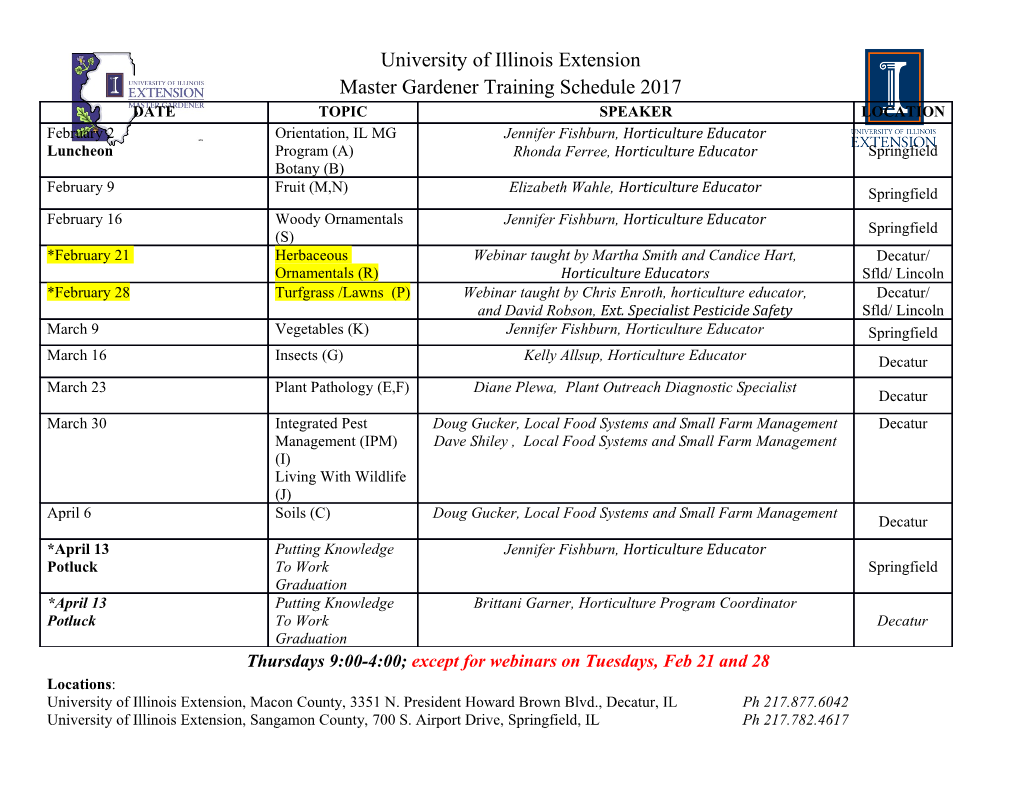
<p>// Codes for Car Inventory Control Application (ITERATOR Pattern)</p><p>//PRORAM.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class Program { static void Main(string[] args) { Cars car; NissanDealershipInventory NissanCars = new NissanDealershipInventory(); ToyotaDealershipInventory ToyotaCars = new ToyotaDealershipInventory(); BmwDealershipInventory BmwCars = new BmwDealershipInventory();</p><p> car = new Cars("Toyota","Corolla"," New red corolla with sports package ", 19999); ToyotaCars.addItems(car); car = new Cars("Toyota", "Camry", " New silver camry with the basic package", 25999); ToyotaCars.addItems(car); car = new Cars("Toyota", "Avalon", " Used black Avalon with leather seats ", 15999); ToyotaCars.addItems(car); car = new Cars("Nissan", "Sentra", " Used black Sentra with basic package", 10999); NissanCars.addItems(car); car = new Cars("Nissan", "Maxima", " New gray Maxima with all the upgrades", 31999); NissanCars.addItems(car); car = new Cars("Nissan", "GT-R", " New blue GT-R with a v8 turbo engine", 35999); NissanCars.addItems(car); car = new Cars("Nissan", "Murano", " New silver Murano great family car", 31999); NissanCars.addItems(car); car = new Cars("BMW", "325I", "New charcoal 2010 bmw325i 5speed manual ", 38999); BmwCars.addItems(car); car = new Cars("BMW", "745I", "New metallic blue 2010 bmw745i luxury pckg ", 80999); BmwCars.addItems(car); car = new Cars("BMW", "X6", "New black 2010 bmwX6 premium package ", 60999); BmwCars.addItems(car); car = new Cars("BMW", "M5", "New Red 2010 bmw M5 6speed manual ", 58999); BmwCars.addItems(car);</p><p>Iterator NissanIterator = NissanCars.CreateIterator(); Iterator ToyotaIterator = ToyotaCars.CreateIterator(); Iterator BmwIterator = BmwCars.CreateIterator(); Console.WriteLine("Nissan Inventory"); Console.WriteLine("Name Model Description Price"); Cars Ncar = NissanIterator.First();</p><p> while (Ncar != null) { Console.WriteLine("{0} {1} {2} {3}", Ncar.getBrand,Ncar.getModel,Ncar.getDescription,Ncar.getPrice); Ncar = NissanIterator.Next(); } Cars Tcar = ToyotaIterator.First(); Console.WriteLine("\nToyota Inventory"); Console.WriteLine("Name Model Description Price");</p><p> while (Tcar != null) { Console.WriteLine("{0} {1} {2} {3}", Tcar.getBrand, Tcar.getModel, Tcar.getDescription, Tcar.getPrice); Tcar = ToyotaIterator.Next(); } Cars Bcar = BmwIterator.First(); Console.WriteLine("\nBMW Inventory"); Console.WriteLine("Name Model Description Price"); while (Bcar != null) { Console.WriteLine("{0} {1} {2} {3}", Bcar.getBrand, Bcar.getModel, Bcar.getDescription, Bcar.getPrice); Bcar = BmwIterator.Next(); }</p><p>Console.ReadKey(); } } } // ITERATOR.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { abstract class Iterator { public abstract Cars First(); public abstract Cars Next(); public abstract bool IsDone(); public abstract Cars CurrentItem();</p><p>} }</p><p>// NISSANDEALERSHIPITERATOR.CS using System; using System.Collections.Generic; using System.Collections; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class NissanDealershipIterator : Iterator { private ArrayList NissanCarsArray = new ArrayList(); private int position = 0; public NissanDealershipIterator(ArrayList NissanCarsArray) { this.NissanCarsArray = NissanCarsArray; } public override Cars First() { return (Cars)NissanCarsArray[0]; }</p><p> public override Cars Next() { Cars ret = null;</p><p> if (position < NissanCarsArray.Count - 1) { ret = (Cars)NissanCarsArray[++position]; }</p><p> return ret; }</p><p> public override bool IsDone() { return (position >= NissanCarsArray.Count); }</p><p> public override Cars CurrentItem() { return (Cars)NissanCarsArray[position]; } } }</p><p>//TOYOTACARDEALERSHIPITERATOR.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class ToyotaDealershipIterator : Iterator { private List<Cars> ToyotaCarsList; private int position = 0; public ToyotaDealershipIterator(List<Cars> ToyotaCarsList) { this.ToyotaCarsList = ToyotaCarsList; }</p><p> public override Cars First() { return (Cars)ToyotaCarsList[0]; }</p><p> public override Cars Next() { Cars ret = null; if (position < ToyotaCarsList.Count - 1) { ret = (Cars)ToyotaCarsList[++position]; }</p><p> return ret; }</p><p> public override bool IsDone() { return position >= ToyotaCarsList.Count; }</p><p> public override Cars CurrentItem() { return (Cars)ToyotaCarsList[position]; } } }</p><p>//BMWCARDEALERSHIPITERATOR using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class BmwDealershipIterator : Iterator { private List<Cars> BmwCarsList; private int position = 0; public BmwDealershipIterator(List<Cars> BmwCarsList) { this.BmwCarsList = BmwCarsList; }</p><p> public override Cars First() { return (Cars)BmwCarsList[0]; }</p><p> public override Cars Next() { Cars ret = null; if (position < BmwCarsList.Count - 1) { ret = (Cars)BmwCarsList[++position]; }</p><p> return ret; }</p><p> public override bool IsDone() { return position >= BmwCarsList.Count; }</p><p> public override Cars CurrentItem() { return (Cars)BmwCarsList[position]; } } }</p><p>// CARABSTRACTINVENTORY.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { abstract class CarAbstractInventory { public abstract Iterator CreateIterator(); } }</p><p>//NISSANDEALERSINVENTORY.CS using System; using System.Collections.Generic; using System.Collections; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class NissanDealershipInventory : CarAbstractInventory { ArrayList NissanCarsArray = new ArrayList();</p><p> public override Iterator CreateIterator() { return new NissanDealershipIterator(NissanCarsArray); }</p><p> public int Count { get { return NissanCarsArray.Count; } }</p><p> public void addItems(Cars car) { NissanCarsArray.Add(car); }</p><p>} }</p><p>//TOYOTADEALERSHIPINVENTORY.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class ToyotaDealershipInventory : CarAbstractInventory {</p><p> private List<Cars> ToyotaCarsList = new List<Cars>();</p><p> public override Iterator CreateIterator() { return new ToyotaDealershipIterator(ToyotaCarsList); }</p><p> public int Count { get { return ToyotaCarsList.Count; } }</p><p> public void addItems(Cars car) { ToyotaCarsList.Add(car); } } } //BMWDEALERSHIPINVENTORY.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class BmwDealershipInventory : CarAbstractInventory { private List<Cars> BmwCarsList = new List<Cars>();</p><p> public override Iterator CreateIterator() { return new ToyotaDealershipIterator(BmwCarsList); }</p><p> public int Count { get { return BmwCarsList.Count; } }</p><p> public void addItems(Cars car) { BmwCarsList.Add(car); } } }</p><p>// CARS.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.IteratorPattern { class Cars { string Brand; string Model; string Description; double price; public Cars(string brand, string model, string description, double price) { this.Brand = brand; this.Model = model; this.Description = description; this.price = price; }</p><p> public string getBrand { get{return Brand;} } public string getModel { get { return Model; } } public string getDescription { get { return Description; } } public double getPrice { get { return price; } } } }</p><p>// Codes for Car Inventory Control Application (Composite Pattern)</p><p>//PRORAM.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.CompositePattern { class Program { static void Main(string[] args) { CarsComponent NissanCars = new Inventory("Nissan Car Inventory "); CarsComponent ToyotaCars = new Inventory("Toyota Car Inventory "); CarsComponent BMWCars = new Inventory(" BMW Car Inventory "); ToyotaCars.addItems(new Cars("Toyota","Corolla"," New red corolla with sports package ", 19999)); ToyotaCars.addItems(new Cars("Toyota", "Camry", " New silver camry with the basic package", 25999)); ToyotaCars.addItems(new Cars("Toyota", "Avalon", " Used black Avalon with leather seats ", 15999)); NissanCars.addItems(new Cars("Nissan", "Sentra", " Used black Sentra with basic package", 10999)); NissanCars.addItems(new Cars("Nissan", "Maxima", " New gray Maxima with all the upgrades", 31999)); NissanCars.addItems(new Cars("Nissan", "GT-R", " New blue GT-R with a v8 turbo engine", 35999)); BMWCars.addItems(new Cars("BMW", "325I", "New charcoal 2010 bmw325i 5speed manual ", 38999)); BMWCars.addItems(new Cars("BMW", "745I", "New metallic blue 2010 bmw745i luxury pckg ", 80999)); BMWCars.addItems(new Cars("BMW", "X6", "New black 2010 bmwX6 premium package ", 60999)); BMWCars.addItems(new Cars("BMW", "M5", "New Red 2010 bmw M5 6speed manual ", 58999)); CarsComponent AllInventory = new Inventory(" All THE CARS ON THE LOT !!!! "); AllInventory.addItems(ToyotaCars); AllInventory.addItems(NissanCars); AllInventory.addItems(BMWCars); AllInventory.PrintInventory(); //Dealer CarSalesMan = new Dealer(AllInventory); //CarSalesMan.print();</p><p>Console.ReadKey();</p><p>} } }</p><p>//CARSCOMPONENT.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.CompositePattern {</p><p> abstract class CarsComponent { public abstract int Count(); public abstract void addItems(CarsComponent CarsComponent); public abstract string getBrand(); public abstract string getModel(); public abstract string getDescription(); public abstract double getPrice(); public abstract void PrintInventory(); public abstract string InventoryName(); } }</p><p>//DEALER.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.CompositePattern { class Dealer { CarsComponent allCarInventory; public Dealer(CarsComponent allCarInventory) { this.allCarInventory = allCarInventory; } public void print() { allCarInventory.PrintInventory(); } } }</p><p>//INVENTORY.CS using System; using System.Collections.Generic; using System.Collections; using System.Linq; using System.Text; namespace Abner._658.CompositePattern { class Inventory : CarsComponent { ArrayList InventoryList = new ArrayList(); string name; //string description; public Inventory(string name) { this.name = name; }</p><p> public override string InventoryName() { return name; } public override string getModel() { throw new NotImplementedException(); } public override string getBrand() { throw new NotImplementedException(); } public override double getPrice() { throw new NotImplementedException(); } public override string getDescription() { throw new NotImplementedException(); } public override void addItems(CarsComponent CarsComponent) { InventoryList.Add(CarsComponent); } public override int Count() { return InventoryList.Count; }</p><p> public override void PrintInventory() { Console.WriteLine("\n" + InventoryName()); Console.WriteLine(); // don't forget to mention the IEnumerator in c# IEnumerator iterator = InventoryList.GetEnumerator();</p><p> while (iterator.MoveNext()) { CarsComponent carComponents = (CarsComponent)iterator.Current; carComponents.PrintInventory(); }</p><p>}</p><p>} } //CARS.CS using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Abner._658.CompositePattern { class Cars : CarsComponent { string Brand; string Model; string Description; double price; public Cars(string brand, string model, string description, double price) { this.Brand = brand; this.Model = model; this.Description = description; this.price = price; } //public override Iterator CreateIterator() //{ // throw new NotImplementedException(); //} public override string InventoryName() { throw new NotImplementedException(); }</p><p> public override string getModel() { return Model; } public override string getBrand() { return Brand; } public override double getPrice() { return price; } public override string getDescription() { return Description; } public override void addItems(CarsComponent CarsComponent) { throw new NotImplementedException(); } public override int Count() { throw new NotImplementedException(); }</p><p> public override void PrintInventory() { Console.WriteLine("{0} {1} {2} {3}", getBrand(), getModel(), getDescription(), getPrice());</p><p>Console.WriteLine("------"); } } }</p><p>// Codes for Vehicle Test (Template Method Pattern) using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Vehicle { class Program { static void Main() { VehicleDesign teamA = new Car(); teamA.Tasks();</p><p>Console.WriteLine("\n"); VehicleDesign teamB = new Truck(); teamB.Tasks();</p><p>Console.ReadKey(); }</p><p>// VehicleDesign.cs //superclass public abstract class VehicleDesign { //Template public void Tasks() { AssignedToCarDesign(); AssignedToEngine(); AssignedToProgram(); //condition if (VehicleFeaturesTested()) { Repair(); Test(); }</p><p>} //abstract for sub to use their own methods public abstract void Repair(); public abstract void Test();</p><p> public void AssignedToCarDesign() { Console.WriteLine("Team A is responsible for vehicle Design"); }</p><p> public void AssignedToEngine() { Console.WriteLine("Team B are assigned to build vehicle Engine"); }</p><p> public void AssignedToProgram() { Console.WriteLine("Joe and Tim are assigned to build software"); }</p><p>//Using Hook method To conform vehicle tests bool VehicleFeaturesTested() { return true; }</p><p>}</p><p>// Car.cs // ConcreteClassA public class Car : VehicleDesign { public override void Repair()</p><p>{ Console.WriteLine("Car components functioning well (y/n)?"); string answer = getUserInput();</p><p> if (answer.ToLower().StartsWith("y")) {</p><p>Console.WriteLine("All components of a car are functioning well"); } else { Console.WriteLine("Patrick repair the brakes"); Console.WriteLine("Brakes need to be fixed"); } }</p><p> public override void Test() { Console.WriteLine("\nCar features working well (y/n)?"); string answer = getUserInput(); if (answer.ToLower().StartsWith("y")) { Console.WriteLine("All features of a car are functioning well");</p><p>} else { Console.WriteLine("Paul test the audio system"); Console.WriteLine("Audio system is not functioning well"); }</p><p>} private string getUserInput() { string answer = null;</p><p> try { answer = Console.ReadLine(); }</p><p> catch { Console.Error.WriteLine("IO error trying to read your answer"); }</p><p> if (answer == "n") { return "no"; } else if (answer == "y") { return "y"; } else { return "no"; } }</p><p>}</p><p>// Truck.cs //ConcreteClassB public class Truck : VehicleDesign {</p><p> public override void Repair() { Console.WriteLine("Truck components functioning well (y/n)?"); string answer = getUserInput();</p><p> if (answer.ToLower().StartsWith("y")) {</p><p>Console.WriteLine("All components of a truck are functioning well"); } else { Console.WriteLine("Tom repair air conditioner"); Console.WriteLine("Air conditioner is not functioning well"); } }</p><p> public override void Test() { Console.WriteLine("\nTruck features working well (y/n)?"); string answer = getUserInput();</p><p> if (answer.ToLower().StartsWith("y")) { Console.WriteLine("All features of truck are functioning well"); } else { Console.WriteLine("Robert test the cruise control"); Console.WriteLine("Cruise control is not functioning well"); } }</p><p> private string getUserInput() { string answer = null;</p><p> try { answer = Console.ReadLine(); } catch { Console.Error.WriteLine("IO error trying to read your answer"); }</p><p> if (answer == "n") { return "no"; } else if (answer == "y") { return "y"; } else { return "no"; } } }</p><p>} }</p><p>// Code for Vehicle Gears application (State Pattern)</p><p>// Code in Program.cs class Program { static void Main(string[] args) {</p><p>AutomaticGear gear = new AutomaticGear(1);</p><p> gear.Info(); gear.parkingGear(); gear.driveGear(); gear.neutralGear(); gear.reverseGear(); gear.changeGear(); Console.WriteLine("\n");</p><p> gear.Info(); gear.reverseGear(); gear.parkingGear(); gear.driveGear(); gear.neutralGear(); gear.changeGear(); Console.WriteLine("\n");</p><p> gear.Info(); gear.neutralGear(); gear.parkingGear(); gear.driveGear(); gear.changeGear(); Console.WriteLine("\n");</p><p> gear.Info(); gear.driveGear(); gear.neutralGear(); gear.reverseGear(); gear.parkingGear(); gear.changeGear(); Console.WriteLine("\n");</p><p> gear.Info();</p><p> gear.driveGear(); gear.neutralGear(); gear.reverseGear(); gear.parkingGear();</p><p>Console.ReadKey(); } } }</p><p>// State.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public interface State { void parkingGear(); void reverseGear(); void changeGear(); void neutralGear(); void driveGear();</p><p>} }</p><p>// AutomaticGear.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class AutomaticGear {</p><p>State parkingState; State driveState; State neutralState; State reverseState; State _state;</p><p> int count = 1; // constructor for state instances public AutomaticGear(int testNumber) { parkingState = new ParkingState(this); reverseState = new ReverseState(this); neutralState = new NeutralState(this); driveState = new DriveState(this); _state = new SetState(this); this.count = testNumber;</p><p> if (testNumber > 0) { _state = parkingState;</p><p>} }</p><p> public void parkingGear() {</p><p>_state.parkingGear();</p><p>}</p><p> public void reverseGear() {</p><p>_state.reverseGear(); }</p><p> public void changeGear() {</p><p>_state.changeGear();</p><p>}</p><p> public void neutralGear() {</p><p>_state.neutralGear(); }</p><p> public void driveGear() {</p><p>_state.driveGear(); } public void setState(State state) { this._state = state; </p><p>}</p><p> public void NumberOfTests() { if (count >= 1) { count += 1; } } // get States public State getParkingState() { return parkingState; } public State getNeutralState() { return neutralState; } public State getReverseState() { return reverseState; } public State getDriveState() { return driveState; }</p><p> public int getCount() { return count; }</p><p> public void Info() { if (count == 1) { Console.WriteLine("Test #1: Shift vehicle gear from parking to reverse\n"); } else if (count == 2) { Console.WriteLine("Test #2: Shift vehicle gear from reverse to neutral\n"); } else if (count == 3) { Console.WriteLine("Test #3: Shift vehicle gear from neutral to drive\n"); } else if (count == 4) { Console.WriteLine("Test #4: Shift vehicle gear from drive to parking\n"); } else { Console.WriteLine("Test #5: Check whether gear shifted to parking"); } }</p><p>}</p><p>}</p><p>// ParkingState.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class ParkingState : State { AutomaticGear automaticGear;</p><p> public ParkingState(AutomaticGear automaticGear) { this.automaticGear = automaticGear;</p><p>} public void parkingGear() {</p><p>Console.WriteLine("Vehicle is currently in Parking gear"); }</p><p> public void reverseGear() { Console.WriteLine("Vehicle cannot switch to reverse gear until brake is applied "); }</p><p> public void changeGear() { Console.WriteLine("Brakes applied .... "); Console.WriteLine("Gear can shift to reverse gear now"); automaticGear.NumberOfTests(); if (automaticGear.getCount() == 2) { automaticGear.setState(automaticGear.getReverseState()); }</p><p>}</p><p> public void neutralGear() { Console.WriteLine("Gear is not in neutral"); // automaticGear.setState(automaticGear.getNeutralState()); }</p><p> public void driveGear() { Console.WriteLine("Gear is not in drive"); } } } // ReverseState.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class ReverseState : State { AutomaticGear automaticGear;</p><p> public ReverseState(AutomaticGear automaticGear) { this.automaticGear = automaticGear; }</p><p> public void parkingGear() { Console.WriteLine("Gear is not in Parking"); }</p><p> public void reverseGear() { Console.WriteLine("Gear is in reverse "); }</p><p> public void changeGear() { Console.WriteLine("Brakes applied .... "); Console.WriteLine("Gear can shift to neutral gear now"); automaticGear.NumberOfTests(); if (automaticGear.getCount() == 3) { automaticGear.setState(automaticGear.getNeutralState());</p><p>} } public void neutralGear() { Console.WriteLine("Brake needs to be applied first before shifting to neutral"); }</p><p> public void driveGear() { Console.WriteLine("Gear is not in drive"); }</p><p>} }</p><p>// NeutralState.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class NeutralState : State { AutomaticGear automaticGear;</p><p> public NeutralState(AutomaticGear automaticGear) { this.automaticGear = automaticGear; }</p><p> public void parkingGear() { Console.WriteLine("Gear is not in parking"); }</p><p> public void reverseGear() { Console.WriteLine("Gear is not in reverse "); }</p><p> public void changeGear() {</p><p>Console.WriteLine("Brakes applied .... "); Console.WriteLine("Gear can shift to drive gear now"); automaticGear.NumberOfTests(); if (automaticGear.getCount() == 4) { automaticGear.setState(automaticGear.getDriveState()); //change this to driveState();</p><p>} } public void neutralGear() { Console.WriteLine("Gear is currently in neutral");</p><p>}</p><p> public void driveGear() { Console.WriteLine("Vehicle cannot switch to drive gear until brake is applied"); }</p><p>} }</p><p>// DriveState.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class DriveState : State { AutomaticGear automaticGear;</p><p> public DriveState(AutomaticGear automaticGear) { this.automaticGear = automaticGear; } public void parkingGear() { Console.WriteLine("Brakes need to be applied before shifting gear to parking"); }</p><p> public void reverseGear() { Console.WriteLine("Gear is not in reverse"); }</p><p> public void changeGear() { Console.WriteLine("Brakes applied .... "); Console.WriteLine("Gear can shift to parking gear now"); automaticGear.NumberOfTests(); if (automaticGear.getCount() == 5) { automaticGear.setState(automaticGear.getParkingState()); } }</p><p> public void neutralGear() { Console.WriteLine("Gear is not in neutral"); }</p><p> public void driveGear() { Console.WriteLine("Vehicle is currently in drive gear"); } }</p><p>} //SetState.cs using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace VehicleGears { public class SetState : State { AutomaticGear automaticGear;</p><p> public SetState(AutomaticGear automaticGear) { this.automaticGear = automaticGear; } public void parkingGear() { } public void reverseGear() { } public void neutralGear() { } public void changeGear() { } public void driveGear() { } } }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages29 Page
-
File Size-