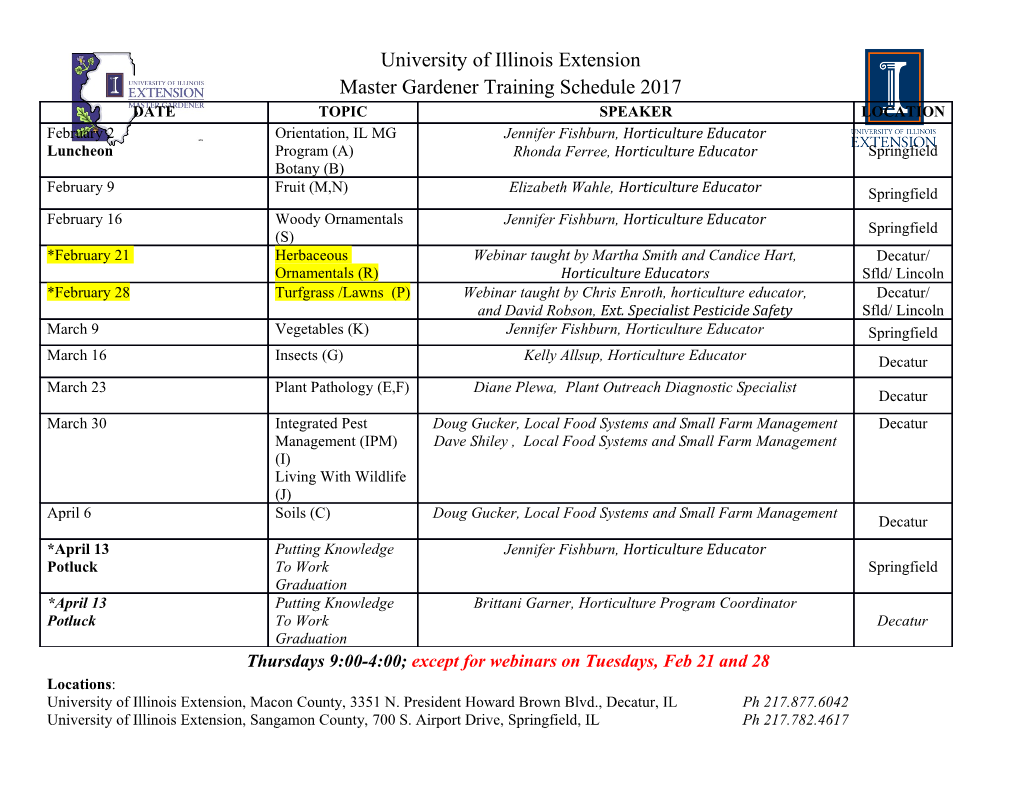
<p>Name______Spring 2013 Sample Exam 3 CompSci117 Each question is worth 5 points unless otherwise noted.</p><p>____1. When an argument is ______it is protected from accidental change in the function. a. overloaded b. pass-by-reference c. pass-by-value d. Global</p><p>______2. The return statement in C++: A. Returns a value to the statement that called the function. B. Is used to stop execution of a function. C. Returns just one value. D. All of the above.</p><p>____3. A local variable is: a. Declared inside brackets{}. b. A private data member. c. Illegal. d. Declared outside any block.</p><p>____4. In the function call pow(2.0, 3.0), 2 and 3 are examples of: A. Subscripts. B. Operators. C. Parameters. D. Arguments.</p><p>____5. An advantage of using functions is: A. They help make decisions. B. They are used to store data. C. They break the program into manageable parts. D. They allow for input from files.</p><p>6. Do all functions need a return statement? Explain.</p><p>7. In the program below circle and label an example of each of the following. (5 points each)</p><p>A function call.</p><p>1 A parameter. A function definition. #include<iostream> using namespace std; double area(double l, double w) { return l * w; } int main() { double length = 3.5, width = 1.5; cout << " rectangle is " << area(length, width)<< " sq.in.\n"; return 0; }</p><p>8.Show the output of this program. #include <iostream> using namespace std; double findPerimeter(double a, double b, double c){ return a + b + c; } int main() { double side_a = 5.0, side_b = 6.0, side_c = 7.0, perimeter; perimeter = findPerimeter(side_a, side_b, side_c); cout << perimeter << " inches\n"; return 0; }</p><p>2 9.What is the output of this program? #include <iostream> using namespace std; void reduceFraction(int& num, int& denom) { if(num % 2 == 0 && denom % 2 == 0) { denom = denom/2; num = num/2; } } int main() { int numerator = 8, denominator = -10; reduceFraction(numerator, denominator); cout << numerator << "/" << denominator; return 0; }</p><p>10. Show the output. #include <iostream> using namespace std; void tick(int &m, int n) { m ++; n ++; return; } int main() { int q = 2, p = 3; cout << p << " and " << q << endl; tick(p,q); cout << "now " << p << " and "<< q << endl; return 0; }</p><p>11. Correct the error that causes the message when a compile is attempted.</p><p>3 1 #include <iostream> 2 using namespace std; 3 4 double oneMore(double num) { 5 x = num + 1.0; 6 return x; 7 8 } 9 int main() { 10 double x = 15.5; 11 cout << oneMore(x)<<endl; 12 return 0; 13 } 14 [jjarboe@onyx errors]$ g++ onemore.cpp onemore.cpp: In function ‘double oneMore(double)’: onemore.cpp:5:4: error: ‘x’ was not declared in this scope</p><p>12. This program finds the area of a circle, using the radius input by the user. The output shows a incorrect value echoed for the radius. Correct the parameter list in the function to protect the radius from this change. //Finds the area of a circle. #include <iostream> #include <cmath> using namespace std; const double PI = 3.14159; void circleArea(double& r, double& a, double& p) { p = 2.0*PI*r; r = r*r; a = PI * r; } int main() { double radius, area, perimeter; cout << "Enter radius:"; cin >> radius;</p><p> circleArea(radius, area, perimeter); //Function Call</p><p> cout << "Circle with radius " << radius << " has area " << area << " and perimeter " << perimeter << endl; return 0; }</p><p>Output: (User input is underlined.) Enter radius:3.0 Circle with radius 9 has area 28.2743 and perimeter 18.8495</p><p>4 13. In the program be below tell what the scope of each of the following names is: (10 points) a. TODEGREES</p><p> b. dist</p><p>#include <iostream> #include <cmath> using namespace std; const double TODEGREES = 180.0/3.14159; void polar(double x, double y, double& r, double& theta); int main() { double rect_x = 2.0, rect_y = 2.0; double dist, angle; polar(rect_x, rect_y, dist, angle); cout << "r = " << dist << endl; cout << "angle = " << angle << endl; return 0; } void polar(double x, double y, double& r, double& theta) { r = sqrt(x*x + y*y); theta = atan(y/x) * TODEGREES; }</p><p>14. Write a function that takes a movie time in minutes, e.g. 125, and relays to the calling program the time in hours and minutes, 2 & 5. Make appropriate use of pass-by-reference and pass-by-value. (10 points)</p><p>5</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-