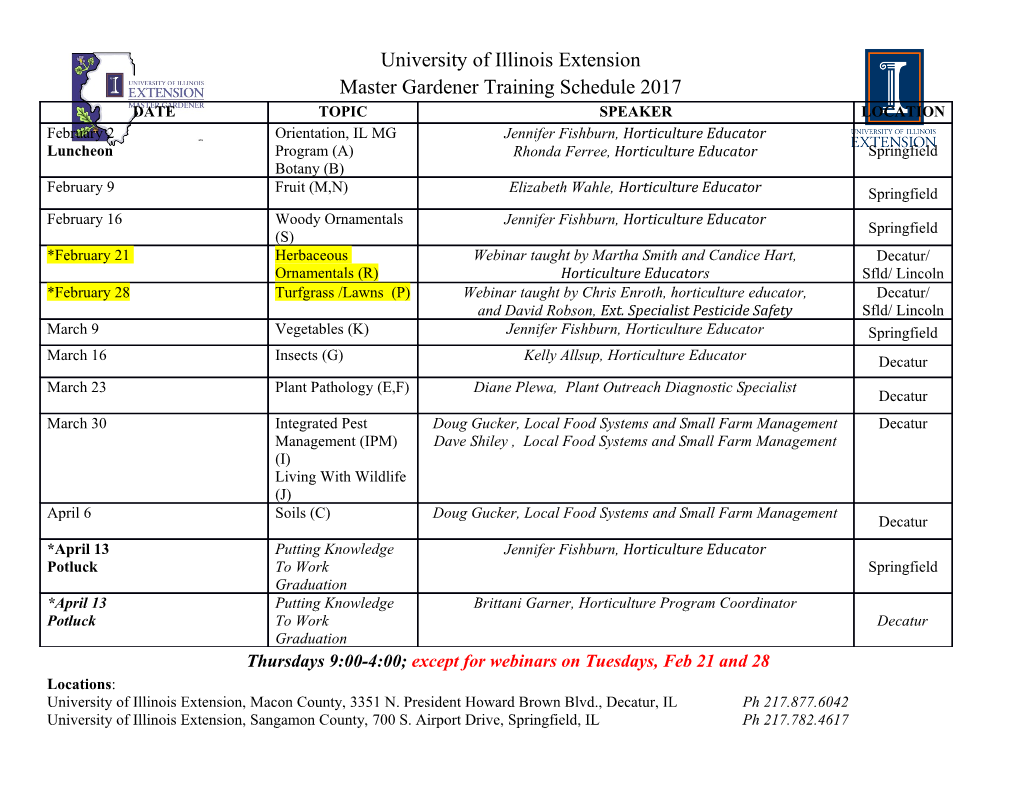
PYTHON PROGRAMMING UNIT-V NAMESPACES Encapsulation in Functions Encapsulation is the packing of data and functions operating on that data into a single component and restricting the access to some of the object’s components. Encapsulation means that the internal representation of an object is generally hidden from view outside of the object’s definition. A class is an example of encapsulation as it encapsulates all the data that is member functions, variables. I. Difference between Abstraction and Encapsulation Abstraction is a mechanism which represents the essential features without including implementation. Encapsulation — Information hiding. Abstraction — Implementation hiding. Private members: Using OOP in Python, we can restrict access to methods and variables. This prevents data from direct modification which is called encapsulation. In Python, we denote private attribute using underscore as prefix i.e single “ _ “ or double “ __“.. Example of accessing private member data class Person: def __init__(self): self.name = 'Manjula' self.__lastname = 'Dube' def PrintName(self): return self.name +' ' + self.__lastname #Outside class P = Person()print(P.name) print(P.PrintName()) print(P.__lastname) #AttributeError: 'Person' object has no attribute '__lastname' II. Code Reuse and Modularity A function is a block of code which is used to perform some action, and it is also called as reusable code. A function provides higher modularity and code re-usability. As Python is an interpreted language, it follows a top-down approach. The most important factor in any programming language is the ‘modules’. The module is a program that can be included or imported to the other programs so that it can be reused in the future without writing the same module again. Python that helps us to invoke the functions automatically by operating the system during run-time or when the program is executed, and this is what we call as the main function. Example : 1 print(“Good Morning”) 2 3 def main(): 4 print(“Hello Python”) 5 6 print(“Good Evening”) 1 Output: Good Morning Good Evening If we observe the above program, it has printed only ‘Good Morning’ and ‘Good Evening’ and it did not print the term ‘Hello Python’ which is because we didn’t call it manually or we didn’t use the python’s main function here. Modularity Modularity refers to the act of partitioning the code into modules building them first followed by linking and finally combining them to form a complete project. Modularity ensures re-usability and minimize the duplication. A module in Python is nothing but a file containing Python definitions followed by methods & statements. The module name is generated out of the file name by removing the suffix “.py”. For example, if the file name is fibonacci.py, the module name is fibonacci. Let's create a module. We save the following code in the file fibonacci.py: def fib(n): if n == 0: return 0 elif n == 1: return 1 else: return fib(n-1) + fib(n-2) def ifib(n): a, b = 0, 1 for i in range(n): a, b = b, a + b return a We can import this module in the interactive python shell and call the functions by prefixing them with "fibonacci.": >>> import fibonacci >>> fibonacci.fib(30) 832040 NAMESPACES A name in Python is a way to access a variable like in any other languages. Python is more flexible when it comes to the variable declaration. We can declare a variable by assigning a name to it. We can use names to reference values. Example: num = 5 str = 'Z' seq = [0, 1, 1, 2, 3, 5] #We can even assign a name to a function. def function(): print('It is a function.') foo = function foo() A namespace is a simple system to control the names in a program. A namespace is a dictionary of variable names (keys) and their corresponding objects (values). Namespaces play a key role in the execution of function calls and the normal control flow of a program. Python implements namespaces in the form of 2 dictionaries. It maintains a name-to-object mapping where names act as keys and the objects as values. Multiple namespaces may have the same name but pointing to a different variable. Types of Namespaces 1. Local Namespace Creation of local functions creates the local namespace. This namespace covers the local names inside a function. Python creates this namespace for every function called in a program. It remains active until the function returns. 2. Global Namespace When a user creates a module, a global namespace gets created. This namespace covers the names from various imported modules used in a project. Python creates this namespace for every module included in your program. It’ll last until the program ends. 3. Built-in Namespace This namespace covers the built-in functions and built-in exception names. Python creates it as the interpreter starts and keeps it until you exit. The built-in namespace encompasses global namespace and global namespace encompasses local namespace. Some functions like print(), id() are examples of built in namespaces. Lifetime of a namespace: A lifetime of a namespace depends upon the scope of objects, if the scope of an object ends, the lifetime of that namespace comes to an end. It is not possible to access inner namespace’s objects from an outer namespace. Scopes A scope refers to a region of a program where a namespace can be directly accessed, i.e. without using a namespace prefix. The scope of a name is the area of a program where this name can be unambiguously used, for example, inside of a function. A name's namespace is identical to its scope. Scopes are defined statically, but they are used dynamically. During program execution there are the following nested scopes available: the innermost scope is searched first and it contains the local names the scopes of any enclosing functions, which are searched starting with the nearest enclosing scope the next-to-last scope contains the current module's global names the outermost scope, which is searched last, is the namespace containing the built-in names. 3 Example: # var1 is in the global namespace var1 = 5 def some_func(): # var2 is in the local namespace var2 = 6 def some_inner_func(): # var3 is in the nested local # namespace var3 = 7 Modules as Namespaces Module is a file consisting of Python code. A module can define functions, classes and variables. When the module is executed (imported), and then the module is (also) a namespace. This namespace has a name, which is the name of the module. In this namespace, the names that are defined in the global scope of the module: the names of functions, values, and classes defined in the module. These names are all referred to as the module’s attributes. 1. Module Attributes To get access to all the functions in the Standard Library module math, we import the module: >>> import math Once a module is imported, the Python built-in function dir() can be used to view all the module’s attributes. dir() is a powerful inbuilt function in Python3, which returns list of the attributes and methods of any object (say functions , modules, strings, lists, dictionaries etc.) Syntax : dir({object}) Example: >>> dir(math) ['__doc__', '__file__', '__name__', '__package__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'exp', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'hypot', 'isinf', 'isnan', 'ldexp', 'log', 'log10', 'log1p', 'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'trunc'] Python - Math Module Some of the most popular mathematical functions are defined in the math module. These include trigonometric functions, representation functions, logarithmic functions, angle conversion functions, etc. Pie (π) is a well-known mathematical constant, which is defined as the ratio of the circumference to the diameter of a circle and its value is 3.141592653589793. >>> import math >>>math.pi 3.141592653589793 Another well-known mathematical constant defined in the math module is e. It is called Euler's number and it is a base of the natural logarithm. Its value is 2.718281828459045. >>>math.e 2.718281828459045 Locating Modules When you import a module, the Python interpreter searches for the module in the following sequences The current directory. If the module is not found, Python then searches each directory in the shell variable PYTHONPATH. If all else fails, Python checks the default path. On UNIX, this default path is normally /usr/local/lib/python3/. 4 The module search path is stored in the system module sys as the sys.path variable. The sys.path variable contains the current directory, PYTHONPATH, and the installation dependent default. 2. Python - Sys Module The sys module provides functions and variables used to manipulate different parts of the Python runtime environment. i) sys.path This is an environment variable that is a search path for all Python modules. >>>sys.path ['', 'C:\\python36\\Lib\\idlelib', 'C:\\python36\\python36.zip', 'C:\\python36\\DLLs', 'C:\\python36\\lib', 'C:\\python36', 'C:\\Users\\acer\\AppData\\Roaming\\Python\\Python36\\site- packages', 'C:\\python36\\lib\\site-packages'] ii) sys.version This attribute displays a string containing the version number of the current Python interpreter. >>>sys.version '3.7.0 (v3.7.0:f59c0932b4, Mar 28 2018, 17:00:18) [MSC v.1900 64 bit (AMD64)]' iii) sys.maxsize Returns the largest integer a variable can take. >>> import sys >>>sys.maxsize 9223372036854775807 iv) sys.exit This causes the script to exit back to either the Python console or the command prompt. This is generally used to safely exit from the program in case of generation of an exception. 3. Top-Level Module A computer application is a program that is typically split across multiple files (i.e., modules).
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages12 Page
-
File Size-