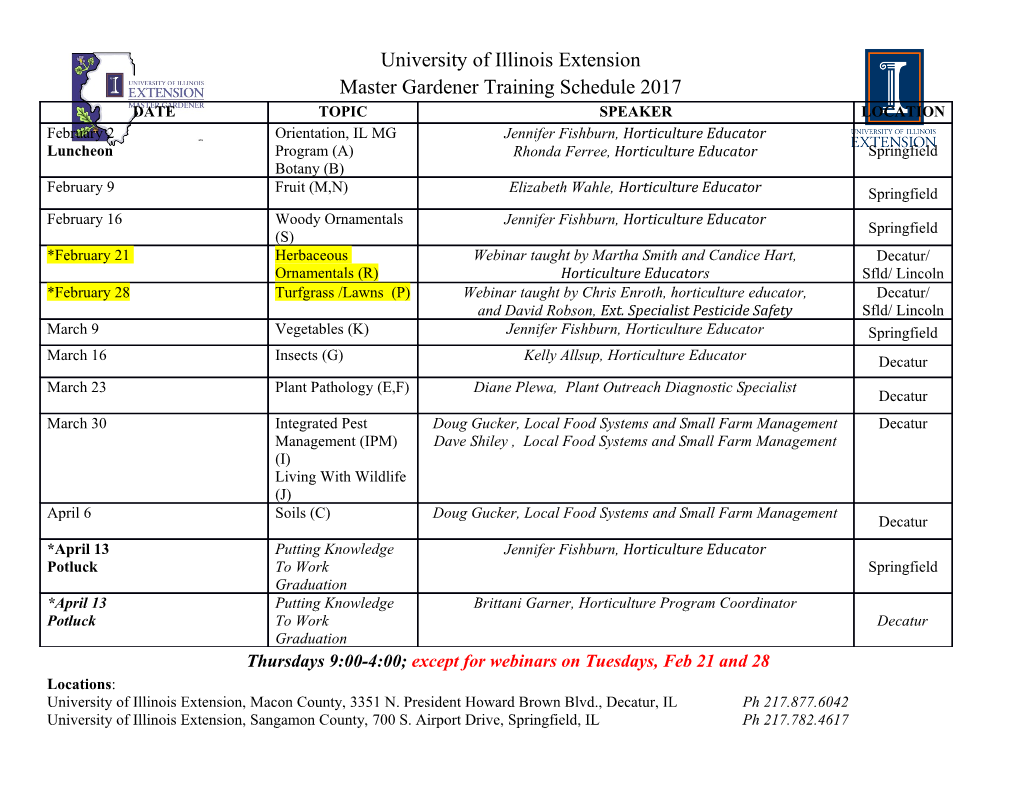
<p> Cucumber ‘Hello world’ setup</p><p>Document date: 17/02/2014 (revised 10/05/2014) (revised 13/10/2017)</p><p>Author: Andrew White</p><p>Overview</p><p>This document explains how to set up cucumber on your PC/Laptop. The software used is the Eclipse Platform which is designed for building integrated development environments (IDEs). It can also be used to create diverse end-to-end computing solutions for multiple execution environments. Two Eclipse plugins are also used, these are Maven and Cucumber.</p><p>Eclipse</p><p>The Eclipse Platform is designed for building integrated development environments (IDEs). It can also be used to create diverse end-to-end computing solutions for multiple execution environments.</p><p> create a folder called ‘C:\eclipse_workspaces’</p><p> create a folder called ‘C:\eclipse_workspaces\Fitnesse’</p><p> Download ‘Eclipse Standard’ from http://www.eclipse.org/downloads/</p><p> Extract the eclipse zip file into a folder called ‘C:\eclipse_workspaces\eclipse’ </p><p> Create a desktop shortcut to the ‘C:\eclipse_workspaces\eclipse\eclipse.exe’ </p><p> Open ‘eclipse.exe’ – Run application you will be asked to select a workspace - Choose ‘c:\Eclipse_Workspaces\Fitnesse (use as default)</p><p> Within Eclipse, delete the welcome screen or minimise as per preference</p><p>Eclipse – install Maven Depending on your Eclipse version, you may have to install Maven; To check whether you have Maven installed; Eclipse | file | new | other | ‘maven project’ should appear as an option</p><p>To install Maven; Eclipse | help | install New Software | Click ‘add’ In the ‘Add Repository’ area copy & paste this url;</p><p> http://download.eclipse.org/technology/m2e/releases/1.4/1.4.0.20130601-0317</p><p> click ‘ok’ next | finish</p><p>MAVEN</p><p>Create a Maven project called ‘cucumber.examples.java.helloworld’ file | new | other | maven project | </p><p> next wait org.apache.maven.archetypes artifact.id = maven-archetype-quickststart version = 1.2 next</p><p> group id = cucumber.examples.java package = cucumber.examples.java.helloworld finish now you should see the ‘helloworld’ project in eclipse looking exactly like this screen shot; delete AppTest.java and App.java pom.xml create dependencies in ‘pom.xml’ pom.xml | left mouse click | dependencies | left mouse click | ‘add’</p><p> pom.xml dependencies to first check that dependencies can be found – type junit in ‘Group Id’, ‘artifact Id’, ETC as per the screenshot below; IF no Search results appear as per the following; </p><p>– then please proceed with the following; ______(Menu) Window -> Show View -> Other -> Maven -> Maven Repositories In the window that appears: </p><p>Right-click on Global Repositories and select "Go Into" Right-click on "central (http://repo.maven.apache.org/maven2)" and select "Rebuild Index" It will take some time (up to 5 minutes) to complete the download. While the index is downloading/rebuilding, the text "central (http://repo.maven.apache.org/maven2)" is italicised. Once it is finished, it goes back to normal text (non-italic). ______ELSE </p><p>Junit (please note that you will need to obtain latest versions as Java/cucumber etc evolves) you will need to add the latest junit version (EG 4.11) and this will overwrite the present 3.8.1 version cucumber-java:1.1.2 (for java 8 we suggest using ‘cucumber—java8’) </p><p> cucumber-junit:1.1.2 press ok then set properties to ‘test’</p><p> cucumber-picocontainer:1.1.2 set properties to test</p><p>Cucumber feature file cucumber feature file folder you will need to create a cucumber feature file first create a folder – this is where you will place your feature file eclipse | project explorer | click on helloworld project | | new | source folder | the new folder src/test/resources</p><p> click finish to create a feature file click to highlight the project right click | new | file call the new file ‘helloworld.feature’</p><p> finish now move the new file into the new folder called src/test/resources (you may need to copy then paste to the new location and then delete the original ‘helloworld.feature) feature file contents add this content into the feature file;</p><p>+++++++++</p><p>Feature: Hello World Scenario: Say hello Given I have a hello app with "Howdy" When I ask it to say hi Then it should answer with "Howdy World"</p><p>+++++++++++++++++++++++++++++ CukesTest (cucumber runner)</p><p>RunCukesTest.java create a new java class in; helloworld | src/test/java | cucumber.examples.java.helloworld | right click on ‘cucumber.examples.java.helloworld’ folder new class</p><p>RunCukesTest finish cucumber runner java code delete all code except for the package definition add this code into the class; import cucumber.api.junit.*; import cucumber.api.*; import org.junit.runner.*; import org.junit.runner.RunWith;</p><p>@RunWith(Cucumber.class) //@Cucumber.Options(format = {"pretty", "html:target/cucumber"}, @CucumberOptions(format = {"pretty", "html:target/cucumber"}, features="src/test/resources" ) public class RunCukesTest { }</p><p>HelloStepdefs.java</p><p>To create a java file which contains all the instructions to begin testing follow these steps; highlight cucumber.examples.java.helloworld | right click | new Class | name | helloStepdefs</p><p> finish helloStepdefs code; highlight RunCukesTest.java | right-click | run as | junit test | ‘given when then’ code is produced which can be copied and pasted into the new java class called Hellostepdefs</p><p>EG this is likely to be the code produced in the console (to see console – Eclipse | Window | show view | console); @Given("^I have a hello app with \"([^\"]*)\"$") public void I_have_a_hello_app_with(String greeting) { // generated code }</p><p>@When("^I ask it to say hi$") public void I_ask_it_to_say_hi() { // generated code }</p><p>@Then("^it should answer with \"([^\"]*)\"$") public void it_should_answer_with(String expectedHi) { assertEquals(expectedHi, hi); }</p><p>^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^</p><p>This is the suggested code that you should put into your HelloStepdefs class; package cucumber.examples.java.helloworld; import static org.junit.Assert.assertEquals; import cucumber.api.java.en.Given; import cucumber.api.java.en.Then; import cucumber.api.java.en.When; public class HelloStepdefs { private Hello hello; private String hi; </p><p>@Given("^I have a hello app with \"([^\"]*)\"$") public void I_have_a_hello_app_with(String greeting) { hello = new Hello(greeting); }</p><p>@When("^I ask it to say hi$") public void I_ask_it_to_say_hi() { hi = hello.sayHi(); }</p><p>@Then("^it should answer with \"([^\"]*)\"$") public void it_should_answer_with(String expectedHi) { assertEquals(expectedHi, hi); } } ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ copy & paste the code into helloStepdefs.java Hello.java we need an implementation of our Hello World system in helloworld/src/maint/java / cucumber.examples.java.helloworld </p><p>In cucumber.examples.java.helloworld Create a class called Hello.java;</p><p> to invoke the whole test you will need to add this code to the ‘Hello’ method package cucumber.examples.java.helloworld; public class Hello { private final String greeting;</p><p> public Hello(String greeting) { this.greeting = greeting; }</p><p> public String sayHi() { return greeting + " World"; } } PERFORM TEST </p><p>In order to verify that the helloworld test works we simply re-run the ‘RunCukesTest.java’</p><p> the test should return a green colour </p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages20 Page
-
File Size-