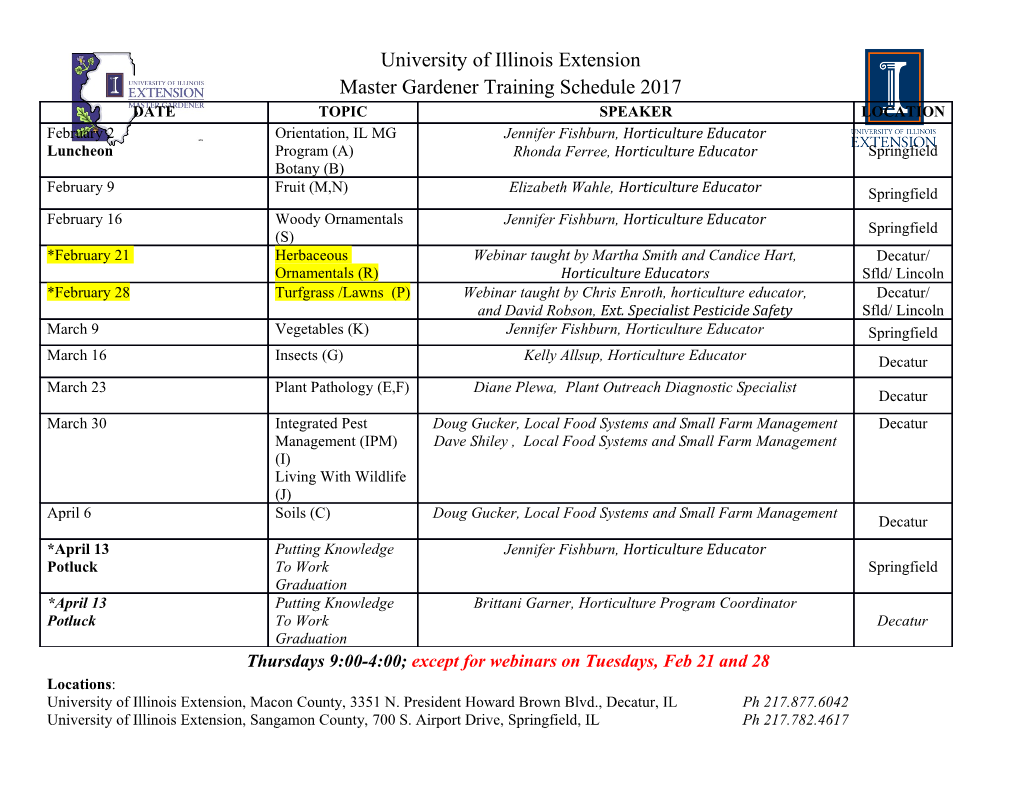
<p> 1</p><p>Chapter Four: Repetion and Recursion Methods for repetion 1. The Backtrack After Fail (BAF) method 2. The Cut And Fail (CAF) method 3. The User-defined Repeat (UDR) method</p><p>The Backtrack After Fail (BAF) method BAF method can be used with an internal goal to find all the possible solutions to the goal. The BAF method uses the fail predicate. EX: The purpose of this program is to list the name of 6 Jordanians cities. domains name = string predicates cities(name) show_cities goal write (“Here are the cities:”), nl, show_cities. clauses cities(“Barka”). Here are the cities: cities(“Muscat”). Barka cities(“Rustaq”). Muscat cities(“Suhar”). Rustaq cities(“Salala”). Suhar cities(“Nizwa”). Salala Nizwa show_cities:- cities(City), write(“ “, City),nl, fail. The fail predicate causes backtracking to the next clause that might satisfy the goal.</p><p>EX: To show the use of selective rules using BAF method by listing the male employees only. 2</p><p> domains name, sex, department = symbol pay_rate = real predicates employee(name, sex, department, pay_rate) show_male_part_time goal write(“Male Part Time Employee”), nl, nl, show_male_part_time. clauses employee(“Osama”, “M”, “CS” , 3.50). employee(“Rasha”, “F”, “MIS” , 4.50). employee(“Hayat”, “F”, “CIS” , 5.00). employee(“Riyad”, “M”, “CS” , 4.00). employee(“Hasan”, “M”, “MIS” , 6.00). employee(“Sawsan”, “F”, “CIS” , 5.00).</p><p> show_male_part_time:- employee(Name, “M”, Dept, Pay_rate), write(Name, Dept, “$”, Pay_rate), nl, fail. OR show_male_part_time:- employee(Name, Sex, Dept, Pay_rate), Sex = “M”, write(Name, Dept, “$”, Pay_rate), nl, fail.</p><p>Male Part Time Employee Osama CS $ 3.50 Riyad CS $ 4.00 Hasan MIS $ 6.00</p><p> The fail predicate will not be needed in the case of failure to match the rule’s conditions because the subgoal fails on its own. The fail predicate is included in the rule to force backtracking when the rule’s conditions are met and the rule succeeds. 3</p><p>EX: To show rule condition and making report using BAF method. domains name, sex, department = symbol pay_rate, hours, gross_pay = real predicates employee(name, sex, department, pay_rate, hours) make_payroll_report compute_gross_pay(pay_rate, hours, gross_pay) goal write(“Employee Payroll report”), nl, nl, make_payroll_report. clauses employee(“Osama”, “M”, “CS” , 3.50, 40.00). employee(“Rasha”, “F”, “MIS” , 4.50, 36.00). employee(“Hayat”, “F”, “CIS” , 5.00, 40.00). employee(“Riyad”, “M”, “CS” , 4.00, 25.00). employee(“Hasan”, “M”, “MIS” , 6.00, 30.00). employee(“Sawsan”, “F”, “CIS” , 5.00, 20.00).</p><p> make_payroll_report:- employee(Name, _, Dept, Pay_rate, Hours), compute_gross_pay(Pay_rate, Hours, Gross_pay) write(Name, Dept, “$”, Gross_pay), nl, fail. compute_gross_pay(Pay_rate, Hours, Gross_pay):- Gross_pay = Pay_rate * Hours.</p><p>Employee Payroll Rerport Osama CS $ 120 Rasha MIS $162 Hayat CIS $200 Riyad CS $ 100 Hasan MIS $ 180 Sawsan CIS $ 100</p><p>Exercise: Solve exercise number 4.5 on page 117 in the first reference. 4</p><p>EX: predicates visit(symbol) What = red_sea middle_east(symbol) What = arab_sea clauses What = mediterranean visit(Sea):- 3 solutions middle_east(Sea). middle_east(dead_sea):- fail. /* prevent printing Dead Sea */ middle_east(red_sea). middle_east(arab_sea). middle_east(mediterranean). External Goal: visit(What)</p><p>The Cut and Fail (CAF) Method The cut predicate (exclamation mark !), which always succeeds, causes the internal unification routines to forget any backtracking markers that have been placed during attempting to satisfy the current subgoal. Subsequent subgoals may generate more backtracking markers and therefore opportunities to backtrack to more solutions; the cut will not prevent these subsequent backtrackings.</p><p>EX: domains person = symbol predicates child(person) show_some_of_them clauses child(“Tom”). Tom child(“Beth”). Beth child(“Jeff”). Jeff child(“Sara”). Sara child(“Larry”). Larry child(“Peter”). Peter child(“Diana”). Diana child(“Judy”). 5</p><p> child(“Sandy”). show_some_of_them:- child(Name), write(Name),nl, Name = “Diana”, !. External Goal: show_some_of_them</p><p>EX: To get the first occurrence of a name. domains person = symbol predicates child(person) get_first_occurrence clauses child(“Tom”). child(“Beth”). child(“Jeff”). child(“Sara”). Sara child(“Larry”). child(“Peter”). child(“Diana”). child(“Judy”). child(“Sara”). get_first_occurrence :- child(Name), Name = “Sara”, write(Name), nl, !. External Goal: get_first_occurrence 6</p><p>EX: predicates color(symbol) combination(symbol, symbol) clauses color(green). color(red). X = green, Y = green combination(X, Y):- X = green, Y = red color(X), !, 2 solutions color(Y). External Goal: combination(X, Y).</p><p>EX: predicates color(symbol) combination(symbol, symbol) clauses color(green). color(red). X = green, Y = green combination(X, Y):- 1 solution color(X), !, color(Y), !. External Goal: combination(X, Y)</p><p>EX: predicates likes(symbol, symbol) common_interest(symbol, symbol) clauses likes(ahmed, ball). likes(ahmed, reading). likes(asma, ball). likes(asma, reading). likes(tariq, ball). likes(tariq, reading). common_interest(Person1, Person2):- likes(Person1, Interest), 7</p><p> likes(Person2, Interest), Person1 <> Person2, !.</p><p>External Goal: common interest(ahmed, Who) /* The first person has ahmed’s interest */ Who = asma 1 Solution</p><p>EX: predicates a b c(integer) d(integer) e(integer) f(integer) clauses a:- b, write(“Success”). a:- write(“Failure”). b:- c(X), d(X), !, e(Y), f(X). b. c(1). c(2). c(3). d(3). e(1). e(2). f(1). External Goal: a 8</p><p>EX: Adam and Eve have no parents, Jesus has one parent, others have two parents. Solution No. 1: predicates number_of_parents(symbol, integer) clauses number_of_parents(adam, 0):- !. number_of_parents(eve, 0):- Who = adam !. 1 Solution number_of_parents(jesus, 1):- !. number_of_parents(_, 2).</p><p>External Goal: number_of_parents(Who, 0)</p><p> The problem in this example is that it can’t find the other solution (Who = eve).</p><p>Solution No. 2: predicates number_of_parents(symbol, integer) clauses number_of_parents(adam, 0). Howmany = 0 number_of_parents(eve, 0). Howmany = 2 number_of_parents(jesus, 1). 2 Solution number_of_parents(_, 2).</p><p>External Goal: number_of_parents(adam, Howmany)</p><p> The problem in this example is that the underscore matches any name, therefore a wrong second solution will be displayed. 9</p><p>Solution No. 3: predicates number_of_parents(symbol, integer) clauses number_of_parents(jesus, 1):- !. number_of_parents(adam, 0). number_of_parents(eve, 0):- !. number_of_parents(X, 2):- X <> adam, X <> eve, X <> jesus. External Goal: number_of_parents(adam, Howmany)</p><p>Howmany = 0 1 Solution</p><p>External Goal: number_of_parents(Who, 0) Who = adam Who = eve 2 Solutions</p><p>EX: Ahmed wants to visit all seas except the dead sea. predicates visit(symbol, string) clauses visit(Seaname, Status):- Seaname = deadsea, !, Status = “It is not OK”. visit(_, Status):- Status = “It is OK”. External Goal: visit (deadsea, S) S = It is not OK External Goal: visit (arabsea, S) S = It is OK 10</p><p>The User Defined Repeat (UDR) Method The UDR method makes backtracking always possible instead of forcing failure and subsequent backtracking. The form of UDR rule is: repeat. repeat:- repeat. Because the first repeat generates no subgoals, the rule always succeeds. Because a variant of the rule exists, a backtracking marker is left of the second repeat. When the second repeat calls the third repeat, it succeeds because the first repeat satisfies the subgoal repeat. Consequently, the repeat rule always succeeds. This implies that repeat is a never failing recursive rule.</p><p>EX: This program accepts string data from the keyboard and echoes it on the screen. When the user types stop, the program terminates. domains name = symbol predicates write_message repeat do_echo check(name) goal write_message, do_echo. clauses repeat. repeat:- repeat. write_message:- write(“Please enter names”), nl, write(“I shall repeat them”), nl, write(“To stop me, enter ‘stop’”), nl, nl. do_echo:- repeat, readln(Name), write(Name), nl, check(Name), !. 11</p><p> check(stop):- write(“OK, bye.”). check(_):- fail. You may choose a rule has other name than repeat, such as loop, iterate, recursive, ……. </p><p>EX: This program to find the summation of two numbers. The number 99 will stop the program.</p><p>/* This program has a problem */ domains number = integer predicates addnumbers loop check(number) goal loop, addnumbers. clauses loop. loop:- loop. addnumbers:- readint(X), readint(Y), Z = X + Y, write(“Sum =”, Z), nl, check(X). check(99):- !. check(_):- fail. 12</p><p>/* This how to solve the problem */ domains number = integer predicates loop check(number) goal loop, readint(X), check(X). clauses loop. loop:- loop. check(99):- !. check(X):- readint(Y), Z = X + Y, write(“Sum =”, Z), nl, fail. 13</p><p>Recursion If a rule contains itself as a component, the rule is said to be recursive. EX: write_string:- /* infinite loop */ write(“Ramadan Kareem), nl, write_string. EX: domains char_data = char predicates write_prompt read_a_character goal write_prompt, read_a_character. clauses write_prompt:- write(“Please enter characters”), nl, write(“To terminate, enter ‘#’.”), nl. read_a_character:- readchar(Char_data), Char_data <> ‘#’, /* Exit Condition */ write(Char_data), read_a_character. The General Recursive Rule (GRR) for defining a recursive is: < recursive rule name >:- < predicate list >, /* 1 */ < exit condition predicate >, /* 2 */ < predicate list >, /* 3 */ < recursive rule name >, /* 4 */ < predicate list >. /* 5 */ 1. A group of predicates. The success or failure of any one of these predicates does not cause recursion. 2. The success or failure of this predicate either allows the recursion to go on or cause it to stop. 3. A group of predicates. The success or failure of any one of these predicates does not cause recursion. 4. The success of this rule involves recursion. 14</p><p>5. A list of predicates, whose success or failure does not influence the recursion. It also receive values pushed onto the stack during the time that recursion was taking place.</p><p>EX: This example for ordering a sequence of numbers in ascending order.</p><p> domains number = integer predicates 1 write_number(number) 2 goal 3 write_number(1), nl. 4 clauses 5 write_number(8). 6 write_number(Number):- 7 /* Number < 8, is needed if external goal is used */ write(Number), nl, Next_number = Number + 1, write_number(Next_number).</p><p>EX: This example to find sum of a series of numbers. domains number, sum = integer S(7) = 28 predicates sum_series(number, sum) sum_series(1, Sum) 1 goal sum_series(2, Sum) 3 sum_series(7, Sum), sum_series(3, Sum) 6 write(“S(7) =”, Sum), nl. sum_series(4, Sum) 10 clauses sum_series(5, Sum) 15 sum_series(1,1):- sum_series(6, Sum) 21 !. sum_series(7, Sum) 28 sum_series(Number, Sum):- Next_number = Number – 1, sum_series(Next_number, Partial_sum), Sum = Number + Parital_sum. 15</p><p>EX: This example to find factorial of a positive number. domains number, product = integer 7! = 5040 predicates factorial(number, product) factorial (1, Result ) 1 goal factorial (2, Result ) 2 factorial (7, Result), factorial (3, Result ) 6 write(“7! =”, Result), nl. factorial (4, Result ) 24 clauses factorial (5, Result ) 120 factorial (1,1):- factorial (6, Result ) 720 !. factorial (7, Result ) 5040 factorial (Number, Result):- Next_number = Number – 1, factorial (Next_number, Partial_factorial), Result = Number * Parital_factorial.</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages15 Page
-
File Size-