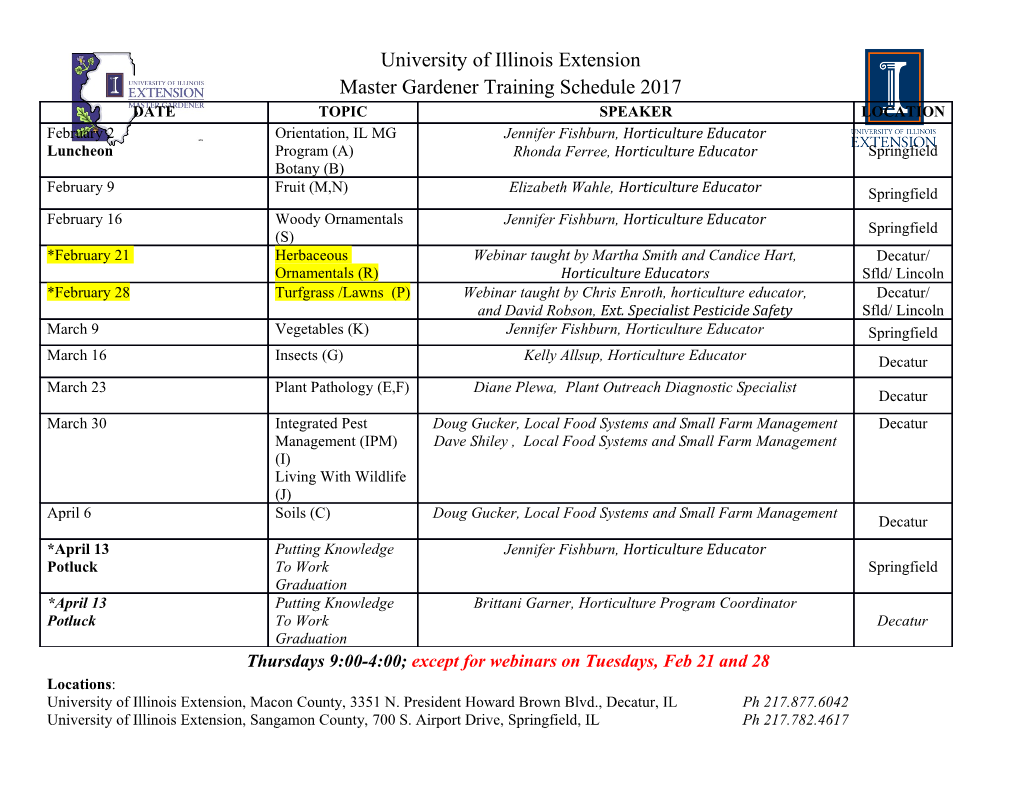
CS 242 Simula 67 • First object‐oriented language • Designed for simulation Simula and Smalltalk – Later recognized as general‐purpose prog language • Extension of Algol 60 John Mitchell • Standardized as Simula (no “67”) in 1977 • Inspiration to many later designers – Smalltalk – C++ – ... Brief history Comparison to Algol 60 • Norwegian Computing Center • Added features – Designers: Dahl, Myhrhaug, Nygaard – class concept – Simula‐1 in 1966 (strictly a simulation language) – reference variables (pointers to objects) – General language ideas – pass‐by‐reference • Influenced by Hoare’s ideas on data types – char, text, I/O • Added classes and prefixing (subtyping) to Algol 60 – coroutines – Nygaard • Removed • Operations Research specialist and political activist • Wanted language to describe social and industrial systems – Changed default par passing from pass‐by‐name • Allow “ordinary people” to understand political (?) changes – some var initialization requirements – Dahl and Myhrhaug – own (=C static) variables • Maintained concern for general programming – string type (in favor of text type) Objects in Simula Example: Circles and lines • Class • Problem – A procedure that returns a pointer to its activation record – Find the center and radius of the circle q passing through three distinct points, p, q, • Object p and r – Activation record produced by call to a class r • Objec t access • Solution – Access any local variable or procedures using dot – Draw intersecting circles Cp, Cq around p,q notation: object. and circles Cq’, Cr around q, r (Picture assumes Cq = Cq’) • Memory management – Draw lines through circle intersections – Objects are garbage collected – The intersection of the lines is the center • user destructors considered undesirable of the desired circle. – Error if the points are colinear. 1 Approach in Simula Simula Point Class • Methodology class Point(x,y); real x,y; formal p is pointer to Point – Represent points, lines, and circles as objects. begin – Equip objects with necessary operations. boolean procedure equals(p); ref(Point) p; • Operations if p =/= none then equals := abs(x ‐ p.x) + abs(y ‐ p.y) < 0.00001 – Point real procedure distance(p); ref(Point) p; equality(anotherPoint) : boolean if p == none then error else distance(anotherPoint) : real (needed to construct circles) distance := sqrt(( x ‐ p.x )**2 + (y ‐ p.y) ** 2); – Line end ***Point*** parallelto(anotherLine) : boolean (to see if lines intersect) meets(anotherLine) : REF(Point) – Circle p :‐ new Point(1.0, 2.5); uninitialized ptr has q :‐ new Point(2.0,3.5); intersects(anotherCircle) : REF(Line) value none if p.distance(q) > 2 then ... pointer assignment Representation of objects Simula line class class Line(a,b,c); real a,b,c; Local variables begin p access link boolean procedure parallelto(l); ref(Line) l; line determined by real x 1.0 code for if l =/= none then parallelto := ... ax+by+c=0 real y 2.5 equals ref(Point) procedure meets(l); ref(Line) l; begin real t; ppqroc equals Procedures if l =/= none and ~lllt(l)~parallelto(l) then ... proc distance code for end; distance real d; d := sqrt(a**2 + b**2); if d = 0.0 then error else begin d := 1/d; a := a*d; b := b*d; c := c*d; Initialization: end; Object is represented by activation record with access link to “normalize” a,b,c end *** Line*** find global variables according to static scoping Derived classes in Simula Subtyping • A class decl may be prefixed by a class name • The type of an object is its class • The type associated with a subclass is treated as a class A subtype of the type assoc with superclass A class B • Example: A class C class A(… ); ... B class D A class B(…); ... ref (A) a :‐ new A(…) • An object of a “prefixed class” is the ref (B) b :‐ new B(…) concatenation of objects of eachA part class in prefix a := b /* legal since B is subclass of A */ – d :‐ new D(…) B part ... d D part b := a /* also legal, but run‐time test */ 2 Main object‐oriented features Features absent from Simula 67 • Classes • Encapsulation • Objects – All data and functions accessible; no private, protected • Inheritance (“class prefixing”) • Self/Super mechanism of Smalltalk • Subtyping – But has an expression this〈class〉 to refer to object itself, regarded as object of type 〈class〉. Not clear how • Virtual methods powerful this is… – A function can be redefined in subclass • Class variables • Inner – But can have global variables – Combines code of superclass with code of subclass • Exceptions • Inspect/Qua – Not fundamentally an OO feature ... – run‐time class/type tests Simula Summary Smalltalk • Class • Major language that popularized objects – ”procedure" that returns ptr to activation record • Developed at Xerox PARC – initialization code always run as procedure body – Smalltalk‐76, Smalltalk‐80 were important versions • Objects: closure created by a class • Object metaphor extended and refined – • Encapsulation UdUsed some ideas from Simu la, but very different lang – Everything is an object, even a class – protected and private not recognized in 1967 – All operations are “messages to objects” – added later and used as basis for C++ – Very flexible and powerful language • Subtyping: determined by class hierarchy • Similar to “everything is a list” in Lisp, but more so • Example: object can detect that it has received a message it • Inheritance: provided by class prefixing does not understand, can try to figure out how to respond. Motivating application: Dynabook Smalltalk language terminology • Concept developed by Alan Kay • Object Instance of some class • Small portable computer • Class Defines behavior of its objects – Revolutionary idea in early 1970’s • At the time, a minicomputer was shared by 10 people, • Selector Name of a message stored in a machine room. • Message SlSelec tor tthtogether with parameter values – What would you compute on an airplane? • Influence on Smalltalk • Method Code used by a class to respond to message – Language intended to be programming language and • Instance variable Data stored in object operating system interface – Intended for “non‐programmer” • Subclass Class defined by giving incremental – Syntax presented by language‐specific editor modifications to some superclass 3 Example: Point class Class messages and methods Three class methods • Class definition written in tabular form Explanation newX:xvalue Y:yvalue | | - selector is mix-fix newX:Y: ^ self new x: xvalue class name Point y: yvalue e.g, Point newX:3 Y:2 super class Object - symbol ^ marks return value class var pi newOrigin | | - new ithdilllis method in all classes, instance var x y ^ self new x: 0 inherited from Object class messages and methods y: 0 - | | marks scope for local decl 〈…names and code for methods...〉 initialize | | - initialize method sets pi, called instance messages and methods pi <‐ 3.14159 automatically 〈…names and code for methods...〉 - <- is syntax for assignment Instance messages and methods Run‐time representation of point Five instance methods Explanation to superclass Object x: xcoord y: ycoord | | set x,y coordinates, Point class x <‐ xcoord e.g, pt x:5 y:3 Template y <‐ ycoord Point object moveDx: dx Dy: dy | | x move poitbint by gi iven amoun t class x <‐ dx + x y y <‐ dy + y x 3 y 2 x | | ^x Method dictionary y | | ^y return hidden inst var x code draw | | return hidden inst var y newX:Y: ... 〈...code to draw point...〉 Detail: class method shown in ... draw point on screen dictionary, but lookup procedure move distinguishes class and instance code methods Inheritance Run‐time representation Point object Point class Template • Define colored points from points Method dictionary x newX:Y: class name ColorPoint 2 y draw ... super class Point 3 move class var new instance instance var color variable ColorPoint class ColorPoint object Template class messages and methods Method dictionary x newX:xv Y:yv C:cv 〈 … code … 〉 new method 4 y newX:Y:C: instance messages and methods color 5 color color | | ^color draw override Point red draw 〈 … code … 〉 method This is a schematic diagram meant to illustrate the main idea. Actual implementations may differ. 4 Encapsulation in Smalltalk Object types • Methods are public • Each object has interface • Instance variables are hidden – Set of instance methods declared in class – Not visible to other objects – Example: • pt x is not allowe d unless x is a metho d Point { x:y:, moveDx:Dy:, x, y, draw} – But may be manipulated by subclass methods ColorPoint { x:y:, moveDx:Dy:, x, y, color, draw} • This limits ability to establish invariants – This is a form of type • Example: Names of methods, does not include type/protocol of – Superclass maintains sorted list of messages with some arguments selector, say insert • Object expression and type – Subclass may access this list directly, rearrange order – Send message to object p draw p x:3 y:4 Subtyping Subtyping and Inheritance • Relation between interfaces • Subtyping is implicit – Suppose expression makes sense – Not a part of the programming language p msg:pars ‐‐ OK if msg is in interface of p – Important aspect of how systems are built – Replace p by q if interface of q contains interface of • IhInher itance is explic it p – Used to implement systems – No forced relationship to subtyping • Subtyping – If interface is superset, then a subtype – Example: ColorPoint subtype of Point – Sometimes called “conformance” Collection Hierarchy Smalltalk Flexibility • Measure of PL expressiveness: Collection isEmpty, size, includes: , … at: – Can constructs of the language be
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-