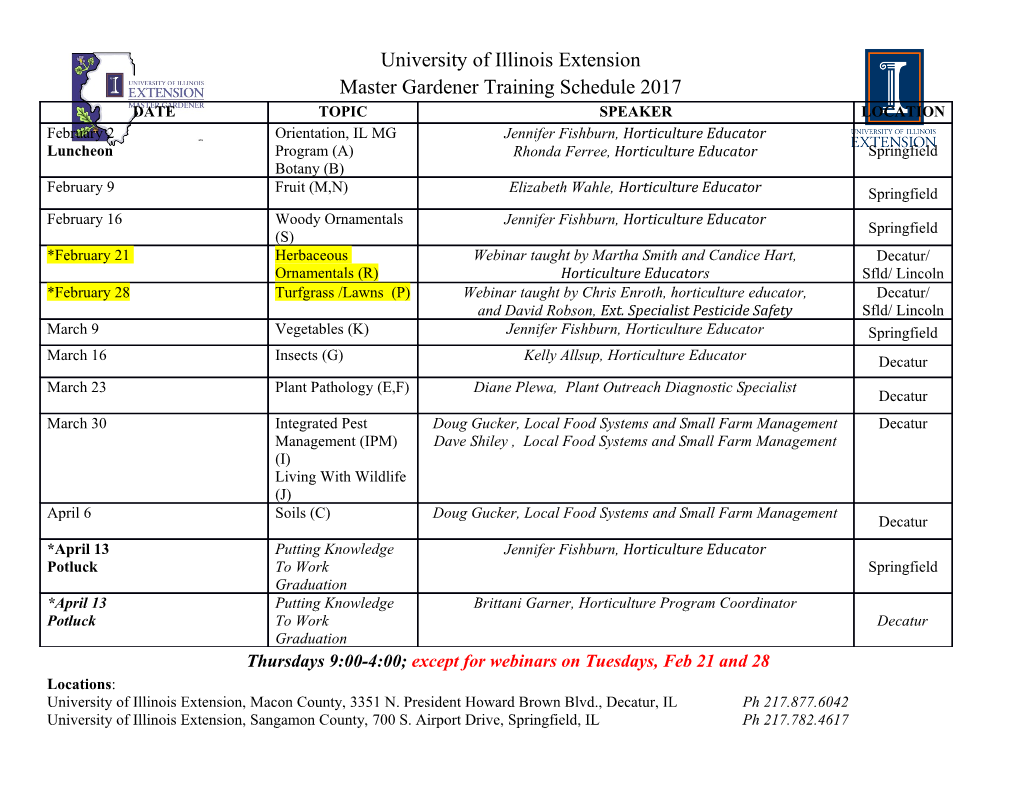
<p> Comp 110 Algorithms & Programming Take Home Midterm Examination #2</p><p>7 五月 2018</p><p>Name:______Answers______</p><p>C. R. Putnam 7 May 2018 1. Write a method that finds the average of all elements in a matrix, i.e., a 2-dimensional array, of integers. The method should accept matrices of all sizes, e.g., the following examples:</p><p>4x4 matrix 4x3 matrix 3x4 matrix</p><p>11 29 13 94 65 78 23 43 48 16 86 76 17 18 29 21 34 87 61 39 63 27 10 11 52 13 23 45 72 28 37 99 35 14 25 16 37 90 87 31</p><p> int avg_matix( int [ ][ ] m, int k; int l) { int sum = 0; for( i = 0; i <= k; i++ ) for( j = 0; j <= l; j++ ) sum += m[ i ][ j ]; return sum; }</p><p>2 2. Write a method that multiplies two matrices. The header of the method is public static int [ ] [ ] multiplyMatrix( int [ ] [ ] a, int [ ] [ ] b) </p><p>In order to multiply matrix a by matrix b, the number of columns in a must be the same as the number of rows in b and the two matrices must have elements of compatible types. Let matrix c be the result of multiplying matrix a by matrix b, i.e, c = a * b; e.g., </p><p> a11 a12 a13 b11 b12 b13 c11 c12 c13 a21 a22 a23 ● b21 b22 b23 c21 c22 c23 b31 b32 b33</p><p> where cij = ai1 * b1j + ai2 * b2j + ai3 * b3j </p><p>As a concrete example</p><p>1 2 3 7 8 9 1*7 + 2*1 + 3*6 1*8 + 2*4 + 3*8 1*9 + 2*3 + 3*4 27 40 27 4 5 6 ● 1 4 3 4*7 + 5*1 + 6*6 4*8 + 5*4 + 6*8 4*9 + 5*3 + 6*4 69 100 75 6 8 4</p><p>In order to construct the required method, with the knowledge covered in the lectures, the header must be expanded to include the dimensions of the matrices. </p><p> public static int [ ] [ ] multiplyMatrix( int [ ] [ ] a, int m, int n, int [ ] [ ] b, int p, int r) { if (n != p) System.out.println(“Matrices are not compatible n, i.e., “+ n +“ != p, i.e., “+p); int c[ m ][ r ]; for( i = 0; i <= m; i++ ) for ( j = 0; j <= n; j++ ) for ( k = 0; k <= r; k++ ) c[ i ][ k ] = a[ i ][ j ] * b[ j ][ k ];</p><p> return c; }</p><p>3 3. Design and implement the Fan class as specified in Liang age 256 problem # 7.2. Submit the UML diagram, the source code for the Fan class, the test program, and the output for the two Fan objects.</p><p>Fan</p><p>+ SLOW = 1 : constant int + MEDIUM = 2 : constant int + FAST = 3 : constant int</p><p> speed = SLOW : int on = FALSE : boolean radius = 5 : double color = “blue” : String</p><p>+ Fan ( ) + get_speed ( ) : int + set_speed (speed : int) : void + get_on ( ) : boolean + set_on (on : boolean) : void + get_radius ( ) : double + set_radius (radius : double) : void + get_color ( ) : String + set_color (color : String) : void + toString( ) : String</p><p> public class Fan { final int SLOW = 1; final int MEDIUM = 2; final int FAST = 3;</p><p> int speed = SLOW; boolean on = FALSE; double radius = 5; String color = “blue”;</p><p>Fan( ) { }</p><p> int get_speed ( ) { return speed; }</p><p>4 void set_speed ( int speed ) { this.speed = speed; }</p><p> boolean get_on ( ) { return on; }</p><p> void set_on (boolean on) { this.on = on; }</p><p> double get_radius ( ) { return radius; }</p><p> void set_radius (double radius) { this.radius = radius; }</p><p>String get_color ( ) { return color; }</p><p> void set_color (String color) { this.color = color; }</p><p>String toString( ) { if (on == TRUE ) return “speed = ”+ speed +“ color = ”+ color + “ radius = ” + radius; else return “color = ”+ color + “ radius = ” + radius + “ FAN IS OFF”; } }</p><p>5 public static void main(String[ ] args) { Fan f1 = new fan( ); Fan f2 = new fan( );</p><p> f1.set_speed(FAST); f1.set_radius(10); f1.set_color(“yellow”); f1.set_on(TRUE);</p><p> f2.set_speed(MEDIUM); f2.set_radius(5); f2.set_color(“blue”); f2.set_on(FALSE);</p><p>System.out.println(f1.toString); System.out.println(f2.toString); } </p><p>6</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages6 Page
-
File Size-