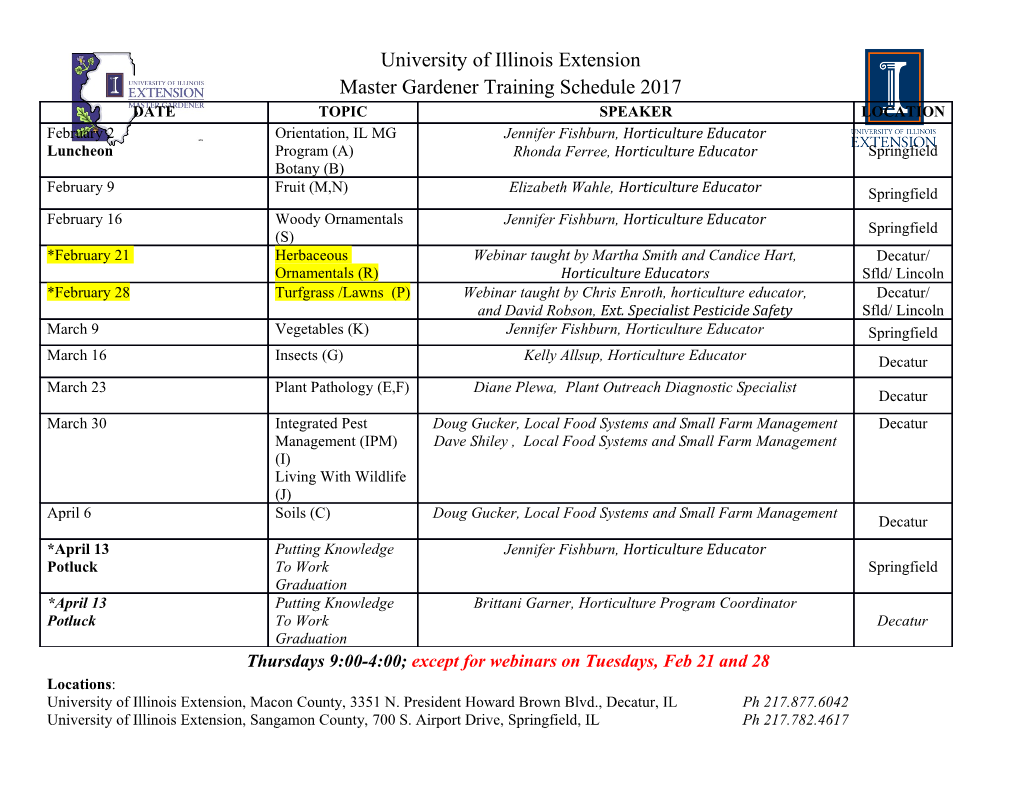
<p>COP 3503 Fall 2012 Exam 2 Sample Questions</p><p>1. Write a method called hasInteger which takes in a Queue<Integer> and an Integer. It returns true or false depending on whether the queue contains that Integer or not. The Queue should remain unchanged after the method is called.</p><p>NOTE: You only have access to the methods enqueue, dequeue and peek. And you can only use Queues, not any other data structures.</p><p>Solution: public boolean hasInteger(Queue<Integer> queue, Integer num) { // temporary Queue Queue<Integer> temp = new Queue<Integer>();</p><p> boolean check = false; while (queue.peek() != null) { Integer n = queue.dequeue(); if (n == num) check = true;</p><p> temp.enqueue(n); }</p><p>// now the temporary queue holds all the values queue = temp;</p><p> return check; } 2. Implement the method void size() for the Linked List class SLL<T>. The method should return the size of the linked list.</p><p>NOTE: You do not have access to any of the other methods inside SLL<T></p><p>Solution:</p><p>Look at lecture notes on Data Structures.</p><p>3. Explain in your own words the concept and process behind the binary search algorithm. When would you use this algorithm? 4. Implement the method void bubbleSort(int[] numbers), which will sort the input array in ascending order using the Bubble Sort algorithm.</p><p>Solution:</p><p>Look at lecture notes on Bubble Sort</p><p>Use these classes for the following question: class GeometricObject { public String color;</p><p> public GeometricObject(String color); } class Circle extends GeometricObject { public double radius;</p><p> public Circle(String color, double radius); } class Rectangle extends GeometricObject { public double width, height;</p><p> public Rectangle(String color, double width, double height); }</p><p>Use the following file format for storing GeometricObjects:</p><p>For Circles: Circle,Color: (color),Radius: (radius) For Rectangles: Rectangle,Color: (color),Width: (width),Height: (height)</p><p>Example File:</p><p>Circle,Color: Red,Radius: 2.5 Rectangle,Color: Black,Width: 10.0,Height: 20.0 Rectangle,Color: Blue,Width: 5.0,Height: 12.5 5. Implement the method public ArrayList<GeometricObject> readObjects(String filename). The method should read from the filename passed in and return a GeometricObject ArrayList with all the GeometricObjects from the file in it. The file format is as described in the previous page. (Make sure you capture all errors) public ArrayList<GeometricObject> readObjects(String filename) { File f = new File(filename);</p><p>ArrayList<GeometricObject> al = new ArrayList<GeometricObject>();</p><p> try { Scanner s = new Scanner(f); while (s.hasNextLine()) { Scanner s2 = new Scanner(s.nextLine(), “,”);</p><p>// type String type = s2.next();</p><p>// color Scanner s3 = new Scanner(s2.next()); s3.next(); String color = s3.next();</p><p>// double1 s3 = new Scanner(s2.next()); s3.next(); double num1 = s3.nextDouble();</p><p>GeometricObject go = null; if (type.equals(“Circle”)) { go = new Circle(color, num1); } else { s3 = new Scanner(s2.next()); s3.next(); double height = s3.nextDouble(); go = new Rectangle(color, num1, height); } al.add(go); } } catch (IOException e) { System.out.println(“Some error reading file”); }</p><p> return al; }</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-