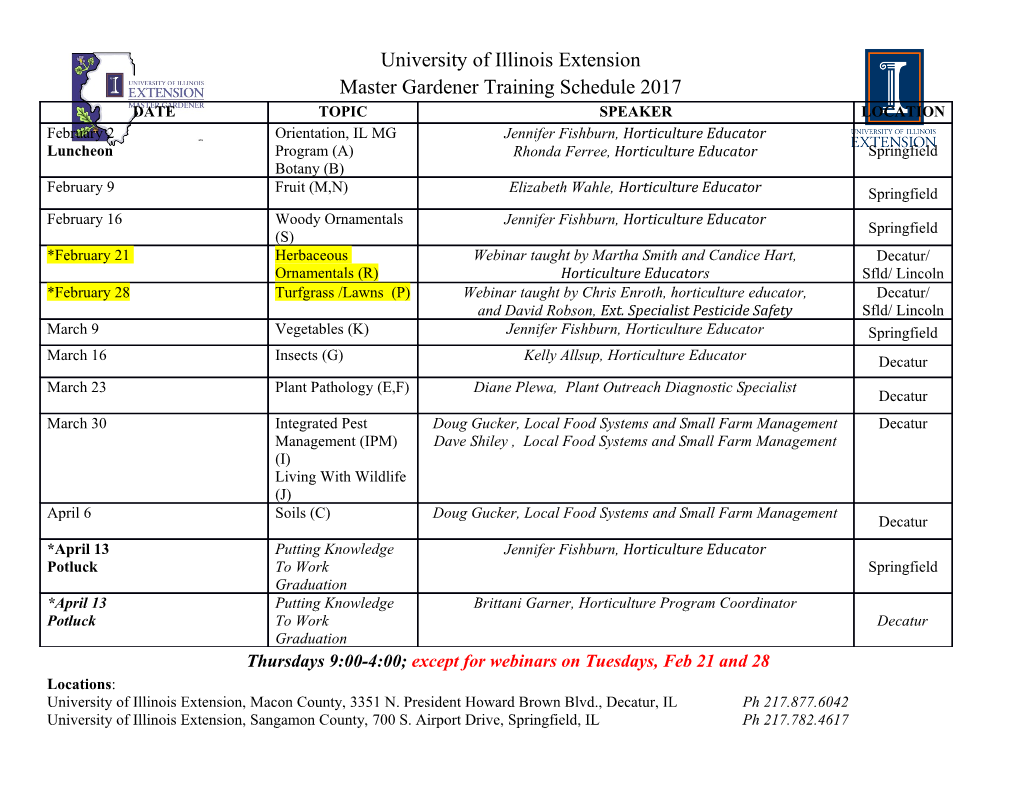
Masaryk University Faculty of Informatics 2D web based game engine Bachelor’s Thesis Martin Petýrek Brno, Spring 2016 Acknowledgement I would like to thank my advisor RNDr. Barbora Kozlíková, Ph.D. for her counsel and the time we spent on this thesis. iii Abstract The goal of this thesis is to create a custom game engine, inspired by features of other engines that are favored by game developers. The result of this thesis should be an easy to use engine which will target simple games and will be easily portable to mobile platforms. It will also feature a website with tutorials on how to start developing, short code samples for individual features of the engine and also complete games. Full API documentation will also be available on the website. iv Keywords game engine, Javascript, Type Script, HTML5. v Contents 1 Introduction ............................1 2 Existing solutions .........................3 2.1 PhaserJS ............................3 2.2 SFML .............................4 2.3 LibGDX ............................5 2.4 Unity3D ............................5 3 mini2d.js Engine .........................7 3.1 Technologies for multi platform web games ..........7 3.1.1 Hybrid applications . .8 3.2 Differences from other solutions ...............8 3.2.1 Mobile first approach . .8 3.2.2 TypeScript . .8 3.2.3 Canvas vs. WebGL . .9 3.3 Performance .......................... 10 3.3.1 SVG . 10 3.3.2 Exporting to mobile platforms . 11 3.3.3 Resolution . 12 3.3.4 Avoiding bad practices . 12 4 Design of mini2d.js ....................... 15 4.1 Classes hierarchy ....................... 15 4.1.1 Game class . 15 4.1.2 Game states . 15 4.1.3 World and UI . 17 4.1.4 Sound . 17 4.1.5 Physics . 17 4.1.6 Resources . 17 4.1.7 Other classes . 17 4.2 Development tools ....................... 18 4.2.1 JavaScript preprocessors . 18 4.2.2 Task runners . 19 5 Implementation .......................... 23 5.1 Game states .......................... 23 5.2 Resource manager ....................... 24 5.3 Input ............................. 24 5.4 Audio ............................. 25 vii 5.5 Camera ............................ 25 5.6 Interfaces ........................... 26 5.6.1 Destroyable . 26 5.6.2 Drawable . 26 5.6.3 Measurable . 26 5.7 UI ............................... 27 5.7.1 Text . 27 5.7.2 Button . 27 5.8 Graphics ............................ 28 5.9 Physics ............................ 28 5.9.1 CollisionEngine . 28 5.9.2 CollisionDetector . 29 5.9.3 CollisionSolver . 29 5.9.4 Body . 29 5.10 Documentation ........................ 30 5.11 JavaDoc ............................ 30 5.12 TypeDoc ............................ 30 6 mini2d.js web page ........................ 31 6.1 Examples ........................... 31 6.2 Tutorials ............................ 31 6.3 Documentation ........................ 33 7 Conclusion ............................. 35 Bibliography . 37 viii 1 Introduction Mobile platforms have grown to the size they are now and have already outgrown other platforms. One could argue that they have pretty much reached the point where nothing significant will ever emerge and therefore the market has become stagnant. There has also been a big shift from traditional devices such as personal computers and laptops. Nowadays more and more people have settled for a mobile device to fulfill their everyday electronic needs. Not only has the market shifted from desktop to mobile devices, it has also been steering towards the web platforms, which despite all their limitations have many advantages. These advantages allow the programmer to write the software once and run it virtually everywhere from a smart phone to a smart TV all the way to the desktop computer. The main difference has always been the performance. However, we are now getting to the state where the performance is sufficient for simple games and therefore HTML51 has become a viable alternative to the classical approach of C/C++ powered engines. And that is the kind of games we will focus on in this thesis. The types of games, that do not require the heavyweight solutions already on the market, crafted to suit the needs of every developer, which are impossible to be used by someone without a prior experi- ence. We are going to focus on simple games, that would not benefit from using an engine under normal circumstances. However, the web is well known for its different behavior on different browsers and platforms. The aim is to write an engine, that will take care of all the inconveniences tied with building mobile games on the web platform and focus on actually developing and designing the game, not dealing with problems related to portability. The goal is to create an engine everyone can use with ease. For those aspiring to build more complex games, there are already very sophisticated and well documented engines with big commu- nities (Unity3D is a great example of such an engine, more on these 1. HTML5 – markup language for the web, fifth and current standard bringing key enhancements to canvas element used for 2D games. 1 1. Introduction later). However, building such a complex system is not the goal of this thesis. This engine will be called mini2d.js and is intended to be stripped of all the unnecessary features which many of these engines offer. This engine aims for portability, consistency, and simplicity. It will feature well described examples showcasing every feature of the engine both separately and combined to create a fully functional mobile first game. It will also offer tutorials for those developers who are in need ofmore in depth explanations. The first part of this work discusses the existing solutions ofHTML5 based game engines and describes the basic techniques behind these, the differences, advantages and disadvantages. The third chapter describes my own implementation and the key enhancements and differences from the existing solutions. It explains the reasoning behind the choices that were made during the process of making this engine. The fourth chapter explains the design of the mini2d.js engine and the development tools chosen for the project. The fifth chapter explains the implementation in detail and demonstrates some of the code required for creating a game in mini2d.js engine. The last part demonstrates the web presentation of the engine, which is an equally important part of this thesis. 2 2 Existing solutions In this chapter we introduce the existing game engines. We then ex- plain their strengths and weaknesses. Some aspects of these solutions have been an inspiration for my work. It was my goal to capture the best concepts of these solutions and combine them into a simple en- gine. The other solutions often possess a feature set that far exceeds what it is required by a developer to do. This could potentially mean using ten megabytes big engine for the game like tic tac toe. Using no engine at all would help to minimize the size of the game, however, it significantly reduces the speed of the development. The existing solu- tions also come with inconveniences specific to those engines when used for the development, so it was also my goal to avoid these and only use the best practice of these solutions. Now we are going to focus on the best solutions that exist as of today on the market. 2.1 PhaserJS PhaserJS[1] is one of the most used HTML5 game frameworks pub- lished on GitHub1. It is an open source solution using Pixi.js2 for rendering. It is actively developed and maintained by Photon Storm Limited[2]. It has a great user base and provides many live examples on its website. I believe the examples are a great way to demonstrate what the engine is capable of and because of this, I have decided to create similar examples for my engine as well. The development team behind PhaserJS has released the last stable version 2.4.6 and is work- ing on rebranding. The new name of the library is going to be Lazer, but it is now in an experimental phase and is not recommended to be used for the development. PhaserJS, although not written in Type- Script, contains TypeScript definitions for TypeScript development and provides the programmer with the ability to take full advantage of TypeScript’s type system. 1. GitHub – the most popular open source git repository hosting service. See https://github.com/. 2. Pixi.js – is a 2D webGL renderer with canvas fallback 3 2. Existing solutions However, it does not come without any shortcomings. PhaserJS is by no means bug free and has many great features that may not be required, making the engine heavyweight. It comes with four different physics engines and many of these features, although useful, have not been authored with the mobile first attitude we strive for. It is still, however, a solid choice for any new game to come. 2.2 SFML SFML[3], created by Laurent Gomila, is very different from PhaserJS and has been the second biggest inspiration for my engine. It takes fundamentally different approach as a game engine. It is written in C++ and the rendering is based on OpenGL3 and Direct3D4. PhaserJS is an engine, that tries to do everything for the programmer and does many things behind the scenes. On the other hand, SFML provides the very basic functionality and the programmer is left to implement the game logic by himself. This is the reason for SFML being lightweight, making it potentially very good, but it does have its big drawbacks which will be mentioned. It is not a complete game engine, but merely a multimedia library, used primarily for simple desktop games. As its homepage says: "It provides a simple interface to the various components of your PC..." It takes care of OpenGL/Direct3D API calls and the creation of a window on any supported operating system. All of this can be either confusing or too complicated for the programmer.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages39 Page
-
File Size-