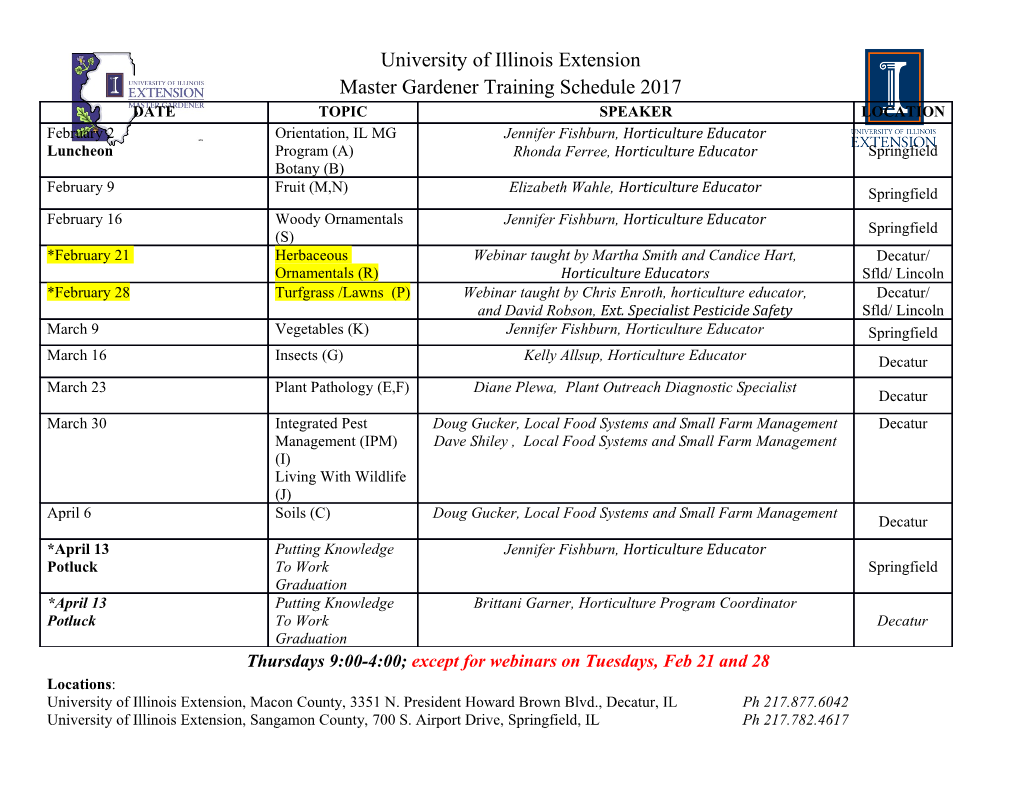
<p> Name:______CSCI 1301 Introduction to Programming Covers Chapter 4 Armstrong Atlantic State University Instructor: Y. Daniel Liang</p><p>I pledge by honor that I will not discuss this exam with anyone until my instructor reviews the exam in the class. Signed by ______.</p><p>Part I. (a) (3 pts) If you enter input 9, show the printout of the following code:</p><p> import java.util.Scanner;</p><p> public class Test { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter an integer: "); int number = input.nextInt(); </p><p> int i;</p><p> boolean isPrime = true; for (i = 2; i < number && isPrime; i++) { if (number % i == 0) { isPrime = false; } }</p><p>System.out.println("i is " + i);</p><p> if (isPrime) System.out.println(number + " is prime"); else System.out.println(number + " is not prime"); } }</p><p>(b) (2 pts) public class Test { public static void main(String[] args) { for (int i = 0; i < 5; i++) System.out.print((i + 4) + " "); } }</p><p>1 (c) (4 pts) public class Test { public static void main(String[] args) { int i = 1; while (i <= 4) { int num = 1; for (int j = 1; j <= i; j++) { System.out.print(num + "bb"); num *= 3; }</p><p>System.out.println(); i++; } } }</p><p>Part II: (8 pts each): </p><p>1. Write a complete program that prints numbers from 1 to 50, but if numbers that are multiples of 5, print HiFive, else if numbers that are divisible by 2, print HiTwo, and else if numbers that are divisible by 3 or 7, print HiThreeOrSeven.</p><p>2 2. Write a loop that computes (No need to write a complete program)</p><p>1 2 3 98 99 ... 2 3 4 99 100</p><p>3 Part III: Multiple Choice Questions: (1 pts each) (1. Mark your answers on the sheet. 2. Login and click Take Instructor Assigned Quiz for Quiz2. 3. Submit it online within 5 mins. 4. Close the Internet browser.) </p><p>1 What is the output for y? int y = 0; for (int i = 0; i<10; ++i) { y += i; } System.out.println(y); a. 45 b. 11 c. 13 d. 12 e. 10 </p><p>#</p><p>2 Analyze the following code.</p><p> int x = 1; while (0 < x) && (x < 100) System.out.println(x++); a. The loop runs forever. b. The code does not compile because the loop body is not in the braces. c. The code does not compile because (0 < x) && (x < 100) is not enclosed in a pair of parentheses. d. The numbers 1 to 99 are displayed. e. The numbers 2 to 100 are displayed. </p><p>#</p><p>3 How many times will the following code print "Welcome to Java"? int count = 0; do { System.out.println("Welcome to Java"); count++; } while (count < 10);</p><p>A. 8 B. 0 C. 11 D. 10 </p><p>4 E. 9 </p><p>#</p><p>4 What is i after the following for loop is finished? int y = 0; for (int i = 0; i<10; ++i) { y += i; }</p><p>A. 10 B. undefined C. 9 D. 11 </p><p>#</p><p>5 Analyze the following code: public class Test { public static void main (String args[]) { int i = 0; for (i = 0; i < 10; i++); System.out.println(i + 4); } } </p><p>A. The program compiles despite the semicolon (;) on the for loop line, and displays 4. B. The program compiles despite the semicolon (;) on the for loop line, and displays 14. C. The program has a compile error because of the semicolon (;) on the for loop line. D. The program has a runtime error because of the semicolon (;) on the for loop line. </p><p>#</p><p>6 Which of the following expression yields an integer between 0 and 100, inclusive? A. (int)(Math.random() * 100 + 1) B. (int)(Math.random() * 101) C. (int)(Math.random() * 100) D. (int)(Math.random() * 100) + 1 </p><p>#</p><p>7 Analyze the following code. int count = 0;</p><p>5 while (count < 100) { // Point A System.out.println("Welcome to Java!"); count++; // Point B }</p><p>// Point C</p><p>A. count < 100 is always false at Point A B. count < 100 is always true at Point B C. count < 100 is always false at Point C D. count < 100 is always true at Point C E. count < 100 is always false at Point B </p><p>#</p><p>8. What is the output of the following fragment? int i = 1; int j = 1; while (i < 5) { i++; j = j * 2; } System.out.println(j); a. 4 b. 8 c. 16 d. 32 e. 64</p><p>6 Solution:</p><p>Part I. (a) i is 4 9 is not prime</p><p>(b) 4 5 6 7 8</p><p>(c) 1bb 1bb3bb 1bb3bb9bb 1bb3bb9bb27bb</p><p>Part II: </p><p>(a) </p><p> public class Test { public static void main(String[] args) { for (int i = 1; i <= 50; i++) if (i % 5 == 0) System.out.println("HiFive"); else if (i % 2 == 0) System.out.println("HiTwo"); else if (i % 3 == 0 || i % 7 == 0) System.out.println("HiThreeOrSeven"); else System.out.println(i + " "); } }</p><p>(b) </p><p> public class Test { /**Main method*/ public static void main(String[] args) { double sum = 0;</p><p> for (int i = 1; i <= 99; i++) sum += (i * 1.0 / (i + 1));</p><p>// Display results System.out.println("Sum is " + sum); } }</p><p>1. What is the output for y? int y = 0;</p><p>7 for (int i = 0; i<10; ++i) { y += i; } System.out.println(y); a. 45 b. 11 c. 13 d. 12 e. 10 Key:a</p><p>#</p><p>2. Analyze the following code.</p><p> int x = 1; while (0 < x) && (x < 100) System.out.println(x++); a. The loop runs forever. b. The code does not compile because the loop body is not in the braces. c. The code does not compile because (0 < x) && (x < 100) is not enclosed in a pair of parentheses. d. The numbers 1 to 99 are displayed. e. The numbers 2 to 100 are displayed. Key:c</p><p>#</p><p>3. How many times will the following code print "Welcome to Java"? int count = 0; do { System.out.println("Welcome to Java"); count++; } while (count < 10);</p><p>A. 8 B. 0 C. 11 D. 10 E. 9 Key:d</p><p>#</p><p>8 4. What is i after the following for loop is finished? int y = 0; for (int i = 0; i<10; ++i) { y += i; }</p><p>A. 10 B. undefined C. 9 D. 11 Key:b</p><p>#</p><p>5. Analyze the following code: public class Test { public static void main (String args[]) { int i = 0; for (i = 0; i < 10; i++); System.out.println(i + 4); } } </p><p>A. The program compiles despite the semicolon (;) on the for loop line, and displays 4. B. The program compiles despite the semicolon (;) on the for loop line, and displays 14. C. The program has a compile error because of the semicolon (;) on the for loop line. D. The program has a runtime error because of the semicolon (;) on the for loop line. Key:b</p><p>#</p><p>6. Which of the following expression yields an integer between 0 and 100, inclusive? A. (int)(Math.random() * 100 + 1) B. (int)(Math.random() * 101) C. (int)(Math.random() * 100) D. (int)(Math.random() * 100) + 1 Key:b</p><p>#</p><p>7. Analyze the following code. int count = 0; while (count < 100) { // Point A</p><p>9 System.out.println("Welcome to Java!"); count++; // Point B }</p><p>// Point C</p><p>A. count < 100 is always false at Point A B. count < 100 is always true at Point B C. count < 100 is always false at Point C D. count < 100 is always true at Point C E. count < 100 is always false at Point B Key:c</p><p>#</p><p>8. What is the output of the following fragment? int i = 1; int j = 1; while (i < 5) { i++; j = j * 2; } System.out.println(j); a. 4 b. 8 c. 16 d. 32 e. 64 Key:c</p><p>10</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages10 Page
-
File Size-