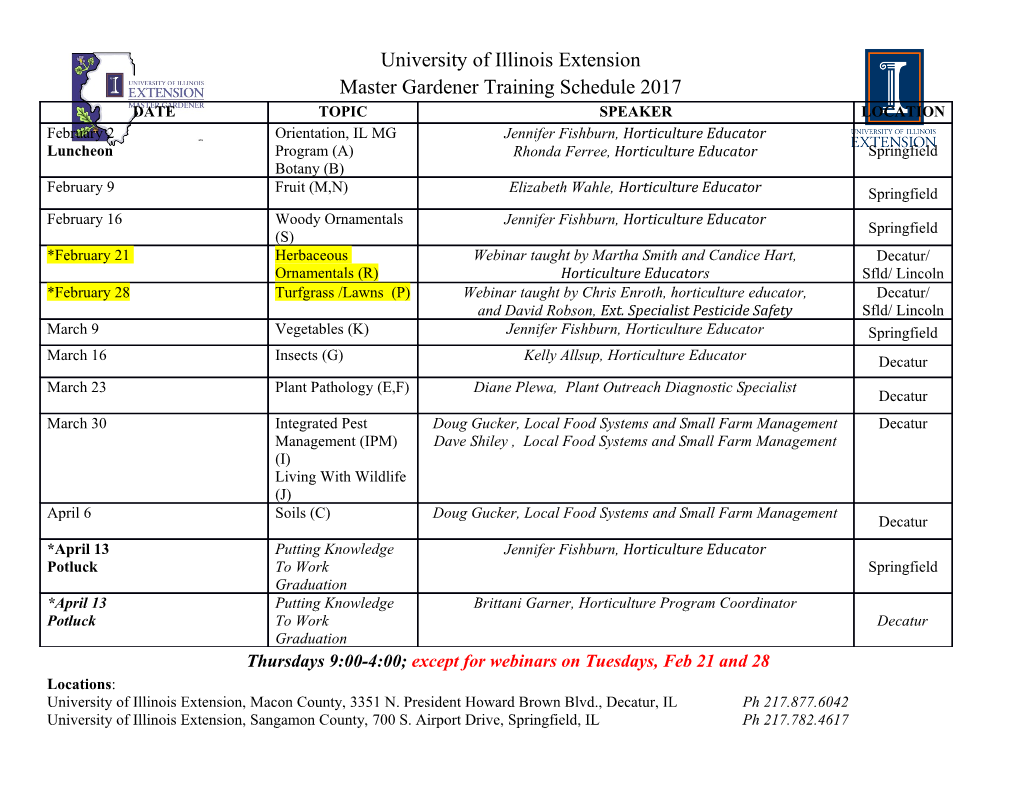
University of British Columbia Reading for Texture Mapping Rendering Pipeline CPSC 314 Computer Graphics Jan-Apr 2013 • FCG Chap 11 Texture Mapping • except 11.7 (except 11.8, 2nd ed) Geometry Processing Geometry Model/View Perspective Tamara Munzner Lighting Clipping • RB Chap Texture Mapping Database Transform. Transform. Texturing Scan Depth Frame- Texturing Blending Textures Conversion Test buffer Rasterization Fragment Processing http://www.ugrad.cs.ubc.ca/~cs314/Vjan2013 2 3 4 Texture Mapping Texture Mapping Color Texture Mapping Texture Coordinates • real life objects have • introduced to increase realism • define color (RGB) for each point on object • texture image: 2D array of color values (texels) nonuniform colors, • lighting/shading models not enough surface • assigning texture coordinates (s,t) at vertex with normals • hide geometric simplicity • two approaches object coordinates (x,y,z,w) • to generate realistic • images convey illusion of geometry • use interpolated (s,t) for texel lookup at each pixel objects, reproduce • surface texture map • map a brick wall texture on a flat polygon • use value to modify a polygon’s color coloring & normal • volumetric texture • or other surface property • create bumpy effect on surface variations = texture • specified by programmer or artist • associate 2D information with 3D surface glTexCoord2f(s,t) • can often replace glVertexf(x,y,z,w) • point on surface corresponds to a point in complex geometric details texture • “paint” image onto polygon 5 6 7 8 Texture Mapping Example Example Texture Map Fractional Texture Coordinates Texture Lookup: Tiling and Clamping texture • what if s or t is outside the interval [0…1]? image + = • multiple choices glTexCoord2d(1,1); • use fractional part of texture coordinates (0,1) (1,1) (0,.5) (.25,.5) glVertex3d (0, 2, 2); • cyclic repetition of texture to tile whole surface glTexParameteri( …, GL_TEXTURE_WRAP_S, GL_REPEAT, GL_TEXTURE_WRAP_T, GL_REPEAT, ... ) • clamp every component to range [0…1] glTexCoord2d(0,0); (0,0) (1,0) (0,0) (.25,0) • re-use color values from texture image border glVertex3d (0, -2, -2); glTexParameteri( …, GL_TEXTURE_WRAP_S, GL_CLAMP, GL_TEXTURE_WRAP_T, GL_CLAMP, ... ) 9 10 11 12 Tiled Texture Map Demo Texture Coordinate Transformation Texture Functions • once have value from the texture map, can: (1,0) (1,1) • Nate Robbins tutors • motivation • directly use as surface color: GL_REPLACE • texture • change scale, orientation of texture on an object • throw away old color, lose lighting effects glTexCoord2d(1, 1); • approach • modulate surface color: GL_MODULATE glVertex3d (x, y, z); • texture matrix stack • multiply old color by new value, keep lighting info • texturing happens after lighting, not relit (0,0) (0,1) • transforms specified (or generated) tex coords • use as surface color, modulate alpha: GL_DECAL glMatrixMode( GL_TEXTURE ); • like replace, but supports texture transparency glLoadIdentity(); • blend surface color with another: GL_BLEND (4,0) (4,4) glRotate(); • new value controls which of 2 colors to use … • indirection, new value not used directly for coloring glTexCoord2d(4, 4); • specify with glTexEnvi(GL_TEXTURE_ENV, • more flexible than changing (s,t) coordinates glVertex3d (x, y, z); GL_TEXTURE_ENV_MODE, <mode>) • [demo] • [demo] (0,0) (0,4) 13 14 15 16 Texture Pipeline Texture Objects and Binding Basic OpenGL Texturing Low-Level Details • texture object • create a texture object and fill it with texture data: • large range of functions for controlling layout of texture data (x, y, z) • an OpenGL data type that keeps textures resident in memory and • glGenTextures(num, &indices) to get identifiers for the objects • state how the data in your image is arranged provides identifiers to easily access them • glBindTexture(GL_TEXTURE_2D, identifier) to bind Object position • e.g.: glPixelStorei(GL_UNPACK_ALIGNMENT, 1) tells • provides efficiency gains over having to repeatedly load and reload a • following texture commands refer to the bound texture OpenGL not to skip bytes at the end of a row (-2.3, 7.1, 17.7) texture • glTexParameteri(GL_TEXTURE_2D, …, …) to specify • you must state how you want the texture to be put in memory: • you can prioritize textures to keep in memory parameters for use when applying the texture how many bits per “pixel”, which channels,… • glTexImage2D(GL_TEXTURE_2D, ….) to specify the texture data (s, t) (s’, t’) • OpenGL uses least recently used (LRU) if no priority is assigned Texel space Texel color (the image itself) • textures must be square and size a power of 2 Parameter space Transformed • texture binding • enable texturing: glEnable(GL_TEXTURE_2D) • common sizes are 32x32, 64x64, 256x256 parameter space (81, 74) (0.9,0.8,0.7) (0.32, 0.29) • which texture to use right now • state how the texture will be used: • smaller uses less memory, and there is a finite amount of (0.52, 0.49) • switch between preloaded textures • glTexEnvf(…) texture memory on graphics cards • specify texture coordinates for the polygon: • ok to use texture template sample code for project 4 • use glTexCoord2f(s,t) before each vertex: • http://nehe.gamedev.net/data/lessons/lesson.asp?lesson=09 Object color • glTexCoord2f(0,0); glVertex3f(x,y,z); Final color (0.5,0.5,0.5) (0.45,0.4,0.35) 17 18 19 20 Texture Mapping Texture Mapping Interpolation: Screen vs. World Space Texture Coordinate Interpolation • perspective correct interpolation • texture coordinates • texture coordinate interpolation • screen space interpolation incorrect • α, β, γ : • specified at vertices • perspective foreshortening problem • problem ignored with shading, but artifacts • barycentric coordinates of a point P in a triangle glTexCoord2f(s,t); more visible with texturing P0(x,y,z) • s0, s1, s2 : glVertexf(x,y,z); • texture coordinates of vertices V (x’,y’) • interpolated across triangle (like R,G,B,Z) 0 • w0, w1,w2 : • …well not quite! • homogeneous coordinates of vertices (s1,t1) (x1,y1,z1,w1) (s,t)? (s2,t2) (α,β,γ) ’ ’ α ⋅ s0 / w0 + β ⋅ s1 / w1 + γ ⋅ s2 / w2 V1(x ,y ) s = (x2,y2,z2,w2) (s0,t0) α / w0 + β / w1 + γ / w2 (x0,y0,z0,w0) 21 22 P1(x,y,z) 23 24 Reconstruction Reconstruction MIPmapping MIPmaps • multum in parvo -- many things in a small place • how to deal with: use “image pyramid” to precompute • prespecify a series of prefiltered texture maps of decreasing • pixels that are much larger than texels? averaged versions of the texture resolutions • apply filtering, “averaging” • requires more texture storage • avoid shimmering and flashing as objects move • gluBuild2DMipmaps • automatically constructs a family of textures from original texture size down to 1x1 • pixels that are much smaller than texels ? Without MIP-mapping • interpolate without with store whole pyramid in single block of memory (image courtesy of Kiriakos Kutulakos, U Rochester) 25 26 With MIP-mapping27 28 MIPmap storage Texture Parameters Bump Mapping: Normals As Texture Bump Mapping • only 1/3 more space required • in addition to color can control other material/ • object surface often not smooth – to recreate correctly object properties need complex geometry model • can control shape “effect” by locally perturbing surface • surface normal (bump mapping) normal • reflected color (environment mapping) • random perturbation • directional change over region 29 30 31 32 Bump Mapping Embossing Displacement Mapping Environment Mapping • at transitions • bump mapping gets • cheap way to achieve reflective effect silhouettes wrong • rotate point’s surface normal by θ or - θ • generate image of surrounding • shadows wrong too • map to object as texture • change surface geometry instead • only recently available with realtime graphics • need to subdivide surface 33 34 35 36 Environment Mapping Sphere Mapping Cube Mapping Cube Mapping F • used to model object that reflects • texture is distorted fish-eye view • 6 planar textures, sides of cube surrounding textures to the eye • point camera at mirrored sphere • spherical texture mapping creates texture coordinates that • point camera in 6 different directions, facing • movie example: cyborg in Terminator 2 correctly index into this texture map out from origin • different approaches • sphere, cube most popular A • OpenGL support C • GL_SPHERE_MAP, GL_CUBE_MAP B • others possible too E D 37 38 39 40 Cube Mapping Volumetric Texture Volumetric Bump Mapping Volumetric Texture Principles • define texture pattern over 3D • direction of reflection vector r selects the face of the cube to • 3D function (x,y,z) be indexed domain - 3D space containing Marble ρ • co-ordinate with largest magnitude the object • texture space – 3D space that holds the • e.g., the vector (-0.2, 0.5, -0.84) selects the –Z face • texture function can be texture (discrete or continuous) digitized or procedural • remaining two coordinates (normalized by the 3rd coordinate) • for each point on object • rendering: for each rendered point P(x,y,z) selects the pixel from the face. compute texture from point compute ρ(x,y,z) • e.g., (-0.2, 0.5) gets mapped to (0.38, 0.80). location in space • volumetric texture mapping function/space • common for natural material/ • difficulty in interpolating across faces transformed with objects irregular textures (stone, Bump wood,etc…) 41 42 43 44 Procedural Textures Procedural Texture Effects: Bombing Procedural Texture Effects • generate “image” on the fly, instead of • randomly drop bombs of various shapes, sizes and • simple marble loading from disk orientation into texture space (store data in
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-