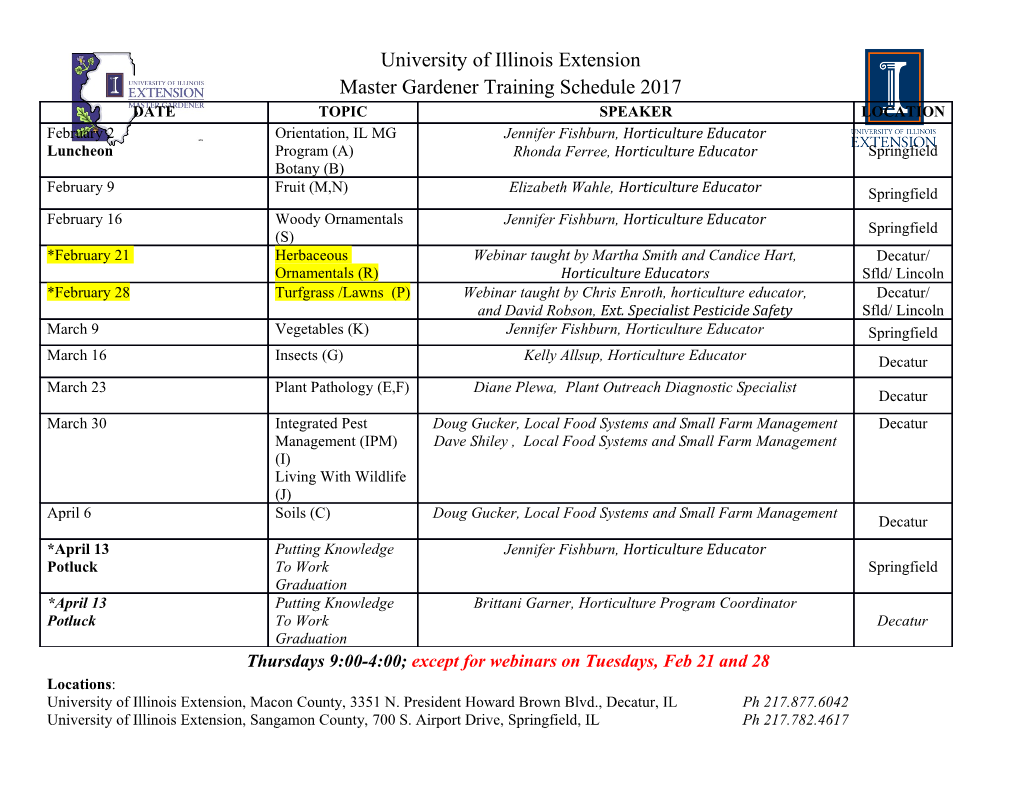
TESSELLATION SHADER DETAIL IMPLEMENTATION FOR SIMPLE SURFACES USING OPENGL A Project Presented to the faculty of the Department of Computer Science California State University, Sacramento Submitted in partial satisfaction of the requirements for the degree of MASTER OF SCIENCE in Computer Science by Matthew Thomas Anderson SPRING 2020 © 2020 Matthew Thomas Anderson ALL RIGHTS RESERVED ii TESSELLATION SHADER DETAIL IMPLEMENTATION FOR SIMPLE SURFACES USING OPENGL A Project by Matthew Thomas Anderson Approved by: __________________________________, Committee Chair Dr. V. Scott Gordon __________________________________, Second Reader Dr. Pinar Muyan-Ozcelik ____________________________ Date iii Student: Matthew Thomas Anderson I certify that this student has met the requirements for format contained in the University format manual, and this project is suitable for electronic submission to the library and credit is to be awarded for the project. __________________________, Graduate Coordinator ___________________ Dr. Jinsong Ouyang Date Department of Computer Science iv Abstract of TESSELLATION SHADER DETAIL IMPLEMENTATION FOR SIMPLE SURFACES USING OPENGL by Matthew Thomas Anderson Shader-based graphics programming can be utilized to create a standard, re-usable method of applying surface detail to a simple shape using tessellation. There is a need for instructional resources outlining how this is accomplished for arbitrary graphics models. The tessellation evaluation shader handles tasks such as vertex position modifications and perspective matrix processing. This implementation also demonstrates how to calculate texture coordinates for the vertices that are generated by the tessellator. The models used are a pyramid with distinct flat surfaces, and a sphere with a continuous curved surface. The benefit of additional surface detail is demonstrated by applying a height map to the models during the tessellation stage. With comparable polygon counts, the RAM usage and GPU usage are both lower with tessellation compared to without tessellation for dense models. The strength of this implementation is that it may be applied universally to pyramids and spheres to add additional surface detail through tessellation. This implementation can also be expanded by applying more performance enhancing tessellation techniques or realistic effects such as noise functions. __________________________________, Committee Chair Dr. V. Scott Gordon ____________________________ Date v ACKNOWLEDGEMENTS I would like to thank Dr. Scott Gordon and Dr. Pinar Muyan-Ozcelik for their time and support for this project. When I returned to CSU, Sacramento for my graduate career, Dr. Muyan-Ozcelik suggested I ask Dr. Gordon to be my faculty advisor for my project. I am very thankful to Dr. Gordon for agreeing to this role and for giving me the idea for this project. His authored book on computer graphics programming has been an invaluable resource in completing this project. vi TABLE OF CONTENTS Page Acknowledgements ................................................................................................................... vi List of Tables ............................................................................................................................ ix List of Figures ............................................................................................................................ x Chapters 1. INTRODUCTION ............................................................................................................. 1 2. BACKGROUND ............................................................................................................... 3 2.1 Graphics pipeline ...................................................................................................... 3 2.2 OpenGL..................................................................................................................... 3 2.3 Models ...................................................................................................................... 4 2.4 Texture mapping ....................................................................................................... 4 2.5 Height mapping ......................................................................................................... 5 2.6 Tessellation ............................................................................................................... 6 3. IMPLEMENTATION ....................................................................................................... 8 3.1 Graphics program overview ...................................................................................... 8 3.2 Defining vertices, texture coordinates, and normal vectors ...................................... 9 3.3 Tessellated vertex positioning and texture mapping ............................................... 11 3.4 Tessellated height mapping ..................................................................................... 14 3.5 Differences between pyramid and sphere implementations .................................... 15 4. RESULTS ....................................................................................................................... 17 4.1 Rendered results ...................................................................................................... 17 4.2 System performance ................................................................................................ 26 5. CONCLUSIONS ............................................................................................................. 29 vii 6. FUTURE WORK ............................................................................................................ 31 Appendix A. Source Code ....................................................................................................... 33 src/code/Code.java – JOGL program ............................................................................ 33 src/code/GLSLOptions.java – Holds runtime variables ................................................ 40 src/code/Camera.java – Simple move/rotate camera implementation .......................... 42 src/code/Mouse.java – Simple click and drag mouse implementation ......................... 43 src/code/Utils.java – Helper class for JOGL calls ........................................................ 44 src/code/shaders/generic_vertShader.glsl – Vertex shader for pyramid and sphere ..... 51 src/code/shaders/pyramid_tessCShader.glsl – Pyramid tessellation control shader ..... 52 src/code/shaders/pyramid_tessEShader.glsl – Pyramid tessellation evaluation shader 53 src/code/shaders/sphere_tessCShader.glsl – Sphere tessellation control shader .......... 54 src/code/shaders/sphere_tessEShader.glsl – Sphere tessellation evaluation shader ..... 55 src/code/shaders/generic_fragShader.glsl – Fragment shader for pyramid and sphere 56 src/models/Pyramid.java – Pyramid model .................................................................. 57 src/models/Sphere.java – Sphere model ....................................................................... 59 src/eventcommands/cmdMoveCamera.java – Move the camera .................................. 62 src/eventcommands/cmdRotateCamera.java – Rotate the camera ................................ 63 src/eventcommands/cmdCloseWindow.java – Close the window ................................ 64 src/eventcommands/cmdSwitchModel.java – Toggle pyramid or sphere model ......... 65 src/eventcommands/cmdCycleTexture.java – Cycle through texture list ..................... 66 src/eventcommands/cmdToggleDrawMode.java – Toggle polygon mode ................... 67 Appendix B. System Specifications ......................................................................................... 68 References ................................................................................................................................ 69 viii LIST OF TABLES Tables Page 1. Performance results with wireframe rendering and no animation ................................. 27 2. Performance results with wireframe rendering and constant Y-axis rotation ................ 27 3. Performance results with painted rendering and no animation ...................................... 28 4. Performance results with painted rendering and constant Y-axis rotation .................... 28 ix LIST OF FIGURES Figures Page 1. Pyramid vertices definition .............................................................................................. 9 2. Pyramid normal vectors calculations ............................................................................. 10 3. Sphere vertices definition .............................................................................................. 10 4. Tessellation level definition ........................................................................................... 11 5. Calculate tessellated vertex position .............................................................................. 12 6. Calculate tessellated texture coordinate ......................................................................... 12 7. Renders of: Pyramid without tessellation (top-left), Pyramid with tessellation (top-right), Sphere without tessellation (bottom-left), Sphere with tessellation (bottom-right) ....... 13 8. Calculate tessellated normal vectors .............................................................................. 14 9. Exploded pyramid .........................................................................................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages80 Page
-
File Size-