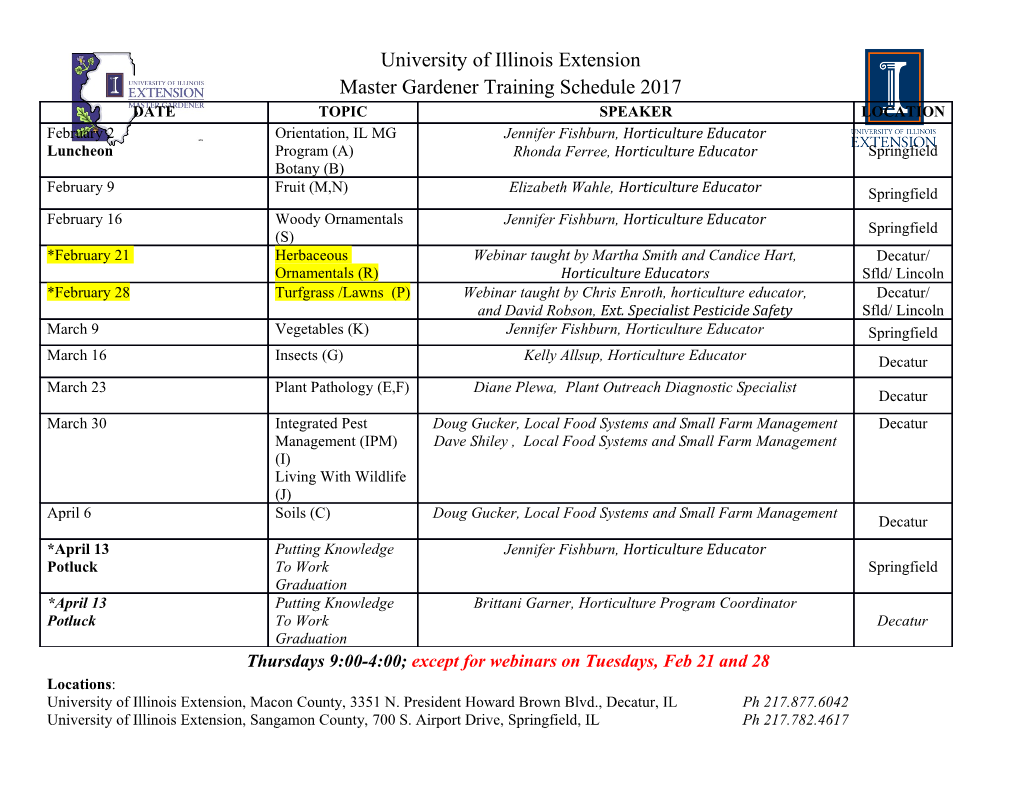
Linked Lists Section 4.3 1 Sequential vs. Linked Allocation Sequential allocation: Put items one after another. • Java: array of objects. Linked allocation: Include in each object a link to the next one. • Java: link is reference to next item. addr value addr value B0 "Alice" C0 "Carol" B1 "Bob" C1 null B2 "Carol" C2 - th Key distinctions: get i item B3 - C3 - Array: random access, fixed size. B4 - C4 "Alice" • B5 - C5 CA Linked list: sequential access, variable size. B6 - C6 - • B7 - C7 - B8 - C8 - get next item B9 - C9 - BA - CA "Bob" BB - CB C0 array linked list (B0) (C4) 2 Singly-Linked Data Structures From the point of view of a particular object: all of these structures look the same! sequential (this lecture) circular parent-link tree rho general case Multiply-linked data structures: Many more possibilities. Section 4.3 3 Linked Lists Linked list: • A recursive data structure. • An item plus a pointer to another linked list (or empty list). – Unwind recursion: linked list is a sequence of items. public class Node { Node data type: public String item; public Node next; • A reference to a String. } • A reference to another Node. first Alice Bob Carol null item next special pointer value null terminates list 4 Building a Linked List addr Value Node third = new Node(); third.item = "Carol"; C0 "Carol" third.next = null; C1 null Node second = new Node(); C2 - second.item = "Bob"; C3 - second.next = third; first C4 C4 "Alice" Node first = new Node(); first.item = "Alice"; second CA C5 CA first.next = second; third C0 C6 - C7 - C8 - C9 - CA "Bob" first second third CB C0 CC - Alice Bob Carol null CD - item next CE - CF - main memory 5 Stack API Section 4.3 6 Stack Push: Linked List Implementation first best the was it first second best the was it Node second = first; first second best the was it first = new Node(); first second first.item = "of"; of best the was it first.next = second; Section 4.3 7 Stack Pop: Linked List Implementation first "of" of best the was it String item = first.item; first of best the was it first = first.next; garbage-collected first best the was it return item; Section 4.3 8 Stack: Linked List Implementation public class LinkedStackOfStrings { private Node first = null; private class Node { private String item; private Node next; } "inner class" public boolean isEmpty() { return first == null; } public void push(String item) { Node second = first; first = new Node(); first.item = item; first.next = second; stack and linked list contents } after 4th push operation public String pop() { String item = first.item; first = first.next; return item; } } 9 Linked List Stack: Test Client Trace push pop Section 4.3 10 Stack Data Structures: Tradeoffs Two data structures to implement Stack data type. Array: • Every push/pop operation take constant time. • But… must fix maximum capacity of stack ahead of time. Linked list: • Every push/pop operation takes constant time. • Memory is proportional to number of items on stack. • But… uses extra space and time to deal with references. a[] to be or not 0 1 2 3 4 5 6 7 8 9 N 11 List Processing Challenge 1 What does the following code fragment do? for (Node x = first; x != null; x = x.next) { System.out.println(x.item); } first Alice Bob Carol null item next Section 4.3 12 List Processing Challenge 2 What does the following code fragment do? Node last = new Node(); last.item = args[0]; last.next = null; Node first = last; for (int i = 1; i < args.length; i++) { last.next = new Node(); last = last.next; last.item = args[i]; last.next = null; } last first Alice Bob Carol null item next Section 4.3 13 .
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages13 Page
-
File Size-