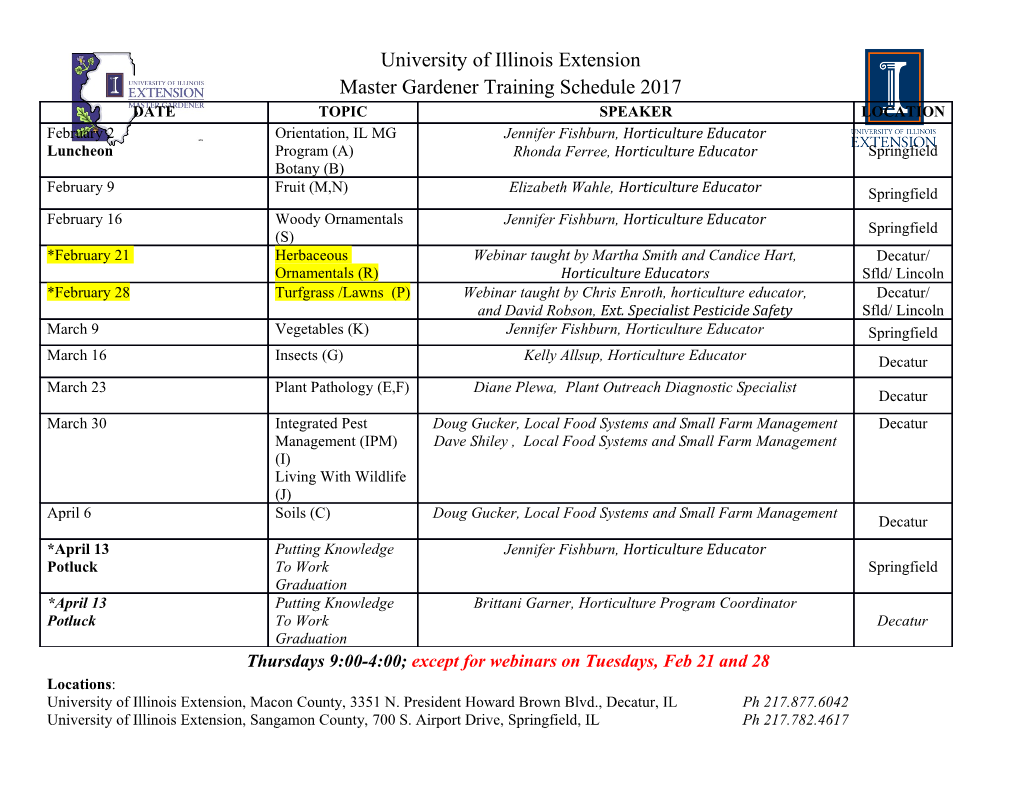
IBM XL C/C++ for Linux, V13.1.2 IBM Optimization and Programming Guide for Little Endian Distributions Version 13.1.2 SC27-6560-01 IBM XL C/C++ for Linux, V13.1.2 IBM Optimization and Programming Guide for Little Endian Distributions Version 13.1.2 SC27-6560-01 Note Before using this information and the product it supports, read the information in “Notices” on page 113. First edition This edition applies to IBM XL C/C++ for Linux, V13.1.2 (Program 5765-J08; 5725-C73) and to all subsequent releases and modifications until otherwise indicated in new editions. Make sure you are using the correct edition for the level of the product. © Copyright IBM Corporation 1996, 2015. US Government Users Restricted Rights – Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp. Contents About this document ......... v Chapter 6. The C++ template model .. 23 Who should read this document........ v How to use this document.......... v Chapter 7. Constructing a library ... 25 How this document is organized ....... v Compiling and linking a library ....... 25 Conventions .............. vi Compiling a static library......... 25 Related information ............ ix Compiling a shared library ........ 25 IBM XL C/C++ information ........ ix Linking a library to an application...... 25 Standards and specifications ........ x Linking a shared library to another shared library 26 Other IBM information.......... xi Initializing static objects in libraries (C++) .... 26 Other information ........... xi Assigning priorities to objects ....... 26 Technical support ............ xi Order of object initialization across libraries .. 27 How to send your comments ........ xi Chapter 8. Optimizing your applications 31 Chapter 1. Porting from 32-bit to 64-bit Distinguishing between optimization and tuning .. 31 mode ................ 1 Steps in the optimization process ....... 32 Assigning long values ........... 1 Basic optimization ............ 32 Assigning constant values to long variables ... 2 Optimizing at level 0 .......... 32 Bit-shifting long values .......... 3 Optimizing at level 2 .......... 33 Assigning pointers ............ 3 Advanced optimization .......... 34 Aligning aggregate data .......... 4 Optimizing at level 3 .......... 35 Calling Fortran code ............ 4 An intermediate step: adding -qhot suboptions at level 3 ............... 36 Chapter 2. Using XL C/C++ with Fortran 5 Optimizing at level 4 .......... 36 Identifiers ............... 5 Optimizing at level 5 .......... 37 Corresponding data types .......... 6 Tuning for your system architecture ...... 38 Character and aggregate data ......... 8 Getting the most out of target machine options 38 Function calls and parameter passing ...... 8 Using high-order loop analysis and transformations 39 Pointers to functions............ 9 Getting the most out of -qhot ....... 40 Sample program: C/C++ calling Fortran ..... 9 Using shared-memory parallelism (SMP) .... 41 Getting the most out of -qsmp ....... 42 Chapter 3. Aligning data ....... 11 Using interprocedural analysis ........ 43 Getting the most from -qipa ........ 44 Using alignment modes .......... 11 Using profile-directed feedback ........ 45 Alignment of aggregates ......... 12 Viewing profiling information with showpdf .. 48 Alignment of bit-fields.......... 13 Object level profile-directed feedback ..... 51 Using alignment modifiers ......... 14 Marking variables as local or imported ..... 53 Getting the most out of -qdatalocal ..... 53 Chapter 4. Handling floating-point Using compiler reports to diagnose optimization operations ............. 17 opportunities .............. 55 Floating-point formats ........... 17 Parsing compiler reports with development tools 56 Handling multiply-add operations....... 17 Other optimization options ......... 56 Compiling for strict IEEE conformance ..... 18 Handling floating-point constant folding and Chapter 9. Debugging optimized code 59 rounding ............... 18 Understanding different results in optimized Matching compile-time and runtime rounding programs ............... 59 modes ............... 19 Debugging in the presence of optimization .... 60 Handling floating-point exceptions ...... 20 Chapter 10. Coding your application to Chapter 5. Using C++ constructors .. 21 improve performance ........ 63 Using delegating constructors (C++11) ..... 21 Finding faster input/output techniques ..... 63 Reducing function-call overhead ....... 63 Using template explicit instantiation declarations (C++11)................ 65 Managing memory efficiently (C++ only) .... 65 © Copyright IBM Corp. 1996, 2015 iii Optimizing variables ........... 66 BLAS function syntax .......... 97 Manipulating strings efficiently ........ 66 Linking the libxlopt library ........ 99 Optimizing expressions and program logic .... 67 Optimizing operations in 64-bit mode ..... 68 Chapter 12. Parallelizing your Using rvalue references (C++11) ....... 68 programs ............. 101 Using visibility attributes (IBM extension) .... 70 Countable loops ............ 101 Types of visibility attributes ........ 71 Enabling automatic parallelization ...... 103 Rules of visibility attributes ........ 73 Data sharing attribute rules......... 103 Propagation rules (C++ only) ....... 78 Using OpenMP directives ......... 105 Specifying visibility attributes using the Shared and private variables in a parallel -fvisibility option .......... 81 environment.............. 106 Specifying visibility attributes using pragma Reduction operations in parallelized loops.... 107 preprocessor directives ......... 81 Chapter 13. Vector element order Chapter 11. Using the high toggling.............. 109 performance libraries ........ 85 Program migration from big endian systems ... 112 Using the Mathematical Acceleration Subsystem libraries (MASS) ............. 85 Using the scalar library ......... 86 Notices .............. 113 Using the vector libraries ......... 88 Trademarks .............. 115 Using the SIMD libraries ......... 92 Compiling and linking a program with MASS .. 95 Index ............... 117 Using the Basic Linear Algebra Subprograms – BLAS ................ 96 iv XL C/C++: Optimization and Programming Guide for Little Endian Distributions About this document This guide discusses advanced topics related to the use of IBM® XL C/C++ for Linux, V13.1.2, with a particular focus on program portability and optimization. The guide provides both reference information and practical tips for getting the most out of the compiler's capabilities through recommended programming practices and compilation procedures. Who should read this document This document is addressed to programmers building complex applications, who already have experience compiling with XL C/C++ and would like to take further advantage of the compiler's capabilities for program optimization and tuning, support for advanced programming language features, and add-on tools and utilities. How to use this document This document uses a task-oriented approach to present the topics by concentrating on a specific programming or compilation problem in each section. Each topic contains extensive cross-references to the relevant sections of the reference guides in the IBM XL C/C++ for Linux, V13.1.2 documentation set, which provides detailed descriptions of compiler options and pragmas, and specific language extensions. How this document is organized This guide includes the following chapters: v Chapter 1, “Porting from 32-bit to 64-bit mode,” on page 1 discusses common problems that arise when you port existing 32-bit applications to 64-bit mode, and it provides recommendations for avoiding these problems. v Chapter 2, “Using XL C/C++ with Fortran,” on page 5 discusses considerations for calling Fortran code from XL C/C++ programs. v Chapter 3, “Aligning data,” on page 11 discusses options available for controlling the alignment of data in aggregates, such as structures and classes. v Chapter 4, “Handling floating-point operations,” on page 17 discusses options available for controlling the way floating-point operations are handled by the compiler. v Chapter 5, “Using C++ constructors,” on page 21 discusses delegating constructors that can concentrate common initializations in one constructor. v Chapter 6, “The C++ template model,” on page 23 discusses options for compiling programs that include C++ templates. v Chapter 7, “Constructing a library,” on page 25 discusses how to compile and link static and shared libraries and how to specify the initialization order of static objects in C++ programs. v Chapter 8, “Optimizing your applications,” on page 31 discusses various options provided by the compiler for optimizing your programs, and it provides recommendations for use of these options. © Copyright IBM Corp. 1996, 2015 v v Chapter 9, “Debugging optimized code,” on page 59 discusses the potential usability problems of optimized programs and the options that can be used to debug the optimized code. v Chapter 10, “Coding your application to improve performance,” on page 63 discusses recommended programming practices and coding techniques for enhancing program performance and compatibility with the compiler's optimization capabilities. v Chapter 11, “Using the high performance libraries,” on page 85 discusses two performance libraries that are shipped with XL C/C++: the Mathematical Acceleration Subsystem (MASS), which contains tuned versions of standard math library functions, and the Basic Linear Algebra Subprograms (BLAS), which contains basic functions for matrix
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages134 Page
-
File Size-