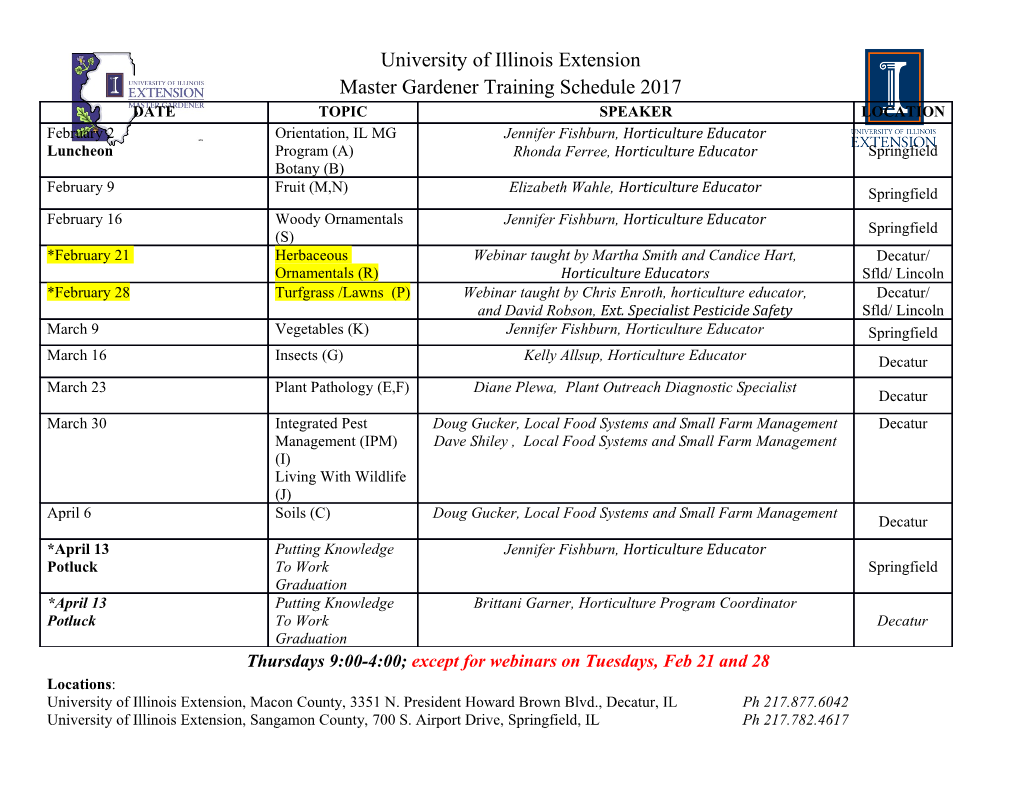
<p> IPC144 - Files Agenda:</p><p>1 Review/Questions 2 More scanf 3 Files 4 Try it! 5 Homework</p><p>Review/Questions</p><p>More scanf() (see example: fun36.c )</p><p>%[] - an additional format specifier available for scanf is %[] where the square brackets contain a list of valid characters. "-" character inside the square [] brackets indicates a range. "^" character inside the square [] brackets indicates that all characters should be accepted except those inside the square brackets. %30s number specifies maximum number of characters to be read. </p><p>Files</p><p>File is a named area of the disk storage. C provides a set of predefined functions to open, read, write, and close files. The standard distinguishes between text files and binary files. Different functions are available for accessing text and binary files.</p><p>When a file is open – it is loaded into the memory. We need to declare a pointer that will be used to access the file. </p><p>Syntax: </p><p>FILE *fp; /* defines a file pointer fp */ fopen and fclose functions fopen() function is used to open a file. fopen() function expects two arguments: the name of the file, as a string, and the mode, also given as a character string. Returns a pointer to a file or NULL if error occurred and file could not be open.</p><p>Mode If the file exists If the file does not exist “r” Opens the file for reading Error “w” Opens a new file for writing Creates a new file “a” Opens a file for appending(writing at the end of Creates a new file the file) “r+” Opens the file for reading and writing Error “w+” Opens a new file for reading and writing Creates a new file “a+” Opens the file for reading and writing at the end Creates a new file of the file.</p><p>Syntax: FILE *fp; /* define a file pointer fp */ char file_name[] = “AnyName.txt”; /* character array to store a filename */ fp = fopen(file_name, “w”); /* open file for writing, if the file exists the old content is discarded */</p><p>A binary files may be opened by adding a ‘b’ to the mode string. E.g. fopen(“file.dat”, “wb”); /* will open binary file file.dat for writing. */ </p><p>When a file is opened, a file position marker is set to some location in the file. It identifies the next place in the file to read or write data. When a file is open in mode “r”, “w”, “r+”, or “w+”, the file position marker is set to the beginning of the file. When a file is opened in mode “a” or “a+”, the file position marker is set to the end of the file. After each input/output operation, the file position marker is automatically repositioned just after the data that were read or written. fclose() function is used to close the file. fclose() expects one argument, a pointer to FILE. It will return 0 (zero) if the file is successfully closed, and EOF, if for some reason file can not be closed. fprintf and fscanf functions fprintf() is used to write to a file. It works the same as printf(), but with one extra argument, the pointer to a file, which was opened for "w" or "a" access.</p><p>Example:</p><p>/* fun43.c – simple example of writing to a file ( no error checking ) */ #include <stdio.h> main(){ FILE *fp; /* declare a pointer to a FILE */ char lname[] = “Smith”; char fname[] = “John”; double salary = 2338.00; char position[] = “Manager”;</p><p> fp = fopen(“records.dat”, “w”); /* open file records.dat */</p><p> fprintf(fp, "%s,%s:%.2lf,%s\n", lname, fname, salary, position);</p><p>/* write a record to a file */</p><p> fclose(fp);</p><p>/* close the file */ } fscanf() is used to read from a file. Since fscanf() reads from a file, it requires a pointer to a file as one of it’s arguments.</p><p>Example: /* fun44.c – example of reading from a file ( no error checking) */ #include <stdio.h> main(){ FILE *fp; /* declare a pointer to a FILE */ char lname[31]; char fname[31]; double salary; char position[31];</p><p> fp = fopen(“records.dat”, “r”); /* open file records.dat */</p><p> fscanf(fp, "%[^,],%[^:]:%lf,%[^\n]\n", lname, fname, &salary, position);</p><p>/* read a record from a file */</p><p> printf(“Last Name: %s\nFirst Name: %s\nSalary: %.2lf\nPosition: %s\n”, lname, fname, salary, position);</p><p>/* display record */</p><p> fclose(fp);</p><p>/* close the file */ } Example: /* fun45.c – example of writing to a file providing error checking */</p><p>#include <stdio.h> main(){</p><p>FILE *fp; /* declare a pointer to a FILE */ char lname[31]; char fname[31]; double salary; char position[31]; int i;</p><p> fp = fopen(“records.dat”, “w”); /* open file records.dat */ if(fp){ /* same as if( fp != NULL ) */ for(i = 0; i < 3; i++){ printf(“Last Name: “); gets(lname); printf(“First Name:”); gets(fname); printf(“Salary:”); scanf(“%lf”, &salary); printf(“Position:”); gets(position); fprintf(fp, "%s,%s:%.2lf,%s\n", lname, fname, salary, position); } printf(“%d records written to the file\n”, i); fclose(fp); } else printf(“Cannot open records.dat file\n”); } /* fun46.c - example of reading from a file */ #include <stdio.h> main(){ FILE *fp; /* declare a pointer to a FILE */ char lname[31]; char fname[31]; double salary; char position[31]; int i;</p><p> fp = fopen("records.dat", "r"); /* open file records.dat */ if(fp){ /* if fp is not NULL */ printf("%-13s%-13s%10s%10s\n", "Last Name", "First Name", "Salary", "Position"); for(i = 0; i < 3; i++){ fscanf(fp, "%[^,],%[^:]:%lf,%[^\n]\n", lname, fname, &salary, position); /* read a record from a file */</p><p> printf("%-13s%-13s%10.2lf%10s\n", lname, fname, salary, position);</p><p>/* display record */ } fclose(fp); /* close the file */ }else printf("Can not open file!\n"); } Built in Function rewind()</p><p>Function rewind() is used to move the file position indicator to the beginning of the file</p><p>Syntax:</p><p>#include <stdio.h> void rewind( FILE *stream );</p><p>Example (see walk19.c)</p><p>Read the content of the file</p><p>When working with files remember that you’ll need to read the content of the file into some variables as you can’t directly manipulate data in the file. </p><p>Example 1 (fun50.c) – read one character at a time from the file, until the last character is reached (EOF)</p><p> while ( fscanf(pin, "%c", &ch) != EOF )</p><p>Example 2 (fun49.c) – read one word at a time from the file, until the last character is reached (EOF)</p><p> while ( fscanf(pin, "%s", word) != EOF )</p><p>Example 3 (fun46.c) – read using format specifiers from the files formatted with delimiters.</p><p> fscanf(fp, "%[^,],%[^:]:%lf,%[^\n]\n", lname, fname, &salary, position);</p><p>Example 4 (fun53.c) – write to a file using fputs()</p><p> fputs(name,fp);</p><p>Example 5 (fun55.c) – read from file using getc()</p><p> while((ch = getc(pin)) != EOF)</p><p>Example 6 (fun67.c) – read from a file a set number of characters using fgets(). Read file up to 79 characters at a time will stop if 79 characters read or at \n or at EOF</p><p> while(fgets(line,80,fp)!= NULL) Read Data from File into Arrays The following example ( fun66.c ) demonstrates how to read a few records from a text file into arrays. #include <stdio.h> main(){ FILE *pin; char description[100][50]; char sale[100]; int quantity[100]; double price[100]; int i = 0, num_records = 0;</p><p> pin = fopen("tempfile.txt","r"); if(pin == NULL){ printf("Error: file can't be open..\n"); } else { /* read from the file until EOF reached */ while(fscanf(pin, "%[^,],%c,%d,%lf\n", description[i], &sale[i], &quantity[i], &price[i]) != EOF){ i++; } fclose(pin);</p><p> printf("%-15s %12s %10s %15s\n","DESCRIPTION", "SALE (Y/N)", "QUANTITY", "UNIT PRICE");</p><p>/* display the content of the arrays */ num_records = i; for(i = 0; i < num_records; i++){ printf("%-15s %12c %10d %15.2lf\n",description[i], sale[i], quantity[i], price[i]); } } } Format of the file is DESCRIPTION,SALE(Y/N),QUANTITY,UNIT PRICE The content of tempfile.txt file:</p><p>PENCIL,Y,7,7.50 PAPER,N,200,3.99 INK,N,50,2.99 STAPLES,N,15,0.75</p><p>Try it! Modify fun66.c as follows: 1. Display a listing of all items on sale Homework #13 (email your solution: [email protected])</p><p>Write a program that will allow the user to add a record to a text file emp.dat. Each record consists of the employee first and last name, employee number (1000 – 6000), and department number (1 – 10).</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-