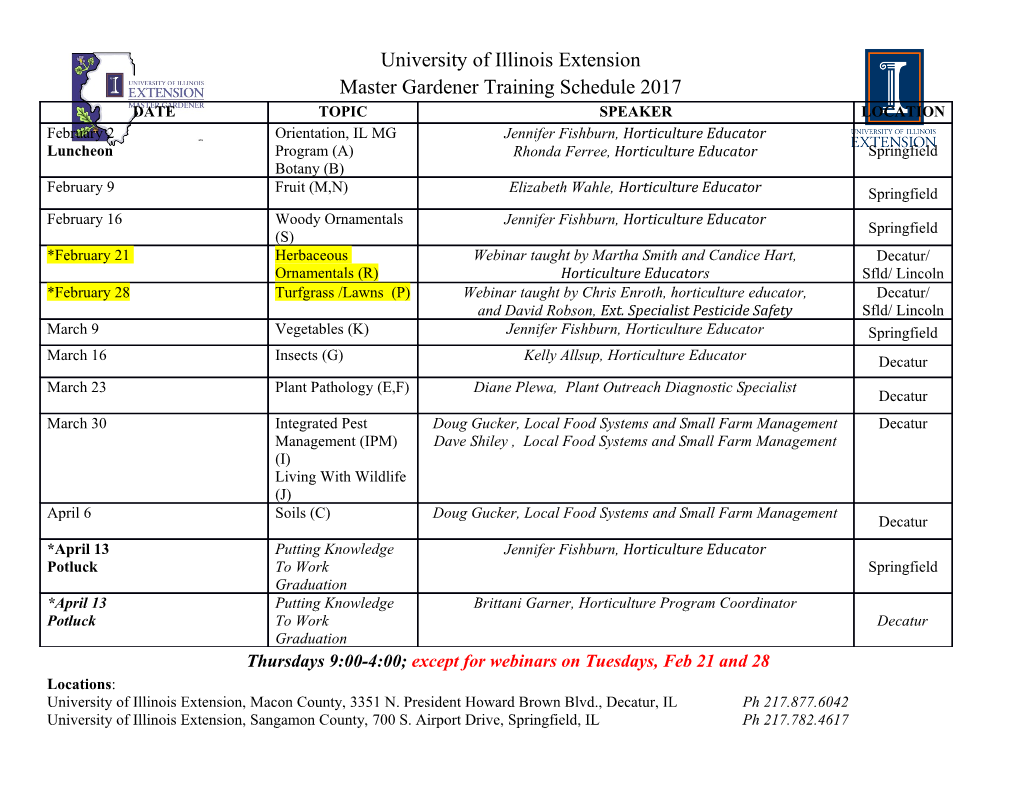
<p>import java.awt.EventQueue; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import javax.swing.JTextArea; import javax.swing.JButton; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.Iterator; import javax.swing.JTextField; import javax.swing.JScrollPane; import java.awt.Font; import javax.swing.JLabel; import javax.swing.JComboBox; import javax.swing.DefaultComboBoxModel; import javax.swing.JSeparator; public class Colecoes extends JFrame {</p><p>/** * */ private static final long serialVersionUID = -7275882428847114650L; private JPanel contentPane; private JTextArea listagemEntrada = new JTextArea(); private ArrayList<String> lista1 = new ArrayList<String>(); private JTextArea listagemSaida = new JTextArea(); private JButton btnListaEntrada = new JButton("Listar"); private JButton btnListaSaida = new JButton("Esvazia lista"); private JScrollPane scrollPane = new JScrollPane(); private JTextField tfEntrada; private final JButton btnSair = new JButton("Sair"); private final JButton btnSort = new JButton("Sort"); private final JButton btnRotate = new JButton("Rotate"); private final JComboBox rotateIndex = new JComboBox(); private final JButton btnSwap = new JButton("Swap"); private final JComboBox swapPos1 = new JComboBox(); private final JLabel lblCom = new JLabel("com"); private final JComboBox swapPos2 = new JComboBox(); private final JButton btnReplaceAll = new JButton("replaceAll"); private JTextField tfOcorrencia; private JTextField tfNovoValor; private final JButton btnFill = new JButton("Fill"); private final JTextField tfFill = new JTextField(); private final JButton btnBinarySearch = new JButton("Binary Search"); private JTextField tfBinarySearch; private final JLabel label_1 = new JLabel(">>"); private final JTextField tfIndexOfSubList = new JTextField(); private final JLabel lblNewLabel_2 = new JLabel("separar por v\u00EDrgula"); private final JButton btnLastIndexOfSubList = new JButton("LastIndexOfSubList"); private final JTextField tfLastIndexOfSubList = new JTextField(); private final JLabel lblNewLabel_3 = new JLabel("separar por v\u00EDrgula"); private final JButton btnFrequency = new JButton("Frequency"); private final JLabel label_2 = new JLabel(">>"); private final JTextField tfFrequency = new JTextField(); private final JButton btnDisjoint = new JButton("Disjoint"); private final JTextField tfDisjoint = new JTextField(); private final JLabel lblSepararPorVrgula = new JLabel("separar por v\u00EDrgula"); private final JButton btnMin = new JButton("Min"); private final JButton btnMax = new JButton("Max");</p><p>/** * Launch the application. */ public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { try { Colecoes frame = new Colecoes(); frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); } /** * Create the frame. */ public Colecoes() { tfDisjoint.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfDisjoint.setBounds(167, 605, 67, 20); tfDisjoint.setColumns(10); tfFrequency.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfFrequency.setBounds(197, 574, 37, 20); tfFrequency.setColumns(10); tfLastIndexOfSubList.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfLastIndexOfSubList.setBounds(167, 543, 67, 20); tfLastIndexOfSubList.setColumns(10); tfIndexOfSubList.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfIndexOfSubList.setBounds(167, 512, 67, 20); tfIndexOfSubList.setColumns(10); tfFill.setBounds(197, 450, 37, 20); tfFill.setColumns(10); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(300, 10, 507, 738); contentPane = new JPanel(); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(null);</p><p>JButton btnEntraValor = new JButton("+"); btnEntraValor.setFont(new Font("Tahoma", Font.PLAIN, 7)); btnEntraValor.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) {</p><p> listagemEntrada.append(tfEntrada.getText()+"\n"); lista1.add(tfEntrada.getText()); tfEntrada.setText(""); tfEntrada.requestFocusInWindow();</p><p>} }); btnEntraValor.setBounds(111, 83, 46, 23); contentPane.add(btnEntraValor); scrollPane.setBounds(276, 247, 181, 141);</p><p> contentPane.add(scrollPane); scrollPane.setViewportView(listagemSaida); btnListaEntrada.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnListaEntrada.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { listagemSaida.setText(""); for (String str : lista1) { listagemSaida.append(str+"\n"); } } }); btnListaEntrada.setBounds(21, 234, 136, 23); contentPane.add(btnListaEntrada); btnListaSaida.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnListaSaida.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemEntrada.setText(""); listagemSaida.setText(""); Iterator<String> i = lista1.iterator(); for (; i.hasNext(); ) { i.next(); i.remove(); }</p><p>} }); btnListaSaida.setBounds(21, 136, 136, 21); contentPane.add(btnListaSaida); tfEntrada = new JTextField(); tfEntrada.setBounds(21, 82, 80, 23); contentPane.add(tfEntrada); tfEntrada.setColumns(10); btnSair.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnSair.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { dispose(); } }); btnSair.setBounds(416, 666, 65, 23); contentPane.add(btnSair); btnSort.setToolTipText("Ordena\u00E7\u00E3o crescente"); btnSort.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); Collections.sort(lista2); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnSort.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnSort.setBounds(21, 268, 136, 20); contentPane.add(btnSort);</p><p>JLabel lblDigiteUmCaractere = new JLabel("Valor"); lblDigiteUmCaractere.setBounds(21, 54, 37, 17); contentPane.add(lblDigiteUmCaractere);</p><p>JLabel lblNewLabel = new JLabel("Valor(es) entrado(s)"); lblNewLabel.setBounds(276, 11, 118, 14); contentPane.add(lblNewLabel);</p><p>JLabel lblResultado = new JLabel("Resultado"); lblResultado.setBounds(276, 222, 80, 14); contentPane.add(lblResultado);</p><p>JScrollPane scrollPane_1 = new JScrollPane(); scrollPane_1.setBounds(278, 36, 179, 151); contentPane.add(scrollPane_1); scrollPane_1.setViewportView(listagemEntrada); listagemEntrada.setColumns(15); JButton btnShuffle = new JButton("Shuffle"); btnShuffle.setToolTipText("Ordena\u00E7\u00E3o aleat\u00F3ria"); btnShuffle.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); Collections.shuffle(lista2); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnShuffle.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnShuffle.setBounds(21, 298, 136, 20); contentPane.add(btnShuffle); btnRotate.setToolTipText("Desloca os elementos \"n\" posi\u00E7\u00F5es"); btnRotate.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); int ri = Integer.parseInt(rotateIndex.getItemAt(rotateIndex.getSelectedIndex()).toString()); Collections.rotate(lista2,ri); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnRotate.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnRotate.setBounds(21, 357, 87, 20); contentPane.add(btnRotate); rotateIndex.setFont(new Font("Tahoma", Font.PLAIN, 10)); rotateIndex.setModel(new DefaultComboBoxModel(new String[] {"1", "2", "3"})); rotateIndex.setBounds(120, 357, 37, 20); contentPane.add(rotateIndex); btnSwap.setToolTipText("Troca dois elementos de posi\u00E7\u00E3o"); btnSwap.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnSwap.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); int pos1 = Integer.parseInt(swapPos1.getItemAt(swapPos1.getSelectedIndex()).toString()); int pos2 = Integer.parseInt(swapPos2.getItemAt(swapPos2.getSelectedIndex()).toString()); Collections.swap(lista2,pos1,pos2); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnSwap.setBounds(21, 388, 87, 20); contentPane.add(btnSwap); swapPos1.setFont(new Font("Tahoma", Font.PLAIN, 10)); swapPos1.setModel(new DefaultComboBoxModel(new String[] {"0", "1", "2", "3"})); swapPos1.setBounds(120, 388, 37, 20); contentPane.add(swapPos1); lblCom.setFont(new Font("Tahoma", Font.PLAIN, 10)); lblCom.setBounds(167, 388, 39, 20); contentPane.add(lblCom); swapPos2.setFont(new Font("Tahoma", Font.PLAIN, 10)); swapPos2.setModel(new DefaultComboBoxModel(new String[] {"0", "1", "2", "3"})); swapPos2.setBounds(197, 388, 37, 20); contentPane.add(swapPos2);</p><p>JSeparator separator = new JSeparator(); separator.setBounds(21, 209, 436, 2); contentPane.add(separator); JButton btnReverse = new JButton("Reverse"); btnReverse.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnReverse.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); Collections.reverse(lista2); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnReverse.setToolTipText("Ordena\u00E7\u00E3o decrescente"); btnReverse.setBounds(21, 326, 136, 20); contentPane.add(btnReverse); btnReplaceAll.setToolTipText("Altera todas as ocorr\u00EAncias por um valor especificado"); btnReplaceAll.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1 .clone(); Collections.replaceAll(lista2, tfOcorrencia.getText(), tfNovoValor.getText()); listagemSaida.setText(""); for (String str : lista2) { listagemSaida.append(str+"\n"); } }</p><p>}); btnReplaceAll.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnReplaceAll.setBounds(21, 419, 87, 23); contentPane.add(btnReplaceAll); tfOcorrencia = new JTextField(); tfOcorrencia.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfOcorrencia.setBounds(120, 419, 37, 20); contentPane.add(tfOcorrencia); tfOcorrencia.setColumns(10);</p><p>JLabel lblNewLabel_1 = new JLabel("por"); lblNewLabel_1.setFont(new Font("Tahoma", Font.PLAIN, 10)); lblNewLabel_1.setBounds(167, 419, 29, 20); contentPane.add(lblNewLabel_1); tfNovoValor = new JTextField(); tfNovoValor.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfNovoValor.setBounds(197, 419, 37, 20); contentPane.add(tfNovoValor); tfNovoValor.setColumns(10); btnFill.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); ArrayList<String> lista2 = (ArrayList<String>) lista1.clone(); Collections.fill(lista2, tfFill.getText()); for (String str : lista2) { listagemSaida.append(str+"\n"); } } }); btnFill.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnFill.setBounds(21, 450, 136, 20); contentPane.add(btnFill); contentPane.add(tfFill); btnBinarySearch.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); int pos = Collections.binarySearch (lista1, tfBinarySearch.getText()); listagemSaida.setText("Posição = " + pos); } }); btnBinarySearch.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnBinarySearch.setToolTipText("Gera uma lista vazia, por c\u00F3pia da lista original"); btnBinarySearch.setBounds(21, 481, 136, 20); contentPane.add(btnBinarySearch);</p><p>JButton btnIndexOfSubList = new JButton("IndexOfSubList"); btnIndexOfSubList.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { listagemSaida.setText(""); String[] arr = tfIndexOfSubList.getText().split( "," ); ArrayList<String> lista2 = new ArrayList<String>(Arrays.asList( arr )); int pos = Collections.indexOfSubList (lista1, lista2); listagemSaida.setText("Posição = " + pos); } }); btnIndexOfSubList.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnIndexOfSubList.setBounds(21, 512, 136, 20); contentPane.add(btnIndexOfSubList);</p><p>JLabel label = new JLabel(">>"); label.setFont(new Font("Tahoma", Font.PLAIN, 10)); label.setBounds(168, 481, 29, 20); contentPane.add(label); tfBinarySearch = new JTextField(); tfBinarySearch.setFont(new Font("Tahoma", Font.PLAIN, 10)); tfBinarySearch.setBounds(197, 481, 37, 20); contentPane.add(tfBinarySearch); tfBinarySearch.setColumns(10); label_1.setFont(new Font("Tahoma", Font.PLAIN, 10)); label_1.setBounds(168, 450, 29, 20); contentPane.add(label_1); contentPane.add(tfIndexOfSubList); lblNewLabel_2.setFont(new Font("Tahoma", Font.PLAIN, 10)); lblNewLabel_2.setBounds(239, 515, 93, 14); contentPane.add(lblNewLabel_2); btnLastIndexOfSubList.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { listagemSaida.setText(""); String[] arr = tfLastIndexOfSubList.getText().split( "," ); ArrayList<String> lista2 = new ArrayList<String>(Arrays.asList( arr )); int pos = Collections.lastIndexOfSubList (lista1, lista2); listagemSaida.setText("Posição = " + pos); } }); btnLastIndexOfSubList.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnLastIndexOfSubList.setBounds(21, 543, 136, 20); contentPane.add(btnLastIndexOfSubList); contentPane.add(tfLastIndexOfSubList); lblNewLabel_3.setFont(new Font("Tahoma", Font.PLAIN, 10)); lblNewLabel_3.setBounds(239, 543, 93, 14); contentPane.add(lblNewLabel_3); btnFrequency.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnFrequency.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); int f = Collections.frequency (lista1, tfFrequency.getText()); listagemSaida.setText("O elemento " + tfFrequency.getText() + " ocorre " + f + " vez(es)."); } }); btnFrequency.setBounds(21, 574, 136, 20); contentPane.add(btnFrequency); label_2.setFont(new Font("Tahoma", Font.PLAIN, 10)); label_2.setBounds(168, 574, 29, 20); contentPane.add(label_2); contentPane.add(tfFrequency); btnDisjoint.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); String[] arr = tfDisjoint.getText().split( "," ); ArrayList<String> lista2 = new ArrayList<String>(Arrays.asList( arr )); String comparacao = Collections.disjoint (lista1, lista2) ? "Não há elementos em comum." : "Há elementos em comum." ; listagemSaida.setText(comparacao); } }); btnDisjoint.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnDisjoint.setBounds(21, 605, 136, 20); contentPane.add(btnDisjoint); contentPane.add(tfDisjoint); lblSepararPorVrgula.setFont(new Font("Tahoma", Font.PLAIN, 10)); lblSepararPorVrgula.setBounds(239, 605, 93, 14); contentPane.add(lblSepararPorVrgula); btnMin.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); String min = Collections.min (lista1); listagemSaida.setText("Menor valor: " + min); } }); btnMin.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnMin.setBounds(21, 636, 136, 20); contentPane.add(btnMin);</p><p> btnMax.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { listagemSaida.setText(""); String max = Collections.max (lista1); listagemSaida.setText("Maior valor: " + max); } }); btnMax.setFont(new Font("Tahoma", Font.PLAIN, 10)); btnMax.setBounds(21, 667, 136, 20);</p><p> contentPane.add(btnMax); } }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-