parent */ waitpid(child1_pid, &status, 0);</p><p> if(WIFEXITED(status)) printf("Child exited with code %d\n", WEXITSTATUS(status)); else printf("Child terminated abnormally\n");</p><p> waitpid(child2_pid, (int *) status, 0); do_wrap_up(*r1p, *r2p); }</p><p> simple_thread.c</p><p>#include <pthread.h></p><p> void do_one_thing(int *); void do_another_thing(int *); void do_wrap_up(int, int); int r1 = 0, r2 = 0;</p><p> main() {</p><p> pthread_t thread1, thread2;</p><p> pthread_create(&thread1, NULL, (void *) do_one_thing, (void *) &r1); pthread_create(&thread2, NULL, (void *) do_another_thing, (void *) &r2);</p><p> pthread_join(thread1, NULL); pthread_join(thread2, NULL);</p><p> do_wrap_up(r1, r2);</p><p>} makefile</p><p>CC = gcc CFLAGS = -g THREAD_CFLAGS = ${CFLAGS} -pthread</p><p> all : simple con_simple simple_thread do_functions.o : do_functions.c $(CC) $(CFLAGS) -c do_functions.c simple : simple.o do_functions.o $(CC) $(CFLAGS) do_functions.o simple.o -o simple con_simple : con_simple.o do_functions.o $(CC) $(CFLAGS) do_functions.o con_simple.o -o con_simple simple_thread : simple_thread.o do_functions.o $(CC) $(THREAD_CFLAGS) do_functions.o simple_thread.o -o simple_thread clean : rm -f *.o *~ *# core a.out\ simple con_simple simple_thread</p><p>Another thread example sumThread.c</p><p>/** * A pthread program illustrating how to * create a simple thread and some of the pthread API * This program implements the summation function where * the summation operation is run as a separate thread. * * Most Unix/Linux/OS X users * gcc thrd.c -lpthread * * Solaris users must enter * gcc thrd.c -lpthreads * */</p><p>#include <pthread.h> #include <stdio.h> int sum; /* this data is shared by the thread(s) */ void *runner(void *param); /* the thread */ int main(int argc, char *argv[]) { pthread_t tid; /* the thread identifier */ pthread_attr_t attr; /* set of attributes for the thread */ if (argc != 2) { fprintf(stderr,"usage: a.out <integer value>\n"); /*exit(1);*/ return -1; } if (atoi(argv[1]) < 0) { fprintf(stderr,"Argument %d must be non-negative\n",atoi(argv[1])); /*exit(1);*/ return -1; }</p><p>/* get the default attributes */ pthread_attr_init(&attr);</p><p>/* create the thread */ pthread_create(&tid,&attr,runner,argv[1]);</p><p>/* now wait for the thread to exit */ pthread_join(tid,NULL); printf("sum = %d\n",sum); }</p><p>/** * The thread will begin control in this function */ void *runner(void *param) { int i, upper = atoi(param); sum = 0;</p><p> if (upper > 0) { for (i = 1; i <= upper; i++) sum += i; }</p><p> pthread_exit(0); }</p><p> mserver.c</p><p>#include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h> #include <string.h> #include <sys/errno.h> #include <stdio.h> #include <stdlib.h></p><p>#define MSGKEY 75 #define ACK "msgserv received the following message: " struct msgform { long mtype; char mtext[2048]; } sndbuf, rcvbuf, *msgp; int msgid; main() { extern int errno; int i, rtrn; msgid = msgget(MSGKEY, 0777|IPC_CREAT);</p><p> for(;;) { msgp = &rcvbuf; msgrcv(msgid, msgp, 2048, 1, 0); printf("\n%s\n", rcvbuf.mtext);</p><p> msgp = &sndbuf; strcpy(sndbuf.mtext, ACK); strcat(sndbuf.mtext, rcvbuf.mtext); msgp->mtype = 10; rtrn=msgsnd(msgid, msgp, sizeof(sndbuf.mtext), 0); if (rtrn == -1) { printf("\n msgsnd() system call failed, Error # = %d\n", errno); exit(0); } } }</p><p> mclient.c</p><p>#include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h> #include <string.h> #include <stdio.h> #include <errno.h> #include <stdio.h> #include <stdlib.h></p><p>#define MSGKEY 75</p><p> struct msgform { long mtype; char mtext[2048]; } sndbuf, rcvbuf, *msgp; main() { extern int errno; int i, c, msgid; int rtrn, msgsz;</p><p> msgid = msgget(MSGKEY, 0777); msgp = &sndbuf; msgp->mtype = 1; printf("\n Enter your message to be delivered to the server:\n"); for (i = 0; ((c=getchar())!=EOF); i++) sndbuf.mtext[i] = c; msgsz = i + 1;</p><p> rtrn = msgsnd(msgid, msgp, msgsz, 0); if (rtrn == -1) { printf("\n msgsnd() system call failed, error # = %d\n", errno); exit(0); }</p><p> msgp = &rcvbuf; msgrcv(msgid, msgp, 2048, 10, 0); printf("\n%s\n", rcvbuf.mtext); } DateClient.java import java.net.*; import java.io.*; public class DateClient { public static void main(String[] args) { try { // this could be changed to an IP name or address other than the localhost Socket sock = new Socket("127.0.0.1",6013); InputStream in = sock.getInputStream(); BufferedReader bin = new BufferedReader(new InputStreamReader(in));</p><p>String line; while( (line = bin.readLine()) != null) System.out.println(line);</p><p> sock.close(); } catch (IOException ioe) { System.err.println(ioe); } } }</p><p>DateServer.java import java.net.*; import java.io.*; public class DateServer { public static void main(String[] args) { try { ServerSocket sock = new ServerSocket(6013);</p><p>// now listen for connections while (true) { Socket client = sock.accept(); // we have a connection</p><p>PrintWriter pout = new PrintWriter(client.getOutputStream(), true); // write the Date to the socket pout.println(new java.util.Date().toString());</p><p>// close the socket and resume listening for more connections client.close(); } } catch (IOException ioe) { System.err.println(ioe); } } }</p><p>Implementing a simple shell by exec()</p><p>#include <stdio.h> #include <string.h> #define MAXLINE 80 #define WHITE " " #define MAXARG 20 int main() { char cmd[MAXLINE]; void background(char *cmd);</p><p> for(;;) { printf("mysh ready$$"); /* prompting mysh reddy $$ */ gets(cmd); /* read a command */ if (strcmp(cmd, "exit") == 0) return(0); background(cmd); /* start a background job */ }</p><p>} void background(char *cmd) { char *argv[MAXARG]; int pid, i = 0; /* to fill in argv */ argv[i++] = strtok(cmd, WHITE); while (i < MAXARG && (argv[i++] = strtok(NULL, WHITE)) != NULL); if ( (pid = fork()) == 0) /* The child process executes background job */ { execv(argv[0], argv); // execl("/bin/ls", "ls", "-a", "-l", 0); exit(1); /* execv failed */ } else if (pid < 0) {</p><p> fprintf(stderr, "fork failed \n"); perror("background"); }</p><p>}</p><p> pexec.c</p><p>#include <unistd.h> #include <stdio.h> #include <stdlib.h> char *const ps_argv[] = {"ps", "-ax", 0};</p><p>//Not useful char *const ps_envp[] = {"PATH=/bin/ls:/usr/bin", "TERM=console", 0}; int main() { printf("Replace this process with new program image by exec family \n");</p><p>// execl("/bin/ps", "ps", "-ax", 0); //assume ps is in bin directory // execlp("ps", "ps", "-ax", 0); //assume /bin is in PATH execle("/bin/ls", "-Fs", 0, ps_envp); //passes own environment</p><p>// execv("/bin/ps", ps_argv); // execvp("ps", ps_argv); // execve("/bin/ps", ps_argv, ps_envp);</p><p> printf("Done.\n"); exit(0); }</p><p> ptrace.c</p><p>#include <stdio.h> #include <sys/ptrace.h> #include <sys/types.h> #include <sys/wait.h> #include <unistd.h> #include <sys/reg.h> int main() { pid_t child; long orig_eax; child = fork(); if(child == 0) { ptrace(PTRACE_TRACEME, 0, NULL, NULL); execl("/bin/ls", "ls", NULL); } else { wait(NULL); orig_eax = ptrace(PTRACE_PEEKUSER, child, 4 * ORIG_EAX, NULL); printf("The child made a " "system call %ld\n", orig_eax); ptrace(PTRACE_CONT, child, NULL, NULL); } return 0; }</p><p> server2.c //UNIX INET domain socket server</p><p>/* Make the necessary includes and set up the variables. */</p><p>#include <sys/types.h> #include <sys/socket.h> #include <stdio.h> #include <netinet/in.h> #include <arpa/inet.h> #include <unistd.h> int main() { int server_sockfd, client_sockfd; int server_len, client_len; struct sockaddr_in server_address; struct sockaddr_in client_address;</p><p>/* Create an unnamed socket for the server. */</p><p> server_sockfd = socket(AF_INET, SOCK_STREAM, 0); /* Name the socket. */</p><p> server_address.sin_family = AF_INET; server_address.sin_addr.s_addr = inet_addr("127.0.0.1"); server_address.sin_port = 9734; server_len = sizeof(server_address); bind(server_sockfd, (struct sockaddr *)&server_address, server_len);</p><p>/* Create a connection queue and wait for clients. */</p><p> listen(server_sockfd, 5); while(1) { char ch;</p><p> printf("server waiting\n");</p><p>/* Accept a connection. */</p><p> client_len = sizeof(client_address); client_sockfd = accept(server_sockfd, (struct sockaddr *)&client_address, &client_len);</p><p>/* We can now read/write to client on client_sockfd. */</p><p> read(client_sockfd, &ch, 1); ch++; write(client_sockfd, &ch, 1); close(client_sockfd); } } client2.c //domain socket</p><p>/* Make the necessary includes and set up the variables. */</p><p>#include <sys/types.h> #include <sys/socket.h> #include <stdio.h> #include <netinet/in.h> #include <arpa/inet.h> #include <unistd.h> int main() { int sockfd; int len; struct sockaddr_in address; int result; char ch = 'A';</p><p>/* Create a socket for the client. */</p><p> sockfd = socket(AF_INET, SOCK_STREAM, 0);</p><p>/* Name the socket, as agreed with the server. */</p><p> address.sin_family = AF_INET; address.sin_addr.s_addr = inet_addr("127.0.0.1"); address.sin_port = 9734; len = sizeof(address);</p><p>/* Now connect our socket to the server's socket. */</p><p> result = connect(sockfd, (struct sockaddr *)&address, len);</p><p> if(result == -1) { perror("oops: client2"); exit(1); }</p><p>/* We can now read/write via sockfd. */</p><p> write(sockfd, &ch, 1); read(sockfd, &ch, 1); printf("char from server = %c\n", ch); close(sockfd); exit(0); }</p><p>UNIX domain sockets sc.h #include <sys/types.h> #include <stdio.h> #include <stdlib.h> #include <sys/socket.h> #include <sys/un.h></p><p>#define SERVER "/tmp/server" unixDomainSocketServer.c</p><p>#include <signal.h> #include "sc.h" void stop () { unlink (SERVER) ; exit (0) ; } void server (void) { int sock_fd, cli_sock_fd ; struct sockaddr_un unix_addr ; char buf[2048] ; int n, addr_len ; pid_t pid ; char *pc ;</p><p> signal (SIGINT, stop) ; signal (SIGQUIT, stop) ; signal (SIGTERM, stop) ;</p><p> if ((sock_fd = socket (AF_UNIX, SOCK_STREAM, 0)) < 0) { perror ("srv: socket ()") ; exit (1) ; }</p><p> unix_addr.sun_family = AF_UNIX ; strcpy (unix_addr.sun_path, SERVER) ; addr_len = sizeof (unix_addr.sun_family) + strlen (unix_addr.sun_path) ; unlink (SERVER) ;</p><p> if (bind (sock_fd, (struct sockaddr *) &unix_addr, addr_len) < 0) { perror ("srv: bind ()") ; exit (1) ; }</p><p> if (listen (sock_fd, 5) < 0) { perror ("srv: client ()") ; exit (1) ; } while ((cli_sock_fd = accept (sock_fd, (struct sockaddr *) &unix_addr , &addr_len)) >= 0) { if ((n = read (cli_sock_fd, buf, 2047)) < 0) { perror ("srv: read ()") ; close (cli_sock_fd) ; continue ; }</p><p> buf[n] = '\0' ; for (pc = buf ; *pc != '\0' && (*pc < '0' || *pc > '9') ; pc ++) ;</p><p> pid = atoi (pc) ; if (pid != 0) { sprintf (buf, "Hello Client %d, this is the Server.\n", pid) ; n = strlen (buf) + 1 ;</p><p> if (write (cli_sock_fd, buf, n) != n) perror ("srv: write ()") ; }</p><p> close (cli_sock_fd) ; }</p><p> perror ("srv: accept ()") ; unlink (SERVER) ; exit (1) ; } int main (void) { int r ; if ((r = fork ()) == 0) { server () ; }</p><p> if (r < 0) { perror ("srv: fork ()") ; exit (1) ; }</p><p> exit (0) ; } unixDomainCLient.c</p><p>#include "sc.h" int main (void) { int sock_fd ; struct sockaddr_un unix_addr ; char buf[2048] ; int n ;</p><p> if ((sock_fd = socket (AF_UNIX, SOCK_STREAM, 0)) < 0) { perror ("cli: socket ()") ; exit (1) ; }</p><p> unix_addr.sun_family = AF_UNIX ; strcpy (unix_addr.sun_path, SERVER) ;</p><p> if (connect (sock_fd, (struct sockaddr *) &unix_addr , sizeof (unix_addr.sun_family) + strlen (unix_addr.sun_path)) < 0) { perror ("cli: connect ()") ; exit (1) ; }</p><p> sprintf (buf, "Hello Server, this is %d.\n", getpid ()) ; n = strlen (buf) + 1 ; if (write (sock_fd, buf, n) != n) { perror ("cli: write ()") ; exit (1) ; }</p><p> if ((n = read (sock_fd, buf, 2047)) < 0) { perror ("cli: read ()") ; exit (1) ; }</p><p> buf[n] = '\0' ; printf ("Client received: %s\n", buf) ;</p><p> exit (0) ; } variableArguments.c</p><p>#include <stdlib.h> #include <stdarg.h> #include <stdio.h> int maxof(int, ...) ; void f(void); main(){ f(); exit(EXIT_SUCCESS); }</p><p> int maxof(int n_args, ...){ register int i; int max, a; va_list ap;</p><p> va_start(ap, n_args); max = va_arg(ap, int); for(i = 2; i <= n_args; i++) { if((a = va_arg(ap, int)) > max) max = a; }</p><p> va_end(ap); return max; } void f(void) { int i = 5; int j[256]; j[42] = 24; printf("%d\n",maxof(3, i, j[42], 0));</p><p>}</p><p>Windows Sockets</p><p>You need to include ws32_32.lib at the end of link property of the Project settings</p><p>WinSockStreamServer.c</p><p>#include <stdio.h> #include <winsock2.h> int main() { WSADATA wsaData; SOCKET sockSvr; SOCKET sockSS; int nlen; struct sockaddr_in addrSockSvr; struct sockaddr_in addrSockclt;</p><p>// Winsock initialization WSAStartup(MAKEWORD(2, 0), &wsaData);</p><p>// Winsock object creation sockSvr = socket(AF_INET, SOCK_STREAM, 0);</p><p>// Winsock server configuration addrSockSvr.sin_family = AF_INET; addrSockSvr.sin_port = htons(6060); addrSockSvr.sin_addr.S_un.S_addr = INADDR_ANY; bind(sockSvr, (struct sockaddr *)&addrSockSvr, sizeof(addrSockSvr));</p><p>// Listening at the port listen(sockSvr, 5);</p><p> while(1) { // Receiving client request nlen = sizeof(addrSockclt); sockSS = accept(sockSvr, (struct sockaddr *)&addrSockclt, &nlen);</p><p>// Sending message to the client printf("Connection from %s (port no. : %d)\n", inet_ntoa(addrSockclt.sin_addr), //IP address ntohs(addrSockclt.sin_port)); // Port Number send(sockSS, "Hello TCP/IP world", 18, 0);</p><p>// TCP session end closesocket(sockSS); }</p><p>// Winsock object cleanup WSACleanup();</p><p> getchar();</p><p> return 0; }</p><p>WinSockStreamCLient.c</p><p>#include <stdio.h> #include <winsock2.h> int main() {</p><p>WSADATA wsaData; SOCKET sockClt; char szMsg[32]; struct sockaddr_in addrSockSvr; int nLet;</p><p>//Winsock Initialization WSAStartup(MAKEWORD(2, 0), &wsaData);</p><p>// Create Winsock Object sockClt = socket(AF_INET, SOCK_STREAM, 0); // Server Configuration for the Winsock object addrSockSvr.sin_family = AF_INET; addrSockSvr.sin_port = htons(6060); addrSockSvr.sin_addr.S_un.S_addr = inet_addr("127.0.0.1");</p><p>// Connect to the server connect(sockClt, (struct sockaddr *)&addrSockSvr, sizeof(addrSockSvr));</p><p>// receive data from socket memset(szMsg, 0, sizeof(szMsg)); nLet = recv(sockClt, szMsg, sizeof(szMsg), 0);</p><p> printf("%s\n", szMsg);</p><p>// Cleanup Winsock object WSACleanup();</p><p> getchar(); return 0; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages29 Page
-
File Size-