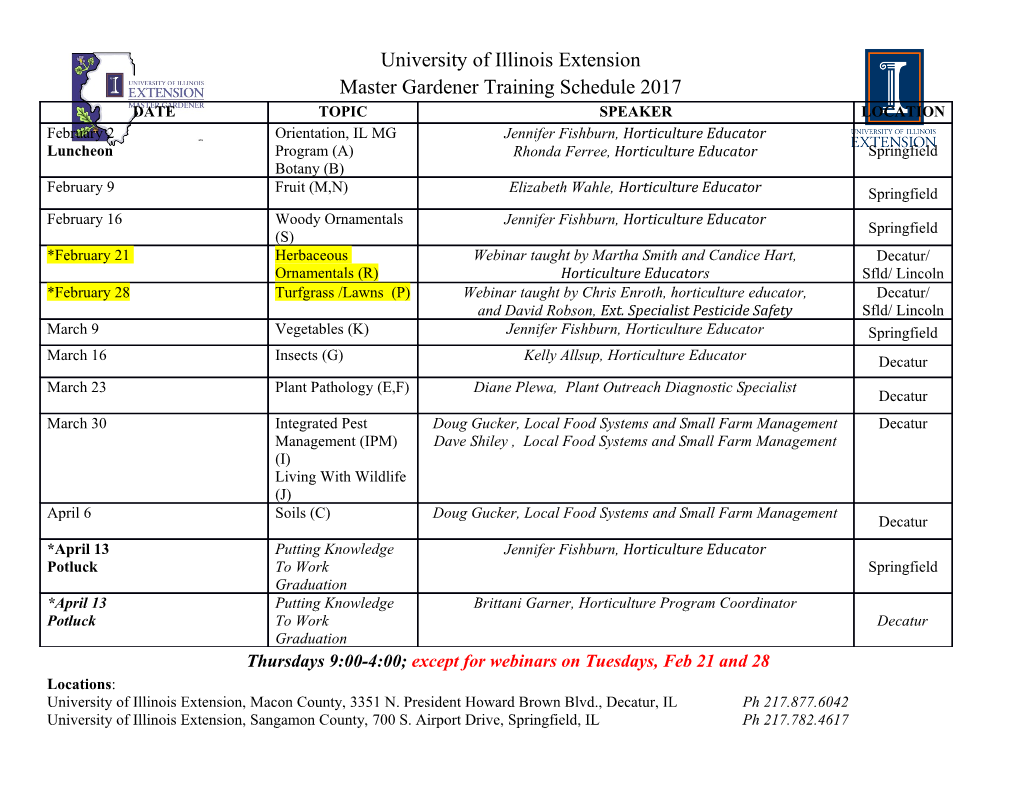
The OpenGL Shading Language Bill Licea-Kane ATI Research, Inc. 1 OpenGL Shading Language Today •Brief History • How we replace Fixed Function • OpenGL Programmer View • OpenGL Shaderwriter View • Examples 2 OpenGL Shading Language Today •Brief History • How we replace Fixed Function • OpenGL Programmer View • OpenGL Shaderwriter View • Examples 3 Brief History 1968 “As far as generating pictures from data is concerned, we feel the display processor should be a specialized device, capable only of generating pictures from read- only representations in core.” [Myer, Sutherland] On the Design of Display Processors. Communications of the ACM, Volume 11 Number 6, June, 1968 4 Brief History 1978 THE PROGRAMMING LANGUAGE [Kernighan, Ritchie] The C Programming Language 1978 5 Brief History 1984 “Shading is an important part of computer imagery, but shaders have been based on fixed models to which all surfaces must conform. As computer imagery becomes more sophisticated, surfaces have more complex shading characteristics and thus require a less rigid shading model." [Cook] Shade Trees SIGGRAPH 1984 6 Brief History 1985 “We introduce the concept of a Pixel Stream Editor. This forms the basis for an interactive synthesizer for designing highly realistic Computer Generated Imagery. The designer works in an interactive Very High Level programming environment which provides a very fast concept/implement/view iteration cycle." [Perlin] An Image Synthesizer SIGGRAPH 1985 7 Brief History 1990 “A shading language provides a means to extend the shading and lighting formulae used by a rendering system." … "…because it is based on a simple subset of C, it is easy to parse and implement, but, because it has many high-level features that customize it for shading and lighting calulations, it is easy to use." [Hanrahan, Lawson] A Language for Shading and Lighting Calculations SIGGRAPH 1990 8 Brief History June 30, 1992 “This document describes the OpenGL graphics system: what it is, how it acts, and what is required to implement it.” “OpenGL does not provide a programming language. … Programmability would conflict with keeping the API close to the hardware and thus with the goal of maximum performance.” [Segal, Akeley] The OpenGL Graphics System: A Specification (V 1.0) June 30, 1992 [Segal, Akeley] The Design of the OpenGL Graphics Interface, 1994 9 Brief History August 23, 1993 Digital Equipment will begin shipping its first OpenGL product next Monday as part of the Open3D 2.0 layered product for Alpha AXP OSF/1. ... -- John Dennis – OpenGL Project Lead [Dennis] comp.graphics.opengl 1993-08-19 10 Brief History October 1993 [email protected] (William C. Archibald) writes: |> 1)Quite a few modern rendering techniques and renderers |> support shader function calls at arbitrary points |> across a surface being rendered. ... What I would |> _like_ to do is call the shader at visible |> (front-facing) points. Is there _any_ way to do |> this kind of thing in OpenGL? Not with the current API. One could extend OpenGL in this manner... [Akeley] comp.graphics.opengl 1993-10-13 11 Brief History Recent • August 2001, SIGGRAPH OpenGL BOF – 3DLabs calls for "programmable" OpenGL • June 2002, OpenGL ARB – ATI chairs arb-gl2 workgroup 12 Brief History Recent • June 2003, OpenGL ARB – ARB approves OpenGL Shading Language • March 2004, OpenGL ARB – Unanimous roadmap to core OpenGL 2.0 13 OpenGL Shading Language Today •Brief History • How we replace Fixed Function • OpenGL Programmer View • OpenGL Shaderwriter View • Examples 14 [Kempf, Frazier] OpenGL Reference Manual (2nd Edition) www.opengl.org 15 OpenGL Fixed Function Vertex Vertex (object) Vertex (clip) Transform -T Normal [MVP],[MV],[MV] Vertex (eye) Color [0,1] FrontColor SecondaryColor Lighting BackColor FrontSecondaryColor [0,1] BackSecondaryColor Texgen Texture TexCoordn Matrixn TexCoordn EdgeFlag EdgeFlag 16 OpenGL Vertex Shader Attribute0 Uniform Texture Position Attribute1 ClipVertex Attribute2 PointSize … Vertex Varying0 Shader Attributen Varying1 Varying2 … Temporaries Varyingn EdgeFlag EdgeFlag 17 Post Vertex Shader "After lighting" Clamp Position Position ClipVertex ClipVertex PointSize PointSize FrontColor [0,1] FrontColor BackColor BackColor FrontSecondaryColor [0,1] FrontSecondaryColor BackSecondaryColor BackSecondaryColor Varyingn Varyingn EdgeFlag EdgeFlag 18 OpenGL Fixed Function Rasterization Position Primitive Assembly ClipVertex Flatshade Varyingn Culling or EdgeFlag Clipping Point Line Face Polygon Processing Rasterization RasterPos Varyingn Pixel Rectangle Bitmap Varyingn Rasterization Coord 19 OpenGL Fixed Function Fragment Color SecondaryColor Tex TE Sum Fog TexCoord[n] Color n n [0,1] z(z |ze|,f ) Depth Depth Coord Coord FrontFacing FrontFacing 20 OpenGL Fragment Shader Varying0 Uniform Texture Varying1 Varying2 … Fragment FragColor Shader Varyingn FragData[n] FragDepth FragDepth FragCoord Temporaries FragCoord FrontFacing FrontFacing 21 OpenGL Shading Language Today •Brief History • How we replace Fixed Function • OpenGL Programmer View • OpenGL Shaderwriter View • Examples 22 Simple Program helloshader int main ( int argc, char **argv ) { GLhandleARB VShader, FShader, PObject; GLint VStatus, VStatus, PStatus; GLint myColorLocation; GLfloat myColor[4] = { 0.0, 0.5, 1.0, 1.0 }; GLcharARB *VSource; GLcharARB *FSource[2]; VSource = "void main( void ){ gl_Position = ftransform(); }" FSource[0] = "uniform vec4 myColor;" FSource[1] = "void main( void ){ gl_FragColor = myColor; }" // Initialize OpenGL context, etc... 23 Simple Program helloshader // Create objects VShader = glCreateShaderObjectARB( GL_VERTEX_SHADER_ARB ); FShader = glCreateShaderObjectARB( GL_FRAGMENT_SHADER_ARB ); PObject = glCreateProgramOjbectARB(); // Attach shader objects to program (even empty objects) glAttachObjectARB( PObject, VShader ); glAttachObjectARB( PObject, FShader ); 24 Simple Program helloshader // Load source glShaderSourceARB( VShader, 1, VSource, NULL ); glShaderSourceARB( FShader, 2, FSource, NULL ); // And compile glCompileShaderARB( VShader ); glCompileShaderARB( FShader ); // Check compile status glGetObjectParameterARB( VShader, &VStatus ); glGetObjectParameterARB( FShader, &FStatus ); if ( !VStatus || !FStatus ) return 0; 25 Simple Program helloshader glLinkProgramARB( PObject ); glGetObjectParameterARB( PObject, &PStatus ); if ( !PStatus ) return 0; myColorLocation = glGetUniformLocation( PObject, "myColor" ); glUseProgramObjectARB( PObject ); if ( myColorLocation != -1 ) // existing behavior INVALID_OPERATION glUniform4fvARB( myColorLocation, myColor ); // Draw Stuff } 26 OpenGL Shading Language Today •Brief History • How we replace Fixed Function • OpenGL Programmer View • OpenGL Shaderwriter View • Examples 27 OpenGL Shading Language Preprocessor directives # #error #define #pragma optimize(on|off) defined() #pragma debug(on|off) #undef #if #line line file #ifdef __LINE__ #ifndef __FILE__ #else __VERSION__ #elif // comment #endif /* comment */ 28 OpenGL Shading Language Types • void • float vec2 vec3 vec4 • mat2 mat3 mat4 • int ivec2 ivec3 ivec4 • bool bvec2 bvec3 bvec4 29 OpenGL Shading Language Reserved Types • double dvec2 dvec3 dvec4 • half hvec2 hvec3 hvec4 • fixed fvec2 fvec3 fvec4 30 OpenGL Shading Language Sampler Types • samplernD • samplerCube • samplernDShadow 31 OpenGL Shading Language Reserved Sampler Types • samplernDRect • samplernDRect__EXT • samplernDRectShadow • samplernDRectShadow__EXT 32 OpenGL Shading Language struct Types • some minor restrictions – no qualifiers – no bit fields – no forward references – no in-place definitions – no anonymous structures 33 OpenGL Shading Language array Types • some minor restrictions – one dimensional – size is integral constant expression – can declare unsized array, but… – specificy size and type of array ONCE – any basic type and struct – no initialization at declaration 34 OpenGL Shading Language Scope of Types • global – Outside function – Shared globals must be same type • local (nested) – within function definition – within function 35 OpenGL Shading Language type qualifiers •const • attribute • uniform • varying • in out • default 36 OpenGL Shading Language operators • grouping: () • array subscript: [] • function call and constructor: () • field selector and swizzle: . • postfix: ++ -- • prefix: ++ -- + - ! 37 OpenGL Shading Language operators • binary: * / + - • relational: < <= > >= • equality: == != • logical: && ^^ || • selection: ?: • assignment: = *= /= += -= 38 OpenGL Shading Language reserved operators • prefix: ~ • binary: % • bitwise: << >> & ^ | • assignment: %= <<= >>= &= ^= |= 39 OpenGL Shading Language constructors • float( ) • int( ) • bool( ) • vec2( ) vec3( ) vec4( ) • mat2( ) mat3( ) mat4 ( ) 40 OpenGL Shading Language scalar constructors float f; int i; bool b; float( b) // b=true?1.0:0.0; int( b) // b=true?1:0; bool( b) // identity float( i) // int to float int( i) // identity bool( i) // i!=0?true:false; float( f) // identity int( f) // float to int bool( f) // f!=0.0?true:false; 41 OpenGL Shading Language vector constructors vec2 v2; vec3 v3; vec4 v4; vec2( 1.0 ,2.0) vec3( 0.0 ,0.0 ,1.0) vec4( 1.0 ,0.5 ,0.0 ,1.0) vec4( 1.0) // all 1.0 vec4( v2 ,v2) // different... vec4( v3 ,1) // ...types vec2( v4) float( v4) 42 OpenGL Shading Language matrix constructors vec4 v4; mat4 m4; mat4( 1.0, 2.0, 3.0, 4.0, // column major 5.0, 6.0, 7.0, 8.0, 9.0, 10., 11., 12., 13., 14., 15., 16.) mat4( v4, v4, v4, v4) mat4( 1.0) // identity matrix mat3( m4) // NOT! upper 3x3 vec4( m4) // upper 4x1 float( m4) // upper
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages130 Page
-
File Size-