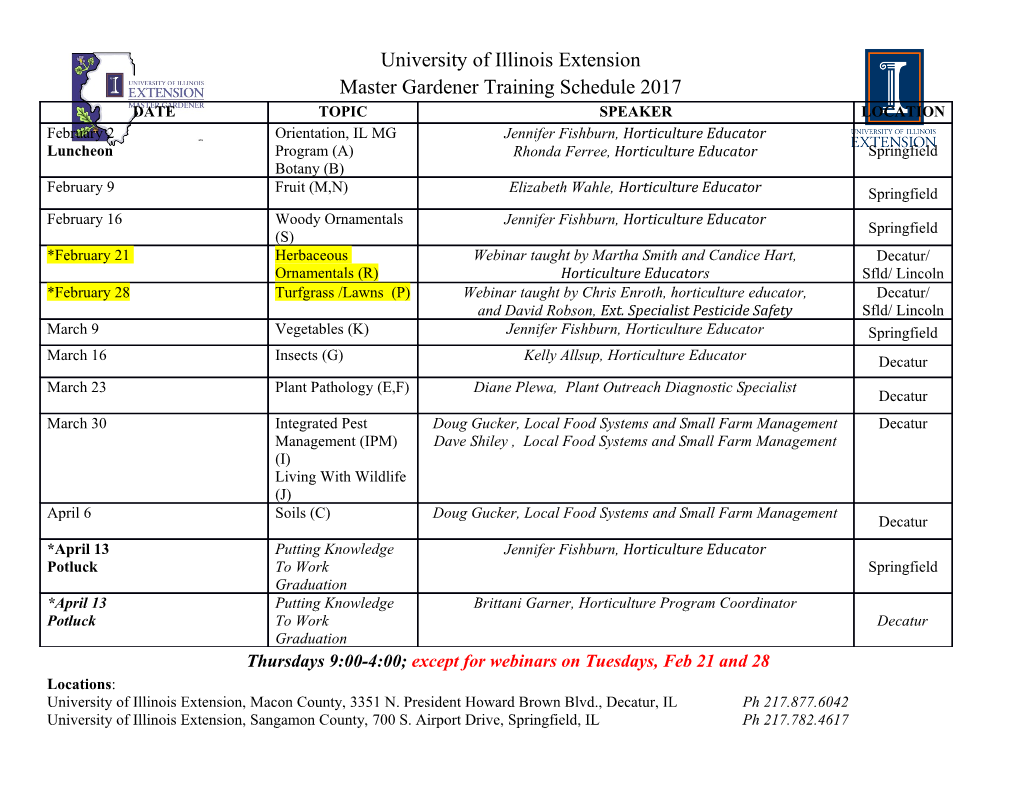
Race Detection for Android Applications Pallavi Maiya Aditya Kanade Rupak Majumdar Indian Institute of Science Indian Institute of Science MPI-SWS [email protected] [email protected] [email protected] Abstract asynchronous tasks to each other. Asynchronous tasks running on the same thread may themselves be reordered non-deterministically Programming environments for smartphones expose a concur- subject to certain rules. While the model can effectively hide laten- rency model that combines multi-threading and asynchronous event- cies, enabling innovative features, programming is complex and pro- based dispatch. While this enables the development of efficient and grams can have many subtle bugs due to non-determinism. feature-rich applications, unforeseen thread interleavings coupled In this paper, we formalize the concurrency semantics of the An- with non-deterministic reorderings of asynchronous tasks can lead droid programming model. Coming up with this formalization re- to subtle concurrency errors in the applications. quired a thorough study of the Android framework and a careful In this paper, we formalize the concurrency semantics of the An- mapping of execution scenarios in Android to more formal execu- happens-before droid programming model. We further define the tion traces. We view an Android application as comprising multi- relation for Android applications, and develop a dynamic race de- ple asynchronous tasks that are executed on one or more threads. tection technique based on this relation. Our relation generalizes An asynchronous task, once started on a thread, runs to completion the so far independently studied happens-before relations for multi- and can make both synchronous and asynchronous procedure calls. threaded programs and single-threaded event-driven programs. Ad- An asynchronous procedure call results in enqueuing of an asyn- ditionally, our race detection technique uses a model of the Android chronous task to the task queue associated with the thread to which it runtime environment to reduce false positives. is posted and control immediately returns to the caller. While the An- We have implemented a tool called DROIDRACER. It generates droid runtime environment creates some threads for the application execution traces by systematically testing Android applications and initially, the application may also spawn threads. A newly spawned detects data races by computing the happens-before relation on thread behaves as a usual thread, but additionally, it can attach a task 15 the traces. We analyzed Android applications including popular queue to itself and receive asynchronous tasks for execution. Often, applications such as Facebook, Twitter and K-9 Mail. Our results an application works with some threads with task queues and others indicate that data races are prevalent in Android applications, and without. In this work, we focus on the semantics of individual ap- that DROIDRACER is an effective tool to identify data races. plications running within their own processes, and omit formalizing Categories and Subject Descriptors D.2.4 [Software Engineer- inter-process communication (IPC) between different applications. ing]: Software/Program Verification; D.2.5 [Software Engineer- Based on the concurrency semantics, we define a happens-before ing]: Testing and Debugging; F.3.1 [Logics and Meanings of Pro- relation over operations in execution traces [15]. A naïve combi- grams]: Specifying and Verifying and Reasoning about Programs nation of rules for asynchronous procedure calls and lock-based syn- chronization introduces spurious happens-before orderings. Specif- General Terms Languages, Reliability, Verification ically, it induces an ordering between two asynchronous tasks run- Keywords Data races, Android concurrency semantics, Happens- ning on the same thread if they use the same lock. This is a spu- before reasoning rious ordering since locks cannot enforce an ordering among tasks running sequentially on the same thread. We overcome this diffi- 1. INTRODUCTION culty by decomposing the relation into (1) a thread-local happens- Touchscreen mobile devices such as smartphones and tablets before relation st which captures the ordering constraints between have become an integral part of our lives. These new devices have asynchronous tasks posted to the same thread and (2) an inter- caught the imagination of the developer community and the end- thread happens-before relation mt which captures the ordering users alike. We are witnessing a significant shift of computing from constraints among multiple threads. These relations are composed desktops to mobile devices. in such a way that the resulting relation captures the happens-before While their hardware is limited in many ways, smartphones have orderings in the Android concurrency model precisely. succeeded in providing programming environments that enable de- We develop a data race detection algorithm based on the happens- velopment of efficient and feature-rich applications. These environ- before relation. A data race occurs if there are two accesses to the ments expose an expressive concurrency model that combines multi- same memory location, with at least one being a write, such that threading and asynchronous event-based dispatch. In this model, there is no happens-before ordering between them. Race detection multiple threads execute concurrently and, in addition, may post for multi-threaded programs is a well-researched topic (e.g., [18, 21, 22, 25, 28]). Recently, race detection for single-threaded event- Permission to make digital or hard copies of all or part of this work for personal or driven programs (also called asynchronous programs) is studied in classroom use is granted without fee provided that copies are not made or distributed the context of client-side web applications (e.g., [20, 24, 30]). Unfor- for profit or commercial advantage and that copies bear this notice and the full citation tunately, race detection for Android applications requires reasoning on the first page. Copyrights for components of this work owned by others than ACM about both thread interleavings and event dispatch; ignoring one or must be honored. Abstracting with credit is permitted. To copy otherwise, or republish, to post on servers or to redistribute to lists, requires prior specific permission and/or a the other leads to false positives. Our happens-before relation gener- fee. Request permissions from [email protected]. alizes these, so far independently studied, happens-before relations PLDI’14, June 9 – June 11, 2014, Edinburgh, United Kingdom. for multi-threaded programs and single-threaded event-driven pro- Copyright c 2014 ACM 978-1-4503-2784-8/14/06. $15.00. grams, enabling precise race detection for Android applications. http://dx.doi.org/10.1145/2594291.2594311 We have implemented our race detection algorithm in a tool 1 public class DwFileAct extends Activity { called DROIDRACER.DROIDRACER provides a framework that 2 boolean isActivityDestroyed = false; generates UI events to systematically test an Android application. 3 It runs unmodified binaries on an instrumented Dalvik VM and in- 4 protected void onResume( ) { 5 super.onResume( ); strumented Android libraries. A run of the application produces an 6 new FileDwTask(this).execute("http://abc/song.mp3"); execution trace, which is analyzed offline for data races by comput- 7 } ing the happens-before relation. The control flow between different 8 public void onPlayClick(View v) { procedures of an Android application is managed to a large extent 9 Intent intent = new Intent(this, MusicPlayActivity.class); 10 intent.putExtra("file", "/sdcard/song.mp3"); by the Android runtime through callbacks. DROIDRACER uses a 11 startActivity(intent); model of the Android runtime environment to reduce false positives 12 } that would be reported otherwise. Further, DROIDRACER assists in 13 protected void onDestroy( ) { debugging the data races by classifying them based on criteria such 14 super.onDestroy( ); 15 isActivityDestroyed = true; as whether one involves multiple threads posting to the same thread 16 } or two co-enabled events executing in an interleaved manner. 17 ... We analyzed 10 open-source Android applications together com- 18 } prising 200K lines of code, and used them to improve the accuracy 19 20 public class FileDwTask extends AsyncTask<String, Integer, Void> { of DROIDRACER. We then applied DROIDRACER on 5 proprietary 21 DwFileAct act; applications including popular and mature applications like Face- 22 ProgressDialog dialog; book and Twitter. Our results indicate that data races are prevalent 23 in Android applications and that DROIDRACER is an effective tool 24 public FileDwTask(DwFileAct act) { 25 this.act = act; to identify data races. Of the 215 races reported by DROIDRACER 26 } on 10 open source Android applications, 80 were verified to be true 27 protected void onPreExecute( ) { positives and 6 of these were found to exhibit bad behaviours. 28 super.onPreExecute( ); In summary, this paper makes the following contributions: 29 dialog = new ProgressDialog(act); 30 ... • The first formulation of Android concurrency semantics. 31 dialog.show( ); • 32 } An encoding of the happens-before relation for Android which 33 protected Void doInBackground(String... params) { generalizes happens-before relations for multi-threaded pro- 34 InputStream input = ... grams and single-threaded event-driven programs. 35 ... • A tool for dynamic race detection augmented with systematic 36 byte data[] = new
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages10 Page
-
File Size-