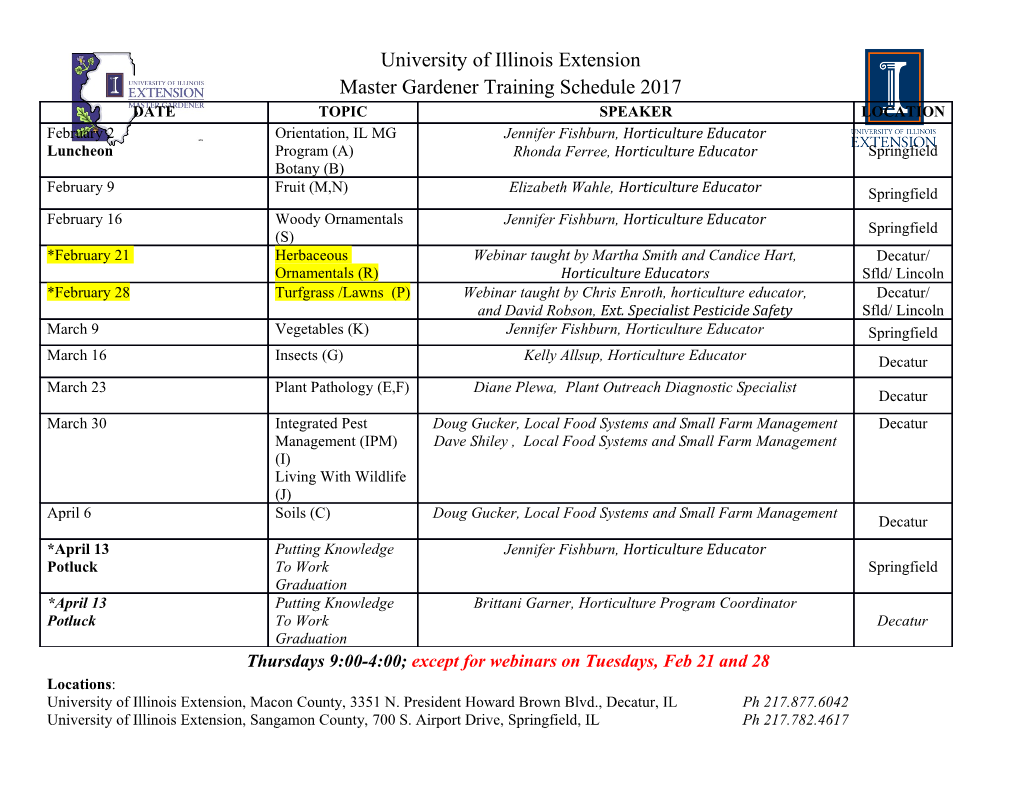
ZHAW/HSR Print date: 04.02.19 TSM_Alg & FTP_Optimiz COMBINATORIAL PROBLEMS - P-CLASS Graph Search Given: 퐺 = (푉, 퐸), start node Goal: Search in a graph DFS 1. Start at a, put it on stack. Insert from g h Depth-First- Stack = LIFO "Last In - First Out" top ↓ e e e e e Search 2. Whenever there is an unmarked neighbour, Access c f f f f f f f go there and and put it on stack from top ↓ d d d d d d d d d d d 3. If there is no unmarked neighbour, backtrack; b b b b b b b b b b b b b i.e. remove current node from stack (grey ⇒ green) and a a a a a a a a a a a a a a a go to step 2. BFS 1. Start at a, put it in queue. Insert from top ↓ h g Breadth-First Queue = FIFO "First In - First Out" f h g Search 2. Output first vertex from queue (grey ⇒ green). Mark d e c f h g all neighbors and put them in queue (white ⇒ grey). Do Access from bottom ↑ a b d e c f h g so until queue is empty Minimum Spanning Tree (MST) Given: Graph 퐺 = (푉, 퐸, 푊) with undirected edges set 퐸, with positive weights 푊 Goal: Find a set of edges that connects all vertices of G and has minimum total weight. Application: Network design (water pipes, electricity cables, chip design) Algorithm: Kruskal's, Prim's, Optimistic, Pessimistic Optimistic Approach Successively build the cheapest connection available =Kruskal's algorithm that is not redundant. 1956 Sort edges of G by increasing weight (Greedy) Set 퐸푇 to ∅ ab dh fh ef df be eh cd eg bd ad total For 푘 = 1. 푛푒, do: 1 2 3 4 5 6 7 8 9 10 11 33 푂(|퐸| + log|퐸|) If 퐸푇 ∪ {푒푘} has no cycle with union-find- Set 퐸푇 = 퐸푇 ∪ {푒푘} datastructure Return 푇 = (푉, 퐸푇) Pessimistic Successively rule out the most expensive line that is ad bd eg cd eh be df ef fh dh ab total Approach not absolutely needed. 11 10 9 8 7 6 5 4 3 2 1 33 Prim's Algorithm Choose an arbitrary start vertex 푣 and set 푀 = {푣 }. 0 0 a b e f h d c g total 1957 Iteratively add to M a vertex in 푉 \ 푀 that can be a-b b-e e-f f-h h-d d-c e-g (Greedy) reached the cheapest from the current set M. Select 1 6 4 3 2 8 9 33 the corresponding edge. Continue until 푀 = 푉. 푂(|퐸| + |푉| log|푉|) For each vertex 푢 ∈ 푉 do: if L is managed with 휆[푢] = ∞ a Brodal queue 푝[푢] = emptyset Choose a vertex 푠 and set 휆[푠] ≔ 0 퐸푇 = emptyset 퐿 = 푉 // List of vertices not yet in T While 퐿 ≠ ∅ do Remove from L the vertex u with lower 휆[푢] If 푢 ≠ 푠 then 퐸푇 ≔ 퐸푇 ∪ {푝[푢], 푢} For each vertex 푣 ∈ 푎푑푗[푢] do If 푣 ∈ 퐿 and 휆[푣] > 푐푢푣 then 휆[푣] = 푐푢푣 푝[푣] = 푢 Return 푇 = (푉, 퐸푇) Marcel Meschenmoser Lecturer: Prof. Dr. Mark Cieliebak & Prof. Dr. Lin Himmelmann Page 1 of 13 ZHAW/HSR Print date: 04.02.19 TSM_Alg & FTP_Optimiz Shortest paths Given: Graph 퐺 = (푉, 퐸, 퐶) with cost 푐푖푗 ≥ 0 for each edge 푒 ∈ 퐸 and a start vertex 푠 ∈ 푉 Goal 1: Find the shortest path from start 푠 to 푗. Goal 2: Find the shortest path from start 푠 to all other vertices. Goal 3: Find the shortest path between all pairs of vertices. Algorithm: (Goal 1+2) Dijkstra's, (Goal 3) Floyd-Warshall Dijkstra's algorithm We iteratively compute the shortest distance 퐼(푣) for a b=3 d=8 c=14 c=14 c=14 c=14 g=15 1959 the vertex v closest to 푣0 that has not been reached yet. d=9 e=10 e=10 f=14 f=13 g=15 Not working with negative weights. f=14 h=11 g=15 퐺 = (푉, 퐸, 퐶) 휆푗: length of the shortest path h=16 g=15 푝푗: predecessor of j on the shortest path 푂(|퐸| + |푉| log|푉|) 푠: start vertex if L is managed with For all 푗 ∈ 푉 do 휆푗 = ∞; 푝푗 = ∅ a Brodal queue 휆푠 = 0; 퐿 = 푉 While 퐿 ≠ ∅ Find i such that 휆푖 = min(휆푘|푘 ∈ 퐿) 퐿 = 퐿 표ℎ푛푒 {푖} For all 푗 ∈ 푠푢푐푐[푖] do If 푗 ∈ 퐿 and 휆푗 > 휆푖 + 푐푖푗 휆푗 = 휆푖 + 푐푖푗; 푝푗 = 푖 Return Lenghts 휆 and predecessors p Floyd-Warshall 푚푖푛푃푎푡ℎ(푖, 푗, 푛) are the shortest distances from 푣푖 to 푖 = 1 algorithm 푣푗, ∞ if not existing. 푎 푏 푐 푑 푒 푓 푔 ℎ Iterate through all rows/columns: 푎 − 3 ∞ 9 → 8 ∞ ∞ ∞ ∞ relies on dynamic 푚푖푛푃푎푡ℎ(푖, 푗, 푘) 푏 3 − ∞ 5 7 ∞ ∞ ∞ programming 푚푖푛푃푎푡ℎ(푖, 푗, 푘 + 1) = min {푚푖푛푃푎푡ℎ(푖, 푘 + 1, 푘) + 푐 ∞ ∞ − 6 ∞ ∞ ∞ ∞ 푚푖푛푃푎푡ℎ(푘 + 1, 푗, 푘) 푑 9 → 8 5 6 − ∞ → 12 6 ∞ 8 use recursion 푒 ∞ 7 ∞ ∞ → 12 − 6 5 1 푓 ∞ ∞ ∞ 6 6 − ∞ 2 negative weighs 푔 ∞ ∞ ∞ ∞ 5 ∞ − ∞ allowed as long as ℎ ∞ ∞ ∞ 8 1 2 ∞ − no negative cycles Var: Pathfinding Given: start and goal coordinates Problem: We only see the immediate neighborhood of our position. Marcel Meschenmoser Lecturer: Prof. Dr. Mark Cieliebak & Prof. Dr. Lin Himmelmann Page 2 of 13 ZHAW/HSR Print date: 04.02.19 TSM_Alg & FTP_Optimiz Max-Flow Input: directed graph 퐺 = (푉, 퐸) with capacity 푐(푒) > 0 Two special nodes, source s, and ,sink/target t, are given, 푠 ≠ 푡 Goal: Maximize the total amount of flow from s to t, where the flow on edge e doesn't exceed 푐(푒) and for every node 푣 ≠ 푠 푎푛푑 푣 ≠ 푡, incoming flow is equal to outgoing flow. Applications: Network routing, Transportation, Bipartite Match Algorithm: Ford-Fulkerson-Alg, Edmonds-Karp Greedily finding s-b-e-t s-b-d-t s-d-f-t total augmenting 1 8 3 12 paths s-b: 9 → 8 s-b: 8 → 0 s-d: 9 → 6 b-e: 5 → 4 b-d: 9 → 1 d-f: 3 → 0 e-t: 1 → 0 d-t: 8 → 0 f-t: 4 → 1 Ford-Fulkerson Idea: Insert backwards edges that can be used to (partially) undo previous paths. path 1: Set 푓푡표푡푎푙 = 0 While there is a path from s to t in G: ∗ 푂((푛푣 + 푛푒) 푓 ) Determine smallest capacity g along the path P 푓∗: optimal flow Add f to 푓푡표푡푎푙 Foreach edge 푢 → 푣 on the path decrease 푐(푢 → 푣) by f increase 푐(푣 → 푢) by f (backward) delete edge if capacity 푐 = 0 s-b-e-t s-b-d-t s-d-f-t s-d-b-e-f-t path 2: 1 8 3 1 Problem: Inefficient behaviour if the augmenting path is chosen arbitrarily. Solution: Edmonds-Karp algorithm Edmonds-Karp Idea: In each iteration, use a shortest augmenting path, i.e. a path from s to t with algorithm the fewest number of edges. Can be found with BFS in time 푂(|퐸|). Insert from top ↓ t=2 starting at s d=1 e=2 f=2 Access from bottom ↑ s=0 b=1 d=1 e=2 shortest augmenting path: s-d-t with vertex Restriction: Maximum flow through vertices is restricted. restrictions Solution: Add additional vertex before the restricted vertex and add new edge with weight = vertex restriction. Direct all ingoing edges to the new vertex. e.g. b by 3: Distribution Insert source s and connect it with capacity ∞ edges to first layer. problem reduced Insert sink t and connect it with capacity ∞ edges to last layer. to Max-Flow Bipartite Make edges directed. Add source and sink vertices s and t with corresponding matching edges. Set all edge weights to 1. Find integer maximum flow with Ford-Fulkerson algorithm. Marcel Meschenmoser Lecturer: Prof. Dr. Mark Cieliebak & Prof. Dr. Lin Himmelmann Page 3 of 13 ZHAW/HSR Print date: 04.02.19 TSM_Alg & FTP_Optimiz Var: Goal: Find a minimum partition of the vertex set which divides S and T Max-Flow into two sets. Min-Cut Applicationgs: Redundancy in networks, reliability in telecommunication, military strategy, optimizing traffic systems The maximum st flow equals the minimum value of all (exponentially many) possible st-cuts. Var: Each edge e has capacity 푐(푒) and cost 푐표푠푡(푒) Min-Cost Goal: Find the maximum flow that has the minimum total cost Max-Flow harder Often there are several possible maximum flows with a cost per flow unit. Solution: Extend Ford-Fulkerson algorithm to take cost criterion into account. s-b-d-t s-b-e-t s-c-e-t 2*6=16 1*5=5 1*4 capacity = 4 cost = 21 Until now, we just used the Ford-Fulkerson algorithm with additionally annotating the costs. Now, look for cycles, respecting the capacities. This does not change the total flow from s to t, but decreases the total cost. s-c-e-b-s capacity 1*(3-4) s-b-e → s-c-e capacity = 4 new cost = 20 Marcel Meschenmoser Lecturer: Prof. Dr. Mark Cieliebak & Prof. Dr. Lin Himmelmann Page 4 of 13 ZHAW/HSR Print date: 04.02.19 TSM_Alg & FTP_Optimiz COMBINATORIAL PROBLEMS - NP-CLASS TSP Input: 푛 cities, 푑푖푗 distances matrix between cities 푖 and 푗. Traveling Goal: Find the shortes tour passing exactly once in each city. Salesperson 푛−1 ∑ 푑푝푖푝푖+1 + 푑푝푛푝1 Problem 푖=1 Application: Network Design, pipes, cables, chip design, ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages13 Page
-
File Size-